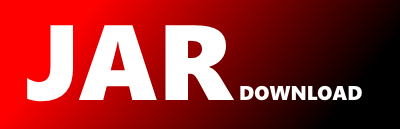
org.ioc.commons.impl.android.ui.HasEnabledWrapper Maven / Gradle / Ivy
package org.ioc.commons.impl.android.ui;
import org.ioc.commons.ui.HasEnabled;
import org.ioc.commons.ui.HasStorage;
import android.view.MenuItem;
import android.view.View;
public class HasEnabledWrapper implements HasEnabled {
private final View wrappedView;
private final MenuItem wrappedMenu;
private HasEnabledWrapper(View wrapped) {
this.wrappedView = wrapped;
this.wrappedMenu = null;
}
private HasEnabledWrapper(MenuItem wrapped) {
this.wrappedView = null;
this.wrappedMenu = wrapped;
}
@Override
public void setEnabled(boolean enabled) {
if (this.wrappedView != null) {
this.wrappedView.setEnabled(enabled);
} else {
this.wrappedMenu.setEnabled(enabled);
}
}
@Override
public boolean isEnabled() {
return this.wrappedView != null ? this.wrappedView.isEnabled() : this.wrappedMenu.isEnabled();
}
/**
* Gets a wrapper for a {@link View}
*
* @param view
*
* @return HasEnabled wrapper
*/
public static HasEnabled from(View view) {
return new HasEnabledWrapper(view);
}
public static HasEnabled from(View view, HasStorage cache) {
Object cached = cache.retrieve(view);
HasEnabled hasEnabled = (cached instanceof HasEnabled) ? (HasEnabled) cached : null;
if (hasEnabled == null) {
hasEnabled = new HasEnabledWrapper(view);
cache.store(view, hasEnabled);
}
return hasEnabled;
}
/**
* Helpful method for setting an enabled property on several components at
* the same time.
*
* @param enabled
* @param viewArray
*/
public static void setEnabled(boolean enabled, View... viewArray) {
if (viewArray != null) {
for (View view : viewArray) {
view.setEnabled(enabled);
}
}
}
/**
* Gets a wrapper for a {@link MenuItem}
*
* @param view
*
* @return HasEnabled wrapper
*/
public static HasEnabled from(MenuItem menu) {
return new HasEnabledWrapper(menu);
}
public static HasEnabled from(MenuItem menu, HasStorage cache) {
Object cached = cache.retrieve(menu);
HasEnabled hasEnabled = (cached instanceof HasEnabled) ? (HasEnabled) cached : null;
if (hasEnabled == null) {
hasEnabled = new HasEnabledWrapper(menu);
cache.store(menu, hasEnabled);
}
return hasEnabled;
}
/**
* Helpful method for setting an enabled property on several components at
* the same time.
*
* @param enabled
* @param menuArray
*/
public static void setEnabled(boolean enabled, MenuItem... menuArray) {
if (menuArray != null) {
for (MenuItem menu : menuArray) {
if (menu != null) {
menu.setEnabled(enabled);
}
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy