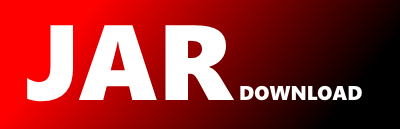
com.sdl.selenium.conditions.Condition Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of Testy Show documentation
Show all versions of Testy Show documentation
Automated Acceptance Testing. Selenium and Selenium WebDriver test framework for web applications.
(optimized for dynamic html, ExtJS, Bootstrap, complex UI, simple web applications/sites)
The newest version!
package com.sdl.selenium.conditions;
import com.google.common.base.Strings;
import java.util.Objects;
public abstract class Condition implements Comparable, ICondition {
private String message;
private String resultMessage;
private String className;
private int priority = 5;
@Override
public int compareTo(Condition condition) {
return getPriority() - condition.getPriority();
}
public boolean isTimeout() {
return false;
}
@Override
public boolean isFail() {
return !isSuccess();
}
public Condition() {
}
public Condition(String message) {
this.message = message;
}
public Condition(String message, String className) {
this.message = message;
this.className = className;
}
public Condition(String message, int priority) {
this(message);
this.priority = priority;
}
public Condition(String message, int priority, String className) {
this(message);
this.priority = priority;
this.className = className;
}
@Override
public String toString() {
String msg = getClassName();
if (Strings.isNullOrEmpty(msg)) {
msg = "Condition@" + getMessage();
} else {
msg += ":Condition@" + getMessage();
}
return msg;
}
@Override
public boolean equals(String message) {
return message != null && message.equals(getMessage());
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
Condition condition = (Condition) o;
return Objects.equals(message, condition.message);
}
@Override
public int hashCode() {
return Objects.hash(message, resultMessage, className, priority);
}
@Override
public String getMessage() {
return message;
}
public String getClassName() {
return className;
}
@Override
public int getPriority() {
return priority;
}
@Override
public void setPriority(int priority) {
this.priority = priority;
}
@Override
public String getResultMessage() {
return resultMessage;
}
protected void setResultMessage(final String resultMessage) {
this.resultMessage = resultMessage;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy