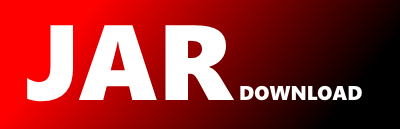
com.sdl.selenium.web.Transform Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of Testy Show documentation
Show all versions of Testy Show documentation
Automated Acceptance Testing. Selenium and Selenium WebDriver test framework for web applications.
(optimized for dynamic html, ExtJS, Bootstrap, complex UI, simple web applications/sites)
The newest version!
package com.sdl.selenium.web;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.node.ObjectNode;
import lombok.SneakyThrows;
import java.lang.reflect.Constructor;
import java.lang.reflect.InvocationTargetException;
import java.util.ArrayList;
import java.util.LinkedList;
import java.util.List;
import java.util.stream.Collectors;
public interface Transform {
ObjectMapper mapper = new ObjectMapper();
@SneakyThrows
default List transformTo(V type, List> actualListOfList, List columnsList) {
mapper.setSerializationInclusion(JsonInclude.Include.NON_NULL);
String json = mapper.writeValueAsString(type);
List names = getNames(json);
if (names.size() > actualListOfList.get(0).size()) {
names.removeIf(i -> columnsList.contains(names.indexOf(i) + 1));
}
int size = names.size();
LinkedList resultList = new LinkedList<>();
for (List actualList : actualListOfList) {
JsonNode jsonNode = mapper.readTree(json);
for (int i = 0; i < size; i++) {
String field = names.get(i);
String value = i >= actualList.size() ? null : actualList.get(i);
((ObjectNode) jsonNode).put(field, value);
}
V object = mapper.treeToValue(jsonNode, (Class) type.getClass());
resultList.add(object);
}
return resultList;
}
private List getNames(String json) throws JsonProcessingException {
List names = new ArrayList<>();
JsonNode jsonNode = mapper.readTree(json);
jsonNode.fields().forEachRemaining(i -> {
if (i.getValue().textValue() != null) {
names.add(i.getKey());
}
});
return names;
}
default List transformToObjectList(Class type, List> actualListOfList) {
Class> newClazz;
int size = actualListOfList.get(0).size();
Constructor> constructor = null;
try {
newClazz = Class.forName(type.getTypeName());
Constructor>[] constructors = newClazz.getConstructors();
for (Constructor> c : constructors) {
int parameterCount = c.getParameterCount();
if (size == parameterCount) {
constructor = c;
}
}
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
final Constructor finalConstructor = (Constructor) constructor;
return actualListOfList.stream().map(t -> {
try {
return finalConstructor.newInstance(t.toArray());
} catch (InstantiationException | IllegalAccessException | InvocationTargetException e) {
e.printStackTrace();
}
return null;
}).collect(Collectors.toList());
}
@SneakyThrows
default V transformToObject(V type, List actualList, List columnsList) {
String json = mapper.writeValueAsString(type);
List names = getNames(json);
if (names.size() > actualList.size()) {
names.removeIf(i -> columnsList.contains(names.indexOf(i) + 1));
}
int size = names.size();
JsonNode jsonNode = mapper.readTree(json);
for (int i = 0; i < size; i++) {
String field = names.get(i);
String value = i >= actualList.size() ? null : actualList.get(i);
((ObjectNode) jsonNode).put(field, value);
}
V object = mapper.treeToValue(jsonNode, (Class) type.getClass());
return object;
}
default V transformToObject(Class type, List cellsText) {
int fieldsCount;
Constructor constructor = null;
try {
Class> newClazz = Class.forName(type.getTypeName());
fieldsCount = cellsText.size();
Constructor[] constructors = newClazz.getConstructors();
for (Constructor c : constructors) {
if (fieldsCount == c.getParameterCount()) {
constructor = c;
}
}
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
try {
Constructor constructorTemp = (Constructor) constructor;
return constructorTemp.newInstance(cellsText.toArray(new Object[0]));
} catch (InstantiationException | IllegalAccessException | InvocationTargetException e) {
e.printStackTrace();
}
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy