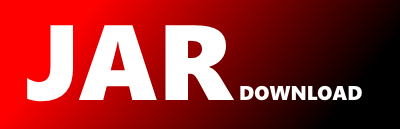
org.ioc.commons.impl.android.integration.service.AndroidRequestManagerWrapper Maven / Gradle / Ivy
package org.ioc.commons.impl.android.integration.service;
import java.lang.reflect.Method;
import java.util.Map;
import org.ioc.commons.flowcontrol.actioncontroller.IsExternalAction;
import org.ioc.commons.flowcontrol.common.Receiver;
import org.ioc.commons.flowcontrol.eventbus.IsEvent;
import org.ioc.commons.flowcontrol.operationmanager.IsOperation;
import org.ioc.commons.flowcontrol.operationmanager.OperationManagerTrigger;
import org.ioc.commons.integration.common.RequestManager;
import org.ioc.commons.ui.HasDisplay;
import org.ioc.commons.ui.IsDisplay;
import android.app.Activity;
import android.app.Fragment;
import android.content.Context;
import android.os.Handler;
import android.os.Looper;
import android.view.HasContext;
import android.view.View;
public abstract class AndroidRequestManagerWrapper implements RequestManager {
// private static final FormatterLogger logger = FormatterLogger.getLogger(AndroidRequestManagerWrapper.class);
private static final long MAX_TRIES = 10l;
private int tries = 0;
public static class OperationTrigger> {
private O operation;
private OperationManagerTrigger trigger;
public OperationTrigger(O operation, OperationManagerTrigger trigger) {
this.operation = operation;
this.trigger = trigger;
}
public void operationBeginning() {
this.trigger.operationBeginning(operation);
}
public void operationFinished(boolean success) {
this.trigger.operationFinished(operation, success);
}
}
private OperationTrigger extends Enum extends IsOperation>> operationTrigger;
private Receiver callback;
private String queueName;
private String[] requiredProperties;
protected final Map queueRequests;
private Object caller;
public AndroidRequestManagerWrapper(Map queueRequests) {
this.queueRequests = queueRequests;
scheduleFire(1l/* false */);
}
@Override
public RequestManager to(Receiver callback) {
this.callback = callback;
return this;
}
@Override
public > RequestManager performing(O operation,
OperationManagerTrigger trigger) {
return performing(new OperationTrigger(operation, trigger));
}
private > RequestManager performing(OperationTrigger operationTrigger) {
this.operationTrigger = operationTrigger;
return this;
}
public OperationTrigger extends Enum extends IsOperation>> getOperaTrigger() {
return operationTrigger;
}
public Receiver getCallback() {
return callback;
}
/*
* (non-Javadoc)
*
* @see
* org.ioc.commons.integration.common.RequestManager#with(java.lang.String
* [])
*/
@Override
public RequestManager with(String... requiredProperties) {
/*
* This operation doesn't have any effect on pure RpcService since all
* properties are serialized, but is used by JAPTSYService based on
* RpcService.
*/
this.requiredProperties = requiredProperties;
return this;
}
@Override
public RequestManager queue(String queueName) {
this.queueName = queueName;
return this;
}
public String getQueueName() {
return queueName;
}
public String[] getRequiredProperties() {
return requiredProperties;
}
private void scheduleFire(final long delayMillis/* boolean useHandlerThread */) {
Handler handler = null;
/*
* if (useHandlerThread) { HandlerThread thread = new
* HandlerThread(this.getClass().getSimpleName() + "_" +
* UUID.randomUUID()); thread.start(); handler = new
* Handler(thread.getLooper()); } else {
*/
handler = new Handler(Looper.getMainLooper());
// }
boolean posted = handler.postDelayed(new Runnable() {
@Override
public void run() {
if (getCaller() != null || tries > MAX_TRIES) {
if (AndroidRequestManagerWrapper.this.getOperaTrigger() != null) {
AndroidRequestManagerWrapper.this.getOperaTrigger().operationBeginning();
}
fire();
} else {
tries++;
scheduleFire(delayMillis + 1);
}
}
}, delayMillis);
if (!posted) {
handleFail(null);
}
}
protected abstract void fire();
public void handleSuccess(T result) {
Receiver callback = this.getCallback();
OperationTrigger extends Enum extends IsOperation>> operaTrigger = this.getOperaTrigger();
String queueName = this.getQueueName();
if (operaTrigger != null) {
operaTrigger.operationFinished(true);
}
if (callback != null) {
callback.onEnd(true);
callback.success(result);
}
if (this.getQueueName() != null && !this.getQueueName().isEmpty()) {
CommandQueue rq = queueRequests.get(queueName);
if (rq != null) {
rq.fireNext();
}
}
}
public void handleFail(Throwable e) {
Receiver callback = this.getCallback();
OperationTrigger extends Enum extends IsOperation>> operaTrigger = this.getOperaTrigger();
String queueName = this.getQueueName();
if (operaTrigger != null) {
operaTrigger.operationFinished(false);
}
if (callback != null) {
callback.onEnd(false);
callback.failure(e);
}
if (this.getQueueName() != null && !this.getQueueName().isEmpty()) {
CommandQueue rq = queueRequests.get(queueName);
if (rq != null && !rq.isCancelNextRequestsOnFail()) {
rq.fireNext();
}
}
}
@Override
public RequestManager caller(Object caller) {
// Object isOrHasContext = caller;
//
// if (isOrHasContext instanceof HasDisplay) {
// HasDisplay, ?, ?, ?> hasDisplay = (HasDisplay, ?, ?, ?>)
// isOrHasContext;
//
// IsDisplay extends Enum extends IsEvent>, ? extends Enum extends
// IsOperation>, ? extends Enum extends IsExternalAction>> display =
// hasDisplay
// .getDisplay();
//
// if (display == null) {
// throw new
// AndroidRequestManagerException("Got an empty display from the caller");
// }
//
// isOrHasContext = display;
// }
//
// if (isOrHasContext instanceof IsDisplay) {
// Object nativeWidget = ((IsDisplay extends Enum extends IsEvent>,
// ? extends Enum extends IsOperation>, ? extends Enum extends
// IsExternalAction>>) isOrHasContext)
// .asWidget();
//
// if (nativeWidget == null) {
// throw new
// AndroidRequestManagerException("Got an empty native widget from display "
// + nativeWidget);
// }
//
// isOrHasContext = nativeWidget;
// }
//
// if (isOrHasContext instanceof Context) {
// context = (Context) isOrHasContext;
// } else if (isOrHasContext instanceof HasContext) {
// context = ((HasContext) isOrHasContext).getContext();
// } else if (isOrHasContext instanceof View) {
// context = ((View) isOrHasContext).getContext();
// } else if (isOrHasContext instanceof android.support.v4.app.Fragment)
// {
// context = ((android.support.v4.app.Fragment)
// isOrHasContext).getActivity();
// } else if (isOrHasContext instanceof Fragment) {
// context = ((Fragment) isOrHasContext).getActivity();
// }
//
if (caller == null) {
throw new IllegalArgumentException("Caller cannot be null");
}
this.caller = caller;
return this;
}
protected Context getContext() {
return getContext(null);
}
protected Context getContext(Method serviceMethod) {
Object caller = getCaller();
if (caller == null) {
String detailMessage = "IOC-Commons services Android implementation requires to invoke "
+ RequestManager.class.getSimpleName() + ".caller() method passing 'this' to get the context.";
if (serviceMethod != null) {
detailMessage += " Invoked from " + serviceMethod;
} else if (this.getClass().getEnclosingMethod() != null) {
detailMessage += " Invoked from " + this.getClass().getEnclosingMethod();
}
throw new AndroidRequestManagerException(detailMessage);
}
return HasContext.Helper.getFromObject(caller, serviceMethod);
}
protected Object getCaller() {
return caller;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy