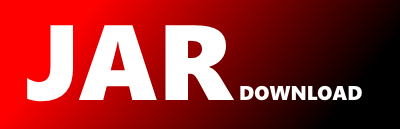
sibApi.ResellerApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sib-api-v3-sdk Show documentation
Show all versions of sib-api-v3-sdk Show documentation
SendinBlue's API v3 Java Library
The newest version!
/*
* SendinBlue API
* SendinBlue provide a RESTFul API that can be used with any languages. With this API, you will be able to : - Manage your campaigns and get the statistics - Manage your contacts - Send transactional Emails and SMS - and much more... You can download our wrappers at https://github.com/orgs/sendinblue **Possible responses** | Code | Message | | :-------------: | ------------- | | 200 | OK. Successful Request | | 201 | OK. Successful Creation | | 202 | OK. Request accepted | | 204 | OK. Successful Update/Deletion | | 400 | Error. Bad Request | | 401 | Error. Authentication Needed | | 402 | Error. Not enough credit, plan upgrade needed | | 403 | Error. Permission denied | | 404 | Error. Object does not exist | | 405 | Error. Method not allowed | | 406 | Error. Not Acceptable |
*
* OpenAPI spec version: 3.0.0
* Contact: [email protected]
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package sibApi;
import okhttp3.Call;
import sendinblue.ApiCallback;
import sendinblue.ApiClient;
import sendinblue.ApiException;
import sendinblue.ApiResponse;
import sendinblue.Configuration;
import sendinblue.Pair;
import sendinblue.ProgressRequestBody;
import sendinblue.ProgressResponseBody;
import com.google.gson.reflect.TypeToken;
import java.io.IOException;
import sibModel.AddChildDomain;
import sibModel.AddCredits;
import sibModel.CreateChild;
import sibModel.CreateReseller;
import sibModel.ErrorModel;
import sibModel.GetChildAccountCreationStatus;
import sibModel.GetChildDomains;
import sibModel.GetChildInfo;
import sibModel.GetChildrenList;
import sibModel.GetSsoToken;
import sibModel.ManageIp;
import sibModel.RemainingCreditModel;
import sibModel.RemoveCredits;
import sibModel.UpdateChild;
import sibModel.UpdateChildAccountStatus;
import sibModel.UpdateChildDomain;
import java.lang.reflect.Type;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class ResellerApi {
private ApiClient apiClient;
public ResellerApi() {
this(Configuration.getDefaultApiClient());
}
public ResellerApi(ApiClient apiClient) {
this.apiClient = apiClient;
}
public ApiClient getApiClient() {
return apiClient;
}
public void setApiClient(ApiClient apiClient) {
this.apiClient = apiClient;
}
/**
* Build call for addCredits
* @param childIdentifier Either auth key or id of reseller's child (required)
* @param addCredits Values to post to add credit to a specific child account (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call addCreditsCall(String childIdentifier, AddCredits addCredits, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = addCredits;
// create path and map variables
String localVarPath = "/reseller/children/{childIdentifier}/credits/add"
.replaceAll("\\{" + "childIdentifier" + "\\}", apiClient.escapeString(childIdentifier.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call addCreditsValidateBeforeCall(String childIdentifier, AddCredits addCredits, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'childIdentifier' is set
if (childIdentifier == null) {
throw new ApiException("Missing the required parameter 'childIdentifier' when calling addCredits(Async)");
}
// verify the required parameter 'addCredits' is set
if (addCredits == null) {
throw new ApiException("Missing the required parameter 'addCredits' when calling addCredits(Async)");
}
Call call = addCreditsCall(childIdentifier, addCredits, progressListener, progressRequestListener);
return call;
}
/**
* Add Email and/or SMS credits to a specific child account
*
* @param childIdentifier Either auth key or id of reseller's child (required)
* @param addCredits Values to post to add credit to a specific child account (required)
* @return RemainingCreditModel
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public RemainingCreditModel addCredits(String childIdentifier, AddCredits addCredits) throws ApiException {
ApiResponse resp = addCreditsWithHttpInfo(childIdentifier, addCredits);
return resp.getData();
}
/**
* Add Email and/or SMS credits to a specific child account
*
* @param childIdentifier Either auth key or id of reseller's child (required)
* @param addCredits Values to post to add credit to a specific child account (required)
* @return ApiResponse<RemainingCreditModel>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse addCreditsWithHttpInfo(String childIdentifier, AddCredits addCredits) throws ApiException {
Call call = addCreditsValidateBeforeCall(childIdentifier, addCredits, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Add Email and/or SMS credits to a specific child account (asynchronously)
*
* @param childIdentifier Either auth key or id of reseller's child (required)
* @param addCredits Values to post to add credit to a specific child account (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call addCreditsAsync(String childIdentifier, AddCredits addCredits, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = addCreditsValidateBeforeCall(childIdentifier, addCredits, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for associateIpToChild
* @param childIdentifier Either auth key or id of reseller's child (required)
* @param ip IP to associate (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call associateIpToChildCall(String childIdentifier, ManageIp ip, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = ip;
// create path and map variables
String localVarPath = "/reseller/children/{childIdentifier}/ips/associate"
.replaceAll("\\{" + "childIdentifier" + "\\}", apiClient.escapeString(childIdentifier.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call associateIpToChildValidateBeforeCall(String childIdentifier, ManageIp ip, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'childIdentifier' is set
if (childIdentifier == null) {
throw new ApiException("Missing the required parameter 'childIdentifier' when calling associateIpToChild(Async)");
}
// verify the required parameter 'ip' is set
if (ip == null) {
throw new ApiException("Missing the required parameter 'ip' when calling associateIpToChild(Async)");
}
Call call = associateIpToChildCall(childIdentifier, ip, progressListener, progressRequestListener);
return call;
}
/**
* Associate a dedicated IP to the child
*
* @param childIdentifier Either auth key or id of reseller's child (required)
* @param ip IP to associate (required)
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public void associateIpToChild(String childIdentifier, ManageIp ip) throws ApiException {
associateIpToChildWithHttpInfo(childIdentifier, ip);
}
/**
* Associate a dedicated IP to the child
*
* @param childIdentifier Either auth key or id of reseller's child (required)
* @param ip IP to associate (required)
* @return ApiResponse<Void>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse associateIpToChildWithHttpInfo(String childIdentifier, ManageIp ip) throws ApiException {
Call call = associateIpToChildValidateBeforeCall(childIdentifier, ip, null, null);
return apiClient.execute(call);
}
/**
* Associate a dedicated IP to the child (asynchronously)
*
* @param childIdentifier Either auth key or id of reseller's child (required)
* @param ip IP to associate (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call associateIpToChildAsync(String childIdentifier, ManageIp ip, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = associateIpToChildValidateBeforeCall(childIdentifier, ip, progressListener, progressRequestListener);
apiClient.executeAsync(call, callback);
return call;
}
/**
* Build call for createChildDomain
* @param childIdentifier Either auth key or id of reseller's child (required)
* @param addChildDomain Sender domain to add for a specific child account. This will not be displayed to the parent account. (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call createChildDomainCall(String childIdentifier, AddChildDomain addChildDomain, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = addChildDomain;
// create path and map variables
String localVarPath = "/reseller/children/{childIdentifier}/domains"
.replaceAll("\\{" + "childIdentifier" + "\\}", apiClient.escapeString(childIdentifier.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call createChildDomainValidateBeforeCall(String childIdentifier, AddChildDomain addChildDomain, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'childIdentifier' is set
if (childIdentifier == null) {
throw new ApiException("Missing the required parameter 'childIdentifier' when calling createChildDomain(Async)");
}
// verify the required parameter 'addChildDomain' is set
if (addChildDomain == null) {
throw new ApiException("Missing the required parameter 'addChildDomain' when calling createChildDomain(Async)");
}
Call call = createChildDomainCall(childIdentifier, addChildDomain, progressListener, progressRequestListener);
return call;
}
/**
* Create a domain for a child account
*
* @param childIdentifier Either auth key or id of reseller's child (required)
* @param addChildDomain Sender domain to add for a specific child account. This will not be displayed to the parent account. (required)
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public void createChildDomain(String childIdentifier, AddChildDomain addChildDomain) throws ApiException {
createChildDomainWithHttpInfo(childIdentifier, addChildDomain);
}
/**
* Create a domain for a child account
*
* @param childIdentifier Either auth key or id of reseller's child (required)
* @param addChildDomain Sender domain to add for a specific child account. This will not be displayed to the parent account. (required)
* @return ApiResponse<Void>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse createChildDomainWithHttpInfo(String childIdentifier, AddChildDomain addChildDomain) throws ApiException {
Call call = createChildDomainValidateBeforeCall(childIdentifier, addChildDomain, null, null);
return apiClient.execute(call);
}
/**
* Create a domain for a child account (asynchronously)
*
* @param childIdentifier Either auth key or id of reseller's child (required)
* @param addChildDomain Sender domain to add for a specific child account. This will not be displayed to the parent account. (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call createChildDomainAsync(String childIdentifier, AddChildDomain addChildDomain, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = createChildDomainValidateBeforeCall(childIdentifier, addChildDomain, progressListener, progressRequestListener);
apiClient.executeAsync(call, callback);
return call;
}
/**
* Build call for createResellerChild
* @param resellerChild reseller child to add (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call createResellerChildCall(CreateChild resellerChild, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = resellerChild;
// create path and map variables
String localVarPath = "/reseller/children";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call createResellerChildValidateBeforeCall(CreateChild resellerChild, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Call call = createResellerChildCall(resellerChild, progressListener, progressRequestListener);
return call;
}
/**
* Creates a reseller child
*
* @param resellerChild reseller child to add (optional)
* @return CreateReseller
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public CreateReseller createResellerChild(CreateChild resellerChild) throws ApiException {
ApiResponse resp = createResellerChildWithHttpInfo(resellerChild);
return resp.getData();
}
/**
* Creates a reseller child
*
* @param resellerChild reseller child to add (optional)
* @return ApiResponse<CreateReseller>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse createResellerChildWithHttpInfo(CreateChild resellerChild) throws ApiException {
Call call = createResellerChildValidateBeforeCall(resellerChild, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Creates a reseller child (asynchronously)
*
* @param resellerChild reseller child to add (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call createResellerChildAsync(CreateChild resellerChild, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = createResellerChildValidateBeforeCall(resellerChild, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for deleteChildDomain
* @param childIdentifier Either auth key or id of reseller's child (required)
* @param domainName Pass the existing domain that needs to be deleted (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call deleteChildDomainCall(String childIdentifier, String domainName, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/reseller/children/{childIdentifier}/domains/{domainName}"
.replaceAll("\\{" + "childIdentifier" + "\\}", apiClient.escapeString(childIdentifier.toString()))
.replaceAll("\\{" + "domainName" + "\\}", apiClient.escapeString(domainName.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "DELETE", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call deleteChildDomainValidateBeforeCall(String childIdentifier, String domainName, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'childIdentifier' is set
if (childIdentifier == null) {
throw new ApiException("Missing the required parameter 'childIdentifier' when calling deleteChildDomain(Async)");
}
// verify the required parameter 'domainName' is set
if (domainName == null) {
throw new ApiException("Missing the required parameter 'domainName' when calling deleteChildDomain(Async)");
}
Call call = deleteChildDomainCall(childIdentifier, domainName, progressListener, progressRequestListener);
return call;
}
/**
* Delete the sender domain of the reseller child based on the childIdentifier and domainName passed
*
* @param childIdentifier Either auth key or id of reseller's child (required)
* @param domainName Pass the existing domain that needs to be deleted (required)
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public void deleteChildDomain(String childIdentifier, String domainName) throws ApiException {
deleteChildDomainWithHttpInfo(childIdentifier, domainName);
}
/**
* Delete the sender domain of the reseller child based on the childIdentifier and domainName passed
*
* @param childIdentifier Either auth key or id of reseller's child (required)
* @param domainName Pass the existing domain that needs to be deleted (required)
* @return ApiResponse<Void>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse deleteChildDomainWithHttpInfo(String childIdentifier, String domainName) throws ApiException {
Call call = deleteChildDomainValidateBeforeCall(childIdentifier, domainName, null, null);
return apiClient.execute(call);
}
/**
* Delete the sender domain of the reseller child based on the childIdentifier and domainName passed (asynchronously)
*
* @param childIdentifier Either auth key or id of reseller's child (required)
* @param domainName Pass the existing domain that needs to be deleted (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call deleteChildDomainAsync(String childIdentifier, String domainName, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = deleteChildDomainValidateBeforeCall(childIdentifier, domainName, progressListener, progressRequestListener);
apiClient.executeAsync(call, callback);
return call;
}
/**
* Build call for deleteResellerChild
* @param childIdentifier Either auth key or child id of reseller's child (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call deleteResellerChildCall(String childIdentifier, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/reseller/children/{childIdentifier}"
.replaceAll("\\{" + "childIdentifier" + "\\}", apiClient.escapeString(childIdentifier.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "DELETE", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call deleteResellerChildValidateBeforeCall(String childIdentifier, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'childIdentifier' is set
if (childIdentifier == null) {
throw new ApiException("Missing the required parameter 'childIdentifier' when calling deleteResellerChild(Async)");
}
Call call = deleteResellerChildCall(childIdentifier, progressListener, progressRequestListener);
return call;
}
/**
* Delete a single reseller child based on the child identifier supplied
*
* @param childIdentifier Either auth key or child id of reseller's child (required)
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public void deleteResellerChild(String childIdentifier) throws ApiException {
deleteResellerChildWithHttpInfo(childIdentifier);
}
/**
* Delete a single reseller child based on the child identifier supplied
*
* @param childIdentifier Either auth key or child id of reseller's child (required)
* @return ApiResponse<Void>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse deleteResellerChildWithHttpInfo(String childIdentifier) throws ApiException {
Call call = deleteResellerChildValidateBeforeCall(childIdentifier, null, null);
return apiClient.execute(call);
}
/**
* Delete a single reseller child based on the child identifier supplied (asynchronously)
*
* @param childIdentifier Either auth key or child id of reseller's child (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call deleteResellerChildAsync(String childIdentifier, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = deleteResellerChildValidateBeforeCall(childIdentifier, progressListener, progressRequestListener);
apiClient.executeAsync(call, callback);
return call;
}
/**
* Build call for dissociateIpFromChild
* @param childIdentifier Either auth key or id of reseller's child (required)
* @param ip IP to dissociate (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call dissociateIpFromChildCall(String childIdentifier, ManageIp ip, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = ip;
// create path and map variables
String localVarPath = "/reseller/children/{childIdentifier}/ips/dissociate"
.replaceAll("\\{" + "childIdentifier" + "\\}", apiClient.escapeString(childIdentifier.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call dissociateIpFromChildValidateBeforeCall(String childIdentifier, ManageIp ip, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'childIdentifier' is set
if (childIdentifier == null) {
throw new ApiException("Missing the required parameter 'childIdentifier' when calling dissociateIpFromChild(Async)");
}
// verify the required parameter 'ip' is set
if (ip == null) {
throw new ApiException("Missing the required parameter 'ip' when calling dissociateIpFromChild(Async)");
}
Call call = dissociateIpFromChildCall(childIdentifier, ip, progressListener, progressRequestListener);
return call;
}
/**
* Dissociate a dedicated IP to the child
*
* @param childIdentifier Either auth key or id of reseller's child (required)
* @param ip IP to dissociate (required)
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public void dissociateIpFromChild(String childIdentifier, ManageIp ip) throws ApiException {
dissociateIpFromChildWithHttpInfo(childIdentifier, ip);
}
/**
* Dissociate a dedicated IP to the child
*
* @param childIdentifier Either auth key or id of reseller's child (required)
* @param ip IP to dissociate (required)
* @return ApiResponse<Void>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse dissociateIpFromChildWithHttpInfo(String childIdentifier, ManageIp ip) throws ApiException {
Call call = dissociateIpFromChildValidateBeforeCall(childIdentifier, ip, null, null);
return apiClient.execute(call);
}
/**
* Dissociate a dedicated IP to the child (asynchronously)
*
* @param childIdentifier Either auth key or id of reseller's child (required)
* @param ip IP to dissociate (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call dissociateIpFromChildAsync(String childIdentifier, ManageIp ip, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = dissociateIpFromChildValidateBeforeCall(childIdentifier, ip, progressListener, progressRequestListener);
apiClient.executeAsync(call, callback);
return call;
}
/**
* Build call for getChildAccountCreationStatus
* @param childIdentifier Either auth key or id of reseller's child (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call getChildAccountCreationStatusCall(String childIdentifier, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/reseller/children/{childIdentifier}/accountCreationStatus"
.replaceAll("\\{" + "childIdentifier" + "\\}", apiClient.escapeString(childIdentifier.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call getChildAccountCreationStatusValidateBeforeCall(String childIdentifier, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'childIdentifier' is set
if (childIdentifier == null) {
throw new ApiException("Missing the required parameter 'childIdentifier' when calling getChildAccountCreationStatus(Async)");
}
Call call = getChildAccountCreationStatusCall(childIdentifier, progressListener, progressRequestListener);
return call;
}
/**
* Get the status of a reseller's child account creation, whether it is successfully created (exists) or not based on the identifier supplied
*
* @param childIdentifier Either auth key or id of reseller's child (required)
* @return GetChildAccountCreationStatus
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public GetChildAccountCreationStatus getChildAccountCreationStatus(String childIdentifier) throws ApiException {
ApiResponse resp = getChildAccountCreationStatusWithHttpInfo(childIdentifier);
return resp.getData();
}
/**
* Get the status of a reseller's child account creation, whether it is successfully created (exists) or not based on the identifier supplied
*
* @param childIdentifier Either auth key or id of reseller's child (required)
* @return ApiResponse<GetChildAccountCreationStatus>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getChildAccountCreationStatusWithHttpInfo(String childIdentifier) throws ApiException {
Call call = getChildAccountCreationStatusValidateBeforeCall(childIdentifier, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get the status of a reseller's child account creation, whether it is successfully created (exists) or not based on the identifier supplied (asynchronously)
*
* @param childIdentifier Either auth key or id of reseller's child (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call getChildAccountCreationStatusAsync(String childIdentifier, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = getChildAccountCreationStatusValidateBeforeCall(childIdentifier, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for getChildDomains
* @param childIdentifier Either auth key or id of reseller's child (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call getChildDomainsCall(String childIdentifier, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/reseller/children/{childIdentifier}/domains"
.replaceAll("\\{" + "childIdentifier" + "\\}", apiClient.escapeString(childIdentifier.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call getChildDomainsValidateBeforeCall(String childIdentifier, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'childIdentifier' is set
if (childIdentifier == null) {
throw new ApiException("Missing the required parameter 'childIdentifier' when calling getChildDomains(Async)");
}
Call call = getChildDomainsCall(childIdentifier, progressListener, progressRequestListener);
return call;
}
/**
* Get all sender domains for a specific child account
*
* @param childIdentifier Either auth key or id of reseller's child (required)
* @return GetChildDomains
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public GetChildDomains getChildDomains(String childIdentifier) throws ApiException {
ApiResponse resp = getChildDomainsWithHttpInfo(childIdentifier);
return resp.getData();
}
/**
* Get all sender domains for a specific child account
*
* @param childIdentifier Either auth key or id of reseller's child (required)
* @return ApiResponse<GetChildDomains>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getChildDomainsWithHttpInfo(String childIdentifier) throws ApiException {
Call call = getChildDomainsValidateBeforeCall(childIdentifier, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get all sender domains for a specific child account (asynchronously)
*
* @param childIdentifier Either auth key or id of reseller's child (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call getChildDomainsAsync(String childIdentifier, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = getChildDomainsValidateBeforeCall(childIdentifier, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for getChildInfo
* @param childIdentifier Either auth key or id of reseller's child (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call getChildInfoCall(String childIdentifier, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/reseller/children/{childIdentifier}"
.replaceAll("\\{" + "childIdentifier" + "\\}", apiClient.escapeString(childIdentifier.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call getChildInfoValidateBeforeCall(String childIdentifier, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'childIdentifier' is set
if (childIdentifier == null) {
throw new ApiException("Missing the required parameter 'childIdentifier' when calling getChildInfo(Async)");
}
Call call = getChildInfoCall(childIdentifier, progressListener, progressRequestListener);
return call;
}
/**
* Get a child account's details
*
* @param childIdentifier Either auth key or id of reseller's child (required)
* @return GetChildInfo
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public GetChildInfo getChildInfo(String childIdentifier) throws ApiException {
ApiResponse resp = getChildInfoWithHttpInfo(childIdentifier);
return resp.getData();
}
/**
* Get a child account's details
*
* @param childIdentifier Either auth key or id of reseller's child (required)
* @return ApiResponse<GetChildInfo>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getChildInfoWithHttpInfo(String childIdentifier) throws ApiException {
Call call = getChildInfoValidateBeforeCall(childIdentifier, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get a child account's details (asynchronously)
*
* @param childIdentifier Either auth key or id of reseller's child (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call getChildInfoAsync(String childIdentifier, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = getChildInfoValidateBeforeCall(childIdentifier, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for getResellerChilds
* @param limit Number of documents for child accounts information per page (optional, default to 10)
* @param offset Index of the first document in the page (optional, default to 0)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call getResellerChildsCall(Long limit, Long offset, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/reseller/children";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
if (limit != null)
localVarQueryParams.addAll(apiClient.parameterToPair("limit", limit));
if (offset != null)
localVarQueryParams.addAll(apiClient.parameterToPair("offset", offset));
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call getResellerChildsValidateBeforeCall(Long limit, Long offset, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Call call = getResellerChildsCall(limit, offset, progressListener, progressRequestListener);
return call;
}
/**
* Get the list of all children accounts
*
* @param limit Number of documents for child accounts information per page (optional, default to 10)
* @param offset Index of the first document in the page (optional, default to 0)
* @return GetChildrenList
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public GetChildrenList getResellerChilds(Long limit, Long offset) throws ApiException {
ApiResponse resp = getResellerChildsWithHttpInfo(limit, offset);
return resp.getData();
}
/**
* Get the list of all children accounts
*
* @param limit Number of documents for child accounts information per page (optional, default to 10)
* @param offset Index of the first document in the page (optional, default to 0)
* @return ApiResponse<GetChildrenList>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getResellerChildsWithHttpInfo(Long limit, Long offset) throws ApiException {
Call call = getResellerChildsValidateBeforeCall(limit, offset, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get the list of all children accounts (asynchronously)
*
* @param limit Number of documents for child accounts information per page (optional, default to 10)
* @param offset Index of the first document in the page (optional, default to 0)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call getResellerChildsAsync(Long limit, Long offset, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = getResellerChildsValidateBeforeCall(limit, offset, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for getSsoToken
* @param childIdentifier Either auth key or id of reseller's child (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call getSsoTokenCall(String childIdentifier, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/reseller/children/{childIdentifier}/auth"
.replaceAll("\\{" + "childIdentifier" + "\\}", apiClient.escapeString(childIdentifier.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call getSsoTokenValidateBeforeCall(String childIdentifier, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'childIdentifier' is set
if (childIdentifier == null) {
throw new ApiException("Missing the required parameter 'childIdentifier' when calling getSsoToken(Async)");
}
Call call = getSsoTokenCall(childIdentifier, progressListener, progressRequestListener);
return call;
}
/**
* Get session token to access Sendinblue (SSO)
* It returns a session [token] which will remain valid for a short period of time. A child account will be able to access a white-labeled section by using the following url pattern => https:/email.mydomain.com/login/sso?token=[token]
* @param childIdentifier Either auth key or id of reseller's child (required)
* @return GetSsoToken
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public GetSsoToken getSsoToken(String childIdentifier) throws ApiException {
ApiResponse resp = getSsoTokenWithHttpInfo(childIdentifier);
return resp.getData();
}
/**
* Get session token to access Sendinblue (SSO)
* It returns a session [token] which will remain valid for a short period of time. A child account will be able to access a white-labeled section by using the following url pattern => https:/email.mydomain.com/login/sso?token=[token]
* @param childIdentifier Either auth key or id of reseller's child (required)
* @return ApiResponse<GetSsoToken>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getSsoTokenWithHttpInfo(String childIdentifier) throws ApiException {
Call call = getSsoTokenValidateBeforeCall(childIdentifier, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get session token to access Sendinblue (SSO) (asynchronously)
* It returns a session [token] which will remain valid for a short period of time. A child account will be able to access a white-labeled section by using the following url pattern => https:/email.mydomain.com/login/sso?token=[token]
* @param childIdentifier Either auth key or id of reseller's child (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call getSsoTokenAsync(String childIdentifier, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = getSsoTokenValidateBeforeCall(childIdentifier, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for removeCredits
* @param childIdentifier Either auth key or id of reseller's child (required)
* @param removeCredits Values to post to remove email or SMS credits from a specific child account (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call removeCreditsCall(String childIdentifier, RemoveCredits removeCredits, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = removeCredits;
// create path and map variables
String localVarPath = "/reseller/children/{childIdentifier}/credits/remove"
.replaceAll("\\{" + "childIdentifier" + "\\}", apiClient.escapeString(childIdentifier.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call removeCreditsValidateBeforeCall(String childIdentifier, RemoveCredits removeCredits, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'childIdentifier' is set
if (childIdentifier == null) {
throw new ApiException("Missing the required parameter 'childIdentifier' when calling removeCredits(Async)");
}
// verify the required parameter 'removeCredits' is set
if (removeCredits == null) {
throw new ApiException("Missing the required parameter 'removeCredits' when calling removeCredits(Async)");
}
Call call = removeCreditsCall(childIdentifier, removeCredits, progressListener, progressRequestListener);
return call;
}
/**
* Remove Email and/or SMS credits from a specific child account
*
* @param childIdentifier Either auth key or id of reseller's child (required)
* @param removeCredits Values to post to remove email or SMS credits from a specific child account (required)
* @return RemainingCreditModel
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public RemainingCreditModel removeCredits(String childIdentifier, RemoveCredits removeCredits) throws ApiException {
ApiResponse resp = removeCreditsWithHttpInfo(childIdentifier, removeCredits);
return resp.getData();
}
/**
* Remove Email and/or SMS credits from a specific child account
*
* @param childIdentifier Either auth key or id of reseller's child (required)
* @param removeCredits Values to post to remove email or SMS credits from a specific child account (required)
* @return ApiResponse<RemainingCreditModel>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse removeCreditsWithHttpInfo(String childIdentifier, RemoveCredits removeCredits) throws ApiException {
Call call = removeCreditsValidateBeforeCall(childIdentifier, removeCredits, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Remove Email and/or SMS credits from a specific child account (asynchronously)
*
* @param childIdentifier Either auth key or id of reseller's child (required)
* @param removeCredits Values to post to remove email or SMS credits from a specific child account (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call removeCreditsAsync(String childIdentifier, RemoveCredits removeCredits, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = removeCreditsValidateBeforeCall(childIdentifier, removeCredits, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for updateChildAccountStatus
* @param childIdentifier Either auth key or id of reseller's child (required)
* @param updateChildAccountStatus values to update in child account status (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call updateChildAccountStatusCall(String childIdentifier, UpdateChildAccountStatus updateChildAccountStatus, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = updateChildAccountStatus;
// create path and map variables
String localVarPath = "/reseller/children/{childIdentifier}/accountStatus"
.replaceAll("\\{" + "childIdentifier" + "\\}", apiClient.escapeString(childIdentifier.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "PUT", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call updateChildAccountStatusValidateBeforeCall(String childIdentifier, UpdateChildAccountStatus updateChildAccountStatus, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'childIdentifier' is set
if (childIdentifier == null) {
throw new ApiException("Missing the required parameter 'childIdentifier' when calling updateChildAccountStatus(Async)");
}
// verify the required parameter 'updateChildAccountStatus' is set
if (updateChildAccountStatus == null) {
throw new ApiException("Missing the required parameter 'updateChildAccountStatus' when calling updateChildAccountStatus(Async)");
}
Call call = updateChildAccountStatusCall(childIdentifier, updateChildAccountStatus, progressListener, progressRequestListener);
return call;
}
/**
* Update info of reseller's child account status based on the childIdentifier supplied
*
* @param childIdentifier Either auth key or id of reseller's child (required)
* @param updateChildAccountStatus values to update in child account status (required)
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public void updateChildAccountStatus(String childIdentifier, UpdateChildAccountStatus updateChildAccountStatus) throws ApiException {
updateChildAccountStatusWithHttpInfo(childIdentifier, updateChildAccountStatus);
}
/**
* Update info of reseller's child account status based on the childIdentifier supplied
*
* @param childIdentifier Either auth key or id of reseller's child (required)
* @param updateChildAccountStatus values to update in child account status (required)
* @return ApiResponse<Void>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse updateChildAccountStatusWithHttpInfo(String childIdentifier, UpdateChildAccountStatus updateChildAccountStatus) throws ApiException {
Call call = updateChildAccountStatusValidateBeforeCall(childIdentifier, updateChildAccountStatus, null, null);
return apiClient.execute(call);
}
/**
* Update info of reseller's child account status based on the childIdentifier supplied (asynchronously)
*
* @param childIdentifier Either auth key or id of reseller's child (required)
* @param updateChildAccountStatus values to update in child account status (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call updateChildAccountStatusAsync(String childIdentifier, UpdateChildAccountStatus updateChildAccountStatus, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = updateChildAccountStatusValidateBeforeCall(childIdentifier, updateChildAccountStatus, progressListener, progressRequestListener);
apiClient.executeAsync(call, callback);
return call;
}
/**
* Build call for updateChildDomain
* @param childIdentifier Either auth key or id of reseller's child (required)
* @param domainName Pass the existing domain that needs to be updated (required)
* @param updateChildDomain value to update for sender domain (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call updateChildDomainCall(String childIdentifier, String domainName, UpdateChildDomain updateChildDomain, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = updateChildDomain;
// create path and map variables
String localVarPath = "/reseller/children/{childIdentifier}/domains/{domainName}"
.replaceAll("\\{" + "childIdentifier" + "\\}", apiClient.escapeString(childIdentifier.toString()))
.replaceAll("\\{" + "domainName" + "\\}", apiClient.escapeString(domainName.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "PUT", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call updateChildDomainValidateBeforeCall(String childIdentifier, String domainName, UpdateChildDomain updateChildDomain, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'childIdentifier' is set
if (childIdentifier == null) {
throw new ApiException("Missing the required parameter 'childIdentifier' when calling updateChildDomain(Async)");
}
// verify the required parameter 'domainName' is set
if (domainName == null) {
throw new ApiException("Missing the required parameter 'domainName' when calling updateChildDomain(Async)");
}
// verify the required parameter 'updateChildDomain' is set
if (updateChildDomain == null) {
throw new ApiException("Missing the required parameter 'updateChildDomain' when calling updateChildDomain(Async)");
}
Call call = updateChildDomainCall(childIdentifier, domainName, updateChildDomain, progressListener, progressRequestListener);
return call;
}
/**
* Update the sender domain of reseller's child based on the childIdentifier and domainName passed
*
* @param childIdentifier Either auth key or id of reseller's child (required)
* @param domainName Pass the existing domain that needs to be updated (required)
* @param updateChildDomain value to update for sender domain (required)
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public void updateChildDomain(String childIdentifier, String domainName, UpdateChildDomain updateChildDomain) throws ApiException {
updateChildDomainWithHttpInfo(childIdentifier, domainName, updateChildDomain);
}
/**
* Update the sender domain of reseller's child based on the childIdentifier and domainName passed
*
* @param childIdentifier Either auth key or id of reseller's child (required)
* @param domainName Pass the existing domain that needs to be updated (required)
* @param updateChildDomain value to update for sender domain (required)
* @return ApiResponse<Void>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse updateChildDomainWithHttpInfo(String childIdentifier, String domainName, UpdateChildDomain updateChildDomain) throws ApiException {
Call call = updateChildDomainValidateBeforeCall(childIdentifier, domainName, updateChildDomain, null, null);
return apiClient.execute(call);
}
/**
* Update the sender domain of reseller's child based on the childIdentifier and domainName passed (asynchronously)
*
* @param childIdentifier Either auth key or id of reseller's child (required)
* @param domainName Pass the existing domain that needs to be updated (required)
* @param updateChildDomain value to update for sender domain (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call updateChildDomainAsync(String childIdentifier, String domainName, UpdateChildDomain updateChildDomain, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = updateChildDomainValidateBeforeCall(childIdentifier, domainName, updateChildDomain, progressListener, progressRequestListener);
apiClient.executeAsync(call, callback);
return call;
}
/**
* Build call for updateResellerChild
* @param childIdentifier Either auth key or id of reseller's child (required)
* @param resellerChild values to update in child profile (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call updateResellerChildCall(String childIdentifier, UpdateChild resellerChild, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = resellerChild;
// create path and map variables
String localVarPath = "/reseller/children/{childIdentifier}"
.replaceAll("\\{" + "childIdentifier" + "\\}", apiClient.escapeString(childIdentifier.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "PUT", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call updateResellerChildValidateBeforeCall(String childIdentifier, UpdateChild resellerChild, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'childIdentifier' is set
if (childIdentifier == null) {
throw new ApiException("Missing the required parameter 'childIdentifier' when calling updateResellerChild(Async)");
}
// verify the required parameter 'resellerChild' is set
if (resellerChild == null) {
throw new ApiException("Missing the required parameter 'resellerChild' when calling updateResellerChild(Async)");
}
Call call = updateResellerChildCall(childIdentifier, resellerChild, progressListener, progressRequestListener);
return call;
}
/**
* Update info of reseller's child based on the child identifier supplied
*
* @param childIdentifier Either auth key or id of reseller's child (required)
* @param resellerChild values to update in child profile (required)
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public void updateResellerChild(String childIdentifier, UpdateChild resellerChild) throws ApiException {
updateResellerChildWithHttpInfo(childIdentifier, resellerChild);
}
/**
* Update info of reseller's child based on the child identifier supplied
*
* @param childIdentifier Either auth key or id of reseller's child (required)
* @param resellerChild values to update in child profile (required)
* @return ApiResponse<Void>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse updateResellerChildWithHttpInfo(String childIdentifier, UpdateChild resellerChild) throws ApiException {
Call call = updateResellerChildValidateBeforeCall(childIdentifier, resellerChild, null, null);
return apiClient.execute(call);
}
/**
* Update info of reseller's child based on the child identifier supplied (asynchronously)
*
* @param childIdentifier Either auth key or id of reseller's child (required)
* @param resellerChild values to update in child profile (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call updateResellerChildAsync(String childIdentifier, UpdateChild resellerChild, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = updateResellerChildValidateBeforeCall(childIdentifier, resellerChild, progressListener, progressRequestListener);
apiClient.executeAsync(call, callback);
return call;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy