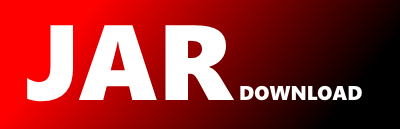
com.segment.publicapi.models.SyncV1 Maven / Gradle / Ivy
/*
* Segment Public API
* The Segment Public API helps you manage your Segment Workspaces and its resources. You can use the API to perform CRUD (create, read, update, delete) operations at no extra charge. This includes working with resources such as Sources, Destinations, Warehouses, Tracking Plans, and the Segment Destinations and Sources Catalogs. All CRUD endpoints in the API follow REST conventions and use standard HTTP methods. Different URL endpoints represent different resources in a Workspace. See the next sections for more information on how to use the Segment Public API.
*
* The version of the OpenAPI document: 32.0.3
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.segment.publicapi.models;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.segment.publicapi.models.SyncNoticeV1;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.math.BigDecimal;
import java.util.ArrayList;
import java.util.List;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.JsonArray;
import com.google.gson.JsonDeserializationContext;
import com.google.gson.JsonDeserializer;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.JsonParseException;
import com.google.gson.TypeAdapterFactory;
import com.google.gson.reflect.TypeToken;
import java.lang.reflect.Type;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Set;
import com.segment.publicapi.JSON;
/**
* Represents a sync between a Source and Warehouse. A sync occurs when data from a Source is loaded into a Warehouse.
*/
@ApiModel(description = "Represents a sync between a Source and Warehouse. A sync occurs when data from a Source is loaded into a Warehouse.")
public class SyncV1 {
public static final String SERIALIZED_NAME_SOURCE_ID = "sourceId";
@SerializedName(SERIALIZED_NAME_SOURCE_ID)
private String sourceId;
public static final String SERIALIZED_NAME_START = "start";
@SerializedName(SERIALIZED_NAME_START)
private String start;
public static final String SERIALIZED_NAME_END = "end";
@SerializedName(SERIALIZED_NAME_END)
private String end;
public static final String SERIALIZED_NAME_STATUS = "status";
@SerializedName(SERIALIZED_NAME_STATUS)
private String status;
public static final String SERIALIZED_NAME_DURATION = "duration";
@SerializedName(SERIALIZED_NAME_DURATION)
private BigDecimal duration;
public static final String SERIALIZED_NAME_HUMAN_DURATION = "humanDuration";
@SerializedName(SERIALIZED_NAME_HUMAN_DURATION)
private String humanDuration;
public static final String SERIALIZED_NAME_COUNT = "count";
@SerializedName(SERIALIZED_NAME_COUNT)
private BigDecimal count;
public static final String SERIALIZED_NAME_NOTICES = "notices";
@SerializedName(SERIALIZED_NAME_NOTICES)
private List notices = new ArrayList<>();
public SyncV1() {
}
public SyncV1 sourceId(String sourceId) {
this.sourceId = sourceId;
return this;
}
/**
* The id of the Source loaded in the sync.
* @return sourceId
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "The id of the Source loaded in the sync.")
public String getSourceId() {
return sourceId;
}
public void setSourceId(String sourceId) {
this.sourceId = sourceId;
}
public SyncV1 start(String start) {
this.start = start;
return this;
}
/**
* The start time of the sync.
* @return start
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "The start time of the sync.")
public String getStart() {
return start;
}
public void setStart(String start) {
this.start = start;
}
public SyncV1 end(String end) {
this.end = end;
return this;
}
/**
* The time the sync completed. Returns null if unfinished.
* @return end
**/
@javax.annotation.Nullable
@ApiModelProperty(required = true, value = "The time the sync completed. Returns null if unfinished.")
public String getEnd() {
return end;
}
public void setEnd(String end) {
this.end = end;
}
public SyncV1 status(String status) {
this.status = status;
return this;
}
/**
* The status of the sync.
* @return status
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "The status of the sync.")
public String getStatus() {
return status;
}
public void setStatus(String status) {
this.status = status;
}
public SyncV1 duration(BigDecimal duration) {
this.duration = duration;
return this;
}
/**
* The duration of the sync in seconds. Returns the partial duration if the sync has not finished yet.
* @return duration
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "The duration of the sync in seconds. Returns the partial duration if the sync has not finished yet.")
public BigDecimal getDuration() {
return duration;
}
public void setDuration(BigDecimal duration) {
this.duration = duration;
}
public SyncV1 humanDuration(String humanDuration) {
this.humanDuration = humanDuration;
return this;
}
/**
* The human-readable counterpart of `duration`.
* @return humanDuration
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "The human-readable counterpart of `duration`.")
public String getHumanDuration() {
return humanDuration;
}
public void setHumanDuration(String humanDuration) {
this.humanDuration = humanDuration;
}
public SyncV1 count(BigDecimal count) {
this.count = count;
return this;
}
/**
* The number of rows synced into the Warehouse.
* @return count
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "The number of rows synced into the Warehouse.")
public BigDecimal getCount() {
return count;
}
public void setCount(BigDecimal count) {
this.count = count;
}
public SyncV1 notices(List notices) {
this.notices = notices;
return this;
}
public SyncV1 addNoticesItem(SyncNoticeV1 noticesItem) {
this.notices.add(noticesItem);
return this;
}
/**
* Notices that contain the events that occurred during the sync.
* @return notices
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "Notices that contain the events that occurred during the sync.")
public List getNotices() {
return notices;
}
public void setNotices(List notices) {
this.notices = notices;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
SyncV1 syncV1 = (SyncV1) o;
return Objects.equals(this.sourceId, syncV1.sourceId) &&
Objects.equals(this.start, syncV1.start) &&
Objects.equals(this.end, syncV1.end) &&
Objects.equals(this.status, syncV1.status) &&
Objects.equals(this.duration, syncV1.duration) &&
Objects.equals(this.humanDuration, syncV1.humanDuration) &&
Objects.equals(this.count, syncV1.count) &&
Objects.equals(this.notices, syncV1.notices);
}
@Override
public int hashCode() {
return Objects.hash(sourceId, start, end, status, duration, humanDuration, count, notices);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class SyncV1 {\n");
sb.append(" sourceId: ").append(toIndentedString(sourceId)).append("\n");
sb.append(" start: ").append(toIndentedString(start)).append("\n");
sb.append(" end: ").append(toIndentedString(end)).append("\n");
sb.append(" status: ").append(toIndentedString(status)).append("\n");
sb.append(" duration: ").append(toIndentedString(duration)).append("\n");
sb.append(" humanDuration: ").append(toIndentedString(humanDuration)).append("\n");
sb.append(" count: ").append(toIndentedString(count)).append("\n");
sb.append(" notices: ").append(toIndentedString(notices)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
public static HashSet openapiFields;
public static HashSet openapiRequiredFields;
static {
// a set of all properties/fields (JSON key names)
openapiFields = new HashSet();
openapiFields.add("sourceId");
openapiFields.add("start");
openapiFields.add("end");
openapiFields.add("status");
openapiFields.add("duration");
openapiFields.add("humanDuration");
openapiFields.add("count");
openapiFields.add("notices");
// a set of required properties/fields (JSON key names)
openapiRequiredFields = new HashSet();
openapiRequiredFields.add("sourceId");
openapiRequiredFields.add("start");
openapiRequiredFields.add("end");
openapiRequiredFields.add("status");
openapiRequiredFields.add("duration");
openapiRequiredFields.add("humanDuration");
openapiRequiredFields.add("count");
openapiRequiredFields.add("notices");
}
/**
* Validates the JSON Object and throws an exception if issues found
*
* @param jsonObj JSON Object
* @throws IOException if the JSON Object is invalid with respect to SyncV1
*/
public static void validateJsonObject(JsonObject jsonObj) throws IOException {
if (jsonObj == null) {
if (!SyncV1.openapiRequiredFields.isEmpty()) { // has required fields but JSON object is null
throw new IllegalArgumentException(String.format("The required field(s) %s in SyncV1 is not found in the empty JSON string", SyncV1.openapiRequiredFields.toString()));
}
}
Set> entries = jsonObj.entrySet();
// check to see if the JSON string contains additional fields
for (Entry entry : entries) {
if (!SyncV1.openapiFields.contains(entry.getKey())) {
throw new IllegalArgumentException(String.format("The field `%s` in the JSON string is not defined in the `SyncV1` properties. JSON: %s", entry.getKey(), jsonObj.toString()));
}
}
// check to make sure all required properties/fields are present in the JSON string
for (String requiredField : SyncV1.openapiRequiredFields) {
if (jsonObj.get(requiredField) == null) {
throw new IllegalArgumentException(String.format("The required field `%s` is not found in the JSON string: %s", requiredField, jsonObj.toString()));
}
}
if (!jsonObj.get("sourceId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `sourceId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("sourceId").toString()));
}
if (!jsonObj.get("start").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `start` to be a primitive type in the JSON string but got `%s`", jsonObj.get("start").toString()));
}
if (!jsonObj.get("end").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `end` to be a primitive type in the JSON string but got `%s`", jsonObj.get("end").toString()));
}
if (!jsonObj.get("status").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `status` to be a primitive type in the JSON string but got `%s`", jsonObj.get("status").toString()));
}
if (!jsonObj.get("humanDuration").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `humanDuration` to be a primitive type in the JSON string but got `%s`", jsonObj.get("humanDuration").toString()));
}
// ensure the json data is an array
if (!jsonObj.get("notices").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `notices` to be an array in the JSON string but got `%s`", jsonObj.get("notices").toString()));
}
JsonArray jsonArraynotices = jsonObj.getAsJsonArray("notices");
}
public static class CustomTypeAdapterFactory implements TypeAdapterFactory {
@SuppressWarnings("unchecked")
@Override
public TypeAdapter create(Gson gson, TypeToken type) {
if (!SyncV1.class.isAssignableFrom(type.getRawType())) {
return null; // this class only serializes 'SyncV1' and its subtypes
}
final TypeAdapter elementAdapter = gson.getAdapter(JsonElement.class);
final TypeAdapter thisAdapter
= gson.getDelegateAdapter(this, TypeToken.get(SyncV1.class));
return (TypeAdapter) new TypeAdapter() {
@Override
public void write(JsonWriter out, SyncV1 value) throws IOException {
JsonObject obj = thisAdapter.toJsonTree(value).getAsJsonObject();
elementAdapter.write(out, obj);
}
@Override
public SyncV1 read(JsonReader in) throws IOException {
JsonObject jsonObj = elementAdapter.read(in).getAsJsonObject();
validateJsonObject(jsonObj);
return thisAdapter.fromJsonTree(jsonObj);
}
}.nullSafe();
}
}
/**
* Create an instance of SyncV1 given an JSON string
*
* @param jsonString JSON string
* @return An instance of SyncV1
* @throws IOException if the JSON string is invalid with respect to SyncV1
*/
public static SyncV1 fromJson(String jsonString) throws IOException {
return JSON.getGson().fromJson(jsonString, SyncV1.class);
}
/**
* Convert an instance of SyncV1 to an JSON string
*
* @return JSON string
*/
public String toJson() {
return JSON.getGson().toJson(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy