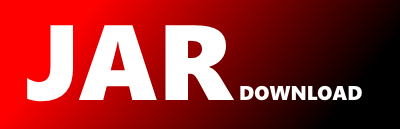
com.selesse.gradle.git.changelog.generator.ComplexChangelogGenerator.groovy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gradle-git-changelog Show documentation
Show all versions of gradle-git-changelog Show documentation
Generate Git changelogs for Gradle projects
package com.selesse.gradle.git.changelog.generator
import com.google.common.base.Joiner
import com.google.common.base.MoreObjects
import com.google.common.base.Splitter
import com.selesse.gradle.git.GitCommandExecutor
class ComplexChangelogGenerator implements ChangelogGenerator {
final Map tagAndDateMap
final GitCommandExecutor executor
final boolean skipFirstTag
ComplexChangelogGenerator(GitCommandExecutor executor, List tags, boolean skipFirstTag) {
this.executor = executor
this.tagAndDateMap = tags.collectEntries {
def tagAndDate = Splitter.on("|").omitEmptyStrings().trimResults().splitToList(it) as List
if (tagAndDate.size() != 2) {
tagAndDate = [tagAndDate.get(0), null]
}
[(tagAndDate.get(0)): tagAndDate.get(1)]
} as Map
this.skipFirstTag = skipFirstTag
}
String generateChangelog() {
List changelogs = []
def dateCommitMap = tagAndDateMap.keySet().collectEntries {
[(executor.getCommitDate(it)): it]
} as Map
def dates = dateCommitMap.keySet().sort()
if (!skipFirstTag) {
appendFirstCommitChangeLog(dates, dateCommitMap, tagAndDateMap, changelogs)
}
for (int i = 0; (i + 1) < dates.size(); i++) {
def firstCommit = dateCommitMap.get(dates.get(i))
def secondCommitDate = dates.get(i + 1)
def secondCommit = dateCommitMap.get(secondCommitDate)
secondCommitDate = MoreObjects.firstNonNull(tagAndDateMap.get(secondCommit), executor.getTagDate(secondCommit))
def sectionTitle = "${executor.getTagName(secondCommit)} (${secondCommitDate})"
def sectionChangelog = executor.getGitChangelog(firstCommit, secondCommit)
changelogs << getChangelogSection(sectionTitle, sectionChangelog)
}
appendLastCommitChangelog(dateCommitMap, dates, changelogs)
def reverseChangelogs = changelogs.reverse()
return Joiner.on("\n").join(reverseChangelogs)
}
private List appendFirstCommitChangeLog(List dates, Map dateCommitMap, Map tagAndDateMap, List changelogs) {
def secondCommitDate = dates.get(0)
def secondCommit = dateCommitMap.get(secondCommitDate)
secondCommitDate = MoreObjects.firstNonNull(tagAndDateMap.get(secondCommit), executor.getTagDate(secondCommit))
def sectionTitle = "${executor.getTagName(secondCommit)} (${secondCommitDate})"
def sectionChangelog = executor.getGitChangelog(secondCommit)
changelogs << getChangelogSection(sectionTitle, sectionChangelog)
}
private void appendLastCommitChangelog(Map dateCommitMap, List dates, List changelogs) {
def firstCommit = dateCommitMap.get(dates.last())
def secondCommit = executor.getLatestCommit()
def changelog = executor.getGitChangelog(firstCommit, secondCommit)
if (changelog.length() > 0) {
changelogs << getChangelogSection("Unreleased", changelog)
}
}
String getChangelogSection(String sectionTitle, String sectionChangelog) {
StringBuilder changelogSection = new StringBuilder()
changelogSection.append(sectionTitle)
.append('\n')
.append('-'.multiply(sectionTitle.length()))
.append('\n')
.append(sectionChangelog)
.append('\n')
return changelogSection.toString()
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy