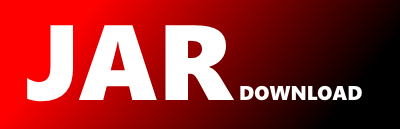
com.seepine.mybatis.tenant.TenantUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of spring-boot-starter-mybatis Show documentation
Show all versions of spring-boot-starter-mybatis Show documentation
Spring Boot Mybatis Dependencies
The newest version!
package com.seepine.mybatis.tenant;
import lombok.experimental.UtilityClass;
import lombok.extern.slf4j.Slf4j;
import java.util.ArrayList;
import java.util.List;
/**
* 租户工具类
*
* @author seepine
*/
@Slf4j
@UtilityClass
public class TenantUtil {
private static final List TENANT_TABLE = new ArrayList<>();
private final ThreadLocal
© 2015 - 2025 Weber Informatics LLC | Privacy Policy