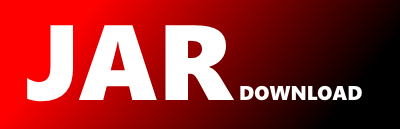
com.sdl.selenium.web.utils.OrderedProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of Testy Show documentation
Show all versions of Testy Show documentation
Automated Acceptance Testing. Selenium and Selenium WebDriver test framework for web applications.
(optimized for dynamic html, ExtJS, Bootstrap, complex UI, simple web applications/sites)
The newest version!
package com.sdl.selenium.web.utils;
import java.util.*;
public class OrderedProperties extends Properties {
private Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy