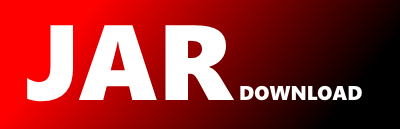
org.ioc.commons.impl.android.ui.HasPendingOperationsLoaderWrapper Maven / Gradle / Ivy
package org.ioc.commons.impl.android.ui;
import org.ioc.commons.ui.HasPendingOperationsLoader;
import org.ioc.commons.ui.HasStorage;
import android.view.View;
/**
* Wrapper for GWT components into {@link HasPendingOperationsLoader}. Similar
* to {@link HasLoaderWrapper} but for being used in several proccesses at the
* same time. It counts how many operation are pending (loading) and keeps
* visible the loader until all of them have finished.
*
* @author Jesús Lunar Pérez
*
*/
public class HasPendingOperationsLoaderWrapper implements HasPendingOperationsLoader {
public static final int DEFAULT_INVISIBLE_MODE = View.GONE;
private int pendingOps;
private Integer[] previousVisibility;
private final View[] notLoadingArray;
private final View[] loadingArray;
private final int invisibleMode;
private HasPendingOperationsLoaderWrapper(View[] notLoading, View[] loading, int invisibleMode) {
this.notLoadingArray = notLoading;
this.loadingArray = loading;
this.invisibleMode = invisibleMode;
}
@Override
public void clearPendingOperations() {
setPendingOps(0);
}
protected void setPendingOps(int pendingOps) {
if (pendingOps < 0) {
pendingOps = 0;
}
this.pendingOps = pendingOps;
boolean loading = this.isLoading();
if (notLoadingArray != null) {
for (int i = 0; i < notLoadingArray.length; i++) {
View notLoadingV = notLoadingArray[i];
if (loading) {
if (previousVisibility == null || previousVisibility.length != notLoadingArray.length) {
previousVisibility = new Integer[notLoadingArray.length];
}
previousVisibility[i] = notLoadingV.getVisibility();
notLoadingV.setVisibility(invisibleMode);
} else if (previousVisibility != null && previousVisibility.length == notLoadingArray.length) {
notLoadingV.setVisibility(previousVisibility[i]);
} else {
notLoadingV.setVisibility(View.VISIBLE);
}
}
}
if (loadingArray != null) {
for (View loadingV : loadingArray) {
loadingV.setVisibility(loading ? View.VISIBLE : invisibleMode);
}
}
}
@Override
public boolean isLoading() {
return this.pendingOps > 0;
}
@Override
public int getPendingOpCount() {
return this.pendingOps;
}
@Override
public void operationBeginning() {
setPendingOps(this.pendingOps + 1);
}
@Override
public void operationFinished() {
setPendingOps(this.pendingOps - 1);
}
/**
* It returns an {@link HasPendingOperationsLoader} instance which will turn
* into visible an indicator when is loading and will hide it when is not.
*
* @param indicator
* The indicator which will appear/disappear
*
* @return The {@link HasPendingOperationsLoader} instance
*/
public static HasPendingOperationsLoader fromVisible(View indicator) {
return fromVisibles((View[]) null, new View[] { indicator });
}
/**
* It returns an {@link HasPendingOperationsLoader} instance which will show
* an indicator when is loading and another one when is not.
*
* @param notLoadingIndicator
* The visible indicator when is not loading
* @param loadingIndicator
* The visible indicator when is loading
*
* @return The {@link HasPendingOperationsLoader} instance
*/
public static HasPendingOperationsLoader fromVisibles(View notLoadingIndicator, View loadingIndicator) {
return fromVisibles(new View[] { notLoadingIndicator }, new View[] { loadingIndicator });
}
/**
* It returns an {@link HasPendingOperationsLoader} instance which will show
* an indicator when is loading and others when is not.
*
* @param notLoadingIndicator
* The visible indicator when is not loading
* @param loadingIndicators
* The visible indicators when is loading
*
* @return The {@link HasPendingOperationsLoader} instance
*/
public static HasPendingOperationsLoader fromVisibles(View notLoadingIndicator, View[] loadingIndicators) {
return fromVisibles(new View[] { notLoadingIndicator }, loadingIndicators);
}
/**
* It returns an {@link HasPendingOperationsLoader} instance which will show
* some indicators when is loading and another one when is not.
*
* @param notLoadingIndicators
* The visible indicators when is not loading
* @param loadingIndicator
* The visible indicator when is loading
*
* @return The {@link HasPendingOperationsLoader} instance
*/
public static HasPendingOperationsLoader fromVisibles(View[] notLoadingIndicators, View loadingIndicator) {
return fromVisibles(notLoadingIndicators, new View[] { loadingIndicator });
}
/**
* It returns an {@link HasPendingOperationsLoader} instance which will show
* some indicators when is loading and others when is not.
*
* @param notLoadingIndicators
* The visible indicators when is not loading
* @param loadingIndicators
* The visible indicators when is loading
*
* @return The {@link HasPendingOperationsLoader} instance
*/
public static HasPendingOperationsLoader fromVisibles(View[] notLoadingIndicators, View[] loadingIndicators) {
return new HasPendingOperationsLoaderWrapper(notLoadingIndicators, loadingIndicators, DEFAULT_INVISIBLE_MODE);
}
/**
* It returns an {@link HasPendingOperationsLoader} instance which will turn
* into visible an indicator when is loading and will hide it when is not.
*
* @param indicator
* The indicator which will appear/disappear
*
* @return The {@link HasPendingOperationsLoader} instance
*/
public static HasPendingOperationsLoader fromVisible(View indicator, int invisibleMode) {
return fromVisibles((View[]) null, new View[] { indicator }, invisibleMode);
}
/**
* It returns an {@link HasPendingOperationsLoader} instance which will show
* an indicator when is loading and another one when is not.
*
* @param notLoadingIndicator
* The visible indicator when is not loading
* @param loadingIndicator
* The visible indicator when is loading
*
* @return The {@link HasPendingOperationsLoader} instance
*/
public static HasPendingOperationsLoader fromVisibles(View notLoadingIndicator, View loadingIndicator,
int invisibleMode) {
return fromVisibles(new View[] { notLoadingIndicator }, new View[] { loadingIndicator }, invisibleMode);
}
/**
* It returns an {@link HasPendingOperationsLoader} instance which will show
* an indicator when is loading and others when is not.
*
* @param notLoadingIndicator
* The visible indicator when is not loading
* @param loadingIndicators
* The visible indicators when is loading
*
* @return The {@link HasPendingOperationsLoader} instance
*/
public static HasPendingOperationsLoader fromVisibles(View notLoadingIndicator, View[] loadingIndicators,
int invisibleMode) {
return fromVisibles(new View[] { notLoadingIndicator }, loadingIndicators, invisibleMode);
}
/**
* It returns an {@link HasPendingOperationsLoader} instance which will show
* some indicators when is loading and another one when is not.
*
* @param notLoadingIndicators
* The visible indicators when is not loading
* @param loadingIndicator
* The visible indicator when is loading
*
* @return The {@link HasPendingOperationsLoader} instance
*/
public static HasPendingOperationsLoader fromVisibles(View[] notLoadingIndicators, View loadingIndicator,
int invisibleMode) {
return fromVisibles(notLoadingIndicators, new View[] { loadingIndicator }, invisibleMode);
}
/**
* It returns an {@link HasPendingOperationsLoader} instance which will show
* some indicators when is loading and others when is not.
*
* @param notLoadingIndicators
* The visible indicators when is not loading
* @param loadingIndicators
* The visible indicators when is loading
*
* @return The {@link HasPendingOperationsLoader} instance
*/
public static HasPendingOperationsLoader fromVisibles(View[] notLoadingIndicators, View[] loadingIndicators,
int invisibleMode) {
return new HasPendingOperationsLoaderWrapper(notLoadingIndicators, loadingIndicators, invisibleMode);
}
/**
* It returns an {@link HasPendingOperationsLoader} instance which will turn
* into visible an indicator when is loading and will hide it when is not.
*
* @param indicator
* The indicator which will appear/disappear
*
* @return The {@link HasPendingOperationsLoader} instance
*/
public static HasPendingOperationsLoader fromVisible(View indicator, HasStorage cache) {
Object cached = cache.retrieve(indicator);
HasPendingOperationsLoader hasLoader = (cached instanceof HasPendingOperationsLoader) ? (HasPendingOperationsLoader) cached
: null;
if (hasLoader == null) {
hasLoader = fromVisible(indicator);
cache.store(indicator, hasLoader);
}
return hasLoader;
}
/**
* It returns an {@link HasPendingOperationsLoader} instance which will turn
* into visible an indicator when is loading and will hide it when is not.
*
* @param indicator
* The indicator which will appear/disappear
*
* @return The {@link HasPendingOperationsLoader} instance
*/
public static HasPendingOperationsLoader fromVisible(View indicator, HasStorage cache, int invisibleMode) {
Object cached = cache.retrieve(indicator);
HasPendingOperationsLoader hasLoader = (cached instanceof HasPendingOperationsLoader) ? (HasPendingOperationsLoader) cached
: null;
if (hasLoader == null) {
hasLoader = fromVisible(indicator, invisibleMode);
cache.store(indicator, hasLoader);
}
return hasLoader;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy