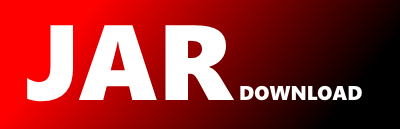
com.sencha.gxt.data.shared.loader.PagingLoader Maven / Gradle / Ivy
package com.sencha.gxt.data.shared.loader;
/**
* A ListLoader
subclass which adds support for paged data (offset,
* limit, and total count).
*
* @param the type of data for the input object
* @param the type of data to be returned by the loader
*/
public class PagingLoader> extends ListLoader {
protected int offset = 0;
protected int limit = 50;
protected int totalCount;
/**
* Creates a new paging loader instance.
*
* @param proxy the data proxy
*/
public PagingLoader(DataProxy proxy) {
super(proxy);
}
/**
* Creates a new paging loader instance.
*
* @param proxy the data proxy
* @param reader the data reader
*/
public PagingLoader(DataProxy proxy, DataReader reader) {
super(proxy, reader);
}
/**
* Returns the current limit.
*
* @return the current limit
*/
public int getLimit() {
return limit;
}
/**
* Returns the offset of the first record.
*
* @return the current offset
*/
public int getOffset() {
return offset;
}
/**
* Returns the total number of models in the dataset as returned by the
* server.
*
* @return the number of models as passed from the server
*/
public int getTotalCount() {
return totalCount;
}
/**
* Loads the data using the specified configuration.
*
* @param offset the offset of the first record to return
* @param limit the page size
*/
public void load(int offset, int limit) {
this.offset = offset;
this.limit = limit;
load();
}
/**
* Sets the limit size.
*
* @param limit the limit
*/
public void setLimit(int limit) {
this.limit = limit;
}
/**
* Sets the offset.
*
* @param offset the offset
*/
public void setOffset(int offset) {
this.offset = offset;
}
/**
* Use the specified LoadConfig for all load calls. The {@link #reuseConfig}
* will be set to true.
*/
@Override
public void useLoadConfig(C loadConfig) {
super.useLoadConfig(loadConfig);
offset = loadConfig.getOffset();
limit = loadConfig.getLimit();
}
@SuppressWarnings("unchecked")
@Override
protected C newLoadConfig() {
return (C) new PagingLoadConfigBean();
}
@Override
protected void onLoadSuccess(C loadConfig, D result) {
totalCount = result.getTotalLength();
offset = result.getOffset();
fireEvent(new LoadEvent(loadConfig, result));
}
@Override
protected C prepareLoadConfig(C config) {
config = super.prepareLoadConfig(config);
config.setOffset(offset);
config.setLimit(limit);
return config;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy