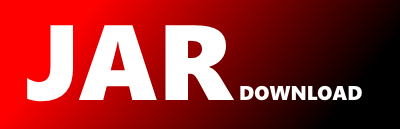
sibModel.ConversationsMessage Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sib-api-v3-sdk Show documentation
Show all versions of sib-api-v3-sdk Show documentation
SendinBlue's API v3 Java Library
The newest version!
/*
* SendinBlue API
* SendinBlue provide a RESTFul API that can be used with any languages. With this API, you will be able to : - Manage your campaigns and get the statistics - Manage your contacts - Send transactional Emails and SMS - and much more... You can download our wrappers at https://github.com/orgs/sendinblue **Possible responses** | Code | Message | | :-------------: | ------------- | | 200 | OK. Successful Request | | 201 | OK. Successful Creation | | 202 | OK. Request accepted | | 204 | OK. Successful Update/Deletion | | 400 | Error. Bad Request | | 401 | Error. Authentication Needed | | 402 | Error. Not enough credit, plan upgrade needed | | 403 | Error. Permission denied | | 404 | Error. Object does not exist | | 405 | Error. Method not allowed | | 406 | Error. Not Acceptable |
*
* OpenAPI spec version: 3.0.0
* Contact: [email protected]
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package sibModel;
import org.apache.commons.lang3.ObjectUtils;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import sibModel.ConversationsMessageFile;
/**
* a Conversations message
*/
@ApiModel(description = "a Conversations message")
@javax.annotation.Generated(value = "io.swagger.codegen.languages.JavaClientCodegen", date = "2023-02-28T15:16:18.937+05:30")
public class ConversationsMessage {
@SerializedName("id")
private String id = null;
/**
* `\"agent\"` for agents’ messages, `\"visitor\"` for visitors’ messages.
*/
@JsonAdapter(TypeEnum.Adapter.class)
public enum TypeEnum {
AGENT("agent"),
VISITOR("visitor");
private String value;
TypeEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static TypeEnum fromValue(String text) {
for (TypeEnum b : TypeEnum.values()) {
if (String.valueOf(b.value).equals(text)) {
return b;
}
}
return null;
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final TypeEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public TypeEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return TypeEnum.fromValue(String.valueOf(value));
}
}
}
@SerializedName("type")
private TypeEnum type = null;
@SerializedName("text")
private String text = null;
@SerializedName("visitorId")
private String visitorId = null;
@SerializedName("agentId")
private String agentId = null;
@SerializedName("agentName")
private String agentName = null;
@SerializedName("createdAt")
private Long createdAt = null;
@SerializedName("isPushed")
private Boolean isPushed = null;
@SerializedName("receivedFrom")
private String receivedFrom = null;
@SerializedName("file")
private ConversationsMessageFile file = null;
public ConversationsMessage id(String id) {
this.id = id;
return this;
}
/**
* Message ID. It can be used for further manipulations with the message.
* @return id
**/
@ApiModelProperty(example = "eYBEm3gq3zc5ayE2g", value = "Message ID. It can be used for further manipulations with the message.")
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public ConversationsMessage type(TypeEnum type) {
this.type = type;
return this;
}
/**
* `\"agent\"` for agents’ messages, `\"visitor\"` for visitors’ messages.
* @return type
**/
@ApiModelProperty(example = "agent", value = "`\"agent\"` for agents’ messages, `\"visitor\"` for visitors’ messages.")
public TypeEnum getType() {
return type;
}
public void setType(TypeEnum type) {
this.type = type;
}
public ConversationsMessage text(String text) {
this.text = text;
return this;
}
/**
* Message text or name of the attached file
* @return text
**/
@ApiModelProperty(example = "Good morning! How can I help you?", value = "Message text or name of the attached file")
public String getText() {
return text;
}
public void setText(String text) {
this.text = text;
}
public ConversationsMessage visitorId(String visitorId) {
this.visitorId = visitorId;
return this;
}
/**
* visitor’s ID
* @return visitorId
**/
@ApiModelProperty(example = "kZMvWhf8npAu3H6qd57w2Hv6nh6rnxvg", value = "visitor’s ID")
public String getVisitorId() {
return visitorId;
}
public void setVisitorId(String visitorId) {
this.visitorId = visitorId;
}
public ConversationsMessage agentId(String agentId) {
this.agentId = agentId;
return this;
}
/**
* ID of the agent on whose behalf the message was sent (only in messages sent by an agent).
* @return agentId
**/
@ApiModelProperty(example = "d9nKoegKSjmCtyK78", value = "ID of the agent on whose behalf the message was sent (only in messages sent by an agent).")
public String getAgentId() {
return agentId;
}
public void setAgentId(String agentId) {
this.agentId = agentId;
}
public ConversationsMessage agentName(String agentName) {
this.agentName = agentName;
return this;
}
/**
* Agent’s name as displayed to the visitor. Only in the messages sent by an agent.
* @return agentName
**/
@ApiModelProperty(example = "Liz", value = "Agent’s name as displayed to the visitor. Only in the messages sent by an agent.")
public String getAgentName() {
return agentName;
}
public void setAgentName(String agentName) {
this.agentName = agentName;
}
public ConversationsMessage createdAt(Long createdAt) {
this.createdAt = createdAt;
return this;
}
/**
* Timestamp in milliseconds.
* minimum: 0
* @return createdAt
**/
@ApiModelProperty(example = "1470222622433", value = "Timestamp in milliseconds.")
public Long getCreatedAt() {
return createdAt;
}
public void setCreatedAt(Long createdAt) {
this.createdAt = createdAt;
}
public ConversationsMessage isPushed(Boolean isPushed) {
this.isPushed = isPushed;
return this;
}
/**
* `true` for pushed messages
* @return isPushed
**/
@ApiModelProperty(example = "true", value = "`true` for pushed messages")
public Boolean isIsPushed() {
return isPushed;
}
public void setIsPushed(Boolean isPushed) {
this.isPushed = isPushed;
}
public ConversationsMessage receivedFrom(String receivedFrom) {
this.receivedFrom = receivedFrom;
return this;
}
/**
* In two-way integrations, messages sent via REST API can be marked with receivedFrom property and then filtered out when received in a webhook to avoid infinite loop.
* @return receivedFrom
**/
@ApiModelProperty(example = "SuperAwesomeHelpdesk", value = "In two-way integrations, messages sent via REST API can be marked with receivedFrom property and then filtered out when received in a webhook to avoid infinite loop.")
public String getReceivedFrom() {
return receivedFrom;
}
public void setReceivedFrom(String receivedFrom) {
this.receivedFrom = receivedFrom;
}
public ConversationsMessage file(ConversationsMessageFile file) {
this.file = file;
return this;
}
/**
* Get file
* @return file
**/
@ApiModelProperty(value = "")
public ConversationsMessageFile getFile() {
return file;
}
public void setFile(ConversationsMessageFile file) {
this.file = file;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
ConversationsMessage conversationsMessage = (ConversationsMessage) o;
return ObjectUtils.equals(this.id, conversationsMessage.id) &&
ObjectUtils.equals(this.type, conversationsMessage.type) &&
ObjectUtils.equals(this.text, conversationsMessage.text) &&
ObjectUtils.equals(this.visitorId, conversationsMessage.visitorId) &&
ObjectUtils.equals(this.agentId, conversationsMessage.agentId) &&
ObjectUtils.equals(this.agentName, conversationsMessage.agentName) &&
ObjectUtils.equals(this.createdAt, conversationsMessage.createdAt) &&
ObjectUtils.equals(this.isPushed, conversationsMessage.isPushed) &&
ObjectUtils.equals(this.receivedFrom, conversationsMessage.receivedFrom) &&
ObjectUtils.equals(this.file, conversationsMessage.file);
}
@Override
public int hashCode() {
return ObjectUtils.hashCodeMulti(id, type, text, visitorId, agentId, agentName, createdAt, isPushed, receivedFrom, file);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class ConversationsMessage {\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" type: ").append(toIndentedString(type)).append("\n");
sb.append(" text: ").append(toIndentedString(text)).append("\n");
sb.append(" visitorId: ").append(toIndentedString(visitorId)).append("\n");
sb.append(" agentId: ").append(toIndentedString(agentId)).append("\n");
sb.append(" agentName: ").append(toIndentedString(agentName)).append("\n");
sb.append(" createdAt: ").append(toIndentedString(createdAt)).append("\n");
sb.append(" isPushed: ").append(toIndentedString(isPushed)).append("\n");
sb.append(" receivedFrom: ").append(toIndentedString(receivedFrom)).append("\n");
sb.append(" file: ").append(toIndentedString(file)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy