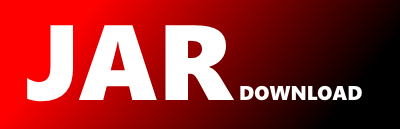
org.ioc.commons.impl.android.ui.HasLoaderWrapper Maven / Gradle / Ivy
package org.ioc.commons.impl.android.ui;
import org.ioc.commons.ui.HasLoader;
import org.ioc.commons.ui.HasStorage;
import android.view.View;
/**
* Wrapper for GWT components into {@link HasLoader}.
*
* @author Jesús Lunar Pérez
*
*/
public class HasLoaderWrapper implements HasLoader {
public static final int DEFAULT_INVISIBLE_MODE = View.GONE;
private final Integer[] previousVisibility;
private final View[] notLoadingArray;
private final View[] loadingArray;
private final int invisibleMode;
private boolean loading;
private HasLoaderWrapper(View[] notLoading, View[] loading, int invisibleMode) {
this.notLoadingArray = notLoading;
this.loadingArray = loading;
this.previousVisibility = notLoadingArray != null ? new Integer[notLoadingArray.length] : null;
this.invisibleMode = invisibleMode;
}
@Override
public void setLoading(boolean loading) {
if (notLoadingArray != null) {
for (int i = 0; i < notLoadingArray.length; i++) {
View notLoadingV = notLoadingArray[i];
if (loading) {
if (previousVisibility[i] == null) {
previousVisibility[i] = notLoadingV.getVisibility();
}
notLoadingV.setVisibility(invisibleMode);
} else if (previousVisibility[i] != null) {
notLoadingV.setVisibility(previousVisibility[i]);
previousVisibility[i] = null;
// } else {
// notLoadingV.setVisible(true);
}
}
}
if (loadingArray != null) {
for (View loadingV : loadingArray) {
loadingV.setVisibility(loading ? View.VISIBLE : this.invisibleMode);
}
}
this.loading = loading;
}
@Override
public boolean isLoading() {
return this.loading;
}
/**
* It returns an {@link HasLoader} instance which will turn into visible an
* indicator when is loading and will hide it when is not.
*
* @param indicator
* The indicator which will appear/disappear
*
* @return The {@link HasLoader} instance
*/
public static HasLoader fromVisible(View indicator) {
return fromVisibles((View[]) null, new View[] { indicator });
}
/**
* It returns an {@link HasLoader} instance which will show an indicator
* when is loading and another one when is not.
*
* @param notLoadingIndicator
* The visible indicator when is not loading
* @param loadingIndicator
* The visible indicator when is loading
*
* @return The {@link HasLoader} instance
*/
public static HasLoader fromVisibles(View notLoadingIndicator, View loadingIndicator) {
return fromVisibles(new View[] { notLoadingIndicator }, new View[] { loadingIndicator });
}
/**
* It returns an {@link HasLoader} instance which will show an indicator
* when is loading and others when is not.
*
* @param notLoadingIndicator
* The visible indicator when is not loading
* @param loadingIndicators
* The visible indicators when is loading
*
* @return The {@link HasLoader} instance
*/
public static HasLoader fromVisibles(View notLoadingIndicator, View[] loadingIndicators) {
return fromVisibles(new View[] { notLoadingIndicator }, loadingIndicators);
}
/**
* It returns an {@link HasLoader} instance which will show some indicators
* when is loading and another one when is not.
*
* @param notLoadingIndicators
* The visible indicators when is not loading
* @param loadingIndicator
* The visible indicator when is loading
*
* @return The {@link HasLoader} instance
*/
public static HasLoader fromVisibles(View[] notLoadingIndicators, View loadingIndicator) {
return fromVisibles(notLoadingIndicators, new View[] { loadingIndicator });
}
/**
* It returns an {@link HasLoader} instance which will show some indicators
* when is loading and others when is not.
*
* @param notLoadingIndicators
* The visible indicators when is not loading
* @param loadingIndicators
* The visible indicators when is loading
*
* @return The {@link HasLoader} instance
*/
public static HasLoader fromVisibles(View[] notLoadingIndicators, View[] loadingIndicators) {
return new HasLoaderWrapper(notLoadingIndicators, loadingIndicators, DEFAULT_INVISIBLE_MODE);
}
/**
* It returns an {@link HasLoader} instance which will turn into visible an
* indicator when is loading and will hide it when is not.
*
* @param indicator
* The indicator which will appear/disappear
*
* @return The {@link HasLoader} instance
*/
public static HasLoader fromVisible(View indicator, HasStorage cache) {
Object cached = cache.retrieve(indicator);
HasLoader hasLoader = (cached instanceof HasLoader) ? (HasLoader) cached : null;
if (hasLoader == null) {
hasLoader = fromVisible(indicator);
cache.store(indicator, hasLoader);
}
return hasLoader;
}
/**
* It returns an {@link HasLoader} instance which will turn into visible an
* indicator when is loading and will hide it when is not.
*
* @param indicator
* The indicator which will appear/disappear
* @param invisibleMode
* Style for invisible such as {@link View#INVISIBLE} or
* {@link View#GONE}
*
* @return The {@link HasLoader} instance
*/
public static HasLoader fromVisible(View indicator, int invisibleMode) {
return fromVisibles((View[]) null, new View[] { indicator }, invisibleMode);
}
/**
* It returns an {@link HasLoader} instance which will show an indicator
* when is loading and another one when is not.
*
* @param notLoadingIndicator
* The visible indicator when is not loading
* @param loadingIndicator
* The visible indicator when is loading
* @param invisibleMode
* Style for invisible such as {@link View#INVISIBLE} or
* {@link View#GONE}
*
* @return The {@link HasLoader} instance
*/
public static HasLoader fromVisibles(View notLoadingIndicator, View loadingIndicator, int invisibleMode) {
return fromVisibles(new View[] { notLoadingIndicator }, new View[] { loadingIndicator }, invisibleMode);
}
/**
* It returns an {@link HasLoader} instance which will show an indicator
* when is loading and others when is not.
*
* @param notLoadingIndicator
* The visible indicator when is not loading
* @param loadingIndicators
* The visible indicators when is loading
* @param invisibleMode
* Style for invisible such as {@link View#INVISIBLE} or
* {@link View#GONE}
*
* @return The {@link HasLoader} instance
*/
public static HasLoader fromVisibles(View notLoadingIndicator, View[] loadingIndicators, int invisibleMode) {
return fromVisibles(new View[] { notLoadingIndicator }, loadingIndicators, invisibleMode);
}
/**
* It returns an {@link HasLoader} instance which will show some indicators
* when is loading and another one when is not.
*
* @param notLoadingIndicators
* The visible indicators when is not loading
* @param loadingIndicator
* The visible indicator when is loading
* @param invisibleMode
* Style for invisible such as {@link View#INVISIBLE} or
* {@link View#GONE}
*
* @return The {@link HasLoader} instance
*/
public static HasLoader fromVisibles(View[] notLoadingIndicators, View loadingIndicator, int invisibleMode) {
return fromVisibles(notLoadingIndicators, new View[] { loadingIndicator }, invisibleMode);
}
/**
* It returns an {@link HasLoader} instance which will show some indicators
* when is loading and others when is not.
*
* @param notLoadingIndicators
* The visible indicators when is not loading
* @param loadingIndicators
* The visible indicators when is loading
* @param invisibleMode
* Style for invisible such as {@link View#INVISIBLE} or
* {@link View#GONE}
*
* @return The {@link HasLoader} instance
*/
public static HasLoader fromVisibles(View[] notLoadingIndicators, View[] loadingIndicators, int invisibleMode) {
return new HasLoaderWrapper(notLoadingIndicators, loadingIndicators, invisibleMode);
}
/**
* It returns an {@link HasLoader} instance which will turn into visible an
* indicator when is loading and will hide it when is not.
*
* @param indicator
* The indicator which will appear/disappear
* @param invisibleMode
* Style for invisible such as {@link View#INVISIBLE} or
* {@link View#GONE}
*
* @return The {@link HasLoader} instance
*/
public static HasLoader fromVisible(View indicator, HasStorage cache, int invisibleMode) {
Object cached = cache.retrieve(indicator);
HasLoader hasLoader = (cached instanceof HasLoader) ? (HasLoader) cached : null;
if (hasLoader == null) {
hasLoader = fromVisible(indicator, invisibleMode);
cache.store(indicator, hasLoader);
}
return hasLoader;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy