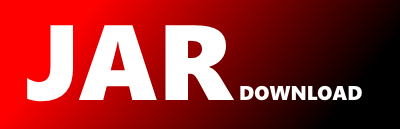
org.ioc.commons.utils.Format Maven / Gradle / Ivy
package org.ioc.commons.utils;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
/**
* Utility class for giving a text format to objects such as parameterized strings, collections or maps.
*
* @author Code partially extracted from Sencha GXT 2.3.0.
* @author Jesús Lunar Pérez
*/
public class Format {
public static interface StringFormatter {
String asString(T obj);
}
/**
* Format a string by using the {n} notation, where 'n' is the position
* number of the parameter.
*
* Example: {@code
*
* String formattedString = Format.substitute("Name: {0}; Address: {1};",
* name, address);
*
* }
*
* @param text
* Text to be formatted
* @param params
* Parameters
* @return Formatted text
*/
public static String substitute(String text, Object... params) {
return substitute(false, text, params);
}
/**
* Format a string by using the {n} notation, where 'n' is the position
* number of the parameter.
*
* Example: {@code
*
* String formattedString = Format.substitute("Name: {0}; Address: {1};",
* name, address);
*
* }
*
*
* @param nullAsEmptyString
* false to get null values as "null"; true to get them as empty
* string ""
* @param text
* Text to be formatted
* @param params
* Parameters
* @return Formatted text
*/
public static String substitute(boolean nullAsEmptyString, String text, Object... params) {
for (int i = 0; i < params.length; i++) {
Object p = params[i];
String value = (p != null || !nullAsEmptyString) ? String.valueOf(p) : null;
text = text.replaceAll("\\{" + i + "\\}", safeRegexReplacement(value));
}
return text;
}
protected static String safeRegexReplacement(String replacement) {
if (replacement == null) {
return "";
}
return replacement.replaceAll("\\\\", "\\\\\\\\").replaceAll("\\$", "\\\\\\$");
}
public static String capitalize(String str) {
int strLen;
if (str == null || (strLen = str.length()) == 0) {
return str;
}
return new StringBuffer(strLen).append(str.substring(0, 1).toUpperCase()).append(str.substring(1)).toString();
}
public static String decapitalize(String str) {
int strLen;
if (str == null || (strLen = str.length()) == 0) {
return str;
}
return new StringBuffer(strLen).append(str.substring(0, 1).toLowerCase()).append(str.substring(1)).toString();
}
public static String collectionToString(Collection collection, StringFormatter formatter) {
return collectionToString("[", ", ", "]", collection, formatter);
}
public static String collectionToString(String prefix, String separator, String suffix, Collection collection) {
return collectionToString(prefix, separator, suffix, collection, new StringFormatter() {
@Override
public String asString(T obj) {
return obj != null ? obj.toString() : "null";
}
});
}
public static String collectionToString(String prefix, String separator, String suffix,
Collection collection, StringFormatter formatter) {
return collectionToString(prefix, separator, null, suffix, collection, formatter);
}
public static String collectionToString(String prefix, String separator, String lastElementSeparator,
String suffix, Collection collection, StringFormatter formatter) {
if (collection == null) {
return "null";
}
StringBuffer sb = new StringBuffer();
if (prefix != null) {
sb.append(prefix);
}
Iterator iterator = collection.iterator();
int size = collection.size();
for (int i = 0; i < size; i++) {
T elm = iterator.next();
if (i != 0) {
if (i != size - 1 || lastElementSeparator == null) {
if (separator != null) {
sb.append(separator);
}
} else {
sb.append(lastElementSeparator);
}
}
sb.append(formatter.asString(elm));
}
if (suffix != null) {
sb.append(suffix);
}
return sb.toString();
}
public static String mapToString(Map map) {
return mapToString(map, Format.getSimpleStringFormatter(), Format.getSimpleStringFormatter());
}
public static String mapToString(Map map, StringFormatter keyFormatter,
StringFormatter valueFormatter) {
return mapToString("[", ", ", "]", map, keyFormatter, valueFormatter);
}
public static String mapToString(String prefix, String elementSeparator, String suffix, Map map) {
return mapToString(prefix, ": ", elementSeparator, suffix, map);
}
public static String mapToString(String prefix, String keyValueSeparator, String elementSeparator,
String suffix, Map map) {
return mapToString(prefix, keyValueSeparator, elementSeparator, elementSeparator, suffix, map,
Format.getSimpleStringFormatter(), Format.getSimpleStringFormatter());
}
private static StringFormatter getSimpleStringFormatter() {
return new StringFormatter() {
@Override
public String asString(V obj) {
return obj != null ? obj.toString() : "null";
}
};
}
public static String mapToString(String prefix, String elementSeparator, String suffix, Map map,
StringFormatter keyFormatter, StringFormatter valueFormatter) {
return mapToString(prefix, elementSeparator, null, suffix, map, keyFormatter, valueFormatter);
}
public static String mapToString(String prefix, String elementSeparator, String lastElementSeparator,
String suffix, Map map, StringFormatter keyFormatter, StringFormatter valueFormatter) {
return mapToString(prefix, ": ", elementSeparator, lastElementSeparator, suffix, map, keyFormatter,
valueFormatter);
}
public static String mapToString(String prefix, String keyValueSeparator, String elementSeparator,
String lastElementSeparator, String suffix, Map map, StringFormatter keyFormatter,
StringFormatter valueFormatter) {
if (map == null) {
return "null";
}
StringBuffer sb = new StringBuffer();
if (prefix != null) {
sb.append(prefix);
}
List keyList = new ArrayList(map.keySet());
int size = keyList.size();
for (int i = 0; i < size; i++) {
K key = keyList.get(i);
if (i != 0) {
if (i != size - 1 || lastElementSeparator == null) {
if (elementSeparator != null) {
sb.append(elementSeparator);
}
} else {
sb.append(lastElementSeparator);
}
}
sb.append(keyFormatter.asString(key));
if (keyValueSeparator != null) {
sb.append(keyValueSeparator);
}
V obj = map.get(key);
sb.append(valueFormatter.asString(obj));
}
if (suffix != null) {
sb.append(suffix);
}
return sb.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy