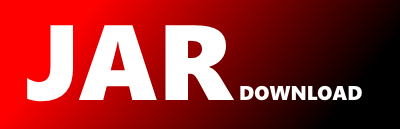
org.ioc.commons.flowcontrol.globalflowcontroller.GlobalFlowController Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ioc-commons Show documentation
Show all versions of ioc-commons Show documentation
This project defines a set of useful java interfaces for helping you in the definition of the structure of your developments in Java-projects which are designed using a Inversion-Of-Control (IOC) pattern. Useful for MVP-Pattern designs in applications coded on GWT, SWT, Android, etc.
package org.ioc.commons.flowcontrol.globalflowcontroller;
import org.ioc.commons.flowcontrol.HasFlowControl;
import org.ioc.commons.flowcontrol.actioncontroller.ActionController;
import org.ioc.commons.flowcontrol.actioncontroller.ActionHandler;
import org.ioc.commons.flowcontrol.actioncontroller.IsExternalAction;
import org.ioc.commons.flowcontrol.common.BindRegistration;
import org.ioc.commons.flowcontrol.eventbus.EventBus;
import org.ioc.commons.flowcontrol.eventbus.EventHandler;
import org.ioc.commons.flowcontrol.eventbus.IsEvent;
import org.ioc.commons.flowcontrol.eventbus.IsEventBus;
import org.ioc.commons.flowcontrol.operationmanager.IsOperation;
import org.ioc.commons.flowcontrol.operationmanager.OperationHandler;
import org.ioc.commons.flowcontrol.operationmanager.OperationManager;
import org.ioc.commons.ui.IsWidget;
/**
* Implementations of this interface are used to manage the global flow on the
* entiry application, that is, global events, global actions and global
* operations which are not related to an specific entity or widget.
*
* @author Jesús Lunar Pérez
*
* @param
* Global event type
* @param
* Global operation type
* @param
* Global action type
*/
public interface GlobalFlowController, O extends Enum extends IsOperation>, A extends Enum extends IsExternalAction>>
extends HasFlowControl {
/**
* Global event bus
*
* @author Jes�s Lunar P�rez
*
* @param
* Global event type
*/
public interface GlobalEventBus> extends EventBus, IsEventBus {
/**
* Binds a global event while the widget passed as parameter is active,
* that is, the widget is being shown at this moment.
*
* If the widget is not active, the global event fired will not be
* managed by this handler until the widget becomes active again.
*
* @param whileDisplayIsActive
* Widget which needs to be active for handling the global
* event.
* @param event
* Global event
* @param handler
* Handler which will process the event in case the widget is
* active
*
* @return A bind registration object for managing the new binding
* registered.
*/
BindRegistration bindEvent(IsWidget whileDisplayIsActive, E event, EventHandler handler);
}
/**
* Global operation manager
*
* @author Jes�s Lunar P�rez
*
* @param
* Global operation type
*/
public interface GlobalOperationManager> extends OperationManager {
/**
* Binds a global operation while the widget passed as parameter is
* active, that is, the widget is being shown at this moment.
*
* If the widget is not active, the global operation will not be managed
* by this handler until the widget becomes active again.
*
* @param whileDisplayIsActive
* Widget which needs to be active for handling the global
* operation.
* @param operation
* Global operation
* @param handler
* Handler which will process the operation in case the
* widget is active
* @return A bind registration object for managing the new binding
* registered.
*/
BindRegistration bindOperation(IsWidget whileDisplayIsActive, O operation, OperationHandler handler);
/**
* Binds a global operation and automatically unbinds it when it
* finishes, while the widget passed as parameter is active, that is,
* the widget is being shown at this moment.
*
* If the widget is not active, the global event fired will not be
* managed by this handler until the widget becomes active again.
*
* @param whileDisplayIsActive
* Widget which needs to be active for handling the global
* operation.
* @param operation
* Global operation
* @param handler
* Handler which will process the operation in case the
* widget is active
* @return A bind registration object for managing the new binding
* registered.
*/
BindRegistration bindOperationOnce(IsWidget whileDisplayIsActive, O operation, OperationHandler handler);
/**
* Bind for all operations, while the widget passed as parameter is
* active, that is, the widget is being shown at this moment. The
* handler will be fired when first operation begins running and when
* last operation ends.
*
* @param whileDisplayIsActive
* Widget which needs to be active for handling the global
* operation.
* @param handler
* Handler which will process the operation in case the
* widget is active
* @return A bind registration object for managing the new binding
* registered.
*/
BindRegistration bindOperationsRunning(IsWidget whileDisplayIsActive, OperationHandler handler);
}
/**
* Global action manager
*
* @author Jes�s Lunar P�rez
*
* @param
* Action type
*/
public interface GlobalActionController> extends ActionController {
/**
* Binds a global action while the widget passed as parameter is active,
* that is, the widget is being shown at this moment.
*
* If the widget is not active, the global action invoked will not be
* managed by this handler until the widget becomes active again.
*
* @param whileDisplayIsActive
* Widget which needs to be active for handling the global
* action.
* @param action
* Global action
* @param handler
* Handler which will process the action in case the widget
* is active
* @return A bind registration object for managing the new binding
* registered.
*/
BindRegistration bindAction(IsWidget whileDisplayIsActive, A action, ActionHandler handler);
}
/**
* @return Global event bus
*/
GlobalEventBus eventBus();
/**
* @return Global operation manager
*/
GlobalOperationManager operationManager();
/**
* @return Global action controller
*/
GlobalActionController actionController();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy