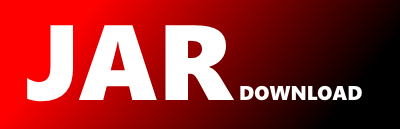
sibApi.TasksApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sib-api-v3-sdk Show documentation
Show all versions of sib-api-v3-sdk Show documentation
SendinBlue's API v3 Java Library
The newest version!
/*
* SendinBlue API
* SendinBlue provide a RESTFul API that can be used with any languages. With this API, you will be able to : - Manage your campaigns and get the statistics - Manage your contacts - Send transactional Emails and SMS - and much more... You can download our wrappers at https://github.com/orgs/sendinblue **Possible responses** | Code | Message | | :-------------: | ------------- | | 200 | OK. Successful Request | | 201 | OK. Successful Creation | | 202 | OK. Request accepted | | 204 | OK. Successful Update/Deletion | | 400 | Error. Bad Request | | 401 | Error. Authentication Needed | | 402 | Error. Not enough credit, plan upgrade needed | | 403 | Error. Permission denied | | 404 | Error. Object does not exist | | 405 | Error. Method not allowed | | 406 | Error. Not Acceptable |
*
* OpenAPI spec version: 3.0.0
* Contact: [email protected]
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package sibApi;
import okhttp3.Call;
import sendinblue.ApiCallback;
import sendinblue.ApiClient;
import sendinblue.ApiException;
import sendinblue.ApiResponse;
import sendinblue.Configuration;
import sendinblue.Pair;
import sendinblue.ProgressRequestBody;
import sendinblue.ProgressResponseBody;
import com.google.gson.reflect.TypeToken;
import java.io.IOException;
import sibModel.Body6;
import sibModel.Body7;
import sibModel.ErrorModel;
import sibModel.InlineResponse2011;
import sibModel.Task;
import sibModel.TaskList;
import sibModel.TaskTypes;
import java.lang.reflect.Type;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class TasksApi {
private ApiClient apiClient;
public TasksApi() {
this(Configuration.getDefaultApiClient());
}
public TasksApi(ApiClient apiClient) {
this.apiClient = apiClient;
}
public ApiClient getApiClient() {
return apiClient;
}
public void setApiClient(ApiClient apiClient) {
this.apiClient = apiClient;
}
/**
* Build call for crmTasksGet
* @param filterType Filter by task type (ID) (optional)
* @param filterStatus Filter by task status (optional)
* @param filterDate Filter by date (optional)
* @param filterAssignTo Filter by assignTo id (optional)
* @param filterContacts Filter by contact ids (optional)
* @param filterDeals Filter by deals ids (optional)
* @param filterCompanies Filter by companies ids (optional)
* @param dateFrom dateFrom to date range filter type (timestamp in milliseconds) (optional)
* @param dateTo dateTo to date range filter type (timestamp in milliseconds) (optional)
* @param offset Index of the first document of the page (optional)
* @param limit Number of documents per page (optional, default to 50)
* @param sort Sort the results in the ascending/descending order. Default order is **descending** by creation if `sort` is not passed (optional)
* @param sortBy The field used to sort field names. (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call crmTasksGetCall(String filterType, String filterStatus, String filterDate, String filterAssignTo, String filterContacts, String filterDeals, String filterCompanies, Integer dateFrom, Integer dateTo, Long offset, Long limit, String sort, String sortBy, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/crm/tasks";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
if (filterType != null)
localVarQueryParams.addAll(apiClient.parameterToPair("filter[type]", filterType));
if (filterStatus != null)
localVarQueryParams.addAll(apiClient.parameterToPair("filter[status]", filterStatus));
if (filterDate != null)
localVarQueryParams.addAll(apiClient.parameterToPair("filter[date]", filterDate));
if (filterAssignTo != null)
localVarQueryParams.addAll(apiClient.parameterToPair("filter[assignTo]", filterAssignTo));
if (filterContacts != null)
localVarQueryParams.addAll(apiClient.parameterToPair("filter[contacts]", filterContacts));
if (filterDeals != null)
localVarQueryParams.addAll(apiClient.parameterToPair("filter[deals]", filterDeals));
if (filterCompanies != null)
localVarQueryParams.addAll(apiClient.parameterToPair("filter[companies]", filterCompanies));
if (dateFrom != null)
localVarQueryParams.addAll(apiClient.parameterToPair("dateFrom", dateFrom));
if (dateTo != null)
localVarQueryParams.addAll(apiClient.parameterToPair("dateTo", dateTo));
if (offset != null)
localVarQueryParams.addAll(apiClient.parameterToPair("offset", offset));
if (limit != null)
localVarQueryParams.addAll(apiClient.parameterToPair("limit", limit));
if (sort != null)
localVarQueryParams.addAll(apiClient.parameterToPair("sort", sort));
if (sortBy != null)
localVarQueryParams.addAll(apiClient.parameterToPair("sortBy", sortBy));
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call crmTasksGetValidateBeforeCall(String filterType, String filterStatus, String filterDate, String filterAssignTo, String filterContacts, String filterDeals, String filterCompanies, Integer dateFrom, Integer dateTo, Long offset, Long limit, String sort, String sortBy, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Call call = crmTasksGetCall(filterType, filterStatus, filterDate, filterAssignTo, filterContacts, filterDeals, filterCompanies, dateFrom, dateTo, offset, limit, sort, sortBy, progressListener, progressRequestListener);
return call;
}
/**
* Get all tasks
*
* @param filterType Filter by task type (ID) (optional)
* @param filterStatus Filter by task status (optional)
* @param filterDate Filter by date (optional)
* @param filterAssignTo Filter by assignTo id (optional)
* @param filterContacts Filter by contact ids (optional)
* @param filterDeals Filter by deals ids (optional)
* @param filterCompanies Filter by companies ids (optional)
* @param dateFrom dateFrom to date range filter type (timestamp in milliseconds) (optional)
* @param dateTo dateTo to date range filter type (timestamp in milliseconds) (optional)
* @param offset Index of the first document of the page (optional)
* @param limit Number of documents per page (optional, default to 50)
* @param sort Sort the results in the ascending/descending order. Default order is **descending** by creation if `sort` is not passed (optional)
* @param sortBy The field used to sort field names. (optional)
* @return TaskList
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public TaskList crmTasksGet(String filterType, String filterStatus, String filterDate, String filterAssignTo, String filterContacts, String filterDeals, String filterCompanies, Integer dateFrom, Integer dateTo, Long offset, Long limit, String sort, String sortBy) throws ApiException {
ApiResponse resp = crmTasksGetWithHttpInfo(filterType, filterStatus, filterDate, filterAssignTo, filterContacts, filterDeals, filterCompanies, dateFrom, dateTo, offset, limit, sort, sortBy);
return resp.getData();
}
/**
* Get all tasks
*
* @param filterType Filter by task type (ID) (optional)
* @param filterStatus Filter by task status (optional)
* @param filterDate Filter by date (optional)
* @param filterAssignTo Filter by assignTo id (optional)
* @param filterContacts Filter by contact ids (optional)
* @param filterDeals Filter by deals ids (optional)
* @param filterCompanies Filter by companies ids (optional)
* @param dateFrom dateFrom to date range filter type (timestamp in milliseconds) (optional)
* @param dateTo dateTo to date range filter type (timestamp in milliseconds) (optional)
* @param offset Index of the first document of the page (optional)
* @param limit Number of documents per page (optional, default to 50)
* @param sort Sort the results in the ascending/descending order. Default order is **descending** by creation if `sort` is not passed (optional)
* @param sortBy The field used to sort field names. (optional)
* @return ApiResponse<TaskList>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse crmTasksGetWithHttpInfo(String filterType, String filterStatus, String filterDate, String filterAssignTo, String filterContacts, String filterDeals, String filterCompanies, Integer dateFrom, Integer dateTo, Long offset, Long limit, String sort, String sortBy) throws ApiException {
Call call = crmTasksGetValidateBeforeCall(filterType, filterStatus, filterDate, filterAssignTo, filterContacts, filterDeals, filterCompanies, dateFrom, dateTo, offset, limit, sort, sortBy, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get all tasks (asynchronously)
*
* @param filterType Filter by task type (ID) (optional)
* @param filterStatus Filter by task status (optional)
* @param filterDate Filter by date (optional)
* @param filterAssignTo Filter by assignTo id (optional)
* @param filterContacts Filter by contact ids (optional)
* @param filterDeals Filter by deals ids (optional)
* @param filterCompanies Filter by companies ids (optional)
* @param dateFrom dateFrom to date range filter type (timestamp in milliseconds) (optional)
* @param dateTo dateTo to date range filter type (timestamp in milliseconds) (optional)
* @param offset Index of the first document of the page (optional)
* @param limit Number of documents per page (optional, default to 50)
* @param sort Sort the results in the ascending/descending order. Default order is **descending** by creation if `sort` is not passed (optional)
* @param sortBy The field used to sort field names. (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call crmTasksGetAsync(String filterType, String filterStatus, String filterDate, String filterAssignTo, String filterContacts, String filterDeals, String filterCompanies, Integer dateFrom, Integer dateTo, Long offset, Long limit, String sort, String sortBy, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = crmTasksGetValidateBeforeCall(filterType, filterStatus, filterDate, filterAssignTo, filterContacts, filterDeals, filterCompanies, dateFrom, dateTo, offset, limit, sort, sortBy, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for crmTasksIdDelete
* @param id (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call crmTasksIdDeleteCall(String id, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/crm/tasks/{id}"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "DELETE", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call crmTasksIdDeleteValidateBeforeCall(String id, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException("Missing the required parameter 'id' when calling crmTasksIdDelete(Async)");
}
Call call = crmTasksIdDeleteCall(id, progressListener, progressRequestListener);
return call;
}
/**
* Delete a task
*
* @param id (required)
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public void crmTasksIdDelete(String id) throws ApiException {
crmTasksIdDeleteWithHttpInfo(id);
}
/**
* Delete a task
*
* @param id (required)
* @return ApiResponse<Void>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse crmTasksIdDeleteWithHttpInfo(String id) throws ApiException {
Call call = crmTasksIdDeleteValidateBeforeCall(id, null, null);
return apiClient.execute(call);
}
/**
* Delete a task (asynchronously)
*
* @param id (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call crmTasksIdDeleteAsync(String id, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = crmTasksIdDeleteValidateBeforeCall(id, progressListener, progressRequestListener);
apiClient.executeAsync(call, callback);
return call;
}
/**
* Build call for crmTasksIdGet
* @param id (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call crmTasksIdGetCall(String id, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/crm/tasks/{id}"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call crmTasksIdGetValidateBeforeCall(String id, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException("Missing the required parameter 'id' when calling crmTasksIdGet(Async)");
}
Call call = crmTasksIdGetCall(id, progressListener, progressRequestListener);
return call;
}
/**
* Get a task
*
* @param id (required)
* @return Task
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public Task crmTasksIdGet(String id) throws ApiException {
ApiResponse resp = crmTasksIdGetWithHttpInfo(id);
return resp.getData();
}
/**
* Get a task
*
* @param id (required)
* @return ApiResponse<Task>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse crmTasksIdGetWithHttpInfo(String id) throws ApiException {
Call call = crmTasksIdGetValidateBeforeCall(id, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get a task (asynchronously)
*
* @param id (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call crmTasksIdGetAsync(String id, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = crmTasksIdGetValidateBeforeCall(id, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for crmTasksIdPatch
* @param id (required)
* @param body Updated task details. (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call crmTasksIdPatchCall(String id, Body7 body, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = body;
// create path and map variables
String localVarPath = "/crm/tasks/{id}"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "PATCH", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call crmTasksIdPatchValidateBeforeCall(String id, Body7 body, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException("Missing the required parameter 'id' when calling crmTasksIdPatch(Async)");
}
// verify the required parameter 'body' is set
if (body == null) {
throw new ApiException("Missing the required parameter 'body' when calling crmTasksIdPatch(Async)");
}
Call call = crmTasksIdPatchCall(id, body, progressListener, progressRequestListener);
return call;
}
/**
* Update a task
*
* @param id (required)
* @param body Updated task details. (required)
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public void crmTasksIdPatch(String id, Body7 body) throws ApiException {
crmTasksIdPatchWithHttpInfo(id, body);
}
/**
* Update a task
*
* @param id (required)
* @param body Updated task details. (required)
* @return ApiResponse<Void>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse crmTasksIdPatchWithHttpInfo(String id, Body7 body) throws ApiException {
Call call = crmTasksIdPatchValidateBeforeCall(id, body, null, null);
return apiClient.execute(call);
}
/**
* Update a task (asynchronously)
*
* @param id (required)
* @param body Updated task details. (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call crmTasksIdPatchAsync(String id, Body7 body, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = crmTasksIdPatchValidateBeforeCall(id, body, progressListener, progressRequestListener);
apiClient.executeAsync(call, callback);
return call;
}
/**
* Build call for crmTasksPost
* @param body Task name. (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call crmTasksPostCall(Body6 body, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = body;
// create path and map variables
String localVarPath = "/crm/tasks";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call crmTasksPostValidateBeforeCall(Body6 body, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'body' is set
if (body == null) {
throw new ApiException("Missing the required parameter 'body' when calling crmTasksPost(Async)");
}
Call call = crmTasksPostCall(body, progressListener, progressRequestListener);
return call;
}
/**
* Create a task
*
* @param body Task name. (required)
* @return InlineResponse2011
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public InlineResponse2011 crmTasksPost(Body6 body) throws ApiException {
ApiResponse resp = crmTasksPostWithHttpInfo(body);
return resp.getData();
}
/**
* Create a task
*
* @param body Task name. (required)
* @return ApiResponse<InlineResponse2011>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse crmTasksPostWithHttpInfo(Body6 body) throws ApiException {
Call call = crmTasksPostValidateBeforeCall(body, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Create a task (asynchronously)
*
* @param body Task name. (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call crmTasksPostAsync(Body6 body, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = crmTasksPostValidateBeforeCall(body, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for crmTasktypesGet
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call crmTasktypesGetCall(final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/crm/tasktypes";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call crmTasktypesGetValidateBeforeCall(final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Call call = crmTasktypesGetCall(progressListener, progressRequestListener);
return call;
}
/**
* Get all task types
*
* @return TaskTypes
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public TaskTypes crmTasktypesGet() throws ApiException {
ApiResponse resp = crmTasktypesGetWithHttpInfo();
return resp.getData();
}
/**
* Get all task types
*
* @return ApiResponse<TaskTypes>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse crmTasktypesGetWithHttpInfo() throws ApiException {
Call call = crmTasktypesGetValidateBeforeCall(null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get all task types (asynchronously)
*
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call crmTasktypesGetAsync(final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = crmTasktypesGetValidateBeforeCall(progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy