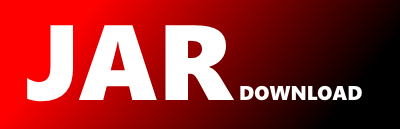
sibApi.TransactionalEmailsApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sib-api-v3-sdk Show documentation
Show all versions of sib-api-v3-sdk Show documentation
SendinBlue's API v3 Java Library
The newest version!
/*
* SendinBlue API
* SendinBlue provide a RESTFul API that can be used with any languages. With this API, you will be able to : - Manage your campaigns and get the statistics - Manage your contacts - Send transactional Emails and SMS - and much more... You can download our wrappers at https://github.com/orgs/sendinblue **Possible responses** | Code | Message | | :-------------: | ------------- | | 200 | OK. Successful Request | | 201 | OK. Successful Creation | | 202 | OK. Request accepted | | 204 | OK. Successful Update/Deletion | | 400 | Error. Bad Request | | 401 | Error. Authentication Needed | | 402 | Error. Not enough credit, plan upgrade needed | | 403 | Error. Permission denied | | 404 | Error. Object does not exist | | 405 | Error. Method not allowed | | 406 | Error. Not Acceptable |
*
* OpenAPI spec version: 3.0.0
* Contact: [email protected]
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package sibApi;
import okhttp3.Call;
import sendinblue.ApiCallback;
import sendinblue.ApiClient;
import sendinblue.ApiException;
import sendinblue.ApiResponse;
import sendinblue.Configuration;
import sendinblue.Pair;
import sendinblue.ProgressRequestBody;
import sendinblue.ProgressResponseBody;
import com.google.gson.reflect.TypeToken;
import java.io.IOException;
import sibModel.BlockDomain;
import sibModel.CreateModel;
import sibModel.CreateSmtpEmail;
import sibModel.CreateSmtpTemplate;
import sibModel.DeleteHardbounces;
import sibModel.ErrorModel;
import sibModel.GetAggregatedReport;
import sibModel.GetBlockedDomains;
import sibModel.GetEmailEventReport;
import sibModel.GetReports;
import sibModel.GetScheduledEmailByBatchId;
import sibModel.GetScheduledEmailByMessageId;
import sibModel.GetSmtpTemplateOverview;
import sibModel.GetSmtpTemplates;
import sibModel.GetTransacBlockedContacts;
import sibModel.GetTransacEmailContent;
import sibModel.GetTransacEmailsList;
import org.threeten.bp.LocalDate;
import sibModel.PostSendFailed;
import sibModel.ScheduleSmtpEmail;
import sibModel.SendSmtpEmail;
import sibModel.SendTestEmail;
import sibModel.UpdateSmtpTemplate;
import java.lang.reflect.Type;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class TransactionalEmailsApi {
private ApiClient apiClient;
public TransactionalEmailsApi() {
this(Configuration.getDefaultApiClient());
}
public TransactionalEmailsApi(ApiClient apiClient) {
this.apiClient = apiClient;
}
public ApiClient getApiClient() {
return apiClient;
}
public void setApiClient(ApiClient apiClient) {
this.apiClient = apiClient;
}
/**
* Build call for blockNewDomain
* @param blockDomain (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call blockNewDomainCall(BlockDomain blockDomain, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = blockDomain;
// create path and map variables
String localVarPath = "/smtp/blockedDomains";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call blockNewDomainValidateBeforeCall(BlockDomain blockDomain, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'blockDomain' is set
if (blockDomain == null) {
throw new ApiException("Missing the required parameter 'blockDomain' when calling blockNewDomain(Async)");
}
Call call = blockNewDomainCall(blockDomain, progressListener, progressRequestListener);
return call;
}
/**
* Add a new domain to the list of blocked domains
* Blocks a new domain in order to avoid messages being sent to the same
* @param blockDomain (required)
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public void blockNewDomain(BlockDomain blockDomain) throws ApiException {
blockNewDomainWithHttpInfo(blockDomain);
}
/**
* Add a new domain to the list of blocked domains
* Blocks a new domain in order to avoid messages being sent to the same
* @param blockDomain (required)
* @return ApiResponse<Void>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse blockNewDomainWithHttpInfo(BlockDomain blockDomain) throws ApiException {
Call call = blockNewDomainValidateBeforeCall(blockDomain, null, null);
return apiClient.execute(call);
}
/**
* Add a new domain to the list of blocked domains (asynchronously)
* Blocks a new domain in order to avoid messages being sent to the same
* @param blockDomain (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call blockNewDomainAsync(BlockDomain blockDomain, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = blockNewDomainValidateBeforeCall(blockDomain, progressListener, progressRequestListener);
apiClient.executeAsync(call, callback);
return call;
}
/**
* Build call for createSmtpTemplate
* @param smtpTemplate values to update in transactional email template (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call createSmtpTemplateCall(CreateSmtpTemplate smtpTemplate, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = smtpTemplate;
// create path and map variables
String localVarPath = "/smtp/templates";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call createSmtpTemplateValidateBeforeCall(CreateSmtpTemplate smtpTemplate, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'smtpTemplate' is set
if (smtpTemplate == null) {
throw new ApiException("Missing the required parameter 'smtpTemplate' when calling createSmtpTemplate(Async)");
}
Call call = createSmtpTemplateCall(smtpTemplate, progressListener, progressRequestListener);
return call;
}
/**
* Create an email template
*
* @param smtpTemplate values to update in transactional email template (required)
* @return CreateModel
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public CreateModel createSmtpTemplate(CreateSmtpTemplate smtpTemplate) throws ApiException {
ApiResponse resp = createSmtpTemplateWithHttpInfo(smtpTemplate);
return resp.getData();
}
/**
* Create an email template
*
* @param smtpTemplate values to update in transactional email template (required)
* @return ApiResponse<CreateModel>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse createSmtpTemplateWithHttpInfo(CreateSmtpTemplate smtpTemplate) throws ApiException {
Call call = createSmtpTemplateValidateBeforeCall(smtpTemplate, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Create an email template (asynchronously)
*
* @param smtpTemplate values to update in transactional email template (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call createSmtpTemplateAsync(CreateSmtpTemplate smtpTemplate, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = createSmtpTemplateValidateBeforeCall(smtpTemplate, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for deleteBlockedDomain
* @param domain The name of the domain to be deleted (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call deleteBlockedDomainCall(String domain, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/smtp/blockedDomains/{domain}"
.replaceAll("\\{" + "domain" + "\\}", apiClient.escapeString(domain.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "DELETE", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call deleteBlockedDomainValidateBeforeCall(String domain, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'domain' is set
if (domain == null) {
throw new ApiException("Missing the required parameter 'domain' when calling deleteBlockedDomain(Async)");
}
Call call = deleteBlockedDomainCall(domain, progressListener, progressRequestListener);
return call;
}
/**
* Unblock an existing domain from the list of blocked domains
* Unblocks an existing domain from the list of blocked domains
* @param domain The name of the domain to be deleted (required)
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public void deleteBlockedDomain(String domain) throws ApiException {
deleteBlockedDomainWithHttpInfo(domain);
}
/**
* Unblock an existing domain from the list of blocked domains
* Unblocks an existing domain from the list of blocked domains
* @param domain The name of the domain to be deleted (required)
* @return ApiResponse<Void>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse deleteBlockedDomainWithHttpInfo(String domain) throws ApiException {
Call call = deleteBlockedDomainValidateBeforeCall(domain, null, null);
return apiClient.execute(call);
}
/**
* Unblock an existing domain from the list of blocked domains (asynchronously)
* Unblocks an existing domain from the list of blocked domains
* @param domain The name of the domain to be deleted (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call deleteBlockedDomainAsync(String domain, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = deleteBlockedDomainValidateBeforeCall(domain, progressListener, progressRequestListener);
apiClient.executeAsync(call, callback);
return call;
}
/**
* Build call for deleteHardbounces
* @param deleteHardbounces values to delete hardbounces (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call deleteHardbouncesCall(DeleteHardbounces deleteHardbounces, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = deleteHardbounces;
// create path and map variables
String localVarPath = "/smtp/deleteHardbounces";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call deleteHardbouncesValidateBeforeCall(DeleteHardbounces deleteHardbounces, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Call call = deleteHardbouncesCall(deleteHardbounces, progressListener, progressRequestListener);
return call;
}
/**
* Delete hardbounces
* Delete hardbounces. To use carefully (e.g. in case of temporary ISP failures)
* @param deleteHardbounces values to delete hardbounces (optional)
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public void deleteHardbounces(DeleteHardbounces deleteHardbounces) throws ApiException {
deleteHardbouncesWithHttpInfo(deleteHardbounces);
}
/**
* Delete hardbounces
* Delete hardbounces. To use carefully (e.g. in case of temporary ISP failures)
* @param deleteHardbounces values to delete hardbounces (optional)
* @return ApiResponse<Void>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse deleteHardbouncesWithHttpInfo(DeleteHardbounces deleteHardbounces) throws ApiException {
Call call = deleteHardbouncesValidateBeforeCall(deleteHardbounces, null, null);
return apiClient.execute(call);
}
/**
* Delete hardbounces (asynchronously)
* Delete hardbounces. To use carefully (e.g. in case of temporary ISP failures)
* @param deleteHardbounces values to delete hardbounces (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call deleteHardbouncesAsync(DeleteHardbounces deleteHardbounces, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = deleteHardbouncesValidateBeforeCall(deleteHardbounces, progressListener, progressRequestListener);
apiClient.executeAsync(call, callback);
return call;
}
/**
* Build call for deleteScheduledEmailById
* @param identifier The `batchId` of scheduled emails batch (Should be a valid UUIDv4) or the `messageId` of scheduled email. (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call deleteScheduledEmailByIdCall(String identifier, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/smtp/email/{identifier}"
.replaceAll("\\{" + "identifier" + "\\}", apiClient.escapeString(identifier.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "DELETE", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call deleteScheduledEmailByIdValidateBeforeCall(String identifier, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'identifier' is set
if (identifier == null) {
throw new ApiException("Missing the required parameter 'identifier' when calling deleteScheduledEmailById(Async)");
}
Call call = deleteScheduledEmailByIdCall(identifier, progressListener, progressRequestListener);
return call;
}
/**
* Delete scheduled emails by batchId or messageId
* Delete scheduled batch of emails by batchId or single scheduled email by messageId
* @param identifier The `batchId` of scheduled emails batch (Should be a valid UUIDv4) or the `messageId` of scheduled email. (required)
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public void deleteScheduledEmailById(String identifier) throws ApiException {
deleteScheduledEmailByIdWithHttpInfo(identifier);
}
/**
* Delete scheduled emails by batchId or messageId
* Delete scheduled batch of emails by batchId or single scheduled email by messageId
* @param identifier The `batchId` of scheduled emails batch (Should be a valid UUIDv4) or the `messageId` of scheduled email. (required)
* @return ApiResponse<Void>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse deleteScheduledEmailByIdWithHttpInfo(String identifier) throws ApiException {
Call call = deleteScheduledEmailByIdValidateBeforeCall(identifier, null, null);
return apiClient.execute(call);
}
/**
* Delete scheduled emails by batchId or messageId (asynchronously)
* Delete scheduled batch of emails by batchId or single scheduled email by messageId
* @param identifier The `batchId` of scheduled emails batch (Should be a valid UUIDv4) or the `messageId` of scheduled email. (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call deleteScheduledEmailByIdAsync(String identifier, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = deleteScheduledEmailByIdValidateBeforeCall(identifier, progressListener, progressRequestListener);
apiClient.executeAsync(call, callback);
return call;
}
/**
* Build call for deleteSmtpTemplate
* @param templateId id of the template (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call deleteSmtpTemplateCall(Long templateId, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/smtp/templates/{templateId}"
.replaceAll("\\{" + "templateId" + "\\}", apiClient.escapeString(templateId.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "DELETE", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call deleteSmtpTemplateValidateBeforeCall(Long templateId, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'templateId' is set
if (templateId == null) {
throw new ApiException("Missing the required parameter 'templateId' when calling deleteSmtpTemplate(Async)");
}
Call call = deleteSmtpTemplateCall(templateId, progressListener, progressRequestListener);
return call;
}
/**
* Delete an inactive email template
*
* @param templateId id of the template (required)
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public void deleteSmtpTemplate(Long templateId) throws ApiException {
deleteSmtpTemplateWithHttpInfo(templateId);
}
/**
* Delete an inactive email template
*
* @param templateId id of the template (required)
* @return ApiResponse<Void>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse deleteSmtpTemplateWithHttpInfo(Long templateId) throws ApiException {
Call call = deleteSmtpTemplateValidateBeforeCall(templateId, null, null);
return apiClient.execute(call);
}
/**
* Delete an inactive email template (asynchronously)
*
* @param templateId id of the template (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call deleteSmtpTemplateAsync(Long templateId, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = deleteSmtpTemplateValidateBeforeCall(templateId, progressListener, progressRequestListener);
apiClient.executeAsync(call, callback);
return call;
}
/**
* Build call for getAggregatedSmtpReport
* @param startDate Mandatory if endDate is used. Starting date of the report (YYYY-MM-DD). Must be lower than equal to endDate (optional)
* @param endDate Mandatory if startDate is used. Ending date of the report (YYYY-MM-DD). Must be greater than equal to startDate (optional)
* @param days Number of days in the past including today (positive integer). Not compatible with 'startDate' and 'endDate' (optional)
* @param tag Tag of the emails (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call getAggregatedSmtpReportCall(String startDate, String endDate, Long days, String tag, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/smtp/statistics/aggregatedReport";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
if (startDate != null)
localVarQueryParams.addAll(apiClient.parameterToPair("startDate", startDate));
if (endDate != null)
localVarQueryParams.addAll(apiClient.parameterToPair("endDate", endDate));
if (days != null)
localVarQueryParams.addAll(apiClient.parameterToPair("days", days));
if (tag != null)
localVarQueryParams.addAll(apiClient.parameterToPair("tag", tag));
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call getAggregatedSmtpReportValidateBeforeCall(String startDate, String endDate, Long days, String tag, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Call call = getAggregatedSmtpReportCall(startDate, endDate, days, tag, progressListener, progressRequestListener);
return call;
}
/**
* Get your transactional email activity aggregated over a period of time
* This endpoint will show the aggregated stats for past 90 days by default if `startDate` and `endDate` OR `days` is not passed. The date range can not exceed 90 days
* @param startDate Mandatory if endDate is used. Starting date of the report (YYYY-MM-DD). Must be lower than equal to endDate (optional)
* @param endDate Mandatory if startDate is used. Ending date of the report (YYYY-MM-DD). Must be greater than equal to startDate (optional)
* @param days Number of days in the past including today (positive integer). Not compatible with 'startDate' and 'endDate' (optional)
* @param tag Tag of the emails (optional)
* @return GetAggregatedReport
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public GetAggregatedReport getAggregatedSmtpReport(String startDate, String endDate, Long days, String tag) throws ApiException {
ApiResponse resp = getAggregatedSmtpReportWithHttpInfo(startDate, endDate, days, tag);
return resp.getData();
}
/**
* Get your transactional email activity aggregated over a period of time
* This endpoint will show the aggregated stats for past 90 days by default if `startDate` and `endDate` OR `days` is not passed. The date range can not exceed 90 days
* @param startDate Mandatory if endDate is used. Starting date of the report (YYYY-MM-DD). Must be lower than equal to endDate (optional)
* @param endDate Mandatory if startDate is used. Ending date of the report (YYYY-MM-DD). Must be greater than equal to startDate (optional)
* @param days Number of days in the past including today (positive integer). Not compatible with 'startDate' and 'endDate' (optional)
* @param tag Tag of the emails (optional)
* @return ApiResponse<GetAggregatedReport>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getAggregatedSmtpReportWithHttpInfo(String startDate, String endDate, Long days, String tag) throws ApiException {
Call call = getAggregatedSmtpReportValidateBeforeCall(startDate, endDate, days, tag, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get your transactional email activity aggregated over a period of time (asynchronously)
* This endpoint will show the aggregated stats for past 90 days by default if `startDate` and `endDate` OR `days` is not passed. The date range can not exceed 90 days
* @param startDate Mandatory if endDate is used. Starting date of the report (YYYY-MM-DD). Must be lower than equal to endDate (optional)
* @param endDate Mandatory if startDate is used. Ending date of the report (YYYY-MM-DD). Must be greater than equal to startDate (optional)
* @param days Number of days in the past including today (positive integer). Not compatible with 'startDate' and 'endDate' (optional)
* @param tag Tag of the emails (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call getAggregatedSmtpReportAsync(String startDate, String endDate, Long days, String tag, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = getAggregatedSmtpReportValidateBeforeCall(startDate, endDate, days, tag, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for getBlockedDomains
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call getBlockedDomainsCall(final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/smtp/blockedDomains";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call getBlockedDomainsValidateBeforeCall(final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Call call = getBlockedDomainsCall(progressListener, progressRequestListener);
return call;
}
/**
* Get the list of blocked domains
* Get the list of blocked domains
* @return GetBlockedDomains
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public GetBlockedDomains getBlockedDomains() throws ApiException {
ApiResponse resp = getBlockedDomainsWithHttpInfo();
return resp.getData();
}
/**
* Get the list of blocked domains
* Get the list of blocked domains
* @return ApiResponse<GetBlockedDomains>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getBlockedDomainsWithHttpInfo() throws ApiException {
Call call = getBlockedDomainsValidateBeforeCall(null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get the list of blocked domains (asynchronously)
* Get the list of blocked domains
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call getBlockedDomainsAsync(final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = getBlockedDomainsValidateBeforeCall(progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for getEmailEventReport
* @param limit Number limitation for the result returned (optional, default to 2500)
* @param offset Beginning point in the list to retrieve from. (optional, default to 0)
* @param startDate Mandatory if endDate is used. Starting date of the report (YYYY-MM-DD). Must be lower than equal to endDate (optional)
* @param endDate Mandatory if startDate is used. Ending date of the report (YYYY-MM-DD). Must be greater than equal to startDate (optional)
* @param days Number of days in the past including today (positive integer). Not compatible with 'startDate' and 'endDate' (optional)
* @param email Filter the report for a specific email addresses (optional)
* @param event Filter the report for a specific event type (optional)
* @param tags Filter the report for tags (serialized and urlencoded array) (optional)
* @param messageId Filter on a specific message id (optional)
* @param templateId Filter on a specific template id (optional)
* @param sort Sort the results in the ascending/descending order of record creation. Default order is **descending** if `sort` is not passed (optional, default to desc)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call getEmailEventReportCall(Long limit, Long offset, String startDate, String endDate, Long days, String email, String event, String tags, String messageId, Long templateId, String sort, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/smtp/statistics/events";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
if (limit != null)
localVarQueryParams.addAll(apiClient.parameterToPair("limit", limit));
if (offset != null)
localVarQueryParams.addAll(apiClient.parameterToPair("offset", offset));
if (startDate != null)
localVarQueryParams.addAll(apiClient.parameterToPair("startDate", startDate));
if (endDate != null)
localVarQueryParams.addAll(apiClient.parameterToPair("endDate", endDate));
if (days != null)
localVarQueryParams.addAll(apiClient.parameterToPair("days", days));
if (email != null)
localVarQueryParams.addAll(apiClient.parameterToPair("email", email));
if (event != null)
localVarQueryParams.addAll(apiClient.parameterToPair("event", event));
if (tags != null)
localVarQueryParams.addAll(apiClient.parameterToPair("tags", tags));
if (messageId != null)
localVarQueryParams.addAll(apiClient.parameterToPair("messageId", messageId));
if (templateId != null)
localVarQueryParams.addAll(apiClient.parameterToPair("templateId", templateId));
if (sort != null)
localVarQueryParams.addAll(apiClient.parameterToPair("sort", sort));
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call getEmailEventReportValidateBeforeCall(Long limit, Long offset, String startDate, String endDate, Long days, String email, String event, String tags, String messageId, Long templateId, String sort, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Call call = getEmailEventReportCall(limit, offset, startDate, endDate, days, email, event, tags, messageId, templateId, sort, progressListener, progressRequestListener);
return call;
}
/**
* Get all your transactional email activity (unaggregated events)
* This endpoint will show the aggregated stats for past 30 days by default if `startDate` and `endDate` OR `days` is not passed. The date range can not exceed 90 days
* @param limit Number limitation for the result returned (optional, default to 2500)
* @param offset Beginning point in the list to retrieve from. (optional, default to 0)
* @param startDate Mandatory if endDate is used. Starting date of the report (YYYY-MM-DD). Must be lower than equal to endDate (optional)
* @param endDate Mandatory if startDate is used. Ending date of the report (YYYY-MM-DD). Must be greater than equal to startDate (optional)
* @param days Number of days in the past including today (positive integer). Not compatible with 'startDate' and 'endDate' (optional)
* @param email Filter the report for a specific email addresses (optional)
* @param event Filter the report for a specific event type (optional)
* @param tags Filter the report for tags (serialized and urlencoded array) (optional)
* @param messageId Filter on a specific message id (optional)
* @param templateId Filter on a specific template id (optional)
* @param sort Sort the results in the ascending/descending order of record creation. Default order is **descending** if `sort` is not passed (optional, default to desc)
* @return GetEmailEventReport
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public GetEmailEventReport getEmailEventReport(Long limit, Long offset, String startDate, String endDate, Long days, String email, String event, String tags, String messageId, Long templateId, String sort) throws ApiException {
ApiResponse resp = getEmailEventReportWithHttpInfo(limit, offset, startDate, endDate, days, email, event, tags, messageId, templateId, sort);
return resp.getData();
}
/**
* Get all your transactional email activity (unaggregated events)
* This endpoint will show the aggregated stats for past 30 days by default if `startDate` and `endDate` OR `days` is not passed. The date range can not exceed 90 days
* @param limit Number limitation for the result returned (optional, default to 2500)
* @param offset Beginning point in the list to retrieve from. (optional, default to 0)
* @param startDate Mandatory if endDate is used. Starting date of the report (YYYY-MM-DD). Must be lower than equal to endDate (optional)
* @param endDate Mandatory if startDate is used. Ending date of the report (YYYY-MM-DD). Must be greater than equal to startDate (optional)
* @param days Number of days in the past including today (positive integer). Not compatible with 'startDate' and 'endDate' (optional)
* @param email Filter the report for a specific email addresses (optional)
* @param event Filter the report for a specific event type (optional)
* @param tags Filter the report for tags (serialized and urlencoded array) (optional)
* @param messageId Filter on a specific message id (optional)
* @param templateId Filter on a specific template id (optional)
* @param sort Sort the results in the ascending/descending order of record creation. Default order is **descending** if `sort` is not passed (optional, default to desc)
* @return ApiResponse<GetEmailEventReport>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getEmailEventReportWithHttpInfo(Long limit, Long offset, String startDate, String endDate, Long days, String email, String event, String tags, String messageId, Long templateId, String sort) throws ApiException {
Call call = getEmailEventReportValidateBeforeCall(limit, offset, startDate, endDate, days, email, event, tags, messageId, templateId, sort, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get all your transactional email activity (unaggregated events) (asynchronously)
* This endpoint will show the aggregated stats for past 30 days by default if `startDate` and `endDate` OR `days` is not passed. The date range can not exceed 90 days
* @param limit Number limitation for the result returned (optional, default to 2500)
* @param offset Beginning point in the list to retrieve from. (optional, default to 0)
* @param startDate Mandatory if endDate is used. Starting date of the report (YYYY-MM-DD). Must be lower than equal to endDate (optional)
* @param endDate Mandatory if startDate is used. Ending date of the report (YYYY-MM-DD). Must be greater than equal to startDate (optional)
* @param days Number of days in the past including today (positive integer). Not compatible with 'startDate' and 'endDate' (optional)
* @param email Filter the report for a specific email addresses (optional)
* @param event Filter the report for a specific event type (optional)
* @param tags Filter the report for tags (serialized and urlencoded array) (optional)
* @param messageId Filter on a specific message id (optional)
* @param templateId Filter on a specific template id (optional)
* @param sort Sort the results in the ascending/descending order of record creation. Default order is **descending** if `sort` is not passed (optional, default to desc)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call getEmailEventReportAsync(Long limit, Long offset, String startDate, String endDate, Long days, String email, String event, String tags, String messageId, Long templateId, String sort, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = getEmailEventReportValidateBeforeCall(limit, offset, startDate, endDate, days, email, event, tags, messageId, templateId, sort, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for getScheduledEmailByBatchId
* @param batchId The batchId of scheduled emails batch (Should be a valid UUIDv4) (required)
* @param startDate Mandatory if `endDate` is used. Starting date (YYYY-MM-DD) from which you want to fetch the list. Can be maximum 30 days older tha current date. (optional)
* @param endDate Mandatory if `startDate` is used. Ending date (YYYY-MM-DD) till which you want to fetch the list. Maximum time period that can be selected is one month. (optional)
* @param sort Sort the results in the ascending/descending order of record creation. Default order is **descending** if `sort` is not passed (optional, default to desc)
* @param status Filter the records by `status` of the scheduled email batch or message. (optional)
* @param limit Number of documents returned per page (optional, default to 100)
* @param offset Index of the first document on the page (optional, default to 0)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call getScheduledEmailByBatchIdCall(String batchId, LocalDate startDate, LocalDate endDate, String sort, String status, Long limit, Long offset, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/smtp/emailStatus/{batchId}"
.replaceAll("\\{" + "batchId" + "\\}", apiClient.escapeString(batchId.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
if (startDate != null)
localVarQueryParams.addAll(apiClient.parameterToPair("startDate", startDate));
if (endDate != null)
localVarQueryParams.addAll(apiClient.parameterToPair("endDate", endDate));
if (sort != null)
localVarQueryParams.addAll(apiClient.parameterToPair("sort", sort));
if (status != null)
localVarQueryParams.addAll(apiClient.parameterToPair("status", status));
if (limit != null)
localVarQueryParams.addAll(apiClient.parameterToPair("limit", limit));
if (offset != null)
localVarQueryParams.addAll(apiClient.parameterToPair("offset", offset));
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call getScheduledEmailByBatchIdValidateBeforeCall(String batchId, LocalDate startDate, LocalDate endDate, String sort, String status, Long limit, Long offset, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'batchId' is set
if (batchId == null) {
throw new ApiException("Missing the required parameter 'batchId' when calling getScheduledEmailByBatchId(Async)");
}
Call call = getScheduledEmailByBatchIdCall(batchId, startDate, endDate, sort, status, limit, offset, progressListener, progressRequestListener);
return call;
}
/**
* Fetch scheduled emails by batchId
* Fetch scheduled batch of emails by batchId (Can retrieve data upto 30 days old)
* @param batchId The batchId of scheduled emails batch (Should be a valid UUIDv4) (required)
* @param startDate Mandatory if `endDate` is used. Starting date (YYYY-MM-DD) from which you want to fetch the list. Can be maximum 30 days older tha current date. (optional)
* @param endDate Mandatory if `startDate` is used. Ending date (YYYY-MM-DD) till which you want to fetch the list. Maximum time period that can be selected is one month. (optional)
* @param sort Sort the results in the ascending/descending order of record creation. Default order is **descending** if `sort` is not passed (optional, default to desc)
* @param status Filter the records by `status` of the scheduled email batch or message. (optional)
* @param limit Number of documents returned per page (optional, default to 100)
* @param offset Index of the first document on the page (optional, default to 0)
* @return GetScheduledEmailByBatchId
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public GetScheduledEmailByBatchId getScheduledEmailByBatchId(String batchId, LocalDate startDate, LocalDate endDate, String sort, String status, Long limit, Long offset) throws ApiException {
ApiResponse resp = getScheduledEmailByBatchIdWithHttpInfo(batchId, startDate, endDate, sort, status, limit, offset);
return resp.getData();
}
/**
* Fetch scheduled emails by batchId
* Fetch scheduled batch of emails by batchId (Can retrieve data upto 30 days old)
* @param batchId The batchId of scheduled emails batch (Should be a valid UUIDv4) (required)
* @param startDate Mandatory if `endDate` is used. Starting date (YYYY-MM-DD) from which you want to fetch the list. Can be maximum 30 days older tha current date. (optional)
* @param endDate Mandatory if `startDate` is used. Ending date (YYYY-MM-DD) till which you want to fetch the list. Maximum time period that can be selected is one month. (optional)
* @param sort Sort the results in the ascending/descending order of record creation. Default order is **descending** if `sort` is not passed (optional, default to desc)
* @param status Filter the records by `status` of the scheduled email batch or message. (optional)
* @param limit Number of documents returned per page (optional, default to 100)
* @param offset Index of the first document on the page (optional, default to 0)
* @return ApiResponse<GetScheduledEmailByBatchId>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getScheduledEmailByBatchIdWithHttpInfo(String batchId, LocalDate startDate, LocalDate endDate, String sort, String status, Long limit, Long offset) throws ApiException {
Call call = getScheduledEmailByBatchIdValidateBeforeCall(batchId, startDate, endDate, sort, status, limit, offset, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Fetch scheduled emails by batchId (asynchronously)
* Fetch scheduled batch of emails by batchId (Can retrieve data upto 30 days old)
* @param batchId The batchId of scheduled emails batch (Should be a valid UUIDv4) (required)
* @param startDate Mandatory if `endDate` is used. Starting date (YYYY-MM-DD) from which you want to fetch the list. Can be maximum 30 days older tha current date. (optional)
* @param endDate Mandatory if `startDate` is used. Ending date (YYYY-MM-DD) till which you want to fetch the list. Maximum time period that can be selected is one month. (optional)
* @param sort Sort the results in the ascending/descending order of record creation. Default order is **descending** if `sort` is not passed (optional, default to desc)
* @param status Filter the records by `status` of the scheduled email batch or message. (optional)
* @param limit Number of documents returned per page (optional, default to 100)
* @param offset Index of the first document on the page (optional, default to 0)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call getScheduledEmailByBatchIdAsync(String batchId, LocalDate startDate, LocalDate endDate, String sort, String status, Long limit, Long offset, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = getScheduledEmailByBatchIdValidateBeforeCall(batchId, startDate, endDate, sort, status, limit, offset, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for getScheduledEmailByMessageId
* @param messageId The messageId of scheduled email (required)
* @param startDate Mandatory if endDate is used. Starting date (YYYY-MM-DD) from which you want to fetch the list. Can be maximum 30 days older tha current date. (optional)
* @param endDate Mandatory if startDate is used. Ending date (YYYY-MM-DD) till which you want to fetch the list. Maximum time period that can be selected is one month. (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call getScheduledEmailByMessageIdCall(String messageId, LocalDate startDate, LocalDate endDate, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/smtp/emailStatus/{messageId}"
.replaceAll("\\{" + "messageId" + "\\}", apiClient.escapeString(messageId.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
if (startDate != null)
localVarQueryParams.addAll(apiClient.parameterToPair("startDate", startDate));
if (endDate != null)
localVarQueryParams.addAll(apiClient.parameterToPair("endDate", endDate));
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call getScheduledEmailByMessageIdValidateBeforeCall(String messageId, LocalDate startDate, LocalDate endDate, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'messageId' is set
if (messageId == null) {
throw new ApiException("Missing the required parameter 'messageId' when calling getScheduledEmailByMessageId(Async)");
}
Call call = getScheduledEmailByMessageIdCall(messageId, startDate, endDate, progressListener, progressRequestListener);
return call;
}
/**
* Fetch scheduled email by messageId
* Fetch scheduled email by messageId (Can retrieve data upto 30 days old)
* @param messageId The messageId of scheduled email (required)
* @param startDate Mandatory if endDate is used. Starting date (YYYY-MM-DD) from which you want to fetch the list. Can be maximum 30 days older tha current date. (optional)
* @param endDate Mandatory if startDate is used. Ending date (YYYY-MM-DD) till which you want to fetch the list. Maximum time period that can be selected is one month. (optional)
* @return GetScheduledEmailByMessageId
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public GetScheduledEmailByMessageId getScheduledEmailByMessageId(String messageId, LocalDate startDate, LocalDate endDate) throws ApiException {
ApiResponse resp = getScheduledEmailByMessageIdWithHttpInfo(messageId, startDate, endDate);
return resp.getData();
}
/**
* Fetch scheduled email by messageId
* Fetch scheduled email by messageId (Can retrieve data upto 30 days old)
* @param messageId The messageId of scheduled email (required)
* @param startDate Mandatory if endDate is used. Starting date (YYYY-MM-DD) from which you want to fetch the list. Can be maximum 30 days older tha current date. (optional)
* @param endDate Mandatory if startDate is used. Ending date (YYYY-MM-DD) till which you want to fetch the list. Maximum time period that can be selected is one month. (optional)
* @return ApiResponse<GetScheduledEmailByMessageId>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getScheduledEmailByMessageIdWithHttpInfo(String messageId, LocalDate startDate, LocalDate endDate) throws ApiException {
Call call = getScheduledEmailByMessageIdValidateBeforeCall(messageId, startDate, endDate, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Fetch scheduled email by messageId (asynchronously)
* Fetch scheduled email by messageId (Can retrieve data upto 30 days old)
* @param messageId The messageId of scheduled email (required)
* @param startDate Mandatory if endDate is used. Starting date (YYYY-MM-DD) from which you want to fetch the list. Can be maximum 30 days older tha current date. (optional)
* @param endDate Mandatory if startDate is used. Ending date (YYYY-MM-DD) till which you want to fetch the list. Maximum time period that can be selected is one month. (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call getScheduledEmailByMessageIdAsync(String messageId, LocalDate startDate, LocalDate endDate, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = getScheduledEmailByMessageIdValidateBeforeCall(messageId, startDate, endDate, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for getSmtpReport
* @param limit Number of documents returned per page (optional, default to 10)
* @param offset Index of the first document on the page (optional, default to 0)
* @param startDate Mandatory if endDate is used. Starting date of the report (YYYY-MM-DD) (optional)
* @param endDate Mandatory if startDate is used. Ending date of the report (YYYY-MM-DD) (optional)
* @param days Number of days in the past including today (positive integer). Not compatible with 'startDate' and 'endDate' (optional)
* @param tag Tag of the emails (optional)
* @param sort Sort the results in the ascending/descending order of record creation. Default order is **descending** if `sort` is not passed (optional, default to desc)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call getSmtpReportCall(Long limit, Long offset, String startDate, String endDate, Long days, String tag, String sort, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/smtp/statistics/reports";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
if (limit != null)
localVarQueryParams.addAll(apiClient.parameterToPair("limit", limit));
if (offset != null)
localVarQueryParams.addAll(apiClient.parameterToPair("offset", offset));
if (startDate != null)
localVarQueryParams.addAll(apiClient.parameterToPair("startDate", startDate));
if (endDate != null)
localVarQueryParams.addAll(apiClient.parameterToPair("endDate", endDate));
if (days != null)
localVarQueryParams.addAll(apiClient.parameterToPair("days", days));
if (tag != null)
localVarQueryParams.addAll(apiClient.parameterToPair("tag", tag));
if (sort != null)
localVarQueryParams.addAll(apiClient.parameterToPair("sort", sort));
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call getSmtpReportValidateBeforeCall(Long limit, Long offset, String startDate, String endDate, Long days, String tag, String sort, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Call call = getSmtpReportCall(limit, offset, startDate, endDate, days, tag, sort, progressListener, progressRequestListener);
return call;
}
/**
* Get your transactional email activity aggregated per day
*
* @param limit Number of documents returned per page (optional, default to 10)
* @param offset Index of the first document on the page (optional, default to 0)
* @param startDate Mandatory if endDate is used. Starting date of the report (YYYY-MM-DD) (optional)
* @param endDate Mandatory if startDate is used. Ending date of the report (YYYY-MM-DD) (optional)
* @param days Number of days in the past including today (positive integer). Not compatible with 'startDate' and 'endDate' (optional)
* @param tag Tag of the emails (optional)
* @param sort Sort the results in the ascending/descending order of record creation. Default order is **descending** if `sort` is not passed (optional, default to desc)
* @return GetReports
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public GetReports getSmtpReport(Long limit, Long offset, String startDate, String endDate, Long days, String tag, String sort) throws ApiException {
ApiResponse resp = getSmtpReportWithHttpInfo(limit, offset, startDate, endDate, days, tag, sort);
return resp.getData();
}
/**
* Get your transactional email activity aggregated per day
*
* @param limit Number of documents returned per page (optional, default to 10)
* @param offset Index of the first document on the page (optional, default to 0)
* @param startDate Mandatory if endDate is used. Starting date of the report (YYYY-MM-DD) (optional)
* @param endDate Mandatory if startDate is used. Ending date of the report (YYYY-MM-DD) (optional)
* @param days Number of days in the past including today (positive integer). Not compatible with 'startDate' and 'endDate' (optional)
* @param tag Tag of the emails (optional)
* @param sort Sort the results in the ascending/descending order of record creation. Default order is **descending** if `sort` is not passed (optional, default to desc)
* @return ApiResponse<GetReports>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getSmtpReportWithHttpInfo(Long limit, Long offset, String startDate, String endDate, Long days, String tag, String sort) throws ApiException {
Call call = getSmtpReportValidateBeforeCall(limit, offset, startDate, endDate, days, tag, sort, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get your transactional email activity aggregated per day (asynchronously)
*
* @param limit Number of documents returned per page (optional, default to 10)
* @param offset Index of the first document on the page (optional, default to 0)
* @param startDate Mandatory if endDate is used. Starting date of the report (YYYY-MM-DD) (optional)
* @param endDate Mandatory if startDate is used. Ending date of the report (YYYY-MM-DD) (optional)
* @param days Number of days in the past including today (positive integer). Not compatible with 'startDate' and 'endDate' (optional)
* @param tag Tag of the emails (optional)
* @param sort Sort the results in the ascending/descending order of record creation. Default order is **descending** if `sort` is not passed (optional, default to desc)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call getSmtpReportAsync(Long limit, Long offset, String startDate, String endDate, Long days, String tag, String sort, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = getSmtpReportValidateBeforeCall(limit, offset, startDate, endDate, days, tag, sort, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for getSmtpTemplate
* @param templateId id of the template (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call getSmtpTemplateCall(Long templateId, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/smtp/templates/{templateId}"
.replaceAll("\\{" + "templateId" + "\\}", apiClient.escapeString(templateId.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call getSmtpTemplateValidateBeforeCall(Long templateId, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'templateId' is set
if (templateId == null) {
throw new ApiException("Missing the required parameter 'templateId' when calling getSmtpTemplate(Async)");
}
Call call = getSmtpTemplateCall(templateId, progressListener, progressRequestListener);
return call;
}
/**
* Returns the template information
*
* @param templateId id of the template (required)
* @return GetSmtpTemplateOverview
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public GetSmtpTemplateOverview getSmtpTemplate(Long templateId) throws ApiException {
ApiResponse resp = getSmtpTemplateWithHttpInfo(templateId);
return resp.getData();
}
/**
* Returns the template information
*
* @param templateId id of the template (required)
* @return ApiResponse<GetSmtpTemplateOverview>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getSmtpTemplateWithHttpInfo(Long templateId) throws ApiException {
Call call = getSmtpTemplateValidateBeforeCall(templateId, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Returns the template information (asynchronously)
*
* @param templateId id of the template (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call getSmtpTemplateAsync(Long templateId, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = getSmtpTemplateValidateBeforeCall(templateId, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for getSmtpTemplates
* @param templateStatus Filter on the status of the template. Active = true, inactive = false (optional)
* @param limit Number of documents returned per page (optional, default to 50)
* @param offset Index of the first document in the page (optional, default to 0)
* @param sort Sort the results in the ascending/descending order of record creation. Default order is **descending** if `sort` is not passed (optional, default to desc)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call getSmtpTemplatesCall(Boolean templateStatus, Long limit, Long offset, String sort, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/smtp/templates";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
if (templateStatus != null)
localVarQueryParams.addAll(apiClient.parameterToPair("templateStatus", templateStatus));
if (limit != null)
localVarQueryParams.addAll(apiClient.parameterToPair("limit", limit));
if (offset != null)
localVarQueryParams.addAll(apiClient.parameterToPair("offset", offset));
if (sort != null)
localVarQueryParams.addAll(apiClient.parameterToPair("sort", sort));
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call getSmtpTemplatesValidateBeforeCall(Boolean templateStatus, Long limit, Long offset, String sort, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Call call = getSmtpTemplatesCall(templateStatus, limit, offset, sort, progressListener, progressRequestListener);
return call;
}
/**
* Get the list of email templates
*
* @param templateStatus Filter on the status of the template. Active = true, inactive = false (optional)
* @param limit Number of documents returned per page (optional, default to 50)
* @param offset Index of the first document in the page (optional, default to 0)
* @param sort Sort the results in the ascending/descending order of record creation. Default order is **descending** if `sort` is not passed (optional, default to desc)
* @return GetSmtpTemplates
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public GetSmtpTemplates getSmtpTemplates(Boolean templateStatus, Long limit, Long offset, String sort) throws ApiException {
ApiResponse resp = getSmtpTemplatesWithHttpInfo(templateStatus, limit, offset, sort);
return resp.getData();
}
/**
* Get the list of email templates
*
* @param templateStatus Filter on the status of the template. Active = true, inactive = false (optional)
* @param limit Number of documents returned per page (optional, default to 50)
* @param offset Index of the first document in the page (optional, default to 0)
* @param sort Sort the results in the ascending/descending order of record creation. Default order is **descending** if `sort` is not passed (optional, default to desc)
* @return ApiResponse<GetSmtpTemplates>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getSmtpTemplatesWithHttpInfo(Boolean templateStatus, Long limit, Long offset, String sort) throws ApiException {
Call call = getSmtpTemplatesValidateBeforeCall(templateStatus, limit, offset, sort, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get the list of email templates (asynchronously)
*
* @param templateStatus Filter on the status of the template. Active = true, inactive = false (optional)
* @param limit Number of documents returned per page (optional, default to 50)
* @param offset Index of the first document in the page (optional, default to 0)
* @param sort Sort the results in the ascending/descending order of record creation. Default order is **descending** if `sort` is not passed (optional, default to desc)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call getSmtpTemplatesAsync(Boolean templateStatus, Long limit, Long offset, String sort, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = getSmtpTemplatesValidateBeforeCall(templateStatus, limit, offset, sort, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for getTransacBlockedContacts
* @param startDate Mandatory if endDate is used. Starting date (YYYY-MM-DD) from which you want to fetch the blocked or unsubscribed contacts (optional)
* @param endDate Mandatory if startDate is used. Ending date (YYYY-MM-DD) till which you want to fetch the blocked or unsubscribed contacts (optional)
* @param limit Number of documents returned per page (optional, default to 50)
* @param offset Index of the first document on the page (optional, default to 0)
* @param senders Comma separated list of emails of the senders from which contacts are blocked or unsubscribed (optional)
* @param sort Sort the results in the ascending/descending order of record creation. Default order is **descending** if `sort` is not passed (optional, default to desc)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call getTransacBlockedContactsCall(String startDate, String endDate, Long limit, Long offset, List senders, String sort, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/smtp/blockedContacts";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
if (startDate != null)
localVarQueryParams.addAll(apiClient.parameterToPair("startDate", startDate));
if (endDate != null)
localVarQueryParams.addAll(apiClient.parameterToPair("endDate", endDate));
if (limit != null)
localVarQueryParams.addAll(apiClient.parameterToPair("limit", limit));
if (offset != null)
localVarQueryParams.addAll(apiClient.parameterToPair("offset", offset));
if (senders != null)
localVarCollectionQueryParams.addAll(apiClient.parameterToPairs("csv", "senders", senders));
if (sort != null)
localVarQueryParams.addAll(apiClient.parameterToPair("sort", sort));
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call getTransacBlockedContactsValidateBeforeCall(String startDate, String endDate, Long limit, Long offset, List senders, String sort, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Call call = getTransacBlockedContactsCall(startDate, endDate, limit, offset, senders, sort, progressListener, progressRequestListener);
return call;
}
/**
* Get the list of blocked or unsubscribed transactional contacts
*
* @param startDate Mandatory if endDate is used. Starting date (YYYY-MM-DD) from which you want to fetch the blocked or unsubscribed contacts (optional)
* @param endDate Mandatory if startDate is used. Ending date (YYYY-MM-DD) till which you want to fetch the blocked or unsubscribed contacts (optional)
* @param limit Number of documents returned per page (optional, default to 50)
* @param offset Index of the first document on the page (optional, default to 0)
* @param senders Comma separated list of emails of the senders from which contacts are blocked or unsubscribed (optional)
* @param sort Sort the results in the ascending/descending order of record creation. Default order is **descending** if `sort` is not passed (optional, default to desc)
* @return GetTransacBlockedContacts
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public GetTransacBlockedContacts getTransacBlockedContacts(String startDate, String endDate, Long limit, Long offset, List senders, String sort) throws ApiException {
ApiResponse resp = getTransacBlockedContactsWithHttpInfo(startDate, endDate, limit, offset, senders, sort);
return resp.getData();
}
/**
* Get the list of blocked or unsubscribed transactional contacts
*
* @param startDate Mandatory if endDate is used. Starting date (YYYY-MM-DD) from which you want to fetch the blocked or unsubscribed contacts (optional)
* @param endDate Mandatory if startDate is used. Ending date (YYYY-MM-DD) till which you want to fetch the blocked or unsubscribed contacts (optional)
* @param limit Number of documents returned per page (optional, default to 50)
* @param offset Index of the first document on the page (optional, default to 0)
* @param senders Comma separated list of emails of the senders from which contacts are blocked or unsubscribed (optional)
* @param sort Sort the results in the ascending/descending order of record creation. Default order is **descending** if `sort` is not passed (optional, default to desc)
* @return ApiResponse<GetTransacBlockedContacts>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getTransacBlockedContactsWithHttpInfo(String startDate, String endDate, Long limit, Long offset, List senders, String sort) throws ApiException {
Call call = getTransacBlockedContactsValidateBeforeCall(startDate, endDate, limit, offset, senders, sort, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get the list of blocked or unsubscribed transactional contacts (asynchronously)
*
* @param startDate Mandatory if endDate is used. Starting date (YYYY-MM-DD) from which you want to fetch the blocked or unsubscribed contacts (optional)
* @param endDate Mandatory if startDate is used. Ending date (YYYY-MM-DD) till which you want to fetch the blocked or unsubscribed contacts (optional)
* @param limit Number of documents returned per page (optional, default to 50)
* @param offset Index of the first document on the page (optional, default to 0)
* @param senders Comma separated list of emails of the senders from which contacts are blocked or unsubscribed (optional)
* @param sort Sort the results in the ascending/descending order of record creation. Default order is **descending** if `sort` is not passed (optional, default to desc)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call getTransacBlockedContactsAsync(String startDate, String endDate, Long limit, Long offset, List senders, String sort, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = getTransacBlockedContactsValidateBeforeCall(startDate, endDate, limit, offset, senders, sort, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for getTransacEmailContent
* @param uuid Unique id of the transactional email that has been sent to a particular contact (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call getTransacEmailContentCall(String uuid, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/smtp/emails/{uuid}"
.replaceAll("\\{" + "uuid" + "\\}", apiClient.escapeString(uuid.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call getTransacEmailContentValidateBeforeCall(String uuid, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'uuid' is set
if (uuid == null) {
throw new ApiException("Missing the required parameter 'uuid' when calling getTransacEmailContent(Async)");
}
Call call = getTransacEmailContentCall(uuid, progressListener, progressRequestListener);
return call;
}
/**
* Get the personalized content of a sent transactional email
*
* @param uuid Unique id of the transactional email that has been sent to a particular contact (required)
* @return GetTransacEmailContent
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public GetTransacEmailContent getTransacEmailContent(String uuid) throws ApiException {
ApiResponse resp = getTransacEmailContentWithHttpInfo(uuid);
return resp.getData();
}
/**
* Get the personalized content of a sent transactional email
*
* @param uuid Unique id of the transactional email that has been sent to a particular contact (required)
* @return ApiResponse<GetTransacEmailContent>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getTransacEmailContentWithHttpInfo(String uuid) throws ApiException {
Call call = getTransacEmailContentValidateBeforeCall(uuid, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get the personalized content of a sent transactional email (asynchronously)
*
* @param uuid Unique id of the transactional email that has been sent to a particular contact (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call getTransacEmailContentAsync(String uuid, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = getTransacEmailContentValidateBeforeCall(uuid, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for getTransacEmailsList
* @param email Mandatory if templateId and messageId are not passed in query filters. Email address to which transactional email has been sent. (optional)
* @param templateId Mandatory if email and messageId are not passed in query filters. Id of the template that was used to compose transactional email. (optional)
* @param messageId Mandatory if templateId and email are not passed in query filters. Message ID of the transactional email sent. (optional)
* @param startDate Mandatory if endDate is used. Starting date (YYYY-MM-DD) from which you want to fetch the list. Maximum time period that can be selected is one month. (optional)
* @param endDate Mandatory if startDate is used. Ending date (YYYY-MM-DD) till which you want to fetch the list. Maximum time period that can be selected is one month. (optional)
* @param sort Sort the results in the ascending/descending order of record creation. Default order is **descending** if `sort` is not passed (optional, default to desc)
* @param limit Number of documents returned per page (optional, default to 500)
* @param offset Index of the first document in the page (optional, default to 0)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call getTransacEmailsListCall(String email, Long templateId, String messageId, String startDate, String endDate, String sort, Long limit, Long offset, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/smtp/emails";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
if (email != null)
localVarQueryParams.addAll(apiClient.parameterToPair("email", email));
if (templateId != null)
localVarQueryParams.addAll(apiClient.parameterToPair("templateId", templateId));
if (messageId != null)
localVarQueryParams.addAll(apiClient.parameterToPair("messageId", messageId));
if (startDate != null)
localVarQueryParams.addAll(apiClient.parameterToPair("startDate", startDate));
if (endDate != null)
localVarQueryParams.addAll(apiClient.parameterToPair("endDate", endDate));
if (sort != null)
localVarQueryParams.addAll(apiClient.parameterToPair("sort", sort));
if (limit != null)
localVarQueryParams.addAll(apiClient.parameterToPair("limit", limit));
if (offset != null)
localVarQueryParams.addAll(apiClient.parameterToPair("offset", offset));
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call getTransacEmailsListValidateBeforeCall(String email, Long templateId, String messageId, String startDate, String endDate, String sort, Long limit, Long offset, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Call call = getTransacEmailsListCall(email, templateId, messageId, startDate, endDate, sort, limit, offset, progressListener, progressRequestListener);
return call;
}
/**
* Get the list of transactional emails on the basis of allowed filters
* This endpoint will show the list of emails for past 30 days by default. To retrieve emails before that time, please pass startDate and endDate in query filters.
* @param email Mandatory if templateId and messageId are not passed in query filters. Email address to which transactional email has been sent. (optional)
* @param templateId Mandatory if email and messageId are not passed in query filters. Id of the template that was used to compose transactional email. (optional)
* @param messageId Mandatory if templateId and email are not passed in query filters. Message ID of the transactional email sent. (optional)
* @param startDate Mandatory if endDate is used. Starting date (YYYY-MM-DD) from which you want to fetch the list. Maximum time period that can be selected is one month. (optional)
* @param endDate Mandatory if startDate is used. Ending date (YYYY-MM-DD) till which you want to fetch the list. Maximum time period that can be selected is one month. (optional)
* @param sort Sort the results in the ascending/descending order of record creation. Default order is **descending** if `sort` is not passed (optional, default to desc)
* @param limit Number of documents returned per page (optional, default to 500)
* @param offset Index of the first document in the page (optional, default to 0)
* @return GetTransacEmailsList
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public GetTransacEmailsList getTransacEmailsList(String email, Long templateId, String messageId, String startDate, String endDate, String sort, Long limit, Long offset) throws ApiException {
ApiResponse resp = getTransacEmailsListWithHttpInfo(email, templateId, messageId, startDate, endDate, sort, limit, offset);
return resp.getData();
}
/**
* Get the list of transactional emails on the basis of allowed filters
* This endpoint will show the list of emails for past 30 days by default. To retrieve emails before that time, please pass startDate and endDate in query filters.
* @param email Mandatory if templateId and messageId are not passed in query filters. Email address to which transactional email has been sent. (optional)
* @param templateId Mandatory if email and messageId are not passed in query filters. Id of the template that was used to compose transactional email. (optional)
* @param messageId Mandatory if templateId and email are not passed in query filters. Message ID of the transactional email sent. (optional)
* @param startDate Mandatory if endDate is used. Starting date (YYYY-MM-DD) from which you want to fetch the list. Maximum time period that can be selected is one month. (optional)
* @param endDate Mandatory if startDate is used. Ending date (YYYY-MM-DD) till which you want to fetch the list. Maximum time period that can be selected is one month. (optional)
* @param sort Sort the results in the ascending/descending order of record creation. Default order is **descending** if `sort` is not passed (optional, default to desc)
* @param limit Number of documents returned per page (optional, default to 500)
* @param offset Index of the first document in the page (optional, default to 0)
* @return ApiResponse<GetTransacEmailsList>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getTransacEmailsListWithHttpInfo(String email, Long templateId, String messageId, String startDate, String endDate, String sort, Long limit, Long offset) throws ApiException {
Call call = getTransacEmailsListValidateBeforeCall(email, templateId, messageId, startDate, endDate, sort, limit, offset, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get the list of transactional emails on the basis of allowed filters (asynchronously)
* This endpoint will show the list of emails for past 30 days by default. To retrieve emails before that time, please pass startDate and endDate in query filters.
* @param email Mandatory if templateId and messageId are not passed in query filters. Email address to which transactional email has been sent. (optional)
* @param templateId Mandatory if email and messageId are not passed in query filters. Id of the template that was used to compose transactional email. (optional)
* @param messageId Mandatory if templateId and email are not passed in query filters. Message ID of the transactional email sent. (optional)
* @param startDate Mandatory if endDate is used. Starting date (YYYY-MM-DD) from which you want to fetch the list. Maximum time period that can be selected is one month. (optional)
* @param endDate Mandatory if startDate is used. Ending date (YYYY-MM-DD) till which you want to fetch the list. Maximum time period that can be selected is one month. (optional)
* @param sort Sort the results in the ascending/descending order of record creation. Default order is **descending** if `sort` is not passed (optional, default to desc)
* @param limit Number of documents returned per page (optional, default to 500)
* @param offset Index of the first document in the page (optional, default to 0)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call getTransacEmailsListAsync(String email, Long templateId, String messageId, String startDate, String endDate, String sort, Long limit, Long offset, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = getTransacEmailsListValidateBeforeCall(email, templateId, messageId, startDate, endDate, sort, limit, offset, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for sendTestTemplate
* @param templateId Id of the template (required)
* @param sendTestEmail (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call sendTestTemplateCall(Long templateId, SendTestEmail sendTestEmail, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = sendTestEmail;
// create path and map variables
String localVarPath = "/smtp/templates/{templateId}/sendTest"
.replaceAll("\\{" + "templateId" + "\\}", apiClient.escapeString(templateId.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call sendTestTemplateValidateBeforeCall(Long templateId, SendTestEmail sendTestEmail, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'templateId' is set
if (templateId == null) {
throw new ApiException("Missing the required parameter 'templateId' when calling sendTestTemplate(Async)");
}
// verify the required parameter 'sendTestEmail' is set
if (sendTestEmail == null) {
throw new ApiException("Missing the required parameter 'sendTestEmail' when calling sendTestTemplate(Async)");
}
Call call = sendTestTemplateCall(templateId, sendTestEmail, progressListener, progressRequestListener);
return call;
}
/**
* Send a template to your test list
*
* @param templateId Id of the template (required)
* @param sendTestEmail (required)
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public void sendTestTemplate(Long templateId, SendTestEmail sendTestEmail) throws ApiException {
sendTestTemplateWithHttpInfo(templateId, sendTestEmail);
}
/**
* Send a template to your test list
*
* @param templateId Id of the template (required)
* @param sendTestEmail (required)
* @return ApiResponse<Void>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse sendTestTemplateWithHttpInfo(Long templateId, SendTestEmail sendTestEmail) throws ApiException {
Call call = sendTestTemplateValidateBeforeCall(templateId, sendTestEmail, null, null);
return apiClient.execute(call);
}
/**
* Send a template to your test list (asynchronously)
*
* @param templateId Id of the template (required)
* @param sendTestEmail (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call sendTestTemplateAsync(Long templateId, SendTestEmail sendTestEmail, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = sendTestTemplateValidateBeforeCall(templateId, sendTestEmail, progressListener, progressRequestListener);
apiClient.executeAsync(call, callback);
return call;
}
/**
* Build call for sendTransacEmail
* @param sendSmtpEmail Values to send a transactional email (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call sendTransacEmailCall(SendSmtpEmail sendSmtpEmail, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = sendSmtpEmail;
// create path and map variables
String localVarPath = "/smtp/email";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call sendTransacEmailValidateBeforeCall(SendSmtpEmail sendSmtpEmail, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'sendSmtpEmail' is set
if (sendSmtpEmail == null) {
throw new ApiException("Missing the required parameter 'sendSmtpEmail' when calling sendTransacEmail(Async)");
}
Call call = sendTransacEmailCall(sendSmtpEmail, progressListener, progressRequestListener);
return call;
}
/**
* Send a transactional email
*
* @param sendSmtpEmail Values to send a transactional email (required)
* @return CreateSmtpEmail
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public CreateSmtpEmail sendTransacEmail(SendSmtpEmail sendSmtpEmail) throws ApiException {
ApiResponse resp = sendTransacEmailWithHttpInfo(sendSmtpEmail);
return resp.getData();
}
/**
* Send a transactional email
*
* @param sendSmtpEmail Values to send a transactional email (required)
* @return ApiResponse<CreateSmtpEmail>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse sendTransacEmailWithHttpInfo(SendSmtpEmail sendSmtpEmail) throws ApiException {
Call call = sendTransacEmailValidateBeforeCall(sendSmtpEmail, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Send a transactional email (asynchronously)
*
* @param sendSmtpEmail Values to send a transactional email (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call sendTransacEmailAsync(SendSmtpEmail sendSmtpEmail, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = sendTransacEmailValidateBeforeCall(sendSmtpEmail, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for smtpBlockedContactsEmailDelete
* @param email contact email (urlencoded) to unblock. (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call smtpBlockedContactsEmailDeleteCall(String email, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/smtp/blockedContacts/{email}"
.replaceAll("\\{" + "email" + "\\}", apiClient.escapeString(email.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "api-key", "partner-key" };
return apiClient.buildCall(localVarPath, "DELETE", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private Call smtpBlockedContactsEmailDeleteValidateBeforeCall(String email, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'email' is set
if (email == null) {
throw new ApiException("Missing the required parameter 'email' when calling smtpBlockedContactsEmailDelete(Async)");
}
Call call = smtpBlockedContactsEmailDeleteCall(email, progressListener, progressRequestListener);
return call;
}
/**
* Unblock or resubscribe a transactional contact
*
* @param email contact email (urlencoded) to unblock. (required)
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public void smtpBlockedContactsEmailDelete(String email) throws ApiException {
smtpBlockedContactsEmailDeleteWithHttpInfo(email);
}
/**
* Unblock or resubscribe a transactional contact
*
* @param email contact email (urlencoded) to unblock. (required)
* @return ApiResponse<Void>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse smtpBlockedContactsEmailDeleteWithHttpInfo(String email) throws ApiException {
Call call = smtpBlockedContactsEmailDeleteValidateBeforeCall(email, null, null);
return apiClient.execute(call);
}
/**
* Unblock or resubscribe a transactional contact (asynchronously)
*
* @param email contact email (urlencoded) to unblock. (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public Call smtpBlockedContactsEmailDeleteAsync(String email, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
Call call = smtpBlockedContactsEmailDeleteValidateBeforeCall(email, progressListener, progressRequestListener);
apiClient.executeAsync(call, callback);
return call;
}
/**
* Build call for smtpLogMessageIdDelete
* @param messageId MessageId of the transactional log to delete (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public Call smtpLogMessageIdDeleteCall(String messageId, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/smtp/log/{messageId}"
.replaceAll("\\{" + "messageId" + "\\}", apiClient.escapeString(messageId.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map