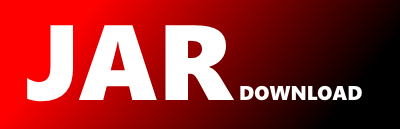
org.ioc.commons.impl.gwt.client.ui.HasValueWrapper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ioc-commons-gwt-impl Show documentation
Show all versions of ioc-commons-gwt-impl Show documentation
GWT implementation for ioc-commons artifacts.
package org.ioc.commons.impl.gwt.client.ui;
import org.ioc.commons.ui.HasStorage;
import org.ioc.commons.ui.HasValue;
import com.google.gwt.user.client.TakesValue;
public class HasValueWrapper implements HasValue {
private com.google.gwt.user.client.TakesValue wrapped;
private Boolean fireEvents;
private HasValueWrapper(com.google.gwt.user.client.TakesValue wrapped) {
this.wrapped = wrapped;
}
private HasValueWrapper(com.google.gwt.user.client.ui.HasValue wrapped, boolean defaultFireEvents) {
this(wrapped);
this.fireEvents = defaultFireEvents;
}
@Override
public void setValue(D value) {
if (fireEvents == null) {
this.wrapped.setValue(value);
} else {
((com.google.gwt.user.client.ui.HasValue) this.wrapped).setValue(value, fireEvents);
}
}
@Override
public D getValue() {
return this.wrapped.getValue();
}
/**
* Get a new wrapper from a gwt TakesValue instance.
*
* @param gwtHasValue
* The instance to wrap
* @return A new wrapper
*/
public static HasValue from(com.google.gwt.user.client.TakesValue gwtHasValue) {
return new HasValueWrapper(gwtHasValue);
}
/**
* Get a new wrapper from a GWT HasValue instance, taking into account if
* fire or not events when setValue method is called.
*
* @param gwtHasValue
* The instance to wrap
* @param fireEvents
* Indicates if it must fire or not events when setValue method
* is called
* @return A new wrapper
*/
public static HasValue from(com.google.gwt.user.client.ui.HasValue gwtHasValue, boolean fireEvents) {
return new HasValueWrapper(gwtHasValue, fireEvents);
}
/**
* Get a wrapper from a GWT TakesValue instance. The same gwtHasValue
* parameter will return the same wrapper instance (cached).
*
* It will try to get the wrapper from storage cache. In case there is no
* one, it will create a new instance and will cache it for next calls.
*
* @param gwtTakesValue
* GWT {@link TakesValue} instance
* @param cache
* Wrappers cache
* @return The wrapper instance for gwtHasValue
*/
public static HasValue from(com.google.gwt.user.client.TakesValue gwtTakesValue, HasStorage cache) {
Object cached = cache.retrieve(gwtTakesValue);
@SuppressWarnings("unchecked")
HasValueWrapper hasValue = (cached instanceof HasValueWrapper ? (HasValueWrapper) cached : null);
if (hasValue == null) {
hasValue = new HasValueWrapper(gwtTakesValue);
cache.store(gwtTakesValue, hasValue);
}
return hasValue;
}
/**
* Get a wrapper from a GWT HasValue instance, taking into account if fire
* or not events when setValue method is called. The same gwtHasValue
* parameter will return the same wrapper instance (cached).
*
* It will try to get the wrapper from storage cache. In case there is no
* one, it will create a new instance and will cache it for next calls.
*
* @param gwtHasValue
* The instance to wrap
* @param fireEvents
* Indicates if it must fire or not events when setValue method
* is called
* @param cache
* Wrappers cache
* @return A new wrapper
*/
public static HasValue from(com.google.gwt.user.client.ui.HasValue gwtHasValue, boolean fireEvents,
HasStorage cache) {
Object cached = cache.retrieve(gwtHasValue);
@SuppressWarnings("unchecked")
HasValueWrapper hasValue = (cached instanceof HasValueWrapper ? (HasValueWrapper) cached : null);
if (hasValue == null) {
hasValue = new HasValueWrapper(gwtHasValue, fireEvents);
cache.store(gwtHasValue, hasValue);
}
return hasValue;
}
/**
* Helpful method for setting a value property on several components at the
* same time.
*
* @param fireEvents
* fire events if true and value is new. See
* {@link com.google.gwt.user.client.ui.HasValue}
* @param value
* the object's new value
* @param gwtHasValueArray
* Array of GWT HasValue components
*/
public static void setValue(boolean fireEvents, D value,
com.google.gwt.user.client.ui.HasValue... gwtHasValueArray) {
if (gwtHasValueArray != null) {
for (com.google.gwt.user.client.ui.HasValue gwtHasValue : gwtHasValueArray) {
gwtHasValue.setValue(value, fireEvents);
}
}
}
/**
* Helpful method for setting a value property on several components at the
* same time.
*
* @param value
* the object's new value
* @param gwtTakesValueArray
* Array of GWT HasValue components
*/
public static void setValue(D value, com.google.gwt.user.client.TakesValue... gwtTakesValueArray) {
if (gwtTakesValueArray != null) {
for (com.google.gwt.user.client.TakesValue gwtTakesValue : gwtTakesValueArray) {
gwtTakesValue.setValue(value);
}
}
}
public static HasValue fromText(final com.google.gwt.user.client.ui.HasText gwtHasText, HasStorage cache) {
return fromText(gwtHasText, cache, "");
}
public static HasValue fromText(final com.google.gwt.user.client.ui.HasText gwtHasText, HasStorage cache,
final String emptyValueString) {
Object cached = cache.retrieve(gwtHasText);
@SuppressWarnings("unchecked")
HasValue hasValue = (cached instanceof HasValue ? (HasValue) cached : null);
if (hasValue == null) {
hasValue = new HasValue() {
private D value;
@Override
public void setValue(D value) {
this.value = value;
gwtHasText.setText(value != null ? value.toString() : emptyValueString);
}
@Override
public D getValue() {
return this.value;
}
};
cache.store(gwtHasText, hasValue);
}
return hasValue;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy