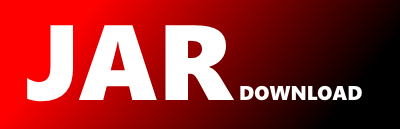
com.sencha.gxt.data.shared.event.StoreAddEvent Maven / Gradle / Ivy
/**
* Sencha GXT 3.0.1 - Sencha for GWT
* Copyright(c) 2007-2012, Sencha, Inc.
* [email protected]
*
* http://www.sencha.com/products/gxt/license/
*/
package com.sencha.gxt.data.shared.event;
import java.util.Collections;
import java.util.List;
import com.google.gwt.event.shared.EventHandler;
import com.google.gwt.event.shared.GwtEvent;
import com.google.gwt.event.shared.HandlerRegistration;
import com.google.gwt.event.shared.HasHandlers;
import com.sencha.gxt.data.shared.event.StoreAddEvent.StoreAddHandler;
/**
* Indicates that an element has been added to the Store, and is visible under
* the current filter settings.
*
* @param the model type
*/
public class StoreAddEvent extends StoreEvent> {
/**
* A class that implements this interface is a public source of
* {@link StoreAddEvent}.
*
* @param the type of data contained in the store
*/
public interface HasStoreAddHandlers extends HasHandlers {
/**
* Adds a {@link StoreAddEvent} handler.
*
* @param handler the handler
* @return the registration for the event
*/
HandlerRegistration addStoreAddHandler(StoreAddHandler handler);
}
/**
* Handler interface for {@link StoreAddEvent}.
*
* @param the type of data contained in the store
*/
public interface StoreAddHandler extends EventHandler {
/**
* Called when {@link StoreAddEvent} is fired.
*
* @param event the {@link StoreAddEvent} that was fired
*/
void onAdd(StoreAddEvent event);
}
private static GwtEvent.Type> TYPE;
/**
* Gets the type associated with this event.
*
* @return returns the handler type
*/
public static GwtEvent.Type> getType() {
if (TYPE == null) {
TYPE = new GwtEvent.Type>();
}
return TYPE;
}
private final List items;
private final int index;
/**
* Creates a new store add event.
*
* @param index the insert index
* @param items the items that were added
*/
public StoreAddEvent(int index, List items) {
this.index = index;
this.items = Collections.unmodifiableList(items);
}
/**
* Creates a new store add event.
*
* @param index the insert index
* @param item the item that was added
*/
public StoreAddEvent(int index, M item) {
this.index = index;
items = Collections.singletonList(item);
}
@SuppressWarnings({"unchecked", "rawtypes"})
@Override
public GwtEvent.Type> getAssociatedType() {
return (GwtEvent.Type) getType();
}
/**
* Returns the insert index for this store add event.
*
* @return the insert index
*/
public int getIndex() {
return index;
}
/**
* Returns the items that were inserted for this store add event.
*
* @return the list of items that were inserted
*/
public List getItems() {
return items;
}
@Override
protected void dispatch(StoreAddHandler handler) {
handler.onAdd(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy