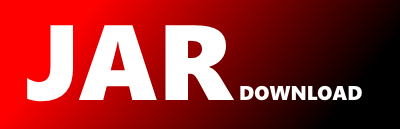
com.sdl.selenium.web.WebLocatorAbstractBuilder Maven / Gradle / Ivy
Show all versions of Testy Show documentation
package com.sdl.selenium.web;
import org.openqa.selenium.By;
import org.openqa.selenium.SearchContext;
import org.openqa.selenium.WebElement;
import java.time.Duration;
import java.util.List;
/**
* This class is used to simple construct xpath for WebLocator's
*/
public abstract class WebLocatorAbstractBuilder {
private XPathBuilder pathBuilder = createXPathBuilder();
protected XPathBuilder createXPathBuilder() {
return new XPathBuilder();
}
/**
* Used for finding element process (to generate xpath address)
*
* @return {@link XPathBuilder}
*/
public XPathBuilder getPathBuilder() {
return pathBuilder;
}
@SuppressWarnings("unchecked")
public T setPathBuilder(XPathBuilder pathBuilder) {
this.pathBuilder = pathBuilder;
return (T) this;
}
protected WebLocatorAbstractBuilder() {
}
// =========================================
// ========== setters ============
// =========================================
/**
* Used for finding element process (to generate xpath address)
*
* @param root If the path starts with // then all elements in the document which fulfill following criteria are selected. eg. // or /
* @param the element which calls this method
* @return this element
*/
@SuppressWarnings("unchecked")
public T setRoot(final String root) {
pathBuilder.setRoot(root);
return (T) this;
}
/**
* Used for finding element process (to generate xpath address)
*
* @param tag (type of DOM element) eg. input or h2
* @param the element which calls this method
* @return this element
*/
@SuppressWarnings("unchecked")
public T setTag(final String tag) {
pathBuilder.setTag(tag);
return (T) this;
}
/**
* Used for finding element process (to generate xpath address)
*
* @param id eg. id="buttonSubmit"
* @param the element which calls this method
* @return this element
*/
@SuppressWarnings("unchecked")
public T setId(final String id) {
pathBuilder.setId(id);
return (T) this;
}
/**
* Used for finding element process (to generate xpath address)
* Once used all other attributes will be ignored. Try using this class to a minimum!
*
* @param elPath absolute way (xpath) to identify element
* @param the element which calls this method
* @return this element
*/
@SuppressWarnings("unchecked")
public T setElPath(final String elPath) {
pathBuilder.setElPath(elPath);
return (T) this;
}
/**
* Used for finding element process (to generate css selectors address)
* Once used all other attributes will be ignored. Try using this class to a minimum!
*
* @param elCssSelector absolute way (css selectors) to identify element
* @param the element which calls this method
* @return this element
*/
@SuppressWarnings("unchecked")
public T setElCssSelector(final String elCssSelector) {
pathBuilder.setElCssSelector(elCssSelector);
return (T) this;
}
/**
* Used for finding element process (to generate xpath address)
*
* @param baseCls base class
* @param the element which calls this method
* @return this element
*/
@SuppressWarnings("unchecked")
public T setBaseCls(final String baseCls) {
pathBuilder.setBaseCls(baseCls);
return (T) this;
}
/**
* Used for finding element process (to generate xpath address)
* Find element with exact math of specified class (equals)
*
* @param cls class of element
* @param the element which calls this method
* @return this element
*/
@SuppressWarnings("unchecked")
public T setCls(final String cls) {
pathBuilder.setCls(cls);
return (T) this;
}
/**
* Used for finding element process (to generate xpath address)
* Use it when element must have all specified css classes (order is not important).
*
* - Provided classes must be conform css rules.
*
*
* @param classes list of classes
* @param the element which calls this method
* @return this element
*/
@SuppressWarnings("unchecked")
public T setClasses(final String... classes) {
pathBuilder.setClasses(classes);
return (T) this;
}
@SuppressWarnings("unchecked")
public T setClasses(Operator classesType, final String... classes) {
pathBuilder.setClasses(classesType, classes);
return (T) this;
}
/**
* Used for finding element process (to generate xpath address)
*
* @param excludeClasses list of class to be excluded
* @param the element which calls this method
* @return this element
*/
@SuppressWarnings("unchecked")
public T setExcludeClasses(final String... excludeClasses) {
pathBuilder.setExcludeClasses(excludeClasses);
return (T) this;
}
/**
* @param childNodes list of WebLocators
* @param the element which calls this method
* @return this element
*/
@SuppressWarnings("unchecked")
public T setChildNodes(final WebLocator... childNodes) {
pathBuilder.setChildNodes(null, childNodes);
return (T) this;
}
public T setChildNodes(final List childNodes) {
pathBuilder.setChildNodes(null, childNodes.toArray(new WebLocator[0]));
return (T) this;
}
public T setChildNodes(SearchType searchType, final WebLocator... childNodes) {
pathBuilder.setChildNodes(searchType, childNodes);
return (T) this;
}
/**
* Used for finding element process (to generate xpath address)
*
* @param name eg. name="buttonSubmit"
* @param the element which calls this method
* @return this element
*/
@SuppressWarnings("unchecked")
public T setName(final String name) {
pathBuilder.setName(name);
return (T) this;
}
/**
* Used for finding element process (to generate xpath address)
*
* @param localName eg. name()="svg"
* @param the element which calls this method
* @return this element
*/
@SuppressWarnings("unchecked")
public T setLocalName(final String localName) {
pathBuilder.setLocalName(localName);
return (T) this;
}
/**
* Used for finding element process (to generate xpath address)
*
* @param text with which to identify the item
* @param searchTypes type search text element: see more details see {@link SearchType}
* @param the element which calls this method
* @return this element
*/
@SuppressWarnings("unchecked")
public T setText(String text, final SearchType... searchTypes) {
pathBuilder.setText(text, searchTypes);
return (T) this;
}
/**
* Used for finding element process (to generate xpath address)
* This method reset searchTextType and set to new searchTextType.
*
* @param searchTextTypes accepted values are: {@link SearchType#EQUALS}
* @param the element which calls this method
* @return this element
*/
@SuppressWarnings("unchecked")
public T setSearchTextType(SearchType... searchTextTypes) {
pathBuilder.setSearchTextType(searchTextTypes);
return (T) this;
}
/**
* Used for finding element process (to generate xpath address)
*
* @param searchLabelTypes accepted values are: {@link SearchType}
* @param the element which calls this method
* @return this element
*/
@SuppressWarnings("unchecked")
private T setSearchLabelType(SearchType... searchLabelTypes) {
pathBuilder.setSearchLabelType(searchLabelTypes);
return (T) this;
}
/**
* Used for finding element process (to generate xpath address)
*
* @param searchTitleTypes accepted values are: {@link SearchType}
* @param the element which calls this method
* @return this element
*/
@SuppressWarnings("unchecked")
public T setSearchTitleType(SearchType... searchTitleTypes) {
pathBuilder.setSearchTitleType(searchTitleTypes);
return (T) this;
}
/**
* Used for finding element process (to generate xpath address)
*
* @param style of element
* @param the element which calls this method
* @return this element
*/
@SuppressWarnings("unchecked")
public T setStyle(final String style) {
pathBuilder.setStyle(style);
return (T) this;
}
/**
* Used for finding element process (to generate xpath address)
*
* @param title of element
* @param searchTypes see {@link SearchType}
* @param the element which calls this method
* @return this element
*/
@SuppressWarnings("unchecked")
public T setTitle(String title, SearchType... searchTypes) {
pathBuilder.setTitle(title, searchTypes);
return (T) this;
}
/**
* @param titleEl title element
* @param the element which calls this method
* @return this element
*/
@SuppressWarnings("unchecked")
public T setTemplateTitle(WebLocator titleEl) {
pathBuilder.setTemplateTitle(titleEl);
return (T) this;
}
/**
* Used for finding element process (to generate xpath address)
* Example:
*
* TODO
*
*
* @param key suffix key
* @param elPathSuffix additional identification xpath element that will be added at the end
* @param the element which calls this method
* @return this element
*/
@SuppressWarnings("unchecked")
public T setElPathSuffix(final String key, final String elPathSuffix) {
pathBuilder.setElPathSuffix(key, elPathSuffix);
return (T) this;
}
/**
* Used for finding element process (to generate xpath address)
* Example:
*
* WebLocator child = new WebLocator().setTemplate("custom", "%1$s = %2$s");
* child.setTemplateValue("custom", "a", "b");
* assertThat(child.getXPath(), equalTo("//*[a = b]"));
*
*
* @param key identify key
* @param value value
* @param the element which calls this method
* @return this element
*/
@SuppressWarnings("unchecked")
public T setTemplateValue(final String key, final String... value) {
pathBuilder.setTemplateValue(key, value);
return (T) this;
}
/**
* For customize template please see here: See http://docs.oracle.com/javase/7/docs/api/java/util/Formatter.html#dpos
*
* @param key name template
* @param value template
* @param the element which calls this method
* @return this element
*/
@SuppressWarnings("unchecked")
public T setTemplate(final String key, final String value) {
pathBuilder.setTemplate(key, value);
return (T) this;
}
/**
* Used in logging process
*
* @param infoMessage info Message
* @param the element which calls this method
* @return this element
*/
@SuppressWarnings("unchecked")
public T setInfoMessage(final String infoMessage) {
pathBuilder.setInfoMessage(infoMessage);
return (T) this;
}
/**
* Used for finding element process (to generate xpath address)
* Example:
*
* TODO
*
*
* @param visibility true or false
* @param the element which calls this method
* @return this element
*/
@SuppressWarnings("unchecked")
public T setVisibility(final boolean visibility) {
pathBuilder.setVisibility(visibility);
return (T) this;
}
/**
* @param renderMillis time
* @param the element which calls this method
* @return this element
*/
@SuppressWarnings("unchecked")
@Deprecated
public T setRenderMillis(final long renderMillis) {
pathBuilder.setRenderMillis(renderMillis);
return (T) this;
}
/**
* @param duration time
* @param the element which calls this method
* @return this element
*/
@SuppressWarnings("unchecked")
public T setRender(Duration duration) {
pathBuilder.setRender(duration);
return (T) this;
}
/**
* @param activateSeconds true or false
* @param the element which calls this method
* @return this element
*/
@SuppressWarnings("unchecked")
@Deprecated
public T setActivateSeconds(final int activateSeconds) {
pathBuilder.setActivateSeconds(activateSeconds);
return (T) this;
}
/**
* @param duration time
* @param the element which calls this method
* @return this element
*/
@SuppressWarnings("unchecked")
public T setActivate(Duration duration) {
pathBuilder.setActivate(duration);
return (T) this;
}
/**
* Used for finding element process (to generate xpath address)
*
* @param container parent containing element.
* @param the element which calls this method
* @return this element
*/
@SuppressWarnings("unchecked")
public T setContainer(WebLocator container) {
pathBuilder.setContainer(container);
return (T) this;
}
/**
* Used for finding element process (to generate xpath address)
*
* @param webElement parent containing element.
* @param the element which calls this method
* @return this element
*/
@SuppressWarnings("unchecked")
public T setWebElement(WebElement webElement) {
pathBuilder.setWebElement(webElement);
return (T) this;
}
/**
* Used for finding element process (to generate xpath address)
*
* @param shadowRoot parent containing element.
* @param the element which calls this method
* @return this element
*/
@SuppressWarnings("unchecked")
public T setShadowRoot(SearchContext shadowRoot) {
pathBuilder.setShadowRoot(shadowRoot);
return (T) this;
}
/**
* Used for finding element process (to generate xpath address)
*
* @param label text label element
* @param searchTypes type search text element: see more details see {@link SearchType}
* @param the element which calls this method
* @return this element
*/
@SuppressWarnings("unchecked")
public T setLabel(String label, final SearchType... searchTypes) {
pathBuilder.setLabel(label, searchTypes);
return (T) this;
}
/**
* Used for finding element process (to generate xpath address)
*
* @param labelTag label tag element
* @param the element which calls this method
* @return this element
*/
@SuppressWarnings("unchecked")
public T setLabelTag(final String labelTag) {
pathBuilder.setLabelTag(labelTag);
return (T) this;
}
/**
* Used for finding element process (to generate xpath address)
*
* @param labelPosition position of this element reported to label
* @param the element which calls this method
* @return this element
* @see http://www.w3schools.com/xpath/xpath_axes.asp"
*/
@SuppressWarnings("unchecked")
public T setLabelPosition(final String labelPosition) {
pathBuilder.setLabelPosition(labelPosition);
return (T) this;
}
/**
* Used for finding element process (to generate xpath address)
* Result Example:
*
* //*[contains(@class, 'x-grid-panel')][position() = 1]
*
*
* @param position starting index = 1
* @param the element which calls this method
* @return this element
*/
@SuppressWarnings("unchecked")
public T setPosition(final int position) {
pathBuilder.setPosition(position);
return (T) this;
}
/**
* Used for finding element process (to generate xpath address)
* Result Example:
*
* //*[contains(@class, 'x-grid-panel')][position() = last()]
*
*
* @param position first() or last()
* @param the element which calls this method
* @return this element
*/
@SuppressWarnings("unchecked")
public T setPosition(final Position position) {
pathBuilder.setPosition(position);
return (T) this;
}
/**
* Used for finding element process (to generate xpath address)
* Result Example:
*
* //*[contains(@class, 'x-grid-panel')][position() = 1]
*
*
* @param resultIdx starting index = 1
* @param the element which calls this method
* @return this element
*/
@SuppressWarnings("unchecked")
public T setResultIdx(final int resultIdx) {
pathBuilder.setResultIdx(resultIdx);
return (T) this;
}
/**
* Used for finding element process (to generate xpath address)
* Result Example:
*
* //*[contains(@class, 'x-grid-panel')][last()]
*
*
* @param resultIdx first() or last()
* @param the element which calls this method
* @return this element
*/
@SuppressWarnings("unchecked")
public T setResultIdx(final Position resultIdx) {
pathBuilder.setResultIdx(resultIdx);
return (T) this;
}
/**
* Used for finding element process (to generate xpath address)
* Result Example:
*
* //*[@type='type']
*
*
* @param type eg. checkbox, button
* @param the element which calls this method
* @return this element
*/
@SuppressWarnings("unchecked")
public T setType(final String type) {
pathBuilder.setType(type);
return (T) this;
}
/**
* Used for finding element process (to generate xpath address)
* Result Example:
*
* //*[@placeholder='Search']
*
*
* @param attribute eg. placeholder
* @param value eg. Search
* @param searchTypes see {@link SearchType}
* @param the element which calls this method
* @return this element
*/
@SuppressWarnings("unchecked")
public T setAttribute(final String attribute, String value, final SearchType... searchTypes) {
pathBuilder.setAttribute(attribute, value, searchTypes);
return (T) this;
}
/**
* Used for finding element process (to generate xpath address)
* Result Example:
*
* new WebLocator().setAttributes("placeholder", "Search", "Text");
* //*[contains(concat(' ', @placeholder, ' '), ' Search ') and contains(concat(' ', @placeholder, ' '), ' Text ')]
*
*
* @param attribute eg. placeholder
* @param values eg. Search,Text
* @param the element which calls this method
* @return this element
*/
@SuppressWarnings("unchecked")
public T setAttributes(final String attribute, SearchText... values) {
pathBuilder.setAttributes(attribute, values);
return (T) this;
}
/**
* Used for finding element process (to generate xpath address)
* Result Example:
*
* new WebLocator().setAttributes("placeholder", Operator.OR, "Search", "Search");
* //*[contains(concat(' ', @placeholder, ' '), ' Search ') or contains(concat(' ', @placeholder, ' '), ' Search ')]
*
*
* @param attribute eg. placeholder
* @param operator eg. AND or OR
* @param values eg. Search,Text
* @param the element which calls this method
* @return this element
*/
@SuppressWarnings("unchecked")
public T setAttributes(final String attribute, Operator operator, SearchText... values) {
pathBuilder.setAttributes(attribute, operator, values);
return (T) this;
}
/**
* Used for finding element process (to generate xpath address)
* Result Example:
*
* new WebLocator().setAttribute("atr", "2").setFinalXPath("//tab[1]")
* //*[@atr='2']//tab[1]
*
*
* @param finalXPath //tab[1]
* @param the element which calls this method
* @return this element
*/
@SuppressWarnings("unchecked")
public T setFinalXPath(final String finalXPath) {
pathBuilder.setFinalXPath(finalXPath);
return (T) this;
}
/**
* Used for finding element process (to generate xpath address)
* This method add new searchTextType to existing searchTextType.
*
* @param searchTextTypes accepted values are: {@link SearchType#EQUALS}
* @param the element which calls this method
* @return this element
*/
@SuppressWarnings("unchecked")
public T addSearchTextType(SearchType... searchTextTypes) {
pathBuilder.addSearchTextType(searchTextTypes);
return (T) this;
}
@SuppressWarnings("unchecked")
public T addToTemplate(final String key, final String value) {
pathBuilder.addToTemplate(key, value);
return (T) this;
}
// =========================================
// =============== Methods =================
// =========================================
/**
* Used only to identify class type of current object
*
* @param className className
*/
protected void setClassName(final String className) {
pathBuilder.setClassName(className);
}
protected boolean hasId() {
return pathBuilder.hasId();
}
protected boolean hasText() {
return pathBuilder.hasText();
}
protected boolean hasLabel() {
return pathBuilder.hasLabel();
}
protected boolean hasTitle() {
return pathBuilder.hasTitle();
}
protected boolean hasPosition() {
return pathBuilder.hasPosition();
}
protected boolean hasResultIdx() {
return pathBuilder.hasResultIdx();
}
public final By getSelector() {
return pathBuilder.getSelector();
}
public final String getCssSelector() {
return pathBuilder.getCssSelector();
}
/**
* @return final xpath (including containers xpath), used for interacting with browser
*/
public String getXPath() {
return pathBuilder.getXPath();
}
/**
* @param disabled true or false
* @return xPath
*/
public final String getXPath(boolean disabled) {
return pathBuilder.getXPath(disabled);
}
protected String applyTemplate(String key, Object... arguments) {
return pathBuilder.applyTemplate(key, arguments);
}
}