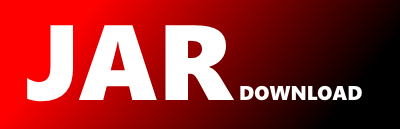
org.ioc.commons.integration.dataaccess.dao.SubscriptionChangesBinder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ioc-commons Show documentation
Show all versions of ioc-commons Show documentation
This project defines a set of useful java interfaces for helping you in the definition of the structure of your developments in Java-projects which are designed using a Inversion-Of-Control (IOC) pattern. Useful for MVP-Pattern designs in applications coded on GWT, SWT, Android, etc.
package org.ioc.commons.integration.dataaccess.dao;
import java.util.List;
import org.ioc.commons.flowcontrol.common.BindRegistration;
import org.ioc.commons.flowcontrol.operationmanager.IsOperation;
import org.ioc.commons.flowcontrol.operationmanager.OperationManagerTrigger;
/**
* Binder for entity changes
*
* @author Jesús Lunar Pérez
*
* @param
* Entity type
* @param
* Entity identifier type
*/
public interface SubscriptionChangesBinder {
/**
* Binds for changes on entities
*
* @param handler
* Handler which will process the some operations when changes
* occurred.
* @return A bind registration object for managing the new binding
* registered.
*/
BindRegistration bindChanges(SubscriptionChangeHandler handler);
/**
* Binds only the first change on some entity and it unbids
* automatically.
*
* @param handler
* Handler which will process the some operations when changes
* occurred.
* @return A bind registration object for managing the new binding
* registered.
*/
BindRegistration bindChangesOnce(SubscriptionChangeHandler handler);
/**
* Update a list of entities when a change has been made on an entity.
*
* A entity will be added when anentity is persisted. A entity will
* be replaced in case the list constains it when is updated. A entity
* will be removed from the lists in case the list constains it when is
* deleted.
*
* @param collection
* List of entities
* @param propertyRefs
* The minimum required properties (accesible attributes of the T
* class through getters) to be filled in the T entity before
* using in the list.
* @return A bind registration object for managing the new binding
* registered.
*/
BindRegistration updateList(List collection, String... propertyRefs);
/**
* It performs the same operation as {@link #updateList(List, String...)}
* but just once and then it unbinds automatically.
*
* @param collection
* List of entities
* @param propertyRefs
* The minimum required properties (accesible attributes of the T
* class through getters) to be filled in the T entity before
* using in the list.
* @return A bind registration object for managing the new binding
* registered.
*/
BindRegistration updateListOnce(List collection, String... propertyRefs);
/**
* It performs the same operation as {@link #updateList(List, String...)}
* but managing an operation during the updation.
*
* @param operation
* Operation
* @param trigger
* Trigger for managing
* @param collection
* List of entities
* @param propertyRefs
* The minimum required properties (accesible attributes of the T
* class through getters) to be filled in the T entity before
* using in the list.
* @return A bind registration object for managing the new binding
* registered.
*/
> BindRegistration updateList(O operation,
OperationManagerTrigger trigger, List collection, String... propertyRefs);
/**
* It performs the same operation as
* {@link #updateList(Enum, OperationManagerTrigger, List, String...)} but
* just once and then it unbinds automatically.
*
* @param operation
* Operation
* @param trigger
* Trigger for managing
* @param collection
* List of entities
* @param propertyRefs
* The minimum required properties (accesible attributes of the T
* class through getters) to be filled in the T entity before
* using in the list.
* @return A bind registration object for managing the new binding
* registered.
*/
> BindRegistration updateListOnce(O operation,
OperationManagerTrigger trigger, List collection, String... propertyRefs);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy