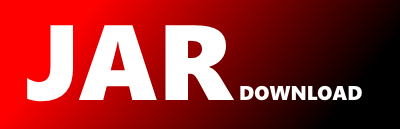
org.ioc.commons.impl.android.ui.HasVisibilityWrapper Maven / Gradle / Ivy
package org.ioc.commons.impl.android.ui;
import org.ioc.commons.ui.HasStorage;
import org.ioc.commons.ui.HasVisibility;
import android.view.MenuItem;
import android.view.View;
public class HasVisibilityWrapper implements HasVisibility {
public static final int DEFAULT_INVISIBLE_MODE = View.GONE;
private final View wrappedView;
private final MenuItem wrappedMenu;
private final int invisibleMode;
private HasVisibilityWrapper(View wrapped, int invisibleMode) {
this.wrappedView = wrapped;
this.wrappedMenu = null;
this.invisibleMode = invisibleMode;
}
private HasVisibilityWrapper(MenuItem wrapped) {
this.wrappedMenu = wrapped;
this.wrappedView = null;
this.invisibleMode = DEFAULT_INVISIBLE_MODE;
}
@Override
public void setVisible(boolean visible) {
if (this.wrappedView != null) {
this.wrappedView.setVisibility(visible ? View.VISIBLE : this.invisibleMode);
} else if (this.wrappedMenu != null) {
this.wrappedMenu.setVisible(visible);
}
}
@Override
public boolean isVisible() {
return this.wrappedView != null ? this.wrappedView.getVisibility() == View.VISIBLE : this.wrappedMenu
.isVisible();
}
public static HasVisibility from(View view) {
return from(view, DEFAULT_INVISIBLE_MODE);
}
public static HasVisibility from(View view, int invisibleMode) {
return new HasVisibilityWrapper(view, invisibleMode);
}
public static HasVisibility from(View view, HasStorage cache) {
return from(view, DEFAULT_INVISIBLE_MODE, cache);
}
public static HasVisibility from(View view, int invisibleMode, HasStorage cache) {
Object cached = cache.retrieve(view);
HasVisibility hasVisibility = (cached instanceof HasVisibility) ? (HasVisibility) cached : null;
if (hasVisibility == null) {
hasVisibility = new HasVisibilityWrapper(view, invisibleMode);
cache.store(view, hasVisibility);
}
return hasVisibility;
}
public static HasVisibility from(MenuItem item) {
return new HasVisibilityWrapper(item);
}
public static HasVisibility from(MenuItem item, HasStorage cache) {
Object cached = cache.retrieve(item);
HasVisibility hasVisibility = (cached instanceof HasVisibility) ? (HasVisibility) cached : null;
if (hasVisibility == null) {
hasVisibility = new HasVisibilityWrapper(item);
cache.store(item, hasVisibility);
}
return hasVisibility;
}
/**
* Helpful method for setting an visible property on several components at
* the same time.
*
* @param visible
* @param viewArray
*/
public static void setVisible(boolean visible, View... viewArray) {
setVisible(visible, DEFAULT_INVISIBLE_MODE, viewArray);
}
/**
* Helpful method for setting an visible property on several components at
* the same time.
*
* @param visible
* @param invisibleMode
* Style for invisible such as {@link View#INVISIBLE} or
* {@link View#GONE}
* @param viewArray
*/
public static void setVisible(boolean visible, int invisibleMode, View... viewArray) {
if (viewArray != null) {
for (View view : viewArray) {
view.setVisibility(visible ? View.VISIBLE : invisibleMode);
}
}
}
public static void setVisible(boolean visible, MenuItem... menuItemArray) {
if (menuItemArray != null) {
for (MenuItem menuItem : menuItemArray) {
menuItem.setVisible(visible);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy