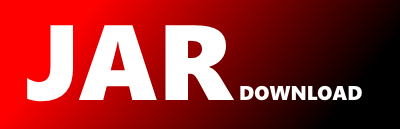
org.ioc.commons.impl.android.flowcontrol.activity.ViewActivity Maven / Gradle / Ivy
package org.ioc.commons.impl.android.flowcontrol.activity;
import java.util.HashMap;
import java.util.Map;
import org.ioc.commons.flowcontrol.actioncontroller.ActionController;
import org.ioc.commons.flowcontrol.actioncontroller.ActionControllerBinder;
import org.ioc.commons.flowcontrol.actioncontroller.IsExternalAction;
import org.ioc.commons.flowcontrol.eventbus.EventBus;
import org.ioc.commons.flowcontrol.eventbus.EventBusBinder;
import org.ioc.commons.flowcontrol.eventbus.IsEvent;
import org.ioc.commons.flowcontrol.operationmanager.IsOperation;
import org.ioc.commons.flowcontrol.operationmanager.OperationManager;
import org.ioc.commons.flowcontrol.operationmanager.OperationManagerBinder;
import org.ioc.commons.impl.android.flowcontrol.AndroidFlowController;
import org.ioc.commons.ui.HasStorage;
import org.ioc.commons.ui.IsView;
import org.ioc.commons.ui.IsViewPresenter;
import org.ioc.commons.ui.IsWidget;
import android.app.Activity;
import android.content.Context;
import android.view.HasContext;
public class ViewActivity, O extends Enum extends IsOperation>, A extends Enum extends IsExternalAction>, P extends IsViewPresenter>
extends Activity implements IsView, HasStorage, HasContext {
protected final AndroidFlowController flowController = new AndroidFlowController(this, false);
protected final EventBus eventbus = flowController.eventBus();
protected final OperationManager operationManager = flowController.operationManager();
protected final ActionController actionController = flowController.actionController();
private final Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy