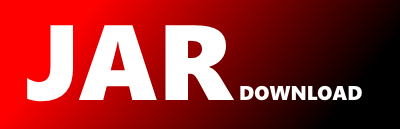
com.segment.publicapi.models.DestinationMetadata Maven / Gradle / Ivy
/*
* Segment Public API
* The Segment Public API helps you manage your Segment Workspaces and its resources. You can use the API to perform CRUD (create, read, update, delete) operations at no extra charge. This includes working with resources such as Sources, Destinations, Warehouses, Tracking Plans, and the Segment Destinations and Sources Catalogs. All CRUD endpoints in the API follow REST conventions and use standard HTTP methods. Different URL endpoints represent different resources in a Workspace. See the next sections for more information on how to use the Segment Public API.
*
* The version of the OpenAPI document: 32.0.3
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.segment.publicapi.models;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.segment.publicapi.models.Contact;
import com.segment.publicapi.models.DestinationMetadataActionV1;
import com.segment.publicapi.models.DestinationMetadataComponentV1;
import com.segment.publicapi.models.DestinationMetadataSubscriptionPresetV1;
import com.segment.publicapi.models.IntegrationOptionBeta;
import com.segment.publicapi.models.Logos;
import com.segment.publicapi.models.SupportedFeatures;
import com.segment.publicapi.models.SupportedMethods;
import com.segment.publicapi.models.SupportedPlatforms;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.JsonArray;
import com.google.gson.JsonDeserializationContext;
import com.google.gson.JsonDeserializer;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.JsonParseException;
import com.google.gson.TypeAdapterFactory;
import com.google.gson.reflect.TypeToken;
import java.lang.reflect.Type;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Set;
import com.segment.publicapi.JSON;
/**
* The catalog item matched by id.
*/
@ApiModel(description = "The catalog item matched by id.")
public class DestinationMetadata {
public static final String SERIALIZED_NAME_ID = "id";
@SerializedName(SERIALIZED_NAME_ID)
private String id;
public static final String SERIALIZED_NAME_NAME = "name";
@SerializedName(SERIALIZED_NAME_NAME)
private String name;
public static final String SERIALIZED_NAME_DESCRIPTION = "description";
@SerializedName(SERIALIZED_NAME_DESCRIPTION)
private String description;
public static final String SERIALIZED_NAME_SLUG = "slug";
@SerializedName(SERIALIZED_NAME_SLUG)
private String slug;
public static final String SERIALIZED_NAME_LOGOS = "logos";
@SerializedName(SERIALIZED_NAME_LOGOS)
private Logos logos;
public static final String SERIALIZED_NAME_OPTIONS = "options";
@SerializedName(SERIALIZED_NAME_OPTIONS)
private List options = new ArrayList<>();
/**
* Support status of the Destination.
*/
@JsonAdapter(StatusEnum.Adapter.class)
public enum StatusEnum {
DEPRECATED("DEPRECATED"),
PRIVATE_BETA("PRIVATE_BETA"),
PRIVATE_BUILDING("PRIVATE_BUILDING"),
PRIVATE_SUBMITTED("PRIVATE_SUBMITTED"),
PUBLIC("PUBLIC"),
PUBLIC_BETA("PUBLIC_BETA"),
UNAVAILABLE("UNAVAILABLE");
private String value;
StatusEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static StatusEnum fromValue(String value) {
for (StatusEnum b : StatusEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final StatusEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public StatusEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return StatusEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_STATUS = "status";
@SerializedName(SERIALIZED_NAME_STATUS)
private StatusEnum status;
public static final String SERIALIZED_NAME_PREVIOUS_NAMES = "previousNames";
@SerializedName(SERIALIZED_NAME_PREVIOUS_NAMES)
private List previousNames = new ArrayList<>();
public static final String SERIALIZED_NAME_CATEGORIES = "categories";
@SerializedName(SERIALIZED_NAME_CATEGORIES)
private List categories = new ArrayList<>();
public static final String SERIALIZED_NAME_WEBSITE = "website";
@SerializedName(SERIALIZED_NAME_WEBSITE)
private String website;
public static final String SERIALIZED_NAME_COMPONENTS = "components";
@SerializedName(SERIALIZED_NAME_COMPONENTS)
private List components = new ArrayList<>();
public static final String SERIALIZED_NAME_SUPPORTED_FEATURES = "supportedFeatures";
@SerializedName(SERIALIZED_NAME_SUPPORTED_FEATURES)
private SupportedFeatures supportedFeatures;
public static final String SERIALIZED_NAME_SUPPORTED_METHODS = "supportedMethods";
@SerializedName(SERIALIZED_NAME_SUPPORTED_METHODS)
private SupportedMethods supportedMethods;
public static final String SERIALIZED_NAME_SUPPORTED_PLATFORMS = "supportedPlatforms";
@SerializedName(SERIALIZED_NAME_SUPPORTED_PLATFORMS)
private SupportedPlatforms supportedPlatforms;
public static final String SERIALIZED_NAME_ACTIONS = "actions";
@SerializedName(SERIALIZED_NAME_ACTIONS)
private List actions = new ArrayList<>();
public static final String SERIALIZED_NAME_PRESETS = "presets";
@SerializedName(SERIALIZED_NAME_PRESETS)
private List presets = new ArrayList<>();
public static final String SERIALIZED_NAME_CONTACTS = "contacts";
@SerializedName(SERIALIZED_NAME_CONTACTS)
private List contacts = null;
public static final String SERIALIZED_NAME_PARTNER_OWNED = "partnerOwned";
@SerializedName(SERIALIZED_NAME_PARTNER_OWNED)
private Boolean partnerOwned;
public DestinationMetadata() {
}
public DestinationMetadata id(String id) {
this.id = id;
return this;
}
/**
* The id of the Destination metadata. Config API note: analogous to `name`.
* @return id
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "The id of the Destination metadata. Config API note: analogous to `name`.")
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public DestinationMetadata name(String name) {
this.name = name;
return this;
}
/**
* The user-friendly name of the Destination. Config API note: equal to `displayName`.
* @return name
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "The user-friendly name of the Destination. Config API note: equal to `displayName`.")
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public DestinationMetadata description(String description) {
this.description = description;
return this;
}
/**
* The description of the Destination.
* @return description
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "The description of the Destination.")
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public DestinationMetadata slug(String slug) {
this.slug = slug;
return this;
}
/**
* The slug used to identify the Destination in the Segment app.
* @return slug
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "The slug used to identify the Destination in the Segment app.")
public String getSlug() {
return slug;
}
public void setSlug(String slug) {
this.slug = slug;
}
public DestinationMetadata logos(Logos logos) {
this.logos = logos;
return this;
}
/**
* Get logos
* @return logos
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "")
public Logos getLogos() {
return logos;
}
public void setLogos(Logos logos) {
this.logos = logos;
}
public DestinationMetadata options(List options) {
this.options = options;
return this;
}
public DestinationMetadata addOptionsItem(IntegrationOptionBeta optionsItem) {
this.options.add(optionsItem);
return this;
}
/**
* Options configured for the Destination.
* @return options
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "Options configured for the Destination.")
public List getOptions() {
return options;
}
public void setOptions(List options) {
this.options = options;
}
public DestinationMetadata status(StatusEnum status) {
this.status = status;
return this;
}
/**
* Support status of the Destination.
* @return status
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "Support status of the Destination.")
public StatusEnum getStatus() {
return status;
}
public void setStatus(StatusEnum status) {
this.status = status;
}
public DestinationMetadata previousNames(List previousNames) {
this.previousNames = previousNames;
return this;
}
public DestinationMetadata addPreviousNamesItem(String previousNamesItem) {
this.previousNames.add(previousNamesItem);
return this;
}
/**
* A list of names previously used by the Destination.
* @return previousNames
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "A list of names previously used by the Destination.")
public List getPreviousNames() {
return previousNames;
}
public void setPreviousNames(List previousNames) {
this.previousNames = previousNames;
}
public DestinationMetadata categories(List categories) {
this.categories = categories;
return this;
}
public DestinationMetadata addCategoriesItem(String categoriesItem) {
this.categories.add(categoriesItem);
return this;
}
/**
* A list of categories with which the Destination is associated.
* @return categories
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "A list of categories with which the Destination is associated.")
public List getCategories() {
return categories;
}
public void setCategories(List categories) {
this.categories = categories;
}
public DestinationMetadata website(String website) {
this.website = website;
return this;
}
/**
* A website URL for this Destination.
* @return website
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "A website URL for this Destination.")
public String getWebsite() {
return website;
}
public void setWebsite(String website) {
this.website = website;
}
public DestinationMetadata components(List components) {
this.components = components;
return this;
}
public DestinationMetadata addComponentsItem(DestinationMetadataComponentV1 componentsItem) {
this.components.add(componentsItem);
return this;
}
/**
* A list of components this Destination provides.
* @return components
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "A list of components this Destination provides.")
public List getComponents() {
return components;
}
public void setComponents(List components) {
this.components = components;
}
public DestinationMetadata supportedFeatures(SupportedFeatures supportedFeatures) {
this.supportedFeatures = supportedFeatures;
return this;
}
/**
* Get supportedFeatures
* @return supportedFeatures
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "")
public SupportedFeatures getSupportedFeatures() {
return supportedFeatures;
}
public void setSupportedFeatures(SupportedFeatures supportedFeatures) {
this.supportedFeatures = supportedFeatures;
}
public DestinationMetadata supportedMethods(SupportedMethods supportedMethods) {
this.supportedMethods = supportedMethods;
return this;
}
/**
* Get supportedMethods
* @return supportedMethods
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "")
public SupportedMethods getSupportedMethods() {
return supportedMethods;
}
public void setSupportedMethods(SupportedMethods supportedMethods) {
this.supportedMethods = supportedMethods;
}
public DestinationMetadata supportedPlatforms(SupportedPlatforms supportedPlatforms) {
this.supportedPlatforms = supportedPlatforms;
return this;
}
/**
* Get supportedPlatforms
* @return supportedPlatforms
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "")
public SupportedPlatforms getSupportedPlatforms() {
return supportedPlatforms;
}
public void setSupportedPlatforms(SupportedPlatforms supportedPlatforms) {
this.supportedPlatforms = supportedPlatforms;
}
public DestinationMetadata actions(List actions) {
this.actions = actions;
return this;
}
public DestinationMetadata addActionsItem(DestinationMetadataActionV1 actionsItem) {
this.actions.add(actionsItem);
return this;
}
/**
* Actions available for the Destination.
* @return actions
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "Actions available for the Destination.")
public List getActions() {
return actions;
}
public void setActions(List actions) {
this.actions = actions;
}
public DestinationMetadata presets(List presets) {
this.presets = presets;
return this;
}
public DestinationMetadata addPresetsItem(DestinationMetadataSubscriptionPresetV1 presetsItem) {
this.presets.add(presetsItem);
return this;
}
/**
* Predefined Destination subscriptions that can optionally be applied when connecting a new instance of the Destination.
* @return presets
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "Predefined Destination subscriptions that can optionally be applied when connecting a new instance of the Destination.")
public List getPresets() {
return presets;
}
public void setPresets(List presets) {
this.presets = presets;
}
public DestinationMetadata contacts(List contacts) {
this.contacts = contacts;
return this;
}
public DestinationMetadata addContactsItem(Contact contactsItem) {
if (this.contacts == null) {
this.contacts = new ArrayList<>();
}
this.contacts.add(contactsItem);
return this;
}
/**
* Contact info for Integration Owners.
* @return contacts
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Contact info for Integration Owners.")
public List getContacts() {
return contacts;
}
public void setContacts(List contacts) {
this.contacts = contacts;
}
public DestinationMetadata partnerOwned(Boolean partnerOwned) {
this.partnerOwned = partnerOwned;
return this;
}
/**
* Partner Owned flag.
* @return partnerOwned
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Partner Owned flag.")
public Boolean getPartnerOwned() {
return partnerOwned;
}
public void setPartnerOwned(Boolean partnerOwned) {
this.partnerOwned = partnerOwned;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
DestinationMetadata destinationMetadata = (DestinationMetadata) o;
return Objects.equals(this.id, destinationMetadata.id) &&
Objects.equals(this.name, destinationMetadata.name) &&
Objects.equals(this.description, destinationMetadata.description) &&
Objects.equals(this.slug, destinationMetadata.slug) &&
Objects.equals(this.logos, destinationMetadata.logos) &&
Objects.equals(this.options, destinationMetadata.options) &&
Objects.equals(this.status, destinationMetadata.status) &&
Objects.equals(this.previousNames, destinationMetadata.previousNames) &&
Objects.equals(this.categories, destinationMetadata.categories) &&
Objects.equals(this.website, destinationMetadata.website) &&
Objects.equals(this.components, destinationMetadata.components) &&
Objects.equals(this.supportedFeatures, destinationMetadata.supportedFeatures) &&
Objects.equals(this.supportedMethods, destinationMetadata.supportedMethods) &&
Objects.equals(this.supportedPlatforms, destinationMetadata.supportedPlatforms) &&
Objects.equals(this.actions, destinationMetadata.actions) &&
Objects.equals(this.presets, destinationMetadata.presets) &&
Objects.equals(this.contacts, destinationMetadata.contacts) &&
Objects.equals(this.partnerOwned, destinationMetadata.partnerOwned);
}
@Override
public int hashCode() {
return Objects.hash(id, name, description, slug, logos, options, status, previousNames, categories, website, components, supportedFeatures, supportedMethods, supportedPlatforms, actions, presets, contacts, partnerOwned);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class DestinationMetadata {\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" slug: ").append(toIndentedString(slug)).append("\n");
sb.append(" logos: ").append(toIndentedString(logos)).append("\n");
sb.append(" options: ").append(toIndentedString(options)).append("\n");
sb.append(" status: ").append(toIndentedString(status)).append("\n");
sb.append(" previousNames: ").append(toIndentedString(previousNames)).append("\n");
sb.append(" categories: ").append(toIndentedString(categories)).append("\n");
sb.append(" website: ").append(toIndentedString(website)).append("\n");
sb.append(" components: ").append(toIndentedString(components)).append("\n");
sb.append(" supportedFeatures: ").append(toIndentedString(supportedFeatures)).append("\n");
sb.append(" supportedMethods: ").append(toIndentedString(supportedMethods)).append("\n");
sb.append(" supportedPlatforms: ").append(toIndentedString(supportedPlatforms)).append("\n");
sb.append(" actions: ").append(toIndentedString(actions)).append("\n");
sb.append(" presets: ").append(toIndentedString(presets)).append("\n");
sb.append(" contacts: ").append(toIndentedString(contacts)).append("\n");
sb.append(" partnerOwned: ").append(toIndentedString(partnerOwned)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
public static HashSet openapiFields;
public static HashSet openapiRequiredFields;
static {
// a set of all properties/fields (JSON key names)
openapiFields = new HashSet();
openapiFields.add("id");
openapiFields.add("name");
openapiFields.add("description");
openapiFields.add("slug");
openapiFields.add("logos");
openapiFields.add("options");
openapiFields.add("status");
openapiFields.add("previousNames");
openapiFields.add("categories");
openapiFields.add("website");
openapiFields.add("components");
openapiFields.add("supportedFeatures");
openapiFields.add("supportedMethods");
openapiFields.add("supportedPlatforms");
openapiFields.add("actions");
openapiFields.add("presets");
openapiFields.add("contacts");
openapiFields.add("partnerOwned");
// a set of required properties/fields (JSON key names)
openapiRequiredFields = new HashSet();
openapiRequiredFields.add("id");
openapiRequiredFields.add("name");
openapiRequiredFields.add("description");
openapiRequiredFields.add("slug");
openapiRequiredFields.add("logos");
openapiRequiredFields.add("options");
openapiRequiredFields.add("status");
openapiRequiredFields.add("previousNames");
openapiRequiredFields.add("categories");
openapiRequiredFields.add("website");
openapiRequiredFields.add("components");
openapiRequiredFields.add("supportedFeatures");
openapiRequiredFields.add("supportedMethods");
openapiRequiredFields.add("supportedPlatforms");
openapiRequiredFields.add("actions");
openapiRequiredFields.add("presets");
}
/**
* Validates the JSON Object and throws an exception if issues found
*
* @param jsonObj JSON Object
* @throws IOException if the JSON Object is invalid with respect to DestinationMetadata
*/
public static void validateJsonObject(JsonObject jsonObj) throws IOException {
if (jsonObj == null) {
if (!DestinationMetadata.openapiRequiredFields.isEmpty()) { // has required fields but JSON object is null
throw new IllegalArgumentException(String.format("The required field(s) %s in DestinationMetadata is not found in the empty JSON string", DestinationMetadata.openapiRequiredFields.toString()));
}
}
Set> entries = jsonObj.entrySet();
// check to see if the JSON string contains additional fields
for (Entry entry : entries) {
if (!DestinationMetadata.openapiFields.contains(entry.getKey())) {
throw new IllegalArgumentException(String.format("The field `%s` in the JSON string is not defined in the `DestinationMetadata` properties. JSON: %s", entry.getKey(), jsonObj.toString()));
}
}
// check to make sure all required properties/fields are present in the JSON string
for (String requiredField : DestinationMetadata.openapiRequiredFields) {
if (jsonObj.get(requiredField) == null) {
throw new IllegalArgumentException(String.format("The required field `%s` is not found in the JSON string: %s", requiredField, jsonObj.toString()));
}
}
if (!jsonObj.get("id").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `id` to be a primitive type in the JSON string but got `%s`", jsonObj.get("id").toString()));
}
if (!jsonObj.get("name").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `name` to be a primitive type in the JSON string but got `%s`", jsonObj.get("name").toString()));
}
if (!jsonObj.get("description").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `description` to be a primitive type in the JSON string but got `%s`", jsonObj.get("description").toString()));
}
if (!jsonObj.get("slug").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `slug` to be a primitive type in the JSON string but got `%s`", jsonObj.get("slug").toString()));
}
// ensure the json data is an array
if (!jsonObj.get("options").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `options` to be an array in the JSON string but got `%s`", jsonObj.get("options").toString()));
}
JsonArray jsonArrayoptions = jsonObj.getAsJsonArray("options");
if (!jsonObj.get("status").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `status` to be a primitive type in the JSON string but got `%s`", jsonObj.get("status").toString()));
}
// ensure the required json array is present
if (jsonObj.get("previousNames") == null) {
throw new IllegalArgumentException("Expected the field `linkedContent` to be an array in the JSON string but got `null`");
} else if (!jsonObj.get("previousNames").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `previousNames` to be an array in the JSON string but got `%s`", jsonObj.get("previousNames").toString()));
}
// ensure the required json array is present
if (jsonObj.get("categories") == null) {
throw new IllegalArgumentException("Expected the field `linkedContent` to be an array in the JSON string but got `null`");
} else if (!jsonObj.get("categories").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `categories` to be an array in the JSON string but got `%s`", jsonObj.get("categories").toString()));
}
if (!jsonObj.get("website").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `website` to be a primitive type in the JSON string but got `%s`", jsonObj.get("website").toString()));
}
// ensure the json data is an array
if (!jsonObj.get("components").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `components` to be an array in the JSON string but got `%s`", jsonObj.get("components").toString()));
}
JsonArray jsonArraycomponents = jsonObj.getAsJsonArray("components");
// ensure the json data is an array
if (!jsonObj.get("actions").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `actions` to be an array in the JSON string but got `%s`", jsonObj.get("actions").toString()));
}
JsonArray jsonArrayactions = jsonObj.getAsJsonArray("actions");
// ensure the json data is an array
if (!jsonObj.get("presets").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `presets` to be an array in the JSON string but got `%s`", jsonObj.get("presets").toString()));
}
JsonArray jsonArraypresets = jsonObj.getAsJsonArray("presets");
if (jsonObj.get("contacts") != null && !jsonObj.get("contacts").isJsonNull()) {
JsonArray jsonArraycontacts = jsonObj.getAsJsonArray("contacts");
if (jsonArraycontacts != null) {
// ensure the json data is an array
if (!jsonObj.get("contacts").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `contacts` to be an array in the JSON string but got `%s`", jsonObj.get("contacts").toString()));
}
}
}
}
public static class CustomTypeAdapterFactory implements TypeAdapterFactory {
@SuppressWarnings("unchecked")
@Override
public TypeAdapter create(Gson gson, TypeToken type) {
if (!DestinationMetadata.class.isAssignableFrom(type.getRawType())) {
return null; // this class only serializes 'DestinationMetadata' and its subtypes
}
final TypeAdapter elementAdapter = gson.getAdapter(JsonElement.class);
final TypeAdapter thisAdapter
= gson.getDelegateAdapter(this, TypeToken.get(DestinationMetadata.class));
return (TypeAdapter) new TypeAdapter() {
@Override
public void write(JsonWriter out, DestinationMetadata value) throws IOException {
JsonObject obj = thisAdapter.toJsonTree(value).getAsJsonObject();
elementAdapter.write(out, obj);
}
@Override
public DestinationMetadata read(JsonReader in) throws IOException {
JsonObject jsonObj = elementAdapter.read(in).getAsJsonObject();
validateJsonObject(jsonObj);
return thisAdapter.fromJsonTree(jsonObj);
}
}.nullSafe();
}
}
/**
* Create an instance of DestinationMetadata given an JSON string
*
* @param jsonString JSON string
* @return An instance of DestinationMetadata
* @throws IOException if the JSON string is invalid with respect to DestinationMetadata
*/
public static DestinationMetadata fromJson(String jsonString) throws IOException {
return JSON.getGson().fromJson(jsonString, DestinationMetadata.class);
}
/**
* Convert an instance of DestinationMetadata to an JSON string
*
* @return JSON string
*/
public String toJson() {
return JSON.getGson().toJson(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy