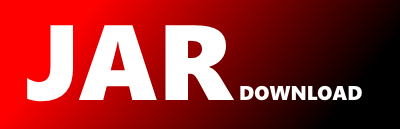
com.semanticcms.news.view.NewsView Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of semanticcms-news-view Show documentation
Show all versions of semanticcms-news-view Show documentation
SemanticCMS view of all news in the current page and all children.
/*
* semanticcms-news-view - SemanticCMS view of all news in the current page and all children.
* Copyright (C) 2016 AO Industries, Inc.
* [email protected]
* 7262 Bull Pen Cir
* Mobile, AL 36695
*
* This file is part of semanticcms-news-view.
*
* semanticcms-news-view is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* semanticcms-news-view is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with semanticcms-news-view. If not, see .
*/
package com.semanticcms.news.view;
import com.aoindustries.servlet.http.Dispatcher;
import com.aoindustries.servlet.http.LastModifiedServlet;
import com.aoindustries.taglib.Link;
import com.semanticcms.core.model.Page;
import com.semanticcms.core.servlet.PageUtils;
import com.semanticcms.core.servlet.View;
import com.semanticcms.news.model.News;
import com.semanticcms.news.servlet.RssUtils;
import java.io.IOException;
import java.util.Collection;
import java.util.Collections;
import java.util.LinkedHashMap;
import java.util.Map;
import javax.servlet.ServletContext;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.jsp.SkipPageException;
public class NewsView extends View {
public static final String VIEW_NAME = "news";
private static final String JSPX_TARGET = "/semanticcms-news-view/view.inc.jsp";
@Override
public Group getGroup() {
return Group.FIXED;
}
@Override
public String getDisplay() {
return "News";
}
@Override
public String getName() {
return VIEW_NAME;
}
@Override
public boolean isApplicable(
ServletContext servletContext,
HttpServletRequest request,
HttpServletResponse response,
Page page
) throws ServletException, IOException {
return PageUtils.hasElement(servletContext, request, response, page, News.class, true);
}
@Override
public String getTitle(
ServletContext servletContext,
HttpServletRequest request,
HttpServletResponse response,
Page page
) {
String bookTitle = page.getPageRef().getBook().getTitle();
if(bookTitle != null && !bookTitle.isEmpty()) {
return "What's New" + TITLE_SEPARATOR + page.getTitle() + TITLE_SEPARATOR + bookTitle;
} else {
return "What's New" + TITLE_SEPARATOR + page.getTitle();
}
}
/**
* Description required by RSS channel.
*/
@Override
public String getDescription(Page page) {
return "What's New in " + page.getTitle();
}
@Override
public String getKeywords(Page page) {
return null;
}
@Override
public Collection getLinks(
ServletContext servletContext,
HttpServletRequest request,
HttpServletResponse response,
Page page
) throws ServletException, IOException {
if(
// RSS module must be loaded
RssUtils.isRssEnabled(servletContext)
// Only link to RSS when this view applies (has news on self or child page)
&& isApplicable(servletContext, request, response, page)
) {
return Collections.singleton(
new Link(
RssUtils.getRssServletPath(page), // href
false, // hrefAbsolute
null, // params
LastModifiedServlet.AddLastModifiedWhen.FALSE,
null, // hreflang
"alternate", // rel
RssUtils.CONTENT_TYPE,
null, // media
getTitle(servletContext, request, response, page)
)
);
} else {
return super.getLinks(servletContext, request, response, page);
}
}
/**
* News entries are not displayed on their page, but rather on their news view.
*
* If the page does not allow robots, this view will also not allow robots.
*
*
* If the page does not have any direct news (child news doesn't count), then robots will be excluded.
* This is to reduce the chances of duplicate content when a parent page also includes child page news.
*
*/
@Override
public boolean getAllowRobots(
ServletContext servletContext,
HttpServletRequest request,
HttpServletResponse response,
Page page
) throws ServletException, IOException {
return
// If the page does not allow robots, this view will also not allow robots.
PageUtils.findAllowRobots(servletContext, request, response, page)
// If the page does not have any direct news (child news doesn't count), then robots will be excluded.
&& PageUtils.hasElement(servletContext, request, response, page, News.class, false)
;
}
@Override
public void doView(ServletContext servletContext, HttpServletRequest request, HttpServletResponse response, Page page) throws ServletException, IOException, SkipPageException {
Map args = new LinkedHashMap();
args.put("page", page);
boolean isRssEnabled = RssUtils.isRssEnabled(servletContext);
args.put("isRssEnabled", isRssEnabled);
if(isRssEnabled) {
args.put("rssServletPath", RssUtils.getRssServletPath(page));
//args.put("rssTitle", getTitle(servletContext, request, response, page));
args.put("rssType", RssUtils.CONTENT_TYPE);
}
Dispatcher.include(
servletContext,
JSPX_TARGET,
request,
response,
args
);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy