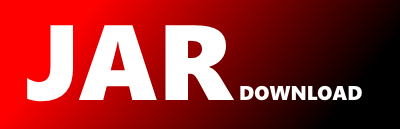
com.segment.analytics.JsonMap Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of core Show documentation
Show all versions of core Show documentation
The hassle-free way to add analytics to your Android app.
package com.segment.analytics;
import java.io.IOException;
import java.lang.reflect.Constructor;
import java.lang.reflect.InvocationTargetException;
import java.util.ArrayList;
import java.util.Collection;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.concurrent.ConcurrentHashMap;
import org.json.JSONObject;
import static com.segment.analytics.Utils.NullableConcurrentHashMap;
/**
* A {@link Map} wrapper to expose Json functionality. Only the {@link #toString()} method is
* modified to return a json formatted string. All other methods will be forwarded to a delegate
* map.
*
* The purpose of this class is to not limit clients to a custom implementation of a Json type,
* they
* can use existing {@link Map} and {@link java.util.List} implementations as they see fit. It adds
* some utility methods, including methods to coerce numeric types from Strings, and a {@link
* #putValue(String, Object)} to be able to chain method calls.
*
* Although it lets you use custom objects for values, note that type information is lost during
* serialization. You should use one of the coercion methods if you're expecting a type after
* serialization.
*/
class JsonMap implements Map {
private final ConcurrentHashMap delegate;
JsonMap() {
delegate = new NullableConcurrentHashMap();
}
JsonMap(Map map) {
if (map == null) {
throw new IllegalArgumentException("Map must not be null.");
}
this.delegate = new NullableConcurrentHashMap(map);
}
JsonMap(String json) {
try {
Map map = JsonUtils.jsonToMap(json);
this.delegate = new NullableConcurrentHashMap(map);
} catch (IOException e) {
throw new RuntimeException(e);
}
}
@Override public void clear() {
delegate.clear();
}
@Override public boolean containsKey(Object key) {
return delegate.containsKey(key);
}
@Override public boolean containsValue(Object value) {
return delegate.containsValue(value);
}
@Override public Set> entrySet() {
return delegate.entrySet();
}
@Override public Object get(Object key) {
return delegate.get(key);
}
@Override public boolean isEmpty() {
return delegate.isEmpty();
}
@Override public Set keySet() {
return delegate.keySet();
}
@Override public Object put(String key, Object value) {
return delegate.put(key, value);
}
@Override public void putAll(Map extends String, ?> map) {
delegate.putAll(map);
}
@Override public Object remove(Object key) {
return delegate.remove(key);
}
@Override public int size() {
return delegate.size();
}
@Override public Collection
© 2015 - 2025 Weber Informatics LLC | Privacy Policy