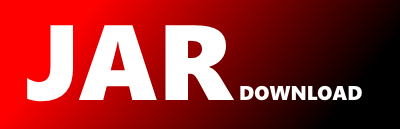
com.segment.analytics.messages.IdentifyMessage Maven / Gradle / Ivy
package com.segment.analytics.messages;
import com.google.auto.value.AutoValue;
import com.segment.analytics.gson.AutoGson;
import java.util.Date;
import java.util.Map;
import javax.annotation.Nullable;
/**
* The identify call ties a customer and their actions to a recognizable ID and traits like their
* email, name, etc.
*
* Use {@link #builder} to construct your own instances.
*
* @see Identify
*/
@AutoValue
@AutoGson //
public abstract class IdentifyMessage implements Message {
/** Start building an {@link IdentifyMessage} instance. */
public static Builder builder() {
return new Builder();
}
@Nullable
public abstract Map traits();
public Builder toBuilder() {
return new Builder(this);
}
/** Fluent API for creating {@link IdentifyMessage} instances. */
public static class Builder extends MessageBuilder {
private Map traits;
private Builder(IdentifyMessage identify) {
super(identify);
traits = identify.traits();
}
private Builder() {
super(Type.identify);
}
/**
* Set a map of information of the user, like email or name.
*
* @see Traits
*/
public Builder traits(Map traits) {
if (traits == null) {
throw new NullPointerException("Null traits");
}
this.traits = ImmutableMap.copyOf(traits);
return this;
}
@Override
protected IdentifyMessage realBuild(
Type type,
String messageId,
Date sentAt,
Date timestamp,
Map context,
String anonymousId,
String userId,
Map integrations) {
if (userId == null && traits == null) {
throw new IllegalStateException("Either userId or traits must be provided.");
}
return new AutoValue_IdentifyMessage(
type, messageId, sentAt, timestamp, context, anonymousId, userId, integrations, traits);
}
@Override
Builder self() {
return this;
}
}
}