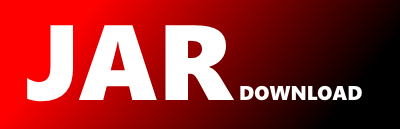
org.ioc.commons.impl.android.flowcontrol.actioncontroller.AndroidActionControllerImpl Maven / Gradle / Ivy
package org.ioc.commons.impl.android.flowcontrol.actioncontroller;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Map;
import java.util.Set;
import org.ioc.commons.flowcontrol.actioncontroller.ActionController;
import org.ioc.commons.flowcontrol.actioncontroller.ActionHandler;
import org.ioc.commons.flowcontrol.actioncontroller.IsExternalAction;
import org.ioc.commons.flowcontrol.common.BindRegistration;
import org.ioc.commons.utils.FormatterLogger;
import android.content.BroadcastReceiver;
import android.content.Context;
import android.content.Intent;
import android.content.IntentFilter;
import android.os.Bundle;
public class AndroidActionControllerImpl> implements ActionController {
private static final FormatterLogger logger = FormatterLogger.getLogger(AndroidActionControllerImpl.class);
private static final String ACTION_INTENT_ACTION = "org.ioc.commons.impl.android.flowcontrol.actioncontroller.ACTION";
private static final String ACTION_KEY = "ACTION";
private static final String ACTION_PARAMETERS_KEY = "ACTION_PARAMETERS";
private class AndroidActionControllerBindRegistration implements BindRegistration {
private final Context context;
private ActionStore store;
public AndroidActionControllerBindRegistration(Context context, ActionStore store) {
this.context = context;
this.store = store;
}
@Override
public void unbind() {
stored.remove(this.store);
this.context.unregisterReceiver(this.store.receiver);
}
}
private static class ActionReceiver> extends BroadcastReceiver {
private final ActionHandler handler;
private final Map, BindRegistration> onceHandlers;
private A action;
public ActionReceiver(A action, ActionHandler handler, Map, BindRegistration> onceHandlers) {
this.action = action;
this.handler = handler;
this.onceHandlers = onceHandlers;
}
@Override
public void onReceive(Context context, Intent intent) {
@SuppressWarnings("unchecked")
A action = (A) intent.getSerializableExtra(ACTION_KEY);
if (this.action.equals(action)) {
if (!onceHandlers.isEmpty()) {
BindRegistration registration = onceHandlers.remove(handler);
if (registration != null) {
registration.unbind();
}
}
handler.handleAction(context, action, intent.getSerializableExtra(ACTION_PARAMETERS_KEY));
}
}
}
private final Map, BindRegistration> onceHandlers = new HashMap, BindRegistration>();
private class ActionStore {
IntentFilter filter;
ActionReceiver receiver;
}
private final Context context;
private final Set stored = new HashSet<>();
private boolean active;
public AndroidActionControllerImpl(Context context, boolean initiallyActive) {
this.context = context;
this.active = initiallyActive;
}
@Override
public BindRegistration bindAction(A action, ActionHandler handler) {
IntentFilter filter = new IntentFilter(ACTION_INTENT_ACTION);
ActionReceiver receiver = new ActionReceiver(action, handler, onceHandlers);
AndroidActionControllerImpl.ActionStore as = addActionReceiver(context, filter, receiver);
return new AndroidActionControllerBindRegistration(context, as);
}
private ActionStore addActionReceiver(Context context, IntentFilter filter, ActionReceiver receiver) {
ActionStore as = new ActionStore();
as.filter = filter;
as.receiver = receiver;
stored.add(as);
if (this.active) {
context.registerReceiver(receiver, filter);
}
return as;
}
@Override
public BindRegistration bindActionOnce(A action, ActionHandler handler) {
BindRegistration registration = bindAction(action, handler);
this.onceHandlers.put(handler, registration);
return registration;
}
@Override
public void invokeAction(A action, Object... params) {
Intent intent = new Intent(ACTION_INTENT_ACTION);
intent.putExtra(ACTION_KEY, action);
intent.putExtra(ACTION_PARAMETERS_KEY, params);
context.sendBroadcast(intent);
}
public void onCreate() {
/*
* Nothing to do
*/
}
public void onStart() {
for (AndroidActionControllerImpl.ActionStore os : this.stored) {
context.registerReceiver(os.receiver, os.filter);
}
}
public void onResume() {
this.active = true;
}
public void onPause() {
this.active = false;
}
public void onStop() {
for (AndroidActionControllerImpl.ActionStore os : this.stored) {
try {
context.unregisterReceiver(os.receiver);
} catch (Exception e) {
logger.warn(e, "Failure during unregistering reciver. Ignoring it.");
}
}
}
public void onDestroy() {
/*
* Nothing to do
*/
}
public void onActivityCreated(Bundle savedInstanceState) {
/*
* Nothing to do
*/
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy