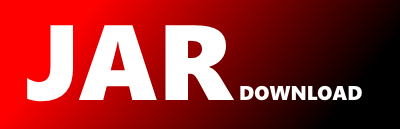
com.sencha.gxt.legacy.client.mvc.AppEvent Maven / Gradle / Ivy
/**
* Sencha GXT 3.1.1 - Sencha for GWT
* Copyright(c) 2007-2014, Sencha, Inc.
* [email protected]
*
* http://www.sencha.com/products/gxt/license/
*/
package com.sencha.gxt.legacy.client.mvc;
import java.util.Map;
import com.google.gwt.event.shared.GwtEvent;
import com.sencha.gxt.core.shared.FastMap;
/**
* AppEvents
are used to pass messages between
* Controllers
and Views
. All events have a specific
* type which are used to identify the event. Typically, applications will
* define all application events in a constants class.
*/
public class AppEvent extends GwtEvent {
private final GwtEvent.Type> type;
private Object data;
private Map dataMap;
private boolean historyEvent;
private String token;
/**
* Creates a new application event.
*
* @param type
* the event type
*/
public AppEvent(GwtEvent.Type> type) {
this.type = type;
}
/**
* Creates a new application event.
*
* @param type
* the event type
* @param data
* the data
*/
public AppEvent(GwtEvent.Type> type, Object data) {
this(type);
this.data = data;
}
/**
* Creates a new application event.
*
* @param type
* the event type
* @param data
* the event data
* @param token
* the history token
*/
public AppEvent(GwtEvent.Type> type, Object data, String token) {
this(type, data);
this.token = token;
historyEvent = true;
}
@SuppressWarnings({ "rawtypes", "unchecked" })
public GwtEvent.Type getAssociatedType() {
return (GwtEvent.Type) type;
}
@Override
protected void dispatch(DispatcherListener handler) {
}
/**
* Returns the application specified data.
*
* @param
* the data type
* @return the data
*/
@SuppressWarnings("unchecked")
public X getData() {
return (X) data;
}
/**
* Returns the application defined property for the given name, or
* null
if it has not been set.
*
* @param key
* the name of the property
* @return the value or null
if it has not been set
*/
@SuppressWarnings("unchecked")
public X getData(String key) {
if (dataMap == null)
return null;
return (X) dataMap.get(key);
}
/**
* Returns the history token.
*
* @return the history token
*/
public String getToken() {
return token;
}
/**
* Returns true if the event is a history event.
*
* @return true for a history event
*/
public boolean isHistoryEvent() {
return historyEvent;
}
/**
* Sets the application defined data.
*
* @param data
* the data
*/
public void setData(Object data) {
this.data = data;
}
/**
* Sets the application defined property with the given name.
*
* @param key
* the name of the property
* @param data
* the new value for the property
*/
public void setData(String key, Object data) {
if (dataMap == null)
dataMap = new FastMap
© 2015 - 2025 Weber Informatics LLC | Privacy Policy