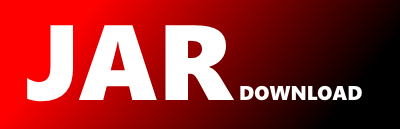
com.sendgrid.helpers.mail.objects.Personalization Maven / Gradle / Ivy
package com.sendgrid;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
@JsonInclude(Include.NON_DEFAULT)
public class Personalization {
@JsonProperty("to") private List tos;
@JsonProperty("cc") private List ccs;
@JsonProperty("bcc") private List bccs;
@JsonProperty("subject") private String subject;
@JsonProperty("headers") private Map headers;
@JsonProperty("substitutions") private Map substitutions;
@JsonProperty("custom_args") private Map customArgs;
@JsonProperty("send_at") private long sendAt;
@JsonProperty("to")
public List getTos() {
return tos;
}
public void addTo(Email email) {
Email newEmail = new Email();
newEmail.setName(email.getName());
newEmail.setEmail(email.getEmail());
if (tos == null) {
tos = new ArrayList();
tos.add(newEmail);
} else {
tos.add(newEmail);
}
}
@JsonProperty("cc")
public List getCcs() {
return ccs;
}
public void addCc(Email email) {
Email newEmail = new Email();
newEmail.setName(email.getName());
newEmail.setEmail(email.getEmail());
if (ccs == null) {
ccs = new ArrayList();
ccs.add(newEmail);
} else {
ccs.add(newEmail);
}
}
@JsonProperty("bcc")
public List getBccs() {
return bccs;
}
public void addBcc(Email email) {
Email newEmail = new Email();
newEmail.setName(email.getName());
newEmail.setEmail(email.getEmail());
if (bccs == null) {
bccs = new ArrayList();
bccs.add(newEmail);
} else {
bccs.add(newEmail);
}
}
@JsonProperty("subject")
public String getSubject() {
return subject;
}
public void setSubject(String subject) {
this.subject = subject;
}
@JsonProperty("headers")
public Map getHeaders() {
return headers;
}
public void addHeader(String key, String value) {
if (headers == null) {
headers = new HashMap();
headers.put(key, value);
} else {
headers.put(key, value);
}
}
@JsonProperty("substitutions")
public Map getSubstitutions() {
return substitutions;
}
public void addSubstitution(String key, String value) {
if (substitutions == null) {
substitutions = new HashMap();
substitutions.put(key, value);
} else {
substitutions.put(key, value);
}
}
@JsonProperty("custom_args")
public Map getCustomArgs() {
return customArgs;
}
public void addCustomArg(String key, String value) {
if (customArgs == null) {
customArgs = new HashMap();
customArgs.put(key, value);
} else {
customArgs.put(key, value);
}
}
@JsonProperty("send_at")
public long sendAt() {
return sendAt;
}
public void setSendAt(long sendAt) {
this.sendAt = sendAt;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy