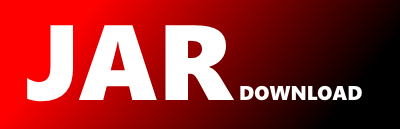
com.sdl.dxa.caching.DefaultNamedCacheProvider Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dxa-tridion-common Show documentation
Show all versions of dxa-tridion-common Show documentation
Tridion Common contains common code for Tridion for other DXA artifacts expect model-specific (like DD4T)
The newest version!
package com.sdl.dxa.caching;
import com.sdl.web.client.cache.CacheProviderInitializer;
import com.sdl.web.client.cache.GeneralCacheProvider;
import com.sdl.web.client.configuration.ClientConstants;
import com.sdl.web.client.configuration.api.ConfigurationException;
import com.sdl.web.content.client.configuration.impl.BaseClientConfigurationLoader;
import com.tridion.util.ReflectionUtil;
import lombok.Getter;
import lombok.extern.slf4j.Slf4j;
import org.apache.commons.lang3.tuple.Triple;
import org.ehcache.config.builders.CacheConfigurationBuilder;
import org.ehcache.config.builders.ResourcePoolsBuilder;
import org.ehcache.expiry.Duration;
import org.ehcache.xml.XmlConfiguration;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.stereotype.Component;
import jakarta.annotation.PostConstruct;
import javax.cache.Cache;
import javax.cache.CacheManager;
import javax.cache.Caching;
import javax.cache.spi.CachingProvider;
import java.io.IOException;
import java.net.MalformedURLException;
import java.net.URI;
import java.net.URISyntaxException;
import java.net.URL;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.Collection;
import java.util.Map;
import java.util.Properties;
import java.util.Set;
import java.util.concurrent.ConcurrentMap;
import java.util.concurrent.ConcurrentSkipListMap;
import java.util.concurrent.ConcurrentSkipListSet;
import java.util.concurrent.TimeUnit;
import static com.sdl.web.client.configuration.ClientConstants.Cache.DEFAULT_CACHE_URI;
import static java.nio.file.Files.exists;
import static org.ehcache.config.builders.CacheConfigurationBuilder.newCacheConfigurationBuilder;
import static org.ehcache.expiry.Duration.of;
import static org.ehcache.expiry.Expirations.timeToLiveExpiration;
import static org.ehcache.jsr107.Eh107Configuration.fromEhcacheCacheConfiguration;
/**
* Default implementation of DXA cache.
*
*/
@Slf4j
@Component
public class DefaultNamedCacheProvider extends BaseClientConfigurationLoader implements NamedCacheProvider {
private static final Logger LOG = LoggerFactory.getLogger(DefaultNamedCacheProvider.class);
@Value("#{'${dxa.caching.disabled.caches}'.split('[,\\s]')}")
private Set disabledCaches;
@Value("#{'${dxa.caching.required.caches}'.split('[,\\s]')}")
private Set requiredCaches;
@Value("${dxa.caching.configuration:#{null}}")
private String cachingConfigurationFile;
private boolean isCilConfigUsed;
@Getter
private CacheManager cacheManager;
private ConcurrentSkipListSet ownCachesNames = new ConcurrentSkipListSet<>();
private ConcurrentMap, Cache> ownCaches = new ConcurrentSkipListMap<>();
private com.sdl.web.client.cache.CacheProvider cilCacheProvider;
public DefaultNamedCacheProvider() throws ConfigurationException {
// empty
}
@PostConstruct
public void init() throws ConfigurationException {
cilCacheProvider = CacheProviderInitializer.getCacheProvider(getCacheConfiguration());
boolean cilUsesGeneralCache = cilCacheProvider instanceof GeneralCacheProvider;
isCilConfigUsed = cilUsesGeneralCache;
String cacheConfigurationUri = cilUsesGeneralCache ?
getCacheConfiguration().getProperty(ClientConstants.Cache.CLIENT_CACHE_URI) :
cachingConfigurationFile;
log.info("Using cache config {}, CIL uses GeneralCacheProvider: {}", cacheConfigurationUri, isCilConfigUsed);
cacheManager = getCacheManager(cacheConfigurationUri);
//cannot be null because of default value
//noinspection ConstantConditions
cacheManager.getCacheNames().forEach(requiredCaches::remove);
log.info("Required caches not yet created: '{}', creating them...", requiredCaches);
requiredCaches.forEach(this::getCache);
}
@Override
protected String getServiceUrl() {
return "";
}
@Override
public Cache getCache(String cacheName, Class keyType, Class valueType) {
log.debug("Trying to get cache name '{}' for key '{}' and value '{}'", cacheName, keyType, valueType);
Cache newCache = cacheManager.getCache(cacheName, keyType, valueType);
if (newCache == null) {
log.debug("Cache name '{}' for such key/value does not exist, auto-creating...", cacheName);
newCache = cacheManager.createCache(cacheName, buildDefaultCacheConfiguration(keyType, valueType));
}
if (ownCachesNames.add(cacheName)) {
Triple triple = Triple.of(cacheName, keyType, valueType);
Cache oldCache = ownCaches.put(triple, newCache);
if (oldCache == null) {
log.debug("Added cache '{}' to own caches of DXA", cacheName);
}
}
return newCache;
}
@Override
public Cache
© 2015 - 2025 Weber Informatics LLC | Privacy Policy