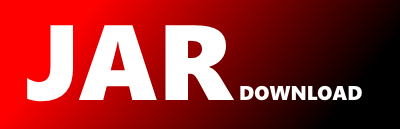
sibApi.ContactsApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sib-api-v3-sdk Show documentation
Show all versions of sib-api-v3-sdk Show documentation
SendinBlue's API v3 Java Library
The newest version!
/*
* SendinBlue API
* SendinBlue provide a RESTFul API that can be used with any languages. With this API, you will be able to : - Manage your campaigns and get the statistics - Manage your contacts - Send transactional Emails and SMS - and much more... You can download our wrappers at https://github.com/orgs/sendinblue **Possible responses** | Code | Message | | :-------------: | ------------- | | 200 | OK. Successful Request | | 201 | OK. Successful Creation | | 202 | OK. Request accepted | | 204 | OK. Successful Update/Deletion | | 400 | Error. Bad Request | | 401 | Error. Authentication Needed | | 402 | Error. Not enough credit, plan upgrade needed | | 403 | Error. Permission denied | | 404 | Error. Object does not exist | | 405 | Error. Method not allowed | | 406 | Error. Not Acceptable |
*
* OpenAPI spec version: 3.0.0
* Contact: [email protected]
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package sibApi;
import okhttp3.Call;
import sendinblue.ApiCallback;
import sendinblue.ApiClient;
import sendinblue.ApiException;
import sendinblue.ApiResponse;
import sendinblue.Configuration;
import sendinblue.Pair;
import sendinblue.ProgressRequestBody;
import sendinblue.ProgressResponseBody;
import com.google.gson.reflect.TypeToken;
import java.io.IOException;
import sibModel.AddContactToList;
import sibModel.CreateAttribute;
import sibModel.CreateContact;
import sibModel.CreateDoiContact;
import sibModel.CreateList;
import sibModel.CreateModel;
import sibModel.CreateUpdateContactModel;
import sibModel.CreateUpdateFolder;
import sibModel.CreatedProcessId;
import sibModel.ErrorModel;
import sibModel.GetAttributes;
import sibModel.GetContactCampaignStats;
import sibModel.GetContacts;
import sibModel.GetExtendedContactDetails;
import sibModel.GetExtendedList;
import sibModel.GetFolder;
import sibModel.GetFolderLists;
import sibModel.GetFolders;
import sibModel.GetLists;
import sibModel.PostContactInfo;
import sibModel.RemoveContactFromList;
import sibModel.RequestContactExport;
import sibModel.RequestContactImport;
import sibModel.UpdateAttribute;
import sibModel.UpdateBatchContacts;
import sibModel.UpdateBatchContactsModel;
import sibModel.UpdateContact;
import sibModel.UpdateList;
import java.lang.reflect.Type;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class ContactsApi {
private ApiClient apiClient;
public ContactsApi() {
this(Configuration.getDefaultApiClient());
}
public ContactsApi(ApiClient apiClient) {
this.apiClient = apiClient;
}
public ApiClient getApiClient() {
return apiClient;
}
public void setApiClient(ApiClient apiClient) {
this.apiClient = apiClient;
}
/**
* Build call for addContactToList
* @param listId Id of the list (required)
* @param contactEmails Emails addresses OR IDs of the contacts (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call addContactToListCall(Long listId, AddContactToList contactEmails, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = contactEmails;
// create path and map variables
String localVarPath = "/contacts/lists/{listId}/contacts/add"
.replaceAll("\\{" + "listId" + "\\}", apiClient.escapeString(listId.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call addContactToListValidateBeforeCall(Long listId, AddContactToList contactEmails, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'listId' is set
if (listId == null) {
throw new ApiException("Missing the required parameter 'listId' when calling addContactToList(Async)");
}
// verify the required parameter 'contactEmails' is set
if (contactEmails == null) {
throw new ApiException("Missing the required parameter 'contactEmails' when calling addContactToList(Async)");
}
Call call = addContactToListCall(listId, contactEmails, progressListener, progressRequestListener);
return call;
}
/**
* Add existing contacts to a list
*
* @param listId Id of the list (required)
* @param contactEmails Emails addresses OR IDs of the contacts (required)
* @return PostContactInfo
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public PostContactInfo addContactToList(Long listId, AddContactToList contactEmails) throws ApiException {
ApiResponse resp = addContactToListWithHttpInfo(listId, contactEmails);
return resp.getData();
}
/**
* Add existing contacts to a list
*
* @param listId Id of the list (required)
* @param contactEmails Emails addresses OR IDs of the contacts (required)
* @return ApiResponse<PostContactInfo>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse addContactToListWithHttpInfo(Long listId, AddContactToList contactEmails) throws ApiException {
Call call = addContactToListValidateBeforeCall(listId, contactEmails, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Add existing contacts to a list (asynchronously)
*
* @param listId Id of the list (required)
* @param contactEmails Emails addresses OR IDs of the contacts (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call addContactToListAsync(Long listId, AddContactToList contactEmails, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = addContactToListValidateBeforeCall(listId, contactEmails, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for createAttribute
* @param attributeCategory Category of the attribute (required)
* @param attributeName Name of the attribute (required)
* @param createAttribute Values to create an attribute (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call createAttributeCall(String attributeCategory, String attributeName, CreateAttribute createAttribute, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = createAttribute;
// create path and map variables
String localVarPath = "/contacts/attributes/{attributeCategory}/{attributeName}"
.replaceAll("\\{" + "attributeCategory" + "\\}", apiClient.escapeString(attributeCategory.toString()))
.replaceAll("\\{" + "attributeName" + "\\}", apiClient.escapeString(attributeName.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call createAttributeValidateBeforeCall(String attributeCategory, String attributeName, CreateAttribute createAttribute, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'attributeCategory' is set
if (attributeCategory == null) {
throw new ApiException("Missing the required parameter 'attributeCategory' when calling createAttribute(Async)");
}
// verify the required parameter 'attributeName' is set
if (attributeName == null) {
throw new ApiException("Missing the required parameter 'attributeName' when calling createAttribute(Async)");
}
// verify the required parameter 'createAttribute' is set
if (createAttribute == null) {
throw new ApiException("Missing the required parameter 'createAttribute' when calling createAttribute(Async)");
}
Call call = createAttributeCall(attributeCategory, attributeName, createAttribute, progressListener, progressRequestListener);
return call;
}
/**
* Create contact attribute
*
* @param attributeCategory Category of the attribute (required)
* @param attributeName Name of the attribute (required)
* @param createAttribute Values to create an attribute (required)
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public void createAttribute(String attributeCategory, String attributeName, CreateAttribute createAttribute) throws ApiException {
createAttributeWithHttpInfo(attributeCategory, attributeName, createAttribute);
}
/**
* Create contact attribute
*
* @param attributeCategory Category of the attribute (required)
* @param attributeName Name of the attribute (required)
* @param createAttribute Values to create an attribute (required)
* @return ApiResponse<Void>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse createAttributeWithHttpInfo(String attributeCategory, String attributeName, CreateAttribute createAttribute) throws ApiException {
Call call = createAttributeValidateBeforeCall(attributeCategory, attributeName, createAttribute, null, null);
return apiClient.execute(call);
}
/**
* Create contact attribute (asynchronously)
*
* @param attributeCategory Category of the attribute (required)
* @param attributeName Name of the attribute (required)
* @param createAttribute Values to create an attribute (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call createAttributeAsync(String attributeCategory, String attributeName, CreateAttribute createAttribute, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = createAttributeValidateBeforeCall(attributeCategory, attributeName, createAttribute, progressListener, progressRequestListener);
apiClient.executeAsync(call, callback);
return call;
}
/**
* Build call for createContact
* @param createContact Values to create a contact (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call createContactCall(CreateContact createContact, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = createContact;
// create path and map variables
String localVarPath = "/contacts";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call createContactValidateBeforeCall(CreateContact createContact, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'createContact' is set
if (createContact == null) {
throw new ApiException("Missing the required parameter 'createContact' when calling createContact(Async)");
}
Call call = createContactCall(createContact, progressListener, progressRequestListener);
return call;
}
/**
* Create a contact
*
* @param createContact Values to create a contact (required)
* @return CreateUpdateContactModel
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public CreateUpdateContactModel createContact(CreateContact createContact) throws ApiException {
ApiResponse resp = createContactWithHttpInfo(createContact);
return resp.getData();
}
/**
* Create a contact
*
* @param createContact Values to create a contact (required)
* @return ApiResponse<CreateUpdateContactModel>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse createContactWithHttpInfo(CreateContact createContact) throws ApiException {
Call call = createContactValidateBeforeCall(createContact, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Create a contact (asynchronously)
*
* @param createContact Values to create a contact (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call createContactAsync(CreateContact createContact, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = createContactValidateBeforeCall(createContact, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for createDoiContact
* @param createDoiContact Values to create the Double opt-in (DOI) contact (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call createDoiContactCall(CreateDoiContact createDoiContact, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = createDoiContact;
// create path and map variables
String localVarPath = "/contacts/doubleOptinConfirmation";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call createDoiContactValidateBeforeCall(CreateDoiContact createDoiContact, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'createDoiContact' is set
if (createDoiContact == null) {
throw new ApiException("Missing the required parameter 'createDoiContact' when calling createDoiContact(Async)");
}
Call call = createDoiContactCall(createDoiContact, progressListener, progressRequestListener);
return call;
}
/**
* Create Contact via DOI (Double-Opt-In) Flow
*
* @param createDoiContact Values to create the Double opt-in (DOI) contact (required)
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public void createDoiContact(CreateDoiContact createDoiContact) throws ApiException {
createDoiContactWithHttpInfo(createDoiContact);
}
/**
* Create Contact via DOI (Double-Opt-In) Flow
*
* @param createDoiContact Values to create the Double opt-in (DOI) contact (required)
* @return ApiResponse<Void>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse createDoiContactWithHttpInfo(CreateDoiContact createDoiContact) throws ApiException {
Call call = createDoiContactValidateBeforeCall(createDoiContact, null, null);
return apiClient.execute(call);
}
/**
* Create Contact via DOI (Double-Opt-In) Flow (asynchronously)
*
* @param createDoiContact Values to create the Double opt-in (DOI) contact (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call createDoiContactAsync(CreateDoiContact createDoiContact, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = createDoiContactValidateBeforeCall(createDoiContact, progressListener, progressRequestListener);
apiClient.executeAsync(call, callback);
return call;
}
/**
* Build call for createFolder
* @param createFolder Name of the folder (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call createFolderCall(CreateUpdateFolder createFolder, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = createFolder;
// create path and map variables
String localVarPath = "/contacts/folders";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call createFolderValidateBeforeCall(CreateUpdateFolder createFolder, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'createFolder' is set
if (createFolder == null) {
throw new ApiException("Missing the required parameter 'createFolder' when calling createFolder(Async)");
}
Call call = createFolderCall(createFolder, progressListener, progressRequestListener);
return call;
}
/**
* Create a folder
*
* @param createFolder Name of the folder (required)
* @return CreateModel
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public CreateModel createFolder(CreateUpdateFolder createFolder) throws ApiException {
ApiResponse resp = createFolderWithHttpInfo(createFolder);
return resp.getData();
}
/**
* Create a folder
*
* @param createFolder Name of the folder (required)
* @return ApiResponse<CreateModel>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse createFolderWithHttpInfo(CreateUpdateFolder createFolder) throws ApiException {
Call call = createFolderValidateBeforeCall(createFolder, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Create a folder (asynchronously)
*
* @param createFolder Name of the folder (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call createFolderAsync(CreateUpdateFolder createFolder, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = createFolderValidateBeforeCall(createFolder, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for createList
* @param createList Values to create a list (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call createListCall(CreateList createList, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = createList;
// create path and map variables
String localVarPath = "/contacts/lists";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call createListValidateBeforeCall(CreateList createList, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'createList' is set
if (createList == null) {
throw new ApiException("Missing the required parameter 'createList' when calling createList(Async)");
}
Call call = createListCall(createList, progressListener, progressRequestListener);
return call;
}
/**
* Create a list
*
* @param createList Values to create a list (required)
* @return CreateModel
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public CreateModel createList(CreateList createList) throws ApiException {
ApiResponse resp = createListWithHttpInfo(createList);
return resp.getData();
}
/**
* Create a list
*
* @param createList Values to create a list (required)
* @return ApiResponse<CreateModel>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse createListWithHttpInfo(CreateList createList) throws ApiException {
Call call = createListValidateBeforeCall(createList, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Create a list (asynchronously)
*
* @param createList Values to create a list (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call createListAsync(CreateList createList, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = createListValidateBeforeCall(createList, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for deleteAttribute
* @param attributeCategory Category of the attribute (required)
* @param attributeName Name of the existing attribute (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call deleteAttributeCall(String attributeCategory, String attributeName, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/contacts/attributes/{attributeCategory}/{attributeName}"
.replaceAll("\\{" + "attributeCategory" + "\\}", apiClient.escapeString(attributeCategory.toString()))
.replaceAll("\\{" + "attributeName" + "\\}", apiClient.escapeString(attributeName.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "DELETE", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call deleteAttributeValidateBeforeCall(String attributeCategory, String attributeName, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'attributeCategory' is set
if (attributeCategory == null) {
throw new ApiException("Missing the required parameter 'attributeCategory' when calling deleteAttribute(Async)");
}
// verify the required parameter 'attributeName' is set
if (attributeName == null) {
throw new ApiException("Missing the required parameter 'attributeName' when calling deleteAttribute(Async)");
}
Call call = deleteAttributeCall(attributeCategory, attributeName, progressListener, progressRequestListener);
return call;
}
/**
* Delete an attribute
*
* @param attributeCategory Category of the attribute (required)
* @param attributeName Name of the existing attribute (required)
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public void deleteAttribute(String attributeCategory, String attributeName) throws ApiException {
deleteAttributeWithHttpInfo(attributeCategory, attributeName);
}
/**
* Delete an attribute
*
* @param attributeCategory Category of the attribute (required)
* @param attributeName Name of the existing attribute (required)
* @return ApiResponse<Void>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse deleteAttributeWithHttpInfo(String attributeCategory, String attributeName) throws ApiException {
Call call = deleteAttributeValidateBeforeCall(attributeCategory, attributeName, null, null);
return apiClient.execute(call);
}
/**
* Delete an attribute (asynchronously)
*
* @param attributeCategory Category of the attribute (required)
* @param attributeName Name of the existing attribute (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call deleteAttributeAsync(String attributeCategory, String attributeName, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = deleteAttributeValidateBeforeCall(attributeCategory, attributeName, progressListener, progressRequestListener);
apiClient.executeAsync(call, callback);
return call;
}
/**
* Build call for deleteContact
* @param identifier Email (urlencoded) OR ID of the contact (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call deleteContactCall(String identifier, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/contacts/{identifier}"
.replaceAll("\\{" + "identifier" + "\\}", apiClient.escapeString(identifier.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "DELETE", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call deleteContactValidateBeforeCall(String identifier, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'identifier' is set
if (identifier == null) {
throw new ApiException("Missing the required parameter 'identifier' when calling deleteContact(Async)");
}
Call call = deleteContactCall(identifier, progressListener, progressRequestListener);
return call;
}
/**
* Delete a contact
*
* @param identifier Email (urlencoded) OR ID of the contact (required)
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public void deleteContact(String identifier) throws ApiException {
deleteContactWithHttpInfo(identifier);
}
/**
* Delete a contact
*
* @param identifier Email (urlencoded) OR ID of the contact (required)
* @return ApiResponse<Void>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse deleteContactWithHttpInfo(String identifier) throws ApiException {
Call call = deleteContactValidateBeforeCall(identifier, null, null);
return apiClient.execute(call);
}
/**
* Delete a contact (asynchronously)
*
* @param identifier Email (urlencoded) OR ID of the contact (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call deleteContactAsync(String identifier, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = deleteContactValidateBeforeCall(identifier, progressListener, progressRequestListener);
apiClient.executeAsync(call, callback);
return call;
}
/**
* Build call for deleteFolder
* @param folderId Id of the folder (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call deleteFolderCall(Long folderId, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/contacts/folders/{folderId}"
.replaceAll("\\{" + "folderId" + "\\}", apiClient.escapeString(folderId.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "DELETE", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call deleteFolderValidateBeforeCall(Long folderId, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'folderId' is set
if (folderId == null) {
throw new ApiException("Missing the required parameter 'folderId' when calling deleteFolder(Async)");
}
Call call = deleteFolderCall(folderId, progressListener, progressRequestListener);
return call;
}
/**
* Delete a folder (and all its lists)
*
* @param folderId Id of the folder (required)
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public void deleteFolder(Long folderId) throws ApiException {
deleteFolderWithHttpInfo(folderId);
}
/**
* Delete a folder (and all its lists)
*
* @param folderId Id of the folder (required)
* @return ApiResponse<Void>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse deleteFolderWithHttpInfo(Long folderId) throws ApiException {
Call call = deleteFolderValidateBeforeCall(folderId, null, null);
return apiClient.execute(call);
}
/**
* Delete a folder (and all its lists) (asynchronously)
*
* @param folderId Id of the folder (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call deleteFolderAsync(Long folderId, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = deleteFolderValidateBeforeCall(folderId, progressListener, progressRequestListener);
apiClient.executeAsync(call, callback);
return call;
}
/**
* Build call for deleteList
* @param listId Id of the list (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call deleteListCall(Long listId, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/contacts/lists/{listId}"
.replaceAll("\\{" + "listId" + "\\}", apiClient.escapeString(listId.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "DELETE", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call deleteListValidateBeforeCall(Long listId, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'listId' is set
if (listId == null) {
throw new ApiException("Missing the required parameter 'listId' when calling deleteList(Async)");
}
Call call = deleteListCall(listId, progressListener, progressRequestListener);
return call;
}
/**
* Delete a list
*
* @param listId Id of the list (required)
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public void deleteList(Long listId) throws ApiException {
deleteListWithHttpInfo(listId);
}
/**
* Delete a list
*
* @param listId Id of the list (required)
* @return ApiResponse<Void>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse deleteListWithHttpInfo(Long listId) throws ApiException {
Call call = deleteListValidateBeforeCall(listId, null, null);
return apiClient.execute(call);
}
/**
* Delete a list (asynchronously)
*
* @param listId Id of the list (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call deleteListAsync(Long listId, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = deleteListValidateBeforeCall(listId, progressListener, progressRequestListener);
apiClient.executeAsync(call, callback);
return call;
}
/**
* Build call for getAttributes
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call getAttributesCall(final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/contacts/attributes";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call getAttributesValidateBeforeCall(final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Call call = getAttributesCall(progressListener, progressRequestListener);
return call;
}
/**
* List all attributes
*
* @return GetAttributes
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public GetAttributes getAttributes() throws ApiException {
ApiResponse resp = getAttributesWithHttpInfo();
return resp.getData();
}
/**
* List all attributes
*
* @return ApiResponse<GetAttributes>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getAttributesWithHttpInfo() throws ApiException {
Call call = getAttributesValidateBeforeCall(null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* List all attributes (asynchronously)
*
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call getAttributesAsync(final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = getAttributesValidateBeforeCall(progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for getContactInfo
* @param identifier Email (urlencoded) OR ID of the contact OR its SMS attribute value (required)
* @param startDate **Mandatory if endDate is used.** Starting date (YYYY-MM-DD) of the statistic events specific to campaigns. Must be lower than equal to endDate (optional)
* @param endDate **Mandatory if startDate is used.** Ending date (YYYY-MM-DD) of the statistic events specific to campaigns. Must be greater than equal to startDate. (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call getContactInfoCall(String identifier, Object startDate, Object endDate, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/contacts/{identifier}"
.replaceAll("\\{" + "identifier" + "\\}", apiClient.escapeString(identifier.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
if (startDate != null)
localVarQueryParams.addAll(apiClient.parameterToPair("startDate", startDate));
if (endDate != null)
localVarQueryParams.addAll(apiClient.parameterToPair("endDate", endDate));
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call getContactInfoValidateBeforeCall(String identifier, Object startDate, Object endDate, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'identifier' is set
if (identifier == null) {
throw new ApiException("Missing the required parameter 'identifier' when calling getContactInfo(Async)");
}
Call call = getContactInfoCall(identifier, startDate, endDate, progressListener, progressRequestListener);
return call;
}
/**
* Get a contact's details
* Along with the contact details, this endpoint will show the statistics of contact for the recent 90 days by default. To fetch the earlier statistics, please use Get contact campaign stats (https://developers.sendinblue.com/reference/contacts-7#getcontactstats) endpoint with the appropriate date ranges.
* @param identifier Email (urlencoded) OR ID of the contact OR its SMS attribute value (required)
* @param startDate **Mandatory if endDate is used.** Starting date (YYYY-MM-DD) of the statistic events specific to campaigns. Must be lower than equal to endDate (optional)
* @param endDate **Mandatory if startDate is used.** Ending date (YYYY-MM-DD) of the statistic events specific to campaigns. Must be greater than equal to startDate. (optional)
* @return GetExtendedContactDetails
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public GetExtendedContactDetails getContactInfo(String identifier, Object startDate, Object endDate) throws ApiException {
ApiResponse resp = getContactInfoWithHttpInfo(identifier, startDate, endDate);
return resp.getData();
}
/**
* Get a contact's details
* Along with the contact details, this endpoint will show the statistics of contact for the recent 90 days by default. To fetch the earlier statistics, please use Get contact campaign stats (https://developers.sendinblue.com/reference/contacts-7#getcontactstats) endpoint with the appropriate date ranges.
* @param identifier Email (urlencoded) OR ID of the contact OR its SMS attribute value (required)
* @param startDate **Mandatory if endDate is used.** Starting date (YYYY-MM-DD) of the statistic events specific to campaigns. Must be lower than equal to endDate (optional)
* @param endDate **Mandatory if startDate is used.** Ending date (YYYY-MM-DD) of the statistic events specific to campaigns. Must be greater than equal to startDate. (optional)
* @return ApiResponse<GetExtendedContactDetails>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getContactInfoWithHttpInfo(String identifier, Object startDate, Object endDate) throws ApiException {
Call call = getContactInfoValidateBeforeCall(identifier, startDate, endDate, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get a contact's details (asynchronously)
* Along with the contact details, this endpoint will show the statistics of contact for the recent 90 days by default. To fetch the earlier statistics, please use Get contact campaign stats (https://developers.sendinblue.com/reference/contacts-7#getcontactstats) endpoint with the appropriate date ranges.
* @param identifier Email (urlencoded) OR ID of the contact OR its SMS attribute value (required)
* @param startDate **Mandatory if endDate is used.** Starting date (YYYY-MM-DD) of the statistic events specific to campaigns. Must be lower than equal to endDate (optional)
* @param endDate **Mandatory if startDate is used.** Ending date (YYYY-MM-DD) of the statistic events specific to campaigns. Must be greater than equal to startDate. (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call getContactInfoAsync(String identifier, Object startDate, Object endDate, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = getContactInfoValidateBeforeCall(identifier, startDate, endDate, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for getContactStats
* @param identifier Email (urlencoded) OR ID of the contact (required)
* @param startDate Mandatory if endDate is used. Starting date (YYYY-MM-DD) of the statistic events specific to campaigns. Must be lower than equal to endDate (optional)
* @param endDate Mandatory if startDate is used. Ending date (YYYY-MM-DD) of the statistic events specific to campaigns. Must be greater than equal to startDate. Maximum difference between startDate and endDate should not be greater than 90 days (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call getContactStatsCall(String identifier, String startDate, String endDate, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/contacts/{identifier}/campaignStats"
.replaceAll("\\{" + "identifier" + "\\}", apiClient.escapeString(identifier.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
if (startDate != null)
localVarQueryParams.addAll(apiClient.parameterToPair("startDate", startDate));
if (endDate != null)
localVarQueryParams.addAll(apiClient.parameterToPair("endDate", endDate));
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call getContactStatsValidateBeforeCall(String identifier, String startDate, String endDate, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'identifier' is set
if (identifier == null) {
throw new ApiException("Missing the required parameter 'identifier' when calling getContactStats(Async)");
}
Call call = getContactStatsCall(identifier, startDate, endDate, progressListener, progressRequestListener);
return call;
}
/**
* Get email campaigns' statistics for a contact
*
* @param identifier Email (urlencoded) OR ID of the contact (required)
* @param startDate Mandatory if endDate is used. Starting date (YYYY-MM-DD) of the statistic events specific to campaigns. Must be lower than equal to endDate (optional)
* @param endDate Mandatory if startDate is used. Ending date (YYYY-MM-DD) of the statistic events specific to campaigns. Must be greater than equal to startDate. Maximum difference between startDate and endDate should not be greater than 90 days (optional)
* @return GetContactCampaignStats
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public GetContactCampaignStats getContactStats(String identifier, String startDate, String endDate) throws ApiException {
ApiResponse resp = getContactStatsWithHttpInfo(identifier, startDate, endDate);
return resp.getData();
}
/**
* Get email campaigns' statistics for a contact
*
* @param identifier Email (urlencoded) OR ID of the contact (required)
* @param startDate Mandatory if endDate is used. Starting date (YYYY-MM-DD) of the statistic events specific to campaigns. Must be lower than equal to endDate (optional)
* @param endDate Mandatory if startDate is used. Ending date (YYYY-MM-DD) of the statistic events specific to campaigns. Must be greater than equal to startDate. Maximum difference between startDate and endDate should not be greater than 90 days (optional)
* @return ApiResponse<GetContactCampaignStats>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getContactStatsWithHttpInfo(String identifier, String startDate, String endDate) throws ApiException {
Call call = getContactStatsValidateBeforeCall(identifier, startDate, endDate, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get email campaigns' statistics for a contact (asynchronously)
*
* @param identifier Email (urlencoded) OR ID of the contact (required)
* @param startDate Mandatory if endDate is used. Starting date (YYYY-MM-DD) of the statistic events specific to campaigns. Must be lower than equal to endDate (optional)
* @param endDate Mandatory if startDate is used. Ending date (YYYY-MM-DD) of the statistic events specific to campaigns. Must be greater than equal to startDate. Maximum difference between startDate and endDate should not be greater than 90 days (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call getContactStatsAsync(String identifier, String startDate, String endDate, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = getContactStatsValidateBeforeCall(identifier, startDate, endDate, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for getContacts
* @param limit Number of documents per page (optional, default to 50)
* @param offset Index of the first document of the page (optional, default to 0)
* @param modifiedSince Filter (urlencoded) the contacts modified after a given UTC date-time (YYYY-MM-DDTHH:mm:ss.SSSZ). Prefer to pass your timezone in date-time format for accurate result. (optional)
* @param sort Sort the results in the ascending/descending order of record creation. Default order is **descending** if `sort` is not passed (optional, default to desc)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call getContactsCall(Long limit, Long offset, String modifiedSince, String sort, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/contacts";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
if (limit != null)
localVarQueryParams.addAll(apiClient.parameterToPair("limit", limit));
if (offset != null)
localVarQueryParams.addAll(apiClient.parameterToPair("offset", offset));
if (modifiedSince != null)
localVarQueryParams.addAll(apiClient.parameterToPair("modifiedSince", modifiedSince));
if (sort != null)
localVarQueryParams.addAll(apiClient.parameterToPair("sort", sort));
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call getContactsValidateBeforeCall(Long limit, Long offset, String modifiedSince, String sort, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Call call = getContactsCall(limit, offset, modifiedSince, sort, progressListener, progressRequestListener);
return call;
}
/**
* Get all the contacts
*
* @param limit Number of documents per page (optional, default to 50)
* @param offset Index of the first document of the page (optional, default to 0)
* @param modifiedSince Filter (urlencoded) the contacts modified after a given UTC date-time (YYYY-MM-DDTHH:mm:ss.SSSZ). Prefer to pass your timezone in date-time format for accurate result. (optional)
* @param sort Sort the results in the ascending/descending order of record creation. Default order is **descending** if `sort` is not passed (optional, default to desc)
* @return GetContacts
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public GetContacts getContacts(Long limit, Long offset, String modifiedSince, String sort) throws ApiException {
ApiResponse resp = getContactsWithHttpInfo(limit, offset, modifiedSince, sort);
return resp.getData();
}
/**
* Get all the contacts
*
* @param limit Number of documents per page (optional, default to 50)
* @param offset Index of the first document of the page (optional, default to 0)
* @param modifiedSince Filter (urlencoded) the contacts modified after a given UTC date-time (YYYY-MM-DDTHH:mm:ss.SSSZ). Prefer to pass your timezone in date-time format for accurate result. (optional)
* @param sort Sort the results in the ascending/descending order of record creation. Default order is **descending** if `sort` is not passed (optional, default to desc)
* @return ApiResponse<GetContacts>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getContactsWithHttpInfo(Long limit, Long offset, String modifiedSince, String sort) throws ApiException {
Call call = getContactsValidateBeforeCall(limit, offset, modifiedSince, sort, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get all the contacts (asynchronously)
*
* @param limit Number of documents per page (optional, default to 50)
* @param offset Index of the first document of the page (optional, default to 0)
* @param modifiedSince Filter (urlencoded) the contacts modified after a given UTC date-time (YYYY-MM-DDTHH:mm:ss.SSSZ). Prefer to pass your timezone in date-time format for accurate result. (optional)
* @param sort Sort the results in the ascending/descending order of record creation. Default order is **descending** if `sort` is not passed (optional, default to desc)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call getContactsAsync(Long limit, Long offset, String modifiedSince, String sort, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = getContactsValidateBeforeCall(limit, offset, modifiedSince, sort, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for getContactsFromList
* @param listId Id of the list (required)
* @param modifiedSince Filter (urlencoded) the contacts modified after a given UTC date-time (YYYY-MM-DDTHH:mm:ss.SSSZ). Prefer to pass your timezone in date-time format for accurate result. (optional)
* @param limit Number of documents per page (optional, default to 50)
* @param offset Index of the first document of the page (optional, default to 0)
* @param sort Sort the results in the ascending/descending order of record creation. Default order is **descending** if `sort` is not passed (optional, default to desc)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call getContactsFromListCall(Long listId, String modifiedSince, Long limit, Long offset, String sort, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/contacts/lists/{listId}/contacts"
.replaceAll("\\{" + "listId" + "\\}", apiClient.escapeString(listId.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
if (modifiedSince != null)
localVarQueryParams.addAll(apiClient.parameterToPair("modifiedSince", modifiedSince));
if (limit != null)
localVarQueryParams.addAll(apiClient.parameterToPair("limit", limit));
if (offset != null)
localVarQueryParams.addAll(apiClient.parameterToPair("offset", offset));
if (sort != null)
localVarQueryParams.addAll(apiClient.parameterToPair("sort", sort));
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call getContactsFromListValidateBeforeCall(Long listId, String modifiedSince, Long limit, Long offset, String sort, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'listId' is set
if (listId == null) {
throw new ApiException("Missing the required parameter 'listId' when calling getContactsFromList(Async)");
}
Call call = getContactsFromListCall(listId, modifiedSince, limit, offset, sort, progressListener, progressRequestListener);
return call;
}
/**
* Get contacts in a list
*
* @param listId Id of the list (required)
* @param modifiedSince Filter (urlencoded) the contacts modified after a given UTC date-time (YYYY-MM-DDTHH:mm:ss.SSSZ). Prefer to pass your timezone in date-time format for accurate result. (optional)
* @param limit Number of documents per page (optional, default to 50)
* @param offset Index of the first document of the page (optional, default to 0)
* @param sort Sort the results in the ascending/descending order of record creation. Default order is **descending** if `sort` is not passed (optional, default to desc)
* @return GetContacts
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public GetContacts getContactsFromList(Long listId, String modifiedSince, Long limit, Long offset, String sort) throws ApiException {
ApiResponse resp = getContactsFromListWithHttpInfo(listId, modifiedSince, limit, offset, sort);
return resp.getData();
}
/**
* Get contacts in a list
*
* @param listId Id of the list (required)
* @param modifiedSince Filter (urlencoded) the contacts modified after a given UTC date-time (YYYY-MM-DDTHH:mm:ss.SSSZ). Prefer to pass your timezone in date-time format for accurate result. (optional)
* @param limit Number of documents per page (optional, default to 50)
* @param offset Index of the first document of the page (optional, default to 0)
* @param sort Sort the results in the ascending/descending order of record creation. Default order is **descending** if `sort` is not passed (optional, default to desc)
* @return ApiResponse<GetContacts>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getContactsFromListWithHttpInfo(Long listId, String modifiedSince, Long limit, Long offset, String sort) throws ApiException {
Call call = getContactsFromListValidateBeforeCall(listId, modifiedSince, limit, offset, sort, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get contacts in a list (asynchronously)
*
* @param listId Id of the list (required)
* @param modifiedSince Filter (urlencoded) the contacts modified after a given UTC date-time (YYYY-MM-DDTHH:mm:ss.SSSZ). Prefer to pass your timezone in date-time format for accurate result. (optional)
* @param limit Number of documents per page (optional, default to 50)
* @param offset Index of the first document of the page (optional, default to 0)
* @param sort Sort the results in the ascending/descending order of record creation. Default order is **descending** if `sort` is not passed (optional, default to desc)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call getContactsFromListAsync(Long listId, String modifiedSince, Long limit, Long offset, String sort, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = getContactsFromListValidateBeforeCall(listId, modifiedSince, limit, offset, sort, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for getFolder
* @param folderId id of the folder (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call getFolderCall(Long folderId, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/contacts/folders/{folderId}"
.replaceAll("\\{" + "folderId" + "\\}", apiClient.escapeString(folderId.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call getFolderValidateBeforeCall(Long folderId, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'folderId' is set
if (folderId == null) {
throw new ApiException("Missing the required parameter 'folderId' when calling getFolder(Async)");
}
Call call = getFolderCall(folderId, progressListener, progressRequestListener);
return call;
}
/**
* Returns a folder's details
*
* @param folderId id of the folder (required)
* @return GetFolder
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public GetFolder getFolder(Long folderId) throws ApiException {
ApiResponse resp = getFolderWithHttpInfo(folderId);
return resp.getData();
}
/**
* Returns a folder's details
*
* @param folderId id of the folder (required)
* @return ApiResponse<GetFolder>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getFolderWithHttpInfo(Long folderId) throws ApiException {
Call call = getFolderValidateBeforeCall(folderId, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Returns a folder's details (asynchronously)
*
* @param folderId id of the folder (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call getFolderAsync(Long folderId, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = getFolderValidateBeforeCall(folderId, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for getFolderLists
* @param folderId Id of the folder (required)
* @param limit Number of documents per page (optional, default to 10)
* @param offset Index of the first document of the page (optional, default to 0)
* @param sort Sort the results in the ascending/descending order of record creation. Default order is **descending** if `sort` is not passed (optional, default to desc)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call getFolderListsCall(Long folderId, Long limit, Long offset, String sort, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/contacts/folders/{folderId}/lists"
.replaceAll("\\{" + "folderId" + "\\}", apiClient.escapeString(folderId.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
if (limit != null)
localVarQueryParams.addAll(apiClient.parameterToPair("limit", limit));
if (offset != null)
localVarQueryParams.addAll(apiClient.parameterToPair("offset", offset));
if (sort != null)
localVarQueryParams.addAll(apiClient.parameterToPair("sort", sort));
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call getFolderListsValidateBeforeCall(Long folderId, Long limit, Long offset, String sort, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'folderId' is set
if (folderId == null) {
throw new ApiException("Missing the required parameter 'folderId' when calling getFolderLists(Async)");
}
Call call = getFolderListsCall(folderId, limit, offset, sort, progressListener, progressRequestListener);
return call;
}
/**
* Get lists in a folder
*
* @param folderId Id of the folder (required)
* @param limit Number of documents per page (optional, default to 10)
* @param offset Index of the first document of the page (optional, default to 0)
* @param sort Sort the results in the ascending/descending order of record creation. Default order is **descending** if `sort` is not passed (optional, default to desc)
* @return GetFolderLists
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public GetFolderLists getFolderLists(Long folderId, Long limit, Long offset, String sort) throws ApiException {
ApiResponse resp = getFolderListsWithHttpInfo(folderId, limit, offset, sort);
return resp.getData();
}
/**
* Get lists in a folder
*
* @param folderId Id of the folder (required)
* @param limit Number of documents per page (optional, default to 10)
* @param offset Index of the first document of the page (optional, default to 0)
* @param sort Sort the results in the ascending/descending order of record creation. Default order is **descending** if `sort` is not passed (optional, default to desc)
* @return ApiResponse<GetFolderLists>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getFolderListsWithHttpInfo(Long folderId, Long limit, Long offset, String sort) throws ApiException {
Call call = getFolderListsValidateBeforeCall(folderId, limit, offset, sort, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get lists in a folder (asynchronously)
*
* @param folderId Id of the folder (required)
* @param limit Number of documents per page (optional, default to 10)
* @param offset Index of the first document of the page (optional, default to 0)
* @param sort Sort the results in the ascending/descending order of record creation. Default order is **descending** if `sort` is not passed (optional, default to desc)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call getFolderListsAsync(Long folderId, Long limit, Long offset, String sort, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = getFolderListsValidateBeforeCall(folderId, limit, offset, sort, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for getFolders
* @param limit Number of documents per page (required)
* @param offset Index of the first document of the page (required)
* @param sort Sort the results in the ascending/descending order of record creation. Default order is **descending** if `sort` is not passed (optional, default to desc)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call getFoldersCall(Long limit, Long offset, String sort, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/contacts/folders";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
if (limit != null)
localVarQueryParams.addAll(apiClient.parameterToPair("limit", limit));
if (offset != null)
localVarQueryParams.addAll(apiClient.parameterToPair("offset", offset));
if (sort != null)
localVarQueryParams.addAll(apiClient.parameterToPair("sort", sort));
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call getFoldersValidateBeforeCall(Long limit, Long offset, String sort, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'limit' is set
if (limit == null) {
throw new ApiException("Missing the required parameter 'limit' when calling getFolders(Async)");
}
// verify the required parameter 'offset' is set
if (offset == null) {
throw new ApiException("Missing the required parameter 'offset' when calling getFolders(Async)");
}
Call call = getFoldersCall(limit, offset, sort, progressListener, progressRequestListener);
return call;
}
/**
* Get all folders
*
* @param limit Number of documents per page (required)
* @param offset Index of the first document of the page (required)
* @param sort Sort the results in the ascending/descending order of record creation. Default order is **descending** if `sort` is not passed (optional, default to desc)
* @return GetFolders
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public GetFolders getFolders(Long limit, Long offset, String sort) throws ApiException {
ApiResponse resp = getFoldersWithHttpInfo(limit, offset, sort);
return resp.getData();
}
/**
* Get all folders
*
* @param limit Number of documents per page (required)
* @param offset Index of the first document of the page (required)
* @param sort Sort the results in the ascending/descending order of record creation. Default order is **descending** if `sort` is not passed (optional, default to desc)
* @return ApiResponse<GetFolders>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getFoldersWithHttpInfo(Long limit, Long offset, String sort) throws ApiException {
Call call = getFoldersValidateBeforeCall(limit, offset, sort, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get all folders (asynchronously)
*
* @param limit Number of documents per page (required)
* @param offset Index of the first document of the page (required)
* @param sort Sort the results in the ascending/descending order of record creation. Default order is **descending** if `sort` is not passed (optional, default to desc)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call getFoldersAsync(Long limit, Long offset, String sort, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = getFoldersValidateBeforeCall(limit, offset, sort, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for getList
* @param listId Id of the list (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call getListCall(Long listId, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/contacts/lists/{listId}"
.replaceAll("\\{" + "listId" + "\\}", apiClient.escapeString(listId.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call getListValidateBeforeCall(Long listId, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'listId' is set
if (listId == null) {
throw new ApiException("Missing the required parameter 'listId' when calling getList(Async)");
}
Call call = getListCall(listId, progressListener, progressRequestListener);
return call;
}
/**
* Get a list's details
*
* @param listId Id of the list (required)
* @return GetExtendedList
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public GetExtendedList getList(Long listId) throws ApiException {
ApiResponse resp = getListWithHttpInfo(listId);
return resp.getData();
}
/**
* Get a list's details
*
* @param listId Id of the list (required)
* @return ApiResponse<GetExtendedList>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getListWithHttpInfo(Long listId) throws ApiException {
Call call = getListValidateBeforeCall(listId, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get a list's details (asynchronously)
*
* @param listId Id of the list (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call getListAsync(Long listId, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = getListValidateBeforeCall(listId, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for getLists
* @param limit Number of documents per page (optional, default to 10)
* @param offset Index of the first document of the page (optional, default to 0)
* @param sort Sort the results in the ascending/descending order of record creation. Default order is **descending** if `sort` is not passed (optional, default to desc)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call getListsCall(Long limit, Long offset, String sort, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/contacts/lists";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
if (limit != null)
localVarQueryParams.addAll(apiClient.parameterToPair("limit", limit));
if (offset != null)
localVarQueryParams.addAll(apiClient.parameterToPair("offset", offset));
if (sort != null)
localVarQueryParams.addAll(apiClient.parameterToPair("sort", sort));
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call getListsValidateBeforeCall(Long limit, Long offset, String sort, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Call call = getListsCall(limit, offset, sort, progressListener, progressRequestListener);
return call;
}
/**
* Get all the lists
*
* @param limit Number of documents per page (optional, default to 10)
* @param offset Index of the first document of the page (optional, default to 0)
* @param sort Sort the results in the ascending/descending order of record creation. Default order is **descending** if `sort` is not passed (optional, default to desc)
* @return GetLists
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public GetLists getLists(Long limit, Long offset, String sort) throws ApiException {
ApiResponse resp = getListsWithHttpInfo(limit, offset, sort);
return resp.getData();
}
/**
* Get all the lists
*
* @param limit Number of documents per page (optional, default to 10)
* @param offset Index of the first document of the page (optional, default to 0)
* @param sort Sort the results in the ascending/descending order of record creation. Default order is **descending** if `sort` is not passed (optional, default to desc)
* @return ApiResponse<GetLists>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getListsWithHttpInfo(Long limit, Long offset, String sort) throws ApiException {
Call call = getListsValidateBeforeCall(limit, offset, sort, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get all the lists (asynchronously)
*
* @param limit Number of documents per page (optional, default to 10)
* @param offset Index of the first document of the page (optional, default to 0)
* @param sort Sort the results in the ascending/descending order of record creation. Default order is **descending** if `sort` is not passed (optional, default to desc)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call getListsAsync(Long limit, Long offset, String sort, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = getListsValidateBeforeCall(limit, offset, sort, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for importContacts
* @param requestContactImport Values to import contacts in Sendinblue. To know more about the expected format, please have a look at ``https://help.sendinblue.com/hc/en-us/articles/209499265-Build-contacts-lists-for-your-email-marketing-campaigns`` (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call importContactsCall(RequestContactImport requestContactImport, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = requestContactImport;
// create path and map variables
String localVarPath = "/contacts/import";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call importContactsValidateBeforeCall(RequestContactImport requestContactImport, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'requestContactImport' is set
if (requestContactImport == null) {
throw new ApiException("Missing the required parameter 'requestContactImport' when calling importContacts(Async)");
}
Call call = importContactsCall(requestContactImport, progressListener, progressRequestListener);
return call;
}
/**
* Import contacts
* It returns the background process ID which on completion calls the notify URL that you have set in the input.
* @param requestContactImport Values to import contacts in Sendinblue. To know more about the expected format, please have a look at ``https://help.sendinblue.com/hc/en-us/articles/209499265-Build-contacts-lists-for-your-email-marketing-campaigns`` (required)
* @return CreatedProcessId
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public CreatedProcessId importContacts(RequestContactImport requestContactImport) throws ApiException {
ApiResponse