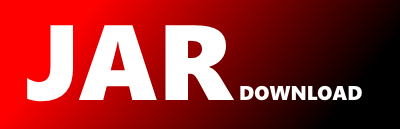
com.sencha.gxt.core.client.XTemplates Maven / Gradle / Ivy
/**
* Ext GWT 3.0.0-beta2 - Ext for GWT
* Copyright(c) 2007-2011, Sencha, Inc.
* [email protected]
*
* http://sencha.com/license
*/
package com.sencha.gxt.core.client;
import java.lang.annotation.Documented;
import java.lang.annotation.ElementType;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
import com.google.gwt.i18n.client.DateTimeFormat;
import com.google.gwt.i18n.client.NumberFormat;
import com.sencha.gxt.core.client.XTemplates.FormatterFactories;
import com.sencha.gxt.core.client.XTemplates.FormatterFactory;
import com.sencha.gxt.core.client.XTemplates.FormatterFactoryMethod;
import com.sencha.gxt.core.client.XTemplates.TemplateCondition;
/**
* Methods can return {@link com.google.gwt.safehtml.shared.SafeHtml} or String
* data, based on the template provided, and the parameters of the method.
*/
@FormatterFactories({
@FormatterFactory(factory = DateTimeFormat.class, methods = @FormatterFactoryMethod(name = "date", defaultArgs = "\"M/d/y\"")),
@FormatterFactory(factory = NumberFormat.class, methods = {
@FormatterFactoryMethod(name = "scientific", method = "getScientificFormat"),
@FormatterFactoryMethod(name = "decimal", method = "getDecimalFormat"),
@FormatterFactoryMethod(name = "currency", method = "getCurrencyFormat"),
@FormatterFactoryMethod(name = "number", method = "getFormat", defaultArgs = "\"#\""),
@FormatterFactoryMethod(name = "percentage", method = "getPercentFormat")
})})
@TemplateCondition(methodName = "equals", type = Object.class)
public interface XTemplates {
/**
* Indicates the string that should be used when generating the template.
* Either an html string can be specified (like
* {@link com.google.gwt.safehtml.client.SafeHtmlTemplates}, or a source file
* may be specified.
*
*/
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.METHOD)
@Documented
public @interface XTemplate {
/**
* The template itself, defined inline
*/
String value() default "";
/**
* The filename of the html file containing the template. The path is
* relative to the current package.
*/
String source() default "";
}
public interface Formatter {
String format(T data);
}
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.TYPE)
public @interface Format {
Class extends Formatter>> type();
String name();
}
/**
* Collection of {@link FormatterFactory} instances.
*/
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.TYPE)
public @interface FormatterFactories {
FormatterFactory[] value();
}
/**
* Declares that a class may act as a factory to provide formatter instances.
* Either the name attribute must be populated with the name that this factory
* will use in an XTemplate and the method getFormat will be called, or the
* methods must indicate a name and a methodName to be invoked on the factory.
*
*/
@Retention(RetentionPolicy.RUNTIME)
public @interface FormatterFactory {
Class> factory();
String name() default "";
FormatterFactoryMethod[] methods() default {};
}
/**
* Allows methods to be called on a factory other than 'getFormat'. More than
* one instance of this may be provided to a {@link FormatterFactory}
* annotation to register multiple method within the same factory.
*
*/
@Retention(RetentionPolicy.RUNTIME)
public @interface FormatterFactoryMethod {
String name();
String method() default "getFormat";
String defaultArgs() default "";
}
@Retention(RetentionPolicy.RUNTIME)
public @interface TemplateCondition {
String name() default "";
Class> type();
String methodName();
}
@Retention(RetentionPolicy.RUNTIME)
public @interface TemplateConditions {
TemplateCondition[] value();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy