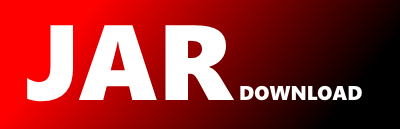
org.ioc.commons.impl.android.i18n.AndroidDateTimeFormatter Maven / Gradle / Ivy
package org.ioc.commons.impl.android.i18n;
import java.text.DateFormat;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.Date;
import java.util.TimeZone;
import org.ioc.commons.i18n.DateTimeFormatter;
import org.ioc.commons.utils.Format;
public class AndroidDateTimeFormatter implements DateTimeFormatter {
@Override
public String format(SystemDefaultFormat format, Date date) {
switch (format) {
case Date:
return DateFormat.getDateInstance().format(date);
case DateTime:
return DateFormat.getDateTimeInstance().format(date);
case Time:
return DateFormat.getTimeInstance().format(date);
}
throw new IllegalArgumentException(Format.substitute("The {0} {1} is not recognized.",
SystemDefaultFormat.class.getName(), format));
}
@Override
public Date parse(SystemDefaultFormat format, String stringDate) {
try {
switch (format) {
case Date:
return DateFormat.getDateInstance().parse(stringDate);
case DateTime:
return DateFormat.getDateTimeInstance().parse(stringDate);
case Time:
return DateFormat.getTimeInstance().parse(stringDate);
}
} catch (ParseException e) {
throw new RuntimeException(e);
}
throw new IllegalArgumentException(Format.substitute("The {0} {1} is not recognized.",
SystemDefaultFormat.class.getName(), format));
}
@Override
public String format(SystemDefaultFormat format, Date date, int timeZoneOffsetInMinutes) {
TimeZone timeZone = TimeZone.getTimeZone("GMT+" + toHour(timeZoneOffsetInMinutes));
switch (format) {
case Date:
DateFormat dateInstance = DateFormat.getDateInstance();
dateInstance.setTimeZone(timeZone);
return dateInstance.format(date);
case DateTime:
DateFormat dateTimeInstance = DateFormat.getDateTimeInstance();
dateTimeInstance.setTimeZone(timeZone);
return dateTimeInstance.format(date);
case Time:
DateFormat timeInstance = DateFormat.getTimeInstance();
timeInstance.setTimeZone(timeZone);
return timeInstance.format(date);
}
throw new IllegalArgumentException(Format.substitute("The {0} {1} is not recognized.",
SystemDefaultFormat.class.getName(), format));
}
@Override
public String format(PredefinedFormat format, Date date) {
switch (format) {
case DAY:
return new SimpleDateFormat("d").format(date);
case HOUR24_MINUTE:
return new SimpleDateFormat("HH:mm").format(date);
case HOUR24_MINUTE_SECOND:
return new SimpleDateFormat("HH:mm:ss").format(date);
case HOUR_MINUTE:
return new SimpleDateFormat("hh:mm").format(date);
case HOUR_MINUTE_SECOND:
return new SimpleDateFormat("hh:mm:ss").format(date);
case MINUTE_SECOND:
return new SimpleDateFormat("mm:ss").format(date);
case MONTH:
return new SimpleDateFormat("MM").format(date);
case MONTH_DAY:
return new SimpleDateFormat("MM-dd").format(date);
case MONTH_NUM_DAY:
return new SimpleDateFormat("MM-D").format(date);
case MONTH_WEEKDAY_DAY:
return new SimpleDateFormat("MM-u").format(date);
case YEAR:
return new SimpleDateFormat("yyyy").format(date);
case YEAR_MONTH:
return new SimpleDateFormat("yyyy-MM").format(date);
case YEAR_MONTH_DAY:
return new SimpleDateFormat("yyyy-MM-dd").format(date);
case YEAR_MONTH_NUM:
return new SimpleDateFormat("yyyy-MM-D").format(date);
case YEAR_MONTH_NUM_DAY:
return new SimpleDateFormat("yyyy-MM-D").format(date);
case YEAR_MONTH_WEEKDAY_DAY:
return new SimpleDateFormat("yyyy-MM-u").format(date);
}
throw new IllegalArgumentException(Format.substitute("The {0} {1} is not recognized.",
SystemDefaultFormat.class.getName(), format));
}
@Override
public Date parse(PredefinedFormat format, String stringDate) {
try {
switch (format) {
case DAY:
return new SimpleDateFormat("d").parse(stringDate);
case HOUR24_MINUTE:
return new SimpleDateFormat("HH:mm").parse(stringDate);
case HOUR24_MINUTE_SECOND:
return new SimpleDateFormat("HH:mm:ss").parse(stringDate);
case HOUR_MINUTE:
return new SimpleDateFormat("hh:mm").parse(stringDate);
case HOUR_MINUTE_SECOND:
return new SimpleDateFormat("hh:mm:ss").parse(stringDate);
case MINUTE_SECOND:
return new SimpleDateFormat("mm:ss").parse(stringDate);
case MONTH:
return new SimpleDateFormat("MM").parse(stringDate);
case MONTH_DAY:
return new SimpleDateFormat("MM-dd").parse(stringDate);
case MONTH_NUM_DAY:
return new SimpleDateFormat("MM-D").parse(stringDate);
case MONTH_WEEKDAY_DAY:
return new SimpleDateFormat("MM-u").parse(stringDate);
case YEAR:
return new SimpleDateFormat("yyyy").parse(stringDate);
case YEAR_MONTH:
return new SimpleDateFormat("yyyy-MM").parse(stringDate);
case YEAR_MONTH_DAY:
return new SimpleDateFormat("yyyy-MM-dd").parse(stringDate);
case YEAR_MONTH_NUM:
return new SimpleDateFormat("yyyy-MM-D").parse(stringDate);
case YEAR_MONTH_NUM_DAY:
return new SimpleDateFormat("yyyy-MM-D").parse(stringDate);
case YEAR_MONTH_WEEKDAY_DAY:
return new SimpleDateFormat("yyyy-MM-u").parse(stringDate);
}
} catch (ParseException e) {
throw new RuntimeException(e);
}
throw new IllegalArgumentException(Format.substitute("The {0} {1} is not recognized.",
SystemDefaultFormat.class.getName(), format));
}
@Override
public String format(PredefinedFormat format, Date date, int timeZoneOffsetInMinutes) {
SimpleDateFormat yearMonthNum = new SimpleDateFormat("yyyy-MM-D");
TimeZone timezone = TimeZone.getTimeZone("GMT+" + toHour(timeZoneOffsetInMinutes));
switch (format) {
case DAY:
SimpleDateFormat day = new SimpleDateFormat("d");
day.setTimeZone(timezone);
return day.format(date);
case HOUR24_MINUTE:
SimpleDateFormat hour24minute = new SimpleDateFormat("HH:mm");
hour24minute.setTimeZone(timezone);
return hour24minute.format(date);
case HOUR24_MINUTE_SECOND:
SimpleDateFormat hour24minuteSecond = new SimpleDateFormat("HH:mm:ss");
hour24minuteSecond.setTimeZone(timezone);
return hour24minuteSecond.format(date);
case HOUR_MINUTE:
SimpleDateFormat hourMinute = new SimpleDateFormat("hh:mm");
hourMinute.setTimeZone(timezone);
return hourMinute.format(date);
case HOUR_MINUTE_SECOND:
SimpleDateFormat hourMinuteSecond = new SimpleDateFormat("hh:mm:ss");
hourMinuteSecond.setTimeZone(timezone);
return hourMinuteSecond.format(date);
case MINUTE_SECOND:
SimpleDateFormat minuteSecond = new SimpleDateFormat("mm:ss");
minuteSecond.setTimeZone(timezone);
return minuteSecond.format(date);
case MONTH:
SimpleDateFormat month = new SimpleDateFormat("MM");
month.setTimeZone(timezone);
return month.format(date);
case MONTH_DAY:
SimpleDateFormat monthDay = new SimpleDateFormat("MM-dd");
monthDay.setTimeZone(timezone);
return monthDay.format(date);
case MONTH_NUM_DAY:
SimpleDateFormat monthNumDay = new SimpleDateFormat("MM-D");
monthNumDay.setTimeZone(timezone);
return monthNumDay.format(date);
case MONTH_WEEKDAY_DAY:
SimpleDateFormat monthWeekday = new SimpleDateFormat("MM-u");
monthWeekday.setTimeZone(timezone);
return monthWeekday.format(date);
case YEAR:
SimpleDateFormat year = new SimpleDateFormat("yyyy");
year.setTimeZone(timezone);
return year.format(date);
case YEAR_MONTH:
SimpleDateFormat yearMonth = new SimpleDateFormat("yyyy-MM");
yearMonth.setTimeZone(timezone);
return yearMonth.format(date);
case YEAR_MONTH_DAY:
SimpleDateFormat yearMonthDay = new SimpleDateFormat("yyyy-MM-dd");
yearMonthDay.setTimeZone(timezone);
return yearMonthDay.format(date);
case YEAR_MONTH_NUM:
yearMonthNum.setTimeZone(timezone);
return yearMonthNum.format(date);
case YEAR_MONTH_NUM_DAY:
yearMonthNum.setTimeZone(timezone);
return yearMonthNum.format(date);
case YEAR_MONTH_WEEKDAY_DAY:
SimpleDateFormat yearMonthWeekday = new SimpleDateFormat("yyyy-MM-u");
return yearMonthWeekday.format(date);
}
throw new IllegalArgumentException(Format.substitute("The {0} {1} is not recognized.",
SystemDefaultFormat.class.getName(), format));
}
private String toHour(int t) {
int hours = t / 60; // since both are ints, you get an int
int minutes = t % 60;
return String.format("%d:%02d", hours, minutes);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy