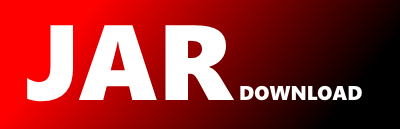
com.sdl.dxa.tridion.pcaclient.DXAGraphQLClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dxa-tridion-common Show documentation
Show all versions of dxa-tridion-common Show documentation
Tridion Common contains common code for Tridion for other DXA artifacts expect model-specific (like DD4T)
The newest version!
package com.sdl.dxa.tridion.pcaclient;
import com.sdl.dxa.caching.NamedCacheProvider;
import com.sdl.dxa.caching.statistics.CacheStatisticsProvider;
import com.sdl.web.content.client.util.ClientCacheKeyEnhancer;
import com.sdl.web.pca.client.DefaultGraphQLClient;
import com.sdl.web.pca.client.auth.Authentication;
import com.sdl.web.pca.client.exception.GraphQLClientException;
import com.sdl.web.pca.client.exception.UnauthorizedException;
import com.tridion.ambientdata.web.WebContext;
import org.slf4j.Logger;
import javax.cache.Cache;
import java.util.Map;
import java.util.UUID;
import java.util.concurrent.atomic.AtomicLong;
import static org.slf4j.LoggerFactory.getLogger;
public class DXAGraphQLClient extends DefaultGraphQLClient {
private static final Logger LOG = getLogger(DXAGraphQLClient.class);
private static final AtomicLong HIT_CACHE = new AtomicLong();
private static final AtomicLong MISS_CACHE = new AtomicLong();
private Cache
© 2015 - 2025 Weber Informatics LLC | Privacy Policy