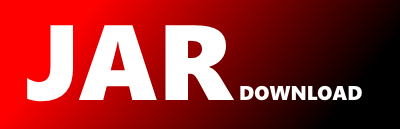
com.sencha.gxt.legacy.client.binding.Bindings Maven / Gradle / Ivy
/**
* Sencha GXT 3.1.1 - Sencha for GWT
* Copyright(c) 2007-2014, Sencha, Inc.
* [email protected]
*
* http://www.sencha.com/products/gxt/license/
*/
package com.sencha.gxt.legacy.client.binding;
import java.util.Collection;
import java.util.Map;
import com.google.gwt.core.client.Scheduler;
import com.google.gwt.core.client.Scheduler.ScheduledCommand;
import com.google.gwt.event.shared.EventHandler;
import com.google.gwt.event.shared.GwtEvent;
import com.google.gwt.event.shared.HandlerManager;
import com.sencha.gxt.core.shared.FastMap;
import com.sencha.gxt.legacy.client.data.ModelData;
import com.sencha.gxt.widget.core.client.form.Field;
/**
* Aggregates one to many field bindings.
*
*
* - Events:
*
* - BeforeBind : BindingEvent(source, model)
* Fires before binding.
*
* - source : this
* - model : the model to bind
*
*
*
* - Bind : BindingEvent(source, model)
* Fires after successful binding.
*
* - source : this
* - model : the binded model
*
*
*
* - UnBind : BindingEvent(source, model)
* Fires after successful unbinding.
*
* - source : this
* - model : the unbound model
*
*
*
*
* @see FieldBinding
*/
public class Bindings {
protected ModelData model;
protected Map bindings;
private final HandlerManager handlerManager = new HandlerManager(this);
/**
* Creates a new bindings instance.
*/
public Bindings() {
bindings = new FastMap();
}
/**
* Adds a field binding.
*
* @param binding the binding instance to add
*/
public void addFieldBinding(FieldBinding binding) {
bindings.put(binding.getField().getId(), binding);
}
/**
* Binds the model instance.
*
* @param model the model
*/
public void bind(final ModelData model) {
Scheduler.get().scheduleDeferred(new ScheduledCommand() {
@Override
public void execute() {
BindingEvent e = new BindingEvent(model, Bind);
handlerManager.fireEvent(e);
if (!e.isCancelled()) {
if (Bindings.this.model != null) {
unbind();
}
Bindings.this.model = model;
for (FieldBinding binding : bindings.values()) {
binding.bind(model);
}
handlerManager.fireEvent(e);
}
}
});
}
/**
* Clears all fields by setting the value for each field to null
.
*/
public void clear() {
for (FieldBinding fieldBinding : getBindings()) {
fieldBinding.getField().clear();
}
}
/**
* Returns the field binding for the given field.
*
* @param field the field
* @return the field binding or null of no match
*/
public FieldBinding getBinding(Field> field) {
return bindings.get(field.getId());
}
/**
* Returns all bindings.
*
* @return the collection of bindings
*/
public Collection getBindings() {
return bindings.values();
}
/**
* Returns the currently bound model;
*
* @return the currently bound model;
*/
public ModelData getModel() {
return model;
}
/**
* Removes a field binding.
*
* @param binding the binding instance to remove
*/
public void removeFieldBinding(FieldBinding binding) {
bindings.remove(binding.getField().getId());
}
/**
* Unbinds the current model.
*/
public void unbind() {
if (model != null) {
for (FieldBinding binding : bindings.values()) {
binding.unbind();
}
handlerManager.fireEvent(new BindingEvent(model, UnBind));
model = null;
}
}
static final GwtEvent.Type UnBind = new GwtEvent.Type();
static final GwtEvent.Type Bind = new GwtEvent.Type();
static final GwtEvent.Type BeforeBind = new GwtEvent.Type();
public static class BindingEvent extends GwtEvent {
private final Type type;
private boolean cancelled;
public BindingEvent(ModelData model, GwtEvent.Type type) {
this.type = type;
}
@Override
public GwtEvent.Type getAssociatedType() {
return type;
}
@Override
protected void dispatch(BindingHandler handler) {
handler.handleEvent(this);
}
public boolean isCancelled() {
return cancelled;
}
public void setCancelled(boolean cancelled) {
this.cancelled = cancelled;
}
}
interface BindingHandler extends EventHandler {
void handleEvent(BindingEvent evt);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy