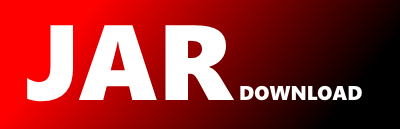
com.segment.analytics.messages.ScreenMessage Maven / Gradle / Ivy
package com.segment.analytics.messages;
import com.google.auto.value.AutoValue;
import com.segment.analytics.gson.AutoGson;
import java.util.Date;
import java.util.Map;
import javax.annotation.Nullable;
/**
* The screen call lets you record whenever a user sees a screen, along with any properties about
* the screen.
*
* Use {@link #builder} to construct your own instances.
*
* @see Screen
*/
@AutoValue
@AutoGson //
public abstract class ScreenMessage implements Message {
/**
* Start building an {@link ScreenMessage} instance.
*
* @param name The name of the screen the user is on.
* @throws IllegalArgumentException if the screen name is null or empty
* @see Name
*/
public static Builder builder(String name) {
return new Builder(name);
}
public abstract String name();
@Nullable
public abstract Map properties();
public Builder toBuilder() {
return new Builder(this);
}
/** Fluent API for creating {@link ScreenMessage} instances. */
public static class Builder extends MessageBuilder {
private String name;
private Map properties;
private Builder(ScreenMessage screen) {
super(screen);
name = screen.name();
properties = screen.properties();
}
private Builder(String name) {
super(Type.screen);
if (isNullOrEmpty(name)) {
throw new IllegalArgumentException("screen name cannot be null or empty.");
}
this.name = name;
}
/**
* Set a map of information that describe the screen. These can be anything you want.
*
* @see Properties
*/
public Builder properties(Map properties) {
if (properties == null) {
throw new NullPointerException("Null properties");
}
this.properties = ImmutableMap.copyOf(properties);
return this;
}
@Override
Builder self() {
return this;
}
@Override
protected ScreenMessage realBuild(
Type type,
String messageId,
Date sentAt,
Date timestamp,
Map context,
String anonymousId,
String userId,
Map integrations) {
return new AutoValue_ScreenMessage(
type,
messageId,
sentAt,
timestamp,
context,
anonymousId,
userId,
integrations,
name,
properties);
}
}
}