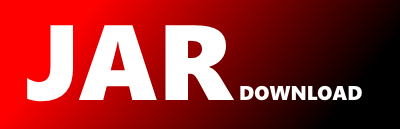
org.ioc.commons.impl.android.ui.HasValueWrapper Maven / Gradle / Ivy
package org.ioc.commons.impl.android.ui;
import java.text.DateFormat;
import java.text.ParseException;
import java.util.Calendar;
import java.util.Date;
import org.ioc.commons.ui.HasStorage;
import org.ioc.commons.ui.HasValue;
import android.widget.CheckBox;
import android.widget.Checkable;
import android.widget.DatePicker;
import android.widget.SearchView;
import android.widget.Spinner;
import android.widget.TextView;
public class HasValueWrapper {
private static final DateFormat DATE_FORMAT = DateFormat.getDateInstance();
public static class TextViewHasValue implements HasValue {
private final TextView widget;
private HasStorage cache;
TextViewHasValue(TextView widget) {
this.widget = widget;
}
public TextViewHasValue(TextView widget, HasStorage cache) {
this(widget);
this.cache = cache;
}
@Override
public void setValue(String value) {
widget.setText(value);
}
@Override
public String getValue() {
return widget.getText().toString();
}
@SuppressWarnings("unchecked")
public HasValue asDate() {
HasValue asDate = null;
if (cache != null) {
Object cached = cache.retrieve(this);
asDate = (cached instanceof HasValue ? (HasValue) cached : null);
}
if (asDate == null) {
asDate = new HasValue() {
@Override
public void setValue(Date value) {
widget.setText(value != null ? DATE_FORMAT.format(value) : null);
}
@Override
public Date getValue() {
try {
return widget.getText() != null ? DATE_FORMAT.parse(widget.getText().toString()) : null;
} catch (ParseException e) {
throw new RuntimeException(e);
}
}
};
cache.store(widget, asDate);
}
return asDate;
}
}
private HasValueWrapper() {
/* No const */
}
/**
* Get a new wrapper from a {@link CheckBox} instance.
*
* @param widget
* The instance to wrap
* @return A new wrapper
*/
public static HasValue from(final CheckBox widget) {
return new HasValue() {
@Override
public void setValue(Boolean value) {
widget.setChecked(Boolean.TRUE.equals(value));
}
@Override
public Boolean getValue() {
return widget.isChecked();
}
};
}
/**
* Get a new wrapper from a {@link CheckBox} instance.
*
* @param widget
* The instance to wrap
* @param cache
* Wrappers cache
* @return A new wrapper
*/
public static HasValue from(CheckBox widget, HasStorage cache) {
Object cached = cache.retrieve(widget);
@SuppressWarnings("unchecked")
HasValue hasValue = (cached instanceof HasValue ? (HasValue) cached : null);
if (hasValue == null) {
hasValue = from(widget);
cache.store(widget, hasValue);
}
return hasValue;
}
/**
* Get a new wrapper from a {@link SearchView} instance.
*
* @param widget
* The instance to wrap
* @return A new wrapper
*/
public static HasValue from(final SearchView widget, final boolean submitQuery) {
return new HasValue() {
@Override
public void setValue(String value) {
widget.setQuery(value, submitQuery);
}
@Override
public String getValue() {
return widget.getQuery().toString();
}
};
}
/**
* Get a new wrapper from a {@link SearchView} instance.
*
* @param widget
* The instance to wrap
* @param cache
* Wrappers cache
* @return A new wrapper
*/
public static HasValue from(SearchView widget, boolean submitQuery, HasStorage cache) {
Object cached = cache.retrieve(widget);
@SuppressWarnings("unchecked")
HasValue hasValue = (cached instanceof HasValue ? (HasValue) cached : null);
if (hasValue == null) {
hasValue = from(widget, submitQuery);
cache.store(widget, hasValue);
}
return hasValue;
}
/**
* Get a new wrapper from a {@link CheckBox} instance.
*
* @param widget
* The instance to wrap
* @return A new wrapper
*/
public static HasValue from(final Checkable widget) {
return new HasValue() {
@Override
public void setValue(Boolean value) {
widget.setChecked(Boolean.TRUE.equals(value));
}
@Override
public Boolean getValue() {
return widget.isChecked();
}
};
}
/**
* Get a new wrapper from a {@link CheckBox} instance.
*
* @param widget
* The instance to wrap
* @param cache
* Wrappers cache
* @return A new wrapper
*/
public static HasValue from(Checkable widget, HasStorage cache) {
Object cached = cache.retrieve(widget);
@SuppressWarnings("unchecked")
HasValue hasValue = (cached instanceof HasValue ? (HasValue) cached : null);
if (hasValue == null) {
hasValue = from(widget);
cache.store(widget, hasValue);
}
return hasValue;
}
/**
* Get a new wrapper from a {@link TextView} instance.
*
* @param widget
* The instance to wrap
* @return A new wrapper
*/
public static TextViewHasValue from(final TextView widget) {
return new TextViewHasValue(widget);
}
/**
* Get a new wrapper from a {@link TextView} instance.
*
* @param widget
* The instance to wrap
* @param cache
* Wrappers cache
* @return A new wrapper
*/
public static TextViewHasValue from(final TextView widget, HasStorage cache) {
Object cached = cache.retrieve(widget);
TextViewHasValue hasValue = (cached instanceof TextViewHasValue ? (TextViewHasValue) cached : null);
if (hasValue == null) {
hasValue = new TextViewHasValue(widget, cache);
cache.store(widget, hasValue);
}
return hasValue;
}
public static > HasValue from(final Spinner spinner, final E[] values) {
return new HasValue() {
@Override
public void setValue(E value) {
for (int i = 0; i < values.length; i++) {
E v = values[i];
if (v == value) {
spinner.setSelection(i);
}
}
}
@Override
public E getValue() {
return values[spinner.getSelectedItemPosition()];
}
};
}
public static > HasValue from(final Spinner widget, HasStorage cache, final E[] values) {
Object cached = cache.retrieve(widget);
@SuppressWarnings("unchecked")
HasValue hasValue = (cached instanceof HasValue ? (HasValue) cached : null);
if (hasValue == null) {
hasValue = from(widget, values);
cache.store(widget, hasValue);
}
return hasValue;
}
/**
* Get a new wrapper from a {@link DatePicker} instance.
*
* @param widget
* The instance to wrap
* @return A new wrapper
*/
public static HasValue from(final DatePicker widget) {
return new HasValue() {
@Override
public void setValue(Date value) {
Calendar dt = Calendar.getInstance();
dt.setTime(value);
int year = dt.get(Calendar.YEAR);
int month = dt.get(Calendar.MONTH);
int day = dt.get(Calendar.DAY_OF_MONTH);
widget.updateDate(year, month, day);
}
@Override
public Date getValue() {
Calendar dt = Calendar.getInstance();
dt.set(widget.getYear(), widget.getMonth(), widget.getDayOfMonth(), 0, 0);
return dt.getTime();
}
};
}
/**
* Get a new wrapper from a {@link DatePicker} instance.
*
* @param widget
* The instance to wrap
* @param cache
* Wrappers cache
* @return A new wrapper
*/
public static HasValue from(DatePicker widget, HasStorage cache) {
Object cached = cache.retrieve(widget);
@SuppressWarnings("unchecked")
HasValue hasValue = (cached instanceof HasValue ? (HasValue) cached : null);
if (hasValue == null) {
hasValue = from(widget);
cache.store(widget, hasValue);
}
return hasValue;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy