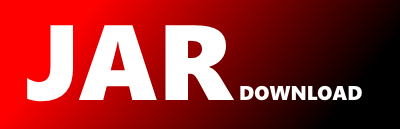
com.segment.analytics.messages.AutoValue_GroupMessage Maven / Gradle / Ivy
The newest version!
package com.segment.analytics.messages;
import java.util.Date;
import java.util.Map;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
// Generated by com.google.auto.value.processor.AutoValueProcessor
final class AutoValue_GroupMessage extends GroupMessage {
private final Message.Type type;
private final String messageId;
private final Date sentAt;
private final Date timestamp;
private final Map context;
private final String anonymousId;
private final String userId;
private final Map integrations;
private final String groupId;
private final Map traits;
AutoValue_GroupMessage(
Message.Type type,
String messageId,
@Nullable Date sentAt,
Date timestamp,
@Nullable Map context,
@Nullable String anonymousId,
@Nullable String userId,
@Nullable Map integrations,
String groupId,
@Nullable Map traits) {
if (type == null) {
throw new NullPointerException("Null type");
}
this.type = type;
if (messageId == null) {
throw new NullPointerException("Null messageId");
}
this.messageId = messageId;
this.sentAt = sentAt;
if (timestamp == null) {
throw new NullPointerException("Null timestamp");
}
this.timestamp = timestamp;
this.context = context;
this.anonymousId = anonymousId;
this.userId = userId;
this.integrations = integrations;
if (groupId == null) {
throw new NullPointerException("Null groupId");
}
this.groupId = groupId;
this.traits = traits;
}
@Nonnull
@Override
public Message.Type type() {
return type;
}
@Nonnull
@Override
public String messageId() {
return messageId;
}
@Nullable
@Override
public Date sentAt() {
return sentAt;
}
@Nonnull
@Override
public Date timestamp() {
return timestamp;
}
@Nullable
@Override
public Map context() {
return context;
}
@Nullable
@Override
public String anonymousId() {
return anonymousId;
}
@Nullable
@Override
public String userId() {
return userId;
}
@Nullable
@Override
public Map integrations() {
return integrations;
}
@Override
public String groupId() {
return groupId;
}
@Nullable
@Override
public Map traits() {
return traits;
}
@Override
public String toString() {
return "GroupMessage{"
+ "type=" + type + ", "
+ "messageId=" + messageId + ", "
+ "sentAt=" + sentAt + ", "
+ "timestamp=" + timestamp + ", "
+ "context=" + context + ", "
+ "anonymousId=" + anonymousId + ", "
+ "userId=" + userId + ", "
+ "integrations=" + integrations + ", "
+ "groupId=" + groupId + ", "
+ "traits=" + traits
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof GroupMessage) {
GroupMessage that = (GroupMessage) o;
return this.type.equals(that.type())
&& this.messageId.equals(that.messageId())
&& (this.sentAt == null ? that.sentAt() == null : this.sentAt.equals(that.sentAt()))
&& this.timestamp.equals(that.timestamp())
&& (this.context == null ? that.context() == null : this.context.equals(that.context()))
&& (this.anonymousId == null ? that.anonymousId() == null : this.anonymousId.equals(that.anonymousId()))
&& (this.userId == null ? that.userId() == null : this.userId.equals(that.userId()))
&& (this.integrations == null ? that.integrations() == null : this.integrations.equals(that.integrations()))
&& this.groupId.equals(that.groupId())
&& (this.traits == null ? that.traits() == null : this.traits.equals(that.traits()));
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= type.hashCode();
h$ *= 1000003;
h$ ^= messageId.hashCode();
h$ *= 1000003;
h$ ^= (sentAt == null) ? 0 : sentAt.hashCode();
h$ *= 1000003;
h$ ^= timestamp.hashCode();
h$ *= 1000003;
h$ ^= (context == null) ? 0 : context.hashCode();
h$ *= 1000003;
h$ ^= (anonymousId == null) ? 0 : anonymousId.hashCode();
h$ *= 1000003;
h$ ^= (userId == null) ? 0 : userId.hashCode();
h$ *= 1000003;
h$ ^= (integrations == null) ? 0 : integrations.hashCode();
h$ *= 1000003;
h$ ^= groupId.hashCode();
h$ *= 1000003;
h$ ^= (traits == null) ? 0 : traits.hashCode();
return h$;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy