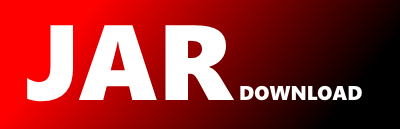
sibApi.MasterAccountApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sib-api-v3-sdk Show documentation
Show all versions of sib-api-v3-sdk Show documentation
SendinBlue's API v3 Java Library
The newest version!
/*
* SendinBlue API
* SendinBlue provide a RESTFul API that can be used with any languages. With this API, you will be able to : - Manage your campaigns and get the statistics - Manage your contacts - Send transactional Emails and SMS - and much more... You can download our wrappers at https://github.com/orgs/sendinblue **Possible responses** | Code | Message | | :-------------: | ------------- | | 200 | OK. Successful Request | | 201 | OK. Successful Creation | | 202 | OK. Request accepted | | 204 | OK. Successful Update/Deletion | | 400 | Error. Bad Request | | 401 | Error. Authentication Needed | | 402 | Error. Not enough credit, plan upgrade needed | | 403 | Error. Permission denied | | 404 | Error. Object does not exist | | 405 | Error. Method not allowed | | 406 | Error. Not Acceptable |
*
* OpenAPI spec version: 3.0.0
* Contact: [email protected]
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package sibApi;
import okhttp3.Call;
import sendinblue.ApiCallback;
import sendinblue.ApiClient;
import sendinblue.ApiException;
import sendinblue.ApiResponse;
import sendinblue.Configuration;
import sendinblue.Pair;
import sendinblue.ProgressRequestBody;
import sendinblue.ProgressResponseBody;
import com.google.gson.reflect.TypeToken;
import java.io.IOException;
import sibModel.CreateApiKeyRequest;
import sibModel.CreateApiKeyResponse;
import sibModel.CreateSubAccount;
import sibModel.CreateSubAccountResponse;
import sibModel.ErrorModel;
import sibModel.GetSsoToken;
import sibModel.MasterDetailsResponse;
import sibModel.SsoTokenRequest;
import sibModel.SubAccountDetailsResponse;
import sibModel.SubAccountUpdatePlanRequest;
import sibModel.SubAccountsResponse;
import java.lang.reflect.Type;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class MasterAccountApi {
private ApiClient apiClient;
public MasterAccountApi() {
this(Configuration.getDefaultApiClient());
}
public MasterAccountApi(ApiClient apiClient) {
this.apiClient = apiClient;
}
public ApiClient getApiClient() {
return apiClient;
}
public void setApiClient(ApiClient apiClient) {
this.apiClient = apiClient;
}
/**
* Build call for corporateMasterAccountGet
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call corporateMasterAccountGetCall(final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/corporate/masterAccount";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call corporateMasterAccountGetValidateBeforeCall(final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Call call = corporateMasterAccountGetCall(progressListener, progressRequestListener);
return call;
}
/**
* Get the details of requested master account
* This endpoint will provide the details of the master account.
* @return MasterDetailsResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public MasterDetailsResponse corporateMasterAccountGet() throws ApiException {
ApiResponse resp = corporateMasterAccountGetWithHttpInfo();
return resp.getData();
}
/**
* Get the details of requested master account
* This endpoint will provide the details of the master account.
* @return ApiResponse<MasterDetailsResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse corporateMasterAccountGetWithHttpInfo() throws ApiException {
Call call = corporateMasterAccountGetValidateBeforeCall(null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get the details of requested master account (asynchronously)
* This endpoint will provide the details of the master account.
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call corporateMasterAccountGetAsync(final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = corporateMasterAccountGetValidateBeforeCall(progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for corporateSubAccountGet
* @param offset Index of the first sub-account in the page (required)
* @param limit Number of sub-accounts to be displayed on each page (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call corporateSubAccountGetCall(Integer offset, Integer limit, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/corporate/subAccount";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
if (offset != null)
localVarQueryParams.addAll(apiClient.parameterToPair("offset", offset));
if (limit != null)
localVarQueryParams.addAll(apiClient.parameterToPair("limit", limit));
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call corporateSubAccountGetValidateBeforeCall(Integer offset, Integer limit, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'offset' is set
if (offset == null) {
throw new ApiException("Missing the required parameter 'offset' when calling corporateSubAccountGet(Async)");
}
// verify the required parameter 'limit' is set
if (limit == null) {
throw new ApiException("Missing the required parameter 'limit' when calling corporateSubAccountGet(Async)");
}
Call call = corporateSubAccountGetCall(offset, limit, progressListener, progressRequestListener);
return call;
}
/**
* Get the list of all the sub-accounts of the master account.
* This endpoint will provide the list all the sub-accounts of the master account.
* @param offset Index of the first sub-account in the page (required)
* @param limit Number of sub-accounts to be displayed on each page (required)
* @return SubAccountsResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public SubAccountsResponse corporateSubAccountGet(Integer offset, Integer limit) throws ApiException {
ApiResponse resp = corporateSubAccountGetWithHttpInfo(offset, limit);
return resp.getData();
}
/**
* Get the list of all the sub-accounts of the master account.
* This endpoint will provide the list all the sub-accounts of the master account.
* @param offset Index of the first sub-account in the page (required)
* @param limit Number of sub-accounts to be displayed on each page (required)
* @return ApiResponse<SubAccountsResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse corporateSubAccountGetWithHttpInfo(Integer offset, Integer limit) throws ApiException {
Call call = corporateSubAccountGetValidateBeforeCall(offset, limit, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get the list of all the sub-accounts of the master account. (asynchronously)
* This endpoint will provide the list all the sub-accounts of the master account.
* @param offset Index of the first sub-account in the page (required)
* @param limit Number of sub-accounts to be displayed on each page (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call corporateSubAccountGetAsync(Integer offset, Integer limit, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = corporateSubAccountGetValidateBeforeCall(offset, limit, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for corporateSubAccountIdDelete
* @param id Id of the sub-account organization to be deleted (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call corporateSubAccountIdDeleteCall(Long id, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/corporate/subAccount/{id}"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "DELETE", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call corporateSubAccountIdDeleteValidateBeforeCall(Long id, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException("Missing the required parameter 'id' when calling corporateSubAccountIdDelete(Async)");
}
Call call = corporateSubAccountIdDeleteCall(id, progressListener, progressRequestListener);
return call;
}
/**
* Delete a sub-account
*
* @param id Id of the sub-account organization to be deleted (required)
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public void corporateSubAccountIdDelete(Long id) throws ApiException {
corporateSubAccountIdDeleteWithHttpInfo(id);
}
/**
* Delete a sub-account
*
* @param id Id of the sub-account organization to be deleted (required)
* @return ApiResponse<Void>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse corporateSubAccountIdDeleteWithHttpInfo(Long id) throws ApiException {
Call call = corporateSubAccountIdDeleteValidateBeforeCall(id, null, null);
return apiClient.execute(call);
}
/**
* Delete a sub-account (asynchronously)
*
* @param id Id of the sub-account organization to be deleted (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call corporateSubAccountIdDeleteAsync(Long id, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = corporateSubAccountIdDeleteValidateBeforeCall(id, progressListener, progressRequestListener);
apiClient.executeAsync(call, callback);
return call;
}
/**
* Build call for corporateSubAccountIdGet
* @param id Id of the sub-account organization (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call corporateSubAccountIdGetCall(Long id, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/corporate/subAccount/{id}"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call corporateSubAccountIdGetValidateBeforeCall(Long id, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException("Missing the required parameter 'id' when calling corporateSubAccountIdGet(Async)");
}
Call call = corporateSubAccountIdGetCall(id, progressListener, progressRequestListener);
return call;
}
/**
* Get sub-account details
* This endpoint will provide the details for the specified sub-account company
* @param id Id of the sub-account organization (required)
* @return SubAccountDetailsResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public SubAccountDetailsResponse corporateSubAccountIdGet(Long id) throws ApiException {
ApiResponse resp = corporateSubAccountIdGetWithHttpInfo(id);
return resp.getData();
}
/**
* Get sub-account details
* This endpoint will provide the details for the specified sub-account company
* @param id Id of the sub-account organization (required)
* @return ApiResponse<SubAccountDetailsResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse corporateSubAccountIdGetWithHttpInfo(Long id) throws ApiException {
Call call = corporateSubAccountIdGetValidateBeforeCall(id, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get sub-account details (asynchronously)
* This endpoint will provide the details for the specified sub-account company
* @param id Id of the sub-account organization (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call corporateSubAccountIdGetAsync(Long id, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = corporateSubAccountIdGetValidateBeforeCall(id, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for corporateSubAccountIdPlanPut
* @param id Id of the sub-account organization (required)
* @param updatePlanDetails Values to update a sub-account plan (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call corporateSubAccountIdPlanPutCall(Long id, SubAccountUpdatePlanRequest updatePlanDetails, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = updatePlanDetails;
// create path and map variables
String localVarPath = "/corporate/subAccount/{id}/plan"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "PUT", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call corporateSubAccountIdPlanPutValidateBeforeCall(Long id, SubAccountUpdatePlanRequest updatePlanDetails, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException("Missing the required parameter 'id' when calling corporateSubAccountIdPlanPut(Async)");
}
// verify the required parameter 'updatePlanDetails' is set
if (updatePlanDetails == null) {
throw new ApiException("Missing the required parameter 'updatePlanDetails' when calling corporateSubAccountIdPlanPut(Async)");
}
Call call = corporateSubAccountIdPlanPutCall(id, updatePlanDetails, progressListener, progressRequestListener);
return call;
}
/**
* Update sub-account plan
* This endpoint will update the sub-account plan
* @param id Id of the sub-account organization (required)
* @param updatePlanDetails Values to update a sub-account plan (required)
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public void corporateSubAccountIdPlanPut(Long id, SubAccountUpdatePlanRequest updatePlanDetails) throws ApiException {
corporateSubAccountIdPlanPutWithHttpInfo(id, updatePlanDetails);
}
/**
* Update sub-account plan
* This endpoint will update the sub-account plan
* @param id Id of the sub-account organization (required)
* @param updatePlanDetails Values to update a sub-account plan (required)
* @return ApiResponse<Void>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse corporateSubAccountIdPlanPutWithHttpInfo(Long id, SubAccountUpdatePlanRequest updatePlanDetails) throws ApiException {
Call call = corporateSubAccountIdPlanPutValidateBeforeCall(id, updatePlanDetails, null, null);
return apiClient.execute(call);
}
/**
* Update sub-account plan (asynchronously)
* This endpoint will update the sub-account plan
* @param id Id of the sub-account organization (required)
* @param updatePlanDetails Values to update a sub-account plan (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call corporateSubAccountIdPlanPutAsync(Long id, SubAccountUpdatePlanRequest updatePlanDetails, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = corporateSubAccountIdPlanPutValidateBeforeCall(id, updatePlanDetails, progressListener, progressRequestListener);
apiClient.executeAsync(call, callback);
return call;
}
/**
* Build call for corporateSubAccountKeyPost
* @param createApiKeyRequest Values to generate API key for sub-account (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call corporateSubAccountKeyPostCall(CreateApiKeyRequest createApiKeyRequest, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = createApiKeyRequest;
// create path and map variables
String localVarPath = "/corporate/subAccount/key";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call corporateSubAccountKeyPostValidateBeforeCall(CreateApiKeyRequest createApiKeyRequest, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'createApiKeyRequest' is set
if (createApiKeyRequest == null) {
throw new ApiException("Missing the required parameter 'createApiKeyRequest' when calling corporateSubAccountKeyPost(Async)");
}
Call call = corporateSubAccountKeyPostCall(createApiKeyRequest, progressListener, progressRequestListener);
return call;
}
/**
* Create an API key for a sub-account
* This endpoint will generate an API v3 key for a sub account
* @param createApiKeyRequest Values to generate API key for sub-account (required)
* @return CreateApiKeyResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public CreateApiKeyResponse corporateSubAccountKeyPost(CreateApiKeyRequest createApiKeyRequest) throws ApiException {
ApiResponse resp = corporateSubAccountKeyPostWithHttpInfo(createApiKeyRequest);
return resp.getData();
}
/**
* Create an API key for a sub-account
* This endpoint will generate an API v3 key for a sub account
* @param createApiKeyRequest Values to generate API key for sub-account (required)
* @return ApiResponse<CreateApiKeyResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse corporateSubAccountKeyPostWithHttpInfo(CreateApiKeyRequest createApiKeyRequest) throws ApiException {
Call call = corporateSubAccountKeyPostValidateBeforeCall(createApiKeyRequest, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Create an API key for a sub-account (asynchronously)
* This endpoint will generate an API v3 key for a sub account
* @param createApiKeyRequest Values to generate API key for sub-account (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call corporateSubAccountKeyPostAsync(CreateApiKeyRequest createApiKeyRequest, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = corporateSubAccountKeyPostValidateBeforeCall(createApiKeyRequest, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for corporateSubAccountPost
* @param subAccountCreate values to create new sub-account (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call corporateSubAccountPostCall(CreateSubAccount subAccountCreate, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = subAccountCreate;
// create path and map variables
String localVarPath = "/corporate/subAccount";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call corporateSubAccountPostValidateBeforeCall(CreateSubAccount subAccountCreate, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'subAccountCreate' is set
if (subAccountCreate == null) {
throw new ApiException("Missing the required parameter 'subAccountCreate' when calling corporateSubAccountPost(Async)");
}
Call call = corporateSubAccountPostCall(subAccountCreate, progressListener, progressRequestListener);
return call;
}
/**
* Create a new sub-account under a master account.
* This endpoint will create a new sub-account under a master account
* @param subAccountCreate values to create new sub-account (required)
* @return CreateSubAccountResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public CreateSubAccountResponse corporateSubAccountPost(CreateSubAccount subAccountCreate) throws ApiException {
ApiResponse resp = corporateSubAccountPostWithHttpInfo(subAccountCreate);
return resp.getData();
}
/**
* Create a new sub-account under a master account.
* This endpoint will create a new sub-account under a master account
* @param subAccountCreate values to create new sub-account (required)
* @return ApiResponse<CreateSubAccountResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse corporateSubAccountPostWithHttpInfo(CreateSubAccount subAccountCreate) throws ApiException {
Call call = corporateSubAccountPostValidateBeforeCall(subAccountCreate, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Create a new sub-account under a master account. (asynchronously)
* This endpoint will create a new sub-account under a master account
* @param subAccountCreate values to create new sub-account (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call corporateSubAccountPostAsync(CreateSubAccount subAccountCreate, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = corporateSubAccountPostValidateBeforeCall(subAccountCreate, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for corporateSubAccountSsoTokenPost
* @param ssoTokenRequest Values to generate SSO token for sub-account (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call corporateSubAccountSsoTokenPostCall(SsoTokenRequest ssoTokenRequest, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = ssoTokenRequest;
// create path and map variables
String localVarPath = "/corporate/subAccount/ssoToken";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call corporateSubAccountSsoTokenPostValidateBeforeCall(SsoTokenRequest ssoTokenRequest, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'ssoTokenRequest' is set
if (ssoTokenRequest == null) {
throw new ApiException("Missing the required parameter 'ssoTokenRequest' when calling corporateSubAccountSsoTokenPost(Async)");
}
Call call = corporateSubAccountSsoTokenPostCall(ssoTokenRequest, progressListener, progressRequestListener);
return call;
}
/**
* Generate SSO token to access Sendinblue
* This endpoint generates an sso token to authenticate and access a sub-account of the master using the account endpoint https://account-app.sendinblue.com/account/login/sub-account/sso/[token], where [token] will be replaced by the actual token.
* @param ssoTokenRequest Values to generate SSO token for sub-account (required)
* @return GetSsoToken
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public GetSsoToken corporateSubAccountSsoTokenPost(SsoTokenRequest ssoTokenRequest) throws ApiException {
ApiResponse resp = corporateSubAccountSsoTokenPostWithHttpInfo(ssoTokenRequest);
return resp.getData();
}
/**
* Generate SSO token to access Sendinblue
* This endpoint generates an sso token to authenticate and access a sub-account of the master using the account endpoint https://account-app.sendinblue.com/account/login/sub-account/sso/[token], where [token] will be replaced by the actual token.
* @param ssoTokenRequest Values to generate SSO token for sub-account (required)
* @return ApiResponse<GetSsoToken>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse corporateSubAccountSsoTokenPostWithHttpInfo(SsoTokenRequest ssoTokenRequest) throws ApiException {
Call call = corporateSubAccountSsoTokenPostValidateBeforeCall(ssoTokenRequest, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Generate SSO token to access Sendinblue (asynchronously)
* This endpoint generates an sso token to authenticate and access a sub-account of the master using the account endpoint https://account-app.sendinblue.com/account/login/sub-account/sso/[token], where [token] will be replaced by the actual token.
* @param ssoTokenRequest Values to generate SSO token for sub-account (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call corporateSubAccountSsoTokenPostAsync(SsoTokenRequest ssoTokenRequest, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = corporateSubAccountSsoTokenPostValidateBeforeCall(ssoTokenRequest, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy