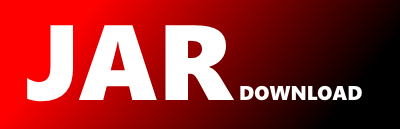
sibApi.EmailCampaignsApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sib-api-v3-sdk Show documentation
Show all versions of sib-api-v3-sdk Show documentation
SendinBlue's API v3 Java Library
The newest version!
/*
* SendinBlue API
* SendinBlue provide a RESTFul API that can be used with any languages. With this API, you will be able to : - Manage your campaigns and get the statistics - Manage your contacts - Send transactional Emails and SMS - and much more... You can download our wrappers at https://github.com/orgs/sendinblue **Possible responses** | Code | Message | | :-------------: | ------------- | | 200 | OK. Successful Request | | 201 | OK. Successful Creation | | 202 | OK. Request accepted | | 204 | OK. Successful Update/Deletion | | 400 | Error. Bad Request | | 401 | Error. Authentication Needed | | 402 | Error. Not enough credit, plan upgrade needed | | 403 | Error. Permission denied | | 404 | Error. Object does not exist | | 405 | Error. Method not allowed | | 406 | Error. Not Acceptable |
*
* OpenAPI spec version: 3.0.0
* Contact: [email protected]
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package sibApi;
import okhttp3.Call;
import sendinblue.ApiCallback;
import sendinblue.ApiClient;
import sendinblue.ApiException;
import sendinblue.ApiResponse;
import sendinblue.Configuration;
import sendinblue.Pair;
import sendinblue.ProgressRequestBody;
import sendinblue.ProgressResponseBody;
import com.google.gson.reflect.TypeToken;
import java.io.IOException;
import sibModel.AbTestCampaignResult;
import sibModel.CreateEmailCampaign;
import sibModel.CreateModel;
import sibModel.CreatedProcessId;
import sibModel.EmailExportRecipients;
import sibModel.ErrorModel;
import sibModel.GetEmailCampaign;
import sibModel.GetEmailCampaigns;
import sibModel.GetSharedTemplateUrl;
import sibModel.PostSendFailed;
import sibModel.SendReport;
import sibModel.SendTestEmail;
import sibModel.UpdateCampaignStatus;
import sibModel.UpdateEmailCampaign;
import sibModel.UploadImageModel;
import sibModel.UploadImageToGallery;
import java.lang.reflect.Type;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class EmailCampaignsApi {
private ApiClient apiClient;
public EmailCampaignsApi() {
this(Configuration.getDefaultApiClient());
}
public EmailCampaignsApi(ApiClient apiClient) {
this.apiClient = apiClient;
}
public ApiClient getApiClient() {
return apiClient;
}
public void setApiClient(ApiClient apiClient) {
this.apiClient = apiClient;
}
/**
* Build call for createEmailCampaign
* @param emailCampaigns Values to create a campaign (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call createEmailCampaignCall(CreateEmailCampaign emailCampaigns, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = emailCampaigns;
// create path and map variables
String localVarPath = "/emailCampaigns";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call createEmailCampaignValidateBeforeCall(CreateEmailCampaign emailCampaigns, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'emailCampaigns' is set
if (emailCampaigns == null) {
throw new ApiException("Missing the required parameter 'emailCampaigns' when calling createEmailCampaign(Async)");
}
Call call = createEmailCampaignCall(emailCampaigns, progressListener, progressRequestListener);
return call;
}
/**
* Create an email campaign
*
* @param emailCampaigns Values to create a campaign (required)
* @return CreateModel
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public CreateModel createEmailCampaign(CreateEmailCampaign emailCampaigns) throws ApiException {
ApiResponse resp = createEmailCampaignWithHttpInfo(emailCampaigns);
return resp.getData();
}
/**
* Create an email campaign
*
* @param emailCampaigns Values to create a campaign (required)
* @return ApiResponse<CreateModel>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse createEmailCampaignWithHttpInfo(CreateEmailCampaign emailCampaigns) throws ApiException {
Call call = createEmailCampaignValidateBeforeCall(emailCampaigns, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Create an email campaign (asynchronously)
*
* @param emailCampaigns Values to create a campaign (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call createEmailCampaignAsync(CreateEmailCampaign emailCampaigns, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = createEmailCampaignValidateBeforeCall(emailCampaigns, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for deleteEmailCampaign
* @param campaignId id of the campaign (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call deleteEmailCampaignCall(Long campaignId, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/emailCampaigns/{campaignId}"
.replaceAll("\\{" + "campaignId" + "\\}", apiClient.escapeString(campaignId.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "DELETE", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call deleteEmailCampaignValidateBeforeCall(Long campaignId, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'campaignId' is set
if (campaignId == null) {
throw new ApiException("Missing the required parameter 'campaignId' when calling deleteEmailCampaign(Async)");
}
Call call = deleteEmailCampaignCall(campaignId, progressListener, progressRequestListener);
return call;
}
/**
* Delete an email campaign
*
* @param campaignId id of the campaign (required)
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public void deleteEmailCampaign(Long campaignId) throws ApiException {
deleteEmailCampaignWithHttpInfo(campaignId);
}
/**
* Delete an email campaign
*
* @param campaignId id of the campaign (required)
* @return ApiResponse<Void>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse deleteEmailCampaignWithHttpInfo(Long campaignId) throws ApiException {
Call call = deleteEmailCampaignValidateBeforeCall(campaignId, null, null);
return apiClient.execute(call);
}
/**
* Delete an email campaign (asynchronously)
*
* @param campaignId id of the campaign (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call deleteEmailCampaignAsync(Long campaignId, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = deleteEmailCampaignValidateBeforeCall(campaignId, progressListener, progressRequestListener);
apiClient.executeAsync(call, callback);
return call;
}
/**
* Build call for emailExportRecipients
* @param campaignId Id of the campaign (required)
* @param recipientExport Values to send for a recipient export request (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call emailExportRecipientsCall(Long campaignId, EmailExportRecipients recipientExport, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = recipientExport;
// create path and map variables
String localVarPath = "/emailCampaigns/{campaignId}/exportRecipients"
.replaceAll("\\{" + "campaignId" + "\\}", apiClient.escapeString(campaignId.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call emailExportRecipientsValidateBeforeCall(Long campaignId, EmailExportRecipients recipientExport, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'campaignId' is set
if (campaignId == null) {
throw new ApiException("Missing the required parameter 'campaignId' when calling emailExportRecipients(Async)");
}
Call call = emailExportRecipientsCall(campaignId, recipientExport, progressListener, progressRequestListener);
return call;
}
/**
* Export the recipients of an email campaign
*
* @param campaignId Id of the campaign (required)
* @param recipientExport Values to send for a recipient export request (optional)
* @return CreatedProcessId
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public CreatedProcessId emailExportRecipients(Long campaignId, EmailExportRecipients recipientExport) throws ApiException {
ApiResponse resp = emailExportRecipientsWithHttpInfo(campaignId, recipientExport);
return resp.getData();
}
/**
* Export the recipients of an email campaign
*
* @param campaignId Id of the campaign (required)
* @param recipientExport Values to send for a recipient export request (optional)
* @return ApiResponse<CreatedProcessId>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse emailExportRecipientsWithHttpInfo(Long campaignId, EmailExportRecipients recipientExport) throws ApiException {
Call call = emailExportRecipientsValidateBeforeCall(campaignId, recipientExport, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Export the recipients of an email campaign (asynchronously)
*
* @param campaignId Id of the campaign (required)
* @param recipientExport Values to send for a recipient export request (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call emailExportRecipientsAsync(Long campaignId, EmailExportRecipients recipientExport, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = emailExportRecipientsValidateBeforeCall(campaignId, recipientExport, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for getAbTestCampaignResult
* @param campaignId Id of the A/B test campaign (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call getAbTestCampaignResultCall(Long campaignId, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/emailCampaigns/{campaignId}/abTestCampaignResult"
.replaceAll("\\{" + "campaignId" + "\\}", apiClient.escapeString(campaignId.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call getAbTestCampaignResultValidateBeforeCall(Long campaignId, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'campaignId' is set
if (campaignId == null) {
throw new ApiException("Missing the required parameter 'campaignId' when calling getAbTestCampaignResult(Async)");
}
Call call = getAbTestCampaignResultCall(campaignId, progressListener, progressRequestListener);
return call;
}
/**
* Get an A/B test email campaign results
* Obtain winning version of an A/B test email campaign
* @param campaignId Id of the A/B test campaign (required)
* @return AbTestCampaignResult
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public AbTestCampaignResult getAbTestCampaignResult(Long campaignId) throws ApiException {
ApiResponse resp = getAbTestCampaignResultWithHttpInfo(campaignId);
return resp.getData();
}
/**
* Get an A/B test email campaign results
* Obtain winning version of an A/B test email campaign
* @param campaignId Id of the A/B test campaign (required)
* @return ApiResponse<AbTestCampaignResult>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getAbTestCampaignResultWithHttpInfo(Long campaignId) throws ApiException {
Call call = getAbTestCampaignResultValidateBeforeCall(campaignId, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get an A/B test email campaign results (asynchronously)
* Obtain winning version of an A/B test email campaign
* @param campaignId Id of the A/B test campaign (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call getAbTestCampaignResultAsync(Long campaignId, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = getAbTestCampaignResultValidateBeforeCall(campaignId, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for getEmailCampaign
* @param campaignId Id of the campaign (required)
* @param statistics Filter on the type of statistics required. Example **globalStats** value will only fetch globalStats info of the campaign in returned response. (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call getEmailCampaignCall(Long campaignId, String statistics, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/emailCampaigns/{campaignId}"
.replaceAll("\\{" + "campaignId" + "\\}", apiClient.escapeString(campaignId.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
if (statistics != null)
localVarQueryParams.addAll(apiClient.parameterToPair("statistics", statistics));
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call getEmailCampaignValidateBeforeCall(Long campaignId, String statistics, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'campaignId' is set
if (campaignId == null) {
throw new ApiException("Missing the required parameter 'campaignId' when calling getEmailCampaign(Async)");
}
Call call = getEmailCampaignCall(campaignId, statistics, progressListener, progressRequestListener);
return call;
}
/**
* Get an email campaign report
*
* @param campaignId Id of the campaign (required)
* @param statistics Filter on the type of statistics required. Example **globalStats** value will only fetch globalStats info of the campaign in returned response. (optional)
* @return GetEmailCampaign
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public GetEmailCampaign getEmailCampaign(Long campaignId, String statistics) throws ApiException {
ApiResponse resp = getEmailCampaignWithHttpInfo(campaignId, statistics);
return resp.getData();
}
/**
* Get an email campaign report
*
* @param campaignId Id of the campaign (required)
* @param statistics Filter on the type of statistics required. Example **globalStats** value will only fetch globalStats info of the campaign in returned response. (optional)
* @return ApiResponse<GetEmailCampaign>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getEmailCampaignWithHttpInfo(Long campaignId, String statistics) throws ApiException {
Call call = getEmailCampaignValidateBeforeCall(campaignId, statistics, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get an email campaign report (asynchronously)
*
* @param campaignId Id of the campaign (required)
* @param statistics Filter on the type of statistics required. Example **globalStats** value will only fetch globalStats info of the campaign in returned response. (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call getEmailCampaignAsync(Long campaignId, String statistics, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = getEmailCampaignValidateBeforeCall(campaignId, statistics, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for getEmailCampaigns
* @param type Filter on the type of the campaigns (optional)
* @param status Filter on the status of the campaign (optional)
* @param statistics Filter on the type of statistics required. Example **globalStats** value will only fetch globalStats info of the campaign in returned response. (optional)
* @param startDate Mandatory if endDate is used. Starting (urlencoded) UTC date-time (YYYY-MM-DDTHH:mm:ss.SSSZ) to filter the sent email campaigns. Prefer to pass your timezone in date-time format for accurate result ( only available if either 'status' not passed and if passed is set to 'sent' ) (optional)
* @param endDate Mandatory if startDate is used. Ending (urlencoded) UTC date-time (YYYY-MM-DDTHH:mm:ss.SSSZ) to filter the sent email campaigns. Prefer to pass your timezone in date-time format for accurate result ( only available if either 'status' not passed and if passed is set to 'sent' ) (optional)
* @param limit Number of documents per page (optional, default to 50)
* @param offset Index of the first document in the page (optional, default to 0)
* @param sort Sort the results in the ascending/descending order of record creation. Default order is **descending** if `sort` is not passed (optional, default to desc)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call getEmailCampaignsCall(String type, String status, String statistics, String startDate, String endDate, Long limit, Long offset, String sort, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/emailCampaigns";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
if (type != null)
localVarQueryParams.addAll(apiClient.parameterToPair("type", type));
if (status != null)
localVarQueryParams.addAll(apiClient.parameterToPair("status", status));
if (statistics != null)
localVarQueryParams.addAll(apiClient.parameterToPair("statistics", statistics));
if (startDate != null)
localVarQueryParams.addAll(apiClient.parameterToPair("startDate", startDate));
if (endDate != null)
localVarQueryParams.addAll(apiClient.parameterToPair("endDate", endDate));
if (limit != null)
localVarQueryParams.addAll(apiClient.parameterToPair("limit", limit));
if (offset != null)
localVarQueryParams.addAll(apiClient.parameterToPair("offset", offset));
if (sort != null)
localVarQueryParams.addAll(apiClient.parameterToPair("sort", sort));
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call getEmailCampaignsValidateBeforeCall(String type, String status, String statistics, String startDate, String endDate, Long limit, Long offset, String sort, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Call call = getEmailCampaignsCall(type, status, statistics, startDate, endDate, limit, offset, sort, progressListener, progressRequestListener);
return call;
}
/**
* Return all your created email campaigns
*
* @param type Filter on the type of the campaigns (optional)
* @param status Filter on the status of the campaign (optional)
* @param statistics Filter on the type of statistics required. Example **globalStats** value will only fetch globalStats info of the campaign in returned response. (optional)
* @param startDate Mandatory if endDate is used. Starting (urlencoded) UTC date-time (YYYY-MM-DDTHH:mm:ss.SSSZ) to filter the sent email campaigns. Prefer to pass your timezone in date-time format for accurate result ( only available if either 'status' not passed and if passed is set to 'sent' ) (optional)
* @param endDate Mandatory if startDate is used. Ending (urlencoded) UTC date-time (YYYY-MM-DDTHH:mm:ss.SSSZ) to filter the sent email campaigns. Prefer to pass your timezone in date-time format for accurate result ( only available if either 'status' not passed and if passed is set to 'sent' ) (optional)
* @param limit Number of documents per page (optional, default to 50)
* @param offset Index of the first document in the page (optional, default to 0)
* @param sort Sort the results in the ascending/descending order of record creation. Default order is **descending** if `sort` is not passed (optional, default to desc)
* @return GetEmailCampaigns
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public GetEmailCampaigns getEmailCampaigns(String type, String status, String statistics, String startDate, String endDate, Long limit, Long offset, String sort) throws ApiException {
ApiResponse resp = getEmailCampaignsWithHttpInfo(type, status, statistics, startDate, endDate, limit, offset, sort);
return resp.getData();
}
/**
* Return all your created email campaigns
*
* @param type Filter on the type of the campaigns (optional)
* @param status Filter on the status of the campaign (optional)
* @param statistics Filter on the type of statistics required. Example **globalStats** value will only fetch globalStats info of the campaign in returned response. (optional)
* @param startDate Mandatory if endDate is used. Starting (urlencoded) UTC date-time (YYYY-MM-DDTHH:mm:ss.SSSZ) to filter the sent email campaigns. Prefer to pass your timezone in date-time format for accurate result ( only available if either 'status' not passed and if passed is set to 'sent' ) (optional)
* @param endDate Mandatory if startDate is used. Ending (urlencoded) UTC date-time (YYYY-MM-DDTHH:mm:ss.SSSZ) to filter the sent email campaigns. Prefer to pass your timezone in date-time format for accurate result ( only available if either 'status' not passed and if passed is set to 'sent' ) (optional)
* @param limit Number of documents per page (optional, default to 50)
* @param offset Index of the first document in the page (optional, default to 0)
* @param sort Sort the results in the ascending/descending order of record creation. Default order is **descending** if `sort` is not passed (optional, default to desc)
* @return ApiResponse<GetEmailCampaigns>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getEmailCampaignsWithHttpInfo(String type, String status, String statistics, String startDate, String endDate, Long limit, Long offset, String sort) throws ApiException {
Call call = getEmailCampaignsValidateBeforeCall(type, status, statistics, startDate, endDate, limit, offset, sort, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Return all your created email campaigns (asynchronously)
*
* @param type Filter on the type of the campaigns (optional)
* @param status Filter on the status of the campaign (optional)
* @param statistics Filter on the type of statistics required. Example **globalStats** value will only fetch globalStats info of the campaign in returned response. (optional)
* @param startDate Mandatory if endDate is used. Starting (urlencoded) UTC date-time (YYYY-MM-DDTHH:mm:ss.SSSZ) to filter the sent email campaigns. Prefer to pass your timezone in date-time format for accurate result ( only available if either 'status' not passed and if passed is set to 'sent' ) (optional)
* @param endDate Mandatory if startDate is used. Ending (urlencoded) UTC date-time (YYYY-MM-DDTHH:mm:ss.SSSZ) to filter the sent email campaigns. Prefer to pass your timezone in date-time format for accurate result ( only available if either 'status' not passed and if passed is set to 'sent' ) (optional)
* @param limit Number of documents per page (optional, default to 50)
* @param offset Index of the first document in the page (optional, default to 0)
* @param sort Sort the results in the ascending/descending order of record creation. Default order is **descending** if `sort` is not passed (optional, default to desc)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call getEmailCampaignsAsync(String type, String status, String statistics, String startDate, String endDate, Long limit, Long offset, String sort, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = getEmailCampaignsValidateBeforeCall(type, status, statistics, startDate, endDate, limit, offset, sort, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for getSharedTemplateUrl
* @param campaignId Id of the campaign or template (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call getSharedTemplateUrlCall(Long campaignId, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/emailCampaigns/{campaignId}/sharedUrl"
.replaceAll("\\{" + "campaignId" + "\\}", apiClient.escapeString(campaignId.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call getSharedTemplateUrlValidateBeforeCall(Long campaignId, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'campaignId' is set
if (campaignId == null) {
throw new ApiException("Missing the required parameter 'campaignId' when calling getSharedTemplateUrl(Async)");
}
Call call = getSharedTemplateUrlCall(campaignId, progressListener, progressRequestListener);
return call;
}
/**
* Get a shared template url
* Get a unique URL to share & import an email template from one Sendinblue account to another.
* @param campaignId Id of the campaign or template (required)
* @return GetSharedTemplateUrl
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public GetSharedTemplateUrl getSharedTemplateUrl(Long campaignId) throws ApiException {
ApiResponse resp = getSharedTemplateUrlWithHttpInfo(campaignId);
return resp.getData();
}
/**
* Get a shared template url
* Get a unique URL to share & import an email template from one Sendinblue account to another.
* @param campaignId Id of the campaign or template (required)
* @return ApiResponse<GetSharedTemplateUrl>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getSharedTemplateUrlWithHttpInfo(Long campaignId) throws ApiException {
Call call = getSharedTemplateUrlValidateBeforeCall(campaignId, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get a shared template url (asynchronously)
* Get a unique URL to share & import an email template from one Sendinblue account to another.
* @param campaignId Id of the campaign or template (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call getSharedTemplateUrlAsync(Long campaignId, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = getSharedTemplateUrlValidateBeforeCall(campaignId, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for sendEmailCampaignNow
* @param campaignId Id of the campaign (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call sendEmailCampaignNowCall(Long campaignId, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/emailCampaigns/{campaignId}/sendNow"
.replaceAll("\\{" + "campaignId" + "\\}", apiClient.escapeString(campaignId.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call sendEmailCampaignNowValidateBeforeCall(Long campaignId, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'campaignId' is set
if (campaignId == null) {
throw new ApiException("Missing the required parameter 'campaignId' when calling sendEmailCampaignNow(Async)");
}
Call call = sendEmailCampaignNowCall(campaignId, progressListener, progressRequestListener);
return call;
}
/**
* Send an email campaign immediately, based on campaignId
*
* @param campaignId Id of the campaign (required)
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public void sendEmailCampaignNow(Long campaignId) throws ApiException {
sendEmailCampaignNowWithHttpInfo(campaignId);
}
/**
* Send an email campaign immediately, based on campaignId
*
* @param campaignId Id of the campaign (required)
* @return ApiResponse<Void>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse sendEmailCampaignNowWithHttpInfo(Long campaignId) throws ApiException {
Call call = sendEmailCampaignNowValidateBeforeCall(campaignId, null, null);
return apiClient.execute(call);
}
/**
* Send an email campaign immediately, based on campaignId (asynchronously)
*
* @param campaignId Id of the campaign (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call sendEmailCampaignNowAsync(Long campaignId, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = sendEmailCampaignNowValidateBeforeCall(campaignId, progressListener, progressRequestListener);
apiClient.executeAsync(call, callback);
return call;
}
/**
* Build call for sendReport
* @param campaignId Id of the campaign (required)
* @param sendReport Values for send a report (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call sendReportCall(Long campaignId, SendReport sendReport, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = sendReport;
// create path and map variables
String localVarPath = "/emailCampaigns/{campaignId}/sendReport"
.replaceAll("\\{" + "campaignId" + "\\}", apiClient.escapeString(campaignId.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call sendReportValidateBeforeCall(Long campaignId, SendReport sendReport, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'campaignId' is set
if (campaignId == null) {
throw new ApiException("Missing the required parameter 'campaignId' when calling sendReport(Async)");
}
// verify the required parameter 'sendReport' is set
if (sendReport == null) {
throw new ApiException("Missing the required parameter 'sendReport' when calling sendReport(Async)");
}
Call call = sendReportCall(campaignId, sendReport, progressListener, progressRequestListener);
return call;
}
/**
* Send the report of a campaign
* A PDF will be sent to the specified email addresses
* @param campaignId Id of the campaign (required)
* @param sendReport Values for send a report (required)
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public void sendReport(Long campaignId, SendReport sendReport) throws ApiException {
sendReportWithHttpInfo(campaignId, sendReport);
}
/**
* Send the report of a campaign
* A PDF will be sent to the specified email addresses
* @param campaignId Id of the campaign (required)
* @param sendReport Values for send a report (required)
* @return ApiResponse<Void>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse sendReportWithHttpInfo(Long campaignId, SendReport sendReport) throws ApiException {
Call call = sendReportValidateBeforeCall(campaignId, sendReport, null, null);
return apiClient.execute(call);
}
/**
* Send the report of a campaign (asynchronously)
* A PDF will be sent to the specified email addresses
* @param campaignId Id of the campaign (required)
* @param sendReport Values for send a report (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call sendReportAsync(Long campaignId, SendReport sendReport, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = sendReportValidateBeforeCall(campaignId, sendReport, progressListener, progressRequestListener);
apiClient.executeAsync(call, callback);
return call;
}
/**
* Build call for sendTestEmail
* @param campaignId Id of the campaign (required)
* @param emailTo (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call sendTestEmailCall(Long campaignId, SendTestEmail emailTo, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = emailTo;
// create path and map variables
String localVarPath = "/emailCampaigns/{campaignId}/sendTest"
.replaceAll("\\{" + "campaignId" + "\\}", apiClient.escapeString(campaignId.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call sendTestEmailValidateBeforeCall(Long campaignId, SendTestEmail emailTo, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'campaignId' is set
if (campaignId == null) {
throw new ApiException("Missing the required parameter 'campaignId' when calling sendTestEmail(Async)");
}
// verify the required parameter 'emailTo' is set
if (emailTo == null) {
throw new ApiException("Missing the required parameter 'emailTo' when calling sendTestEmail(Async)");
}
Call call = sendTestEmailCall(campaignId, emailTo, progressListener, progressRequestListener);
return call;
}
/**
* Send an email campaign to your test list
*
* @param campaignId Id of the campaign (required)
* @param emailTo (required)
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public void sendTestEmail(Long campaignId, SendTestEmail emailTo) throws ApiException {
sendTestEmailWithHttpInfo(campaignId, emailTo);
}
/**
* Send an email campaign to your test list
*
* @param campaignId Id of the campaign (required)
* @param emailTo (required)
* @return ApiResponse<Void>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse sendTestEmailWithHttpInfo(Long campaignId, SendTestEmail emailTo) throws ApiException {
Call call = sendTestEmailValidateBeforeCall(campaignId, emailTo, null, null);
return apiClient.execute(call);
}
/**
* Send an email campaign to your test list (asynchronously)
*
* @param campaignId Id of the campaign (required)
* @param emailTo (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call sendTestEmailAsync(Long campaignId, SendTestEmail emailTo, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = sendTestEmailValidateBeforeCall(campaignId, emailTo, progressListener, progressRequestListener);
apiClient.executeAsync(call, callback);
return call;
}
/**
* Build call for updateCampaignStatus
* @param campaignId Id of the campaign (required)
* @param status Status of the campaign (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call updateCampaignStatusCall(Long campaignId, UpdateCampaignStatus status, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = status;
// create path and map variables
String localVarPath = "/emailCampaigns/{campaignId}/status"
.replaceAll("\\{" + "campaignId" + "\\}", apiClient.escapeString(campaignId.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "PUT", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call updateCampaignStatusValidateBeforeCall(Long campaignId, UpdateCampaignStatus status, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'campaignId' is set
if (campaignId == null) {
throw new ApiException("Missing the required parameter 'campaignId' when calling updateCampaignStatus(Async)");
}
// verify the required parameter 'status' is set
if (status == null) {
throw new ApiException("Missing the required parameter 'status' when calling updateCampaignStatus(Async)");
}
Call call = updateCampaignStatusCall(campaignId, status, progressListener, progressRequestListener);
return call;
}
/**
* Update an email campaign status
*
* @param campaignId Id of the campaign (required)
* @param status Status of the campaign (required)
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public void updateCampaignStatus(Long campaignId, UpdateCampaignStatus status) throws ApiException {
updateCampaignStatusWithHttpInfo(campaignId, status);
}
/**
* Update an email campaign status
*
* @param campaignId Id of the campaign (required)
* @param status Status of the campaign (required)
* @return ApiResponse<Void>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse updateCampaignStatusWithHttpInfo(Long campaignId, UpdateCampaignStatus status) throws ApiException {
Call call = updateCampaignStatusValidateBeforeCall(campaignId, status, null, null);
return apiClient.execute(call);
}
/**
* Update an email campaign status (asynchronously)
*
* @param campaignId Id of the campaign (required)
* @param status Status of the campaign (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call updateCampaignStatusAsync(Long campaignId, UpdateCampaignStatus status, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = updateCampaignStatusValidateBeforeCall(campaignId, status, progressListener, progressRequestListener);
apiClient.executeAsync(call, callback);
return call;
}
/**
* Build call for updateEmailCampaign
* @param campaignId Id of the campaign (required)
* @param emailCampaign Values to update a campaign (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call updateEmailCampaignCall(Long campaignId, UpdateEmailCampaign emailCampaign, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = emailCampaign;
// create path and map variables
String localVarPath = "/emailCampaigns/{campaignId}"
.replaceAll("\\{" + "campaignId" + "\\}", apiClient.escapeString(campaignId.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "PUT", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call updateEmailCampaignValidateBeforeCall(Long campaignId, UpdateEmailCampaign emailCampaign, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'campaignId' is set
if (campaignId == null) {
throw new ApiException("Missing the required parameter 'campaignId' when calling updateEmailCampaign(Async)");
}
// verify the required parameter 'emailCampaign' is set
if (emailCampaign == null) {
throw new ApiException("Missing the required parameter 'emailCampaign' when calling updateEmailCampaign(Async)");
}
Call call = updateEmailCampaignCall(campaignId, emailCampaign, progressListener, progressRequestListener);
return call;
}
/**
* Update an email campaign
*
* @param campaignId Id of the campaign (required)
* @param emailCampaign Values to update a campaign (required)
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public void updateEmailCampaign(Long campaignId, UpdateEmailCampaign emailCampaign) throws ApiException {
updateEmailCampaignWithHttpInfo(campaignId, emailCampaign);
}
/**
* Update an email campaign
*
* @param campaignId Id of the campaign (required)
* @param emailCampaign Values to update a campaign (required)
* @return ApiResponse<Void>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse updateEmailCampaignWithHttpInfo(Long campaignId, UpdateEmailCampaign emailCampaign) throws ApiException {
Call call = updateEmailCampaignValidateBeforeCall(campaignId, emailCampaign, null, null);
return apiClient.execute(call);
}
/**
* Update an email campaign (asynchronously)
*
* @param campaignId Id of the campaign (required)
* @param emailCampaign Values to update a campaign (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call updateEmailCampaignAsync(Long campaignId, UpdateEmailCampaign emailCampaign, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = updateEmailCampaignValidateBeforeCall(campaignId, emailCampaign, progressListener, progressRequestListener);
apiClient.executeAsync(call, callback);
return call;
}
/**
* Build call for uploadImageToGallery
* @param uploadImage Parameters to upload an image (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call uploadImageToGalleryCall(UploadImageToGallery uploadImage, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = uploadImage;
// create path and map variables
String localVarPath = "/emailCampaigns/images";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call uploadImageToGalleryValidateBeforeCall(UploadImageToGallery uploadImage, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'uploadImage' is set
if (uploadImage == null) {
throw new ApiException("Missing the required parameter 'uploadImage' when calling uploadImageToGallery(Async)");
}
Call call = uploadImageToGalleryCall(uploadImage, progressListener, progressRequestListener);
return call;
}
/**
* Upload an image to your account's image gallery
*
* @param uploadImage Parameters to upload an image (required)
* @return UploadImageModel
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public UploadImageModel uploadImageToGallery(UploadImageToGallery uploadImage) throws ApiException {
ApiResponse resp = uploadImageToGalleryWithHttpInfo(uploadImage);
return resp.getData();
}
/**
* Upload an image to your account's image gallery
*
* @param uploadImage Parameters to upload an image (required)
* @return ApiResponse<UploadImageModel>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse uploadImageToGalleryWithHttpInfo(UploadImageToGallery uploadImage) throws ApiException {
Call call = uploadImageToGalleryValidateBeforeCall(uploadImage, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Upload an image to your account's image gallery (asynchronously)
*
* @param uploadImage Parameters to upload an image (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call uploadImageToGalleryAsync(UploadImageToGallery uploadImage, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = uploadImageToGalleryValidateBeforeCall(uploadImage, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy