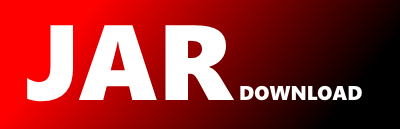
sibModel.CreateEmailCampaign Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sib-api-v3-sdk Show documentation
Show all versions of sib-api-v3-sdk Show documentation
SendinBlue's API v3 Java Library
The newest version!
/*
* SendinBlue API
* SendinBlue provide a RESTFul API that can be used with any languages. With this API, you will be able to : - Manage your campaigns and get the statistics - Manage your contacts - Send transactional Emails and SMS - and much more... You can download our wrappers at https://github.com/orgs/sendinblue **Possible responses** | Code | Message | | :-------------: | ------------- | | 200 | OK. Successful Request | | 201 | OK. Successful Creation | | 202 | OK. Request accepted | | 204 | OK. Successful Update/Deletion | | 400 | Error. Bad Request | | 401 | Error. Authentication Needed | | 402 | Error. Not enough credit, plan upgrade needed | | 403 | Error. Permission denied | | 404 | Error. Object does not exist | | 405 | Error. Method not allowed | | 406 | Error. Not Acceptable |
*
* OpenAPI spec version: 3.0.0
* Contact: [email protected]
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package sibModel;
import org.apache.commons.lang3.ObjectUtils;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import sibModel.CreateEmailCampaignRecipients;
import sibModel.CreateEmailCampaignSender;
/**
* CreateEmailCampaign
*/
@javax.annotation.Generated(value = "io.swagger.codegen.languages.JavaClientCodegen", date = "2023-02-28T15:16:18.937+05:30")
public class CreateEmailCampaign {
@SerializedName("tag")
private String tag = null;
@SerializedName("sender")
private CreateEmailCampaignSender sender = null;
@SerializedName("name")
private String name = null;
@SerializedName("htmlContent")
private String htmlContent = null;
@SerializedName("htmlUrl")
private String htmlUrl = null;
@SerializedName("templateId")
private Long templateId = null;
@SerializedName("scheduledAt")
private String scheduledAt = null;
@SerializedName("subject")
private String subject = null;
@SerializedName("replyTo")
private String replyTo = null;
@SerializedName("toField")
private String toField = null;
@SerializedName("recipients")
private CreateEmailCampaignRecipients recipients = null;
@SerializedName("attachmentUrl")
private String attachmentUrl = null;
@SerializedName("inlineImageActivation")
private Boolean inlineImageActivation = false;
@SerializedName("mirrorActive")
private Boolean mirrorActive = null;
@SerializedName("footer")
private String footer = null;
@SerializedName("header")
private String header = null;
@SerializedName("utmCampaign")
private String utmCampaign = null;
@SerializedName("params")
private Object params = null;
@SerializedName("sendAtBestTime")
private Boolean sendAtBestTime = false;
@SerializedName("abTesting")
private Boolean abTesting = false;
@SerializedName("subjectA")
private String subjectA = null;
@SerializedName("subjectB")
private String subjectB = null;
@SerializedName("splitRule")
private Long splitRule = null;
/**
* Choose the metrics that will determinate the winning version. Mandatory if 'splitRule' >= 1 and < 50. If splitRule = 50, 'winnerCriteria' is ignored if passed
*/
@JsonAdapter(WinnerCriteriaEnum.Adapter.class)
public enum WinnerCriteriaEnum {
OPEN("open"),
CLICK("click");
private String value;
WinnerCriteriaEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static WinnerCriteriaEnum fromValue(String text) {
for (WinnerCriteriaEnum b : WinnerCriteriaEnum.values()) {
if (String.valueOf(b.value).equals(text)) {
return b;
}
}
return null;
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final WinnerCriteriaEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public WinnerCriteriaEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return WinnerCriteriaEnum.fromValue(String.valueOf(value));
}
}
}
@SerializedName("winnerCriteria")
private WinnerCriteriaEnum winnerCriteria = null;
@SerializedName("winnerDelay")
private Long winnerDelay = null;
@SerializedName("ipWarmupEnable")
private Boolean ipWarmupEnable = false;
@SerializedName("initialQuota")
private Long initialQuota = null;
@SerializedName("increaseRate")
private Long increaseRate = null;
@SerializedName("unsubscriptionPageId")
private String unsubscriptionPageId = null;
@SerializedName("updateFormId")
private String updateFormId = null;
public CreateEmailCampaign tag(String tag) {
this.tag = tag;
return this;
}
/**
* Tag of the campaign
* @return tag
**/
@ApiModelProperty(example = "Newsletter", value = "Tag of the campaign")
public String getTag() {
return tag;
}
public void setTag(String tag) {
this.tag = tag;
}
public CreateEmailCampaign sender(CreateEmailCampaignSender sender) {
this.sender = sender;
return this;
}
/**
* Get sender
* @return sender
**/
@ApiModelProperty(required = true, value = "")
public CreateEmailCampaignSender getSender() {
return sender;
}
public void setSender(CreateEmailCampaignSender sender) {
this.sender = sender;
}
public CreateEmailCampaign name(String name) {
this.name = name;
return this;
}
/**
* Name of the campaign
* @return name
**/
@ApiModelProperty(example = "Newsletter - May 2017", required = true, value = "Name of the campaign")
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public CreateEmailCampaign htmlContent(String htmlContent) {
this.htmlContent = htmlContent;
return this;
}
/**
* Mandatory if htmlUrl and templateId are empty. Body of the message (HTML)
* @return htmlContent
**/
@ApiModelProperty(example = " Confirm you email
Please confirm your email address by clicking on the link below
", value = "Mandatory if htmlUrl and templateId are empty. Body of the message (HTML)")
public String getHtmlContent() {
return htmlContent;
}
public void setHtmlContent(String htmlContent) {
this.htmlContent = htmlContent;
}
public CreateEmailCampaign htmlUrl(String htmlUrl) {
this.htmlUrl = htmlUrl;
return this;
}
/**
* Mandatory if htmlContent and templateId are empty. Url to the message (HTML)
* @return htmlUrl
**/
@ApiModelProperty(example = "https://html.domain.com", value = "Mandatory if htmlContent and templateId are empty. Url to the message (HTML)")
public String getHtmlUrl() {
return htmlUrl;
}
public void setHtmlUrl(String htmlUrl) {
this.htmlUrl = htmlUrl;
}
public CreateEmailCampaign templateId(Long templateId) {
this.templateId = templateId;
return this;
}
/**
* Mandatory if htmlContent and htmlUrl are empty. Id of the transactional email template with status 'active'. Used to copy only its content fetched from htmlContent/htmlUrl to an email campaign for RSS feature.
* @return templateId
**/
@ApiModelProperty(value = "Mandatory if htmlContent and htmlUrl are empty. Id of the transactional email template with status 'active'. Used to copy only its content fetched from htmlContent/htmlUrl to an email campaign for RSS feature.")
public Long getTemplateId() {
return templateId;
}
public void setTemplateId(Long templateId) {
this.templateId = templateId;
}
public CreateEmailCampaign scheduledAt(String scheduledAt) {
this.scheduledAt = scheduledAt;
return this;
}
/**
* Sending UTC date-time (YYYY-MM-DDTHH:mm:ss.SSSZ). Prefer to pass your timezone in date-time format for accurate result. If sendAtBestTime is set to true, your campaign will be sent according to the date passed (ignoring the time part).
* @return scheduledAt
**/
@ApiModelProperty(example = "2017-06-01T12:30:00+02:00", value = "Sending UTC date-time (YYYY-MM-DDTHH:mm:ss.SSSZ). Prefer to pass your timezone in date-time format for accurate result. If sendAtBestTime is set to true, your campaign will be sent according to the date passed (ignoring the time part).")
public String getScheduledAt() {
return scheduledAt;
}
public void setScheduledAt(String scheduledAt) {
this.scheduledAt = scheduledAt;
}
public CreateEmailCampaign subject(String subject) {
this.subject = subject;
return this;
}
/**
* Subject of the campaign. Mandatory if abTesting is false. Ignored if abTesting is true.
* @return subject
**/
@ApiModelProperty(example = "Discover the New Collection !", value = "Subject of the campaign. Mandatory if abTesting is false. Ignored if abTesting is true.")
public String getSubject() {
return subject;
}
public void setSubject(String subject) {
this.subject = subject;
}
public CreateEmailCampaign replyTo(String replyTo) {
this.replyTo = replyTo;
return this;
}
/**
* Email on which the campaign recipients will be able to reply to
* @return replyTo
**/
@ApiModelProperty(example = "[email protected]", value = "Email on which the campaign recipients will be able to reply to")
public String getReplyTo() {
return replyTo;
}
public void setReplyTo(String replyTo) {
this.replyTo = replyTo;
}
public CreateEmailCampaign toField(String toField) {
this.toField = toField;
return this;
}
/**
* To personalize the «To» Field. If you want to include the first name and last name of your recipient, add {FNAME} {LNAME}. These contact attributes must already exist in your SendinBlue account. If input parameter 'params' used please use {{contact.FNAME}} {{contact.LNAME}} for personalization
* @return toField
**/
@ApiModelProperty(example = "{FNAME} {LNAME}", value = "To personalize the «To» Field. If you want to include the first name and last name of your recipient, add {FNAME} {LNAME}. These contact attributes must already exist in your SendinBlue account. If input parameter 'params' used please use {{contact.FNAME}} {{contact.LNAME}} for personalization")
public String getToField() {
return toField;
}
public void setToField(String toField) {
this.toField = toField;
}
public CreateEmailCampaign recipients(CreateEmailCampaignRecipients recipients) {
this.recipients = recipients;
return this;
}
/**
* Get recipients
* @return recipients
**/
@ApiModelProperty(value = "")
public CreateEmailCampaignRecipients getRecipients() {
return recipients;
}
public void setRecipients(CreateEmailCampaignRecipients recipients) {
this.recipients = recipients;
}
public CreateEmailCampaign attachmentUrl(String attachmentUrl) {
this.attachmentUrl = attachmentUrl;
return this;
}
/**
* Absolute url of the attachment (no local file). Extension allowed: xlsx, xls, ods, docx, docm, doc, csv, pdf, txt, gif, jpg, jpeg, png, tif, tiff, rtf, bmp, cgm, css, shtml, html, htm, zip, xml, ppt, pptx, tar, ez, ics, mobi, msg, pub and eps
* @return attachmentUrl
**/
@ApiModelProperty(example = "https://attachment.domain.com", value = "Absolute url of the attachment (no local file). Extension allowed: xlsx, xls, ods, docx, docm, doc, csv, pdf, txt, gif, jpg, jpeg, png, tif, tiff, rtf, bmp, cgm, css, shtml, html, htm, zip, xml, ppt, pptx, tar, ez, ics, mobi, msg, pub and eps")
public String getAttachmentUrl() {
return attachmentUrl;
}
public void setAttachmentUrl(String attachmentUrl) {
this.attachmentUrl = attachmentUrl;
}
public CreateEmailCampaign inlineImageActivation(Boolean inlineImageActivation) {
this.inlineImageActivation = inlineImageActivation;
return this;
}
/**
* Use true to embedded the images in your email. Final size of the email should be less than 4MB. Campaigns with embedded images can not be sent to more than 5000 contacts
* @return inlineImageActivation
**/
@ApiModelProperty(example = "true", value = "Use true to embedded the images in your email. Final size of the email should be less than 4MB. Campaigns with embedded images can not be sent to more than 5000 contacts")
public Boolean isInlineImageActivation() {
return inlineImageActivation;
}
public void setInlineImageActivation(Boolean inlineImageActivation) {
this.inlineImageActivation = inlineImageActivation;
}
public CreateEmailCampaign mirrorActive(Boolean mirrorActive) {
this.mirrorActive = mirrorActive;
return this;
}
/**
* Use true to enable the mirror link
* @return mirrorActive
**/
@ApiModelProperty(example = "true", value = "Use true to enable the mirror link")
public Boolean isMirrorActive() {
return mirrorActive;
}
public void setMirrorActive(Boolean mirrorActive) {
this.mirrorActive = mirrorActive;
}
public CreateEmailCampaign footer(String footer) {
this.footer = footer;
return this;
}
/**
* Footer of the email campaign
* @return footer
**/
@ApiModelProperty(example = "[DEFAULT_FOOTER]", value = "Footer of the email campaign")
public String getFooter() {
return footer;
}
public void setFooter(String footer) {
this.footer = footer;
}
public CreateEmailCampaign header(String header) {
this.header = header;
return this;
}
/**
* Header of the email campaign
* @return header
**/
@ApiModelProperty(example = "[DEFAULT_HEADER]", value = "Header of the email campaign")
public String getHeader() {
return header;
}
public void setHeader(String header) {
this.header = header;
}
public CreateEmailCampaign utmCampaign(String utmCampaign) {
this.utmCampaign = utmCampaign;
return this;
}
/**
* Customize the utm_campaign value. If this field is empty, the campaign name will be used. Only alphanumeric characters and spaces are allowed
* @return utmCampaign
**/
@ApiModelProperty(example = "NL_05_2017", value = "Customize the utm_campaign value. If this field is empty, the campaign name will be used. Only alphanumeric characters and spaces are allowed")
public String getUtmCampaign() {
return utmCampaign;
}
public void setUtmCampaign(String utmCampaign) {
this.utmCampaign = utmCampaign;
}
public CreateEmailCampaign params(Object params) {
this.params = params;
return this;
}
/**
* Pass the set of attributes to customize the type classic campaign. For example, {\"FNAME\":\"Joe\", \"LNAME\":\"Doe\"}. Only available if 'type' is 'classic'. It's considered only if campaign is in New Template Language format. The New Template Language is dependent on the values of 'subject', 'htmlContent/htmlUrl', 'sender.name' & 'toField'
* @return params
**/
@ApiModelProperty(example = "{\"FNAME\":\"Joe\",\"LNAME\":\"Doe\"}", value = "Pass the set of attributes to customize the type classic campaign. For example, {\"FNAME\":\"Joe\", \"LNAME\":\"Doe\"}. Only available if 'type' is 'classic'. It's considered only if campaign is in New Template Language format. The New Template Language is dependent on the values of 'subject', 'htmlContent/htmlUrl', 'sender.name' & 'toField'")
public Object getParams() {
return params;
}
public void setParams(Object params) {
this.params = params;
}
public CreateEmailCampaign sendAtBestTime(Boolean sendAtBestTime) {
this.sendAtBestTime = sendAtBestTime;
return this;
}
/**
* Set this to true if you want to send your campaign at best time.
* @return sendAtBestTime
**/
@ApiModelProperty(example = "true", value = "Set this to true if you want to send your campaign at best time.")
public Boolean isSendAtBestTime() {
return sendAtBestTime;
}
public void setSendAtBestTime(Boolean sendAtBestTime) {
this.sendAtBestTime = sendAtBestTime;
}
public CreateEmailCampaign abTesting(Boolean abTesting) {
this.abTesting = abTesting;
return this;
}
/**
* Status of A/B Test. abTesting = false means it is disabled, & abTesting = true means it is enabled. 'subjectA', 'subjectB', 'splitRule', 'winnerCriteria' & 'winnerDelay' will be considered when abTesting is set to true. 'subjectA' & 'subjectB' are mandatory together & 'subject' if passed is ignored. Can be set to true only if 'sendAtBestTime' is 'false'. You will be able to set up two subject lines for your campaign and send them to a random sample of your total recipients. Half of the test group will receive version A, and the other half will receive version B
* @return abTesting
**/
@ApiModelProperty(example = "true", value = "Status of A/B Test. abTesting = false means it is disabled, & abTesting = true means it is enabled. 'subjectA', 'subjectB', 'splitRule', 'winnerCriteria' & 'winnerDelay' will be considered when abTesting is set to true. 'subjectA' & 'subjectB' are mandatory together & 'subject' if passed is ignored. Can be set to true only if 'sendAtBestTime' is 'false'. You will be able to set up two subject lines for your campaign and send them to a random sample of your total recipients. Half of the test group will receive version A, and the other half will receive version B")
public Boolean isAbTesting() {
return abTesting;
}
public void setAbTesting(Boolean abTesting) {
this.abTesting = abTesting;
}
public CreateEmailCampaign subjectA(String subjectA) {
this.subjectA = subjectA;
return this;
}
/**
* Subject A of the campaign. Mandatory if abTesting = true. subjectA & subjectB should have unique value
* @return subjectA
**/
@ApiModelProperty(example = "Discover the New Collection!", value = "Subject A of the campaign. Mandatory if abTesting = true. subjectA & subjectB should have unique value")
public String getSubjectA() {
return subjectA;
}
public void setSubjectA(String subjectA) {
this.subjectA = subjectA;
}
public CreateEmailCampaign subjectB(String subjectB) {
this.subjectB = subjectB;
return this;
}
/**
* Subject B of the campaign. Mandatory if abTesting = true. subjectA & subjectB should have unique value
* @return subjectB
**/
@ApiModelProperty(example = "Want to discover the New Collection?", value = "Subject B of the campaign. Mandatory if abTesting = true. subjectA & subjectB should have unique value")
public String getSubjectB() {
return subjectB;
}
public void setSubjectB(String subjectB) {
this.subjectB = subjectB;
}
public CreateEmailCampaign splitRule(Long splitRule) {
this.splitRule = splitRule;
return this;
}
/**
* Add the size of your test groups. Mandatory if abTesting = true & 'recipients' is passed. We'll send version A and B to a random sample of recipients, and then the winning version to everyone else
* minimum: 1
* maximum: 50
* @return splitRule
**/
@ApiModelProperty(example = "50", value = "Add the size of your test groups. Mandatory if abTesting = true & 'recipients' is passed. We'll send version A and B to a random sample of recipients, and then the winning version to everyone else")
public Long getSplitRule() {
return splitRule;
}
public void setSplitRule(Long splitRule) {
this.splitRule = splitRule;
}
public CreateEmailCampaign winnerCriteria(WinnerCriteriaEnum winnerCriteria) {
this.winnerCriteria = winnerCriteria;
return this;
}
/**
* Choose the metrics that will determinate the winning version. Mandatory if 'splitRule' >= 1 and < 50. If splitRule = 50, 'winnerCriteria' is ignored if passed
* @return winnerCriteria
**/
@ApiModelProperty(example = "open", value = "Choose the metrics that will determinate the winning version. Mandatory if 'splitRule' >= 1 and < 50. If splitRule = 50, 'winnerCriteria' is ignored if passed")
public WinnerCriteriaEnum getWinnerCriteria() {
return winnerCriteria;
}
public void setWinnerCriteria(WinnerCriteriaEnum winnerCriteria) {
this.winnerCriteria = winnerCriteria;
}
public CreateEmailCampaign winnerDelay(Long winnerDelay) {
this.winnerDelay = winnerDelay;
return this;
}
/**
* Choose the duration of the test in hours. Maximum is 7 days, pass 24*7 = 168 hours. The winning version will be sent at the end of the test. Mandatory if 'splitRule' >= 1 and < 50. If splitRule = 50, 'winnerDelay' is ignored if passed
* minimum: 1
* maximum: 168
* @return winnerDelay
**/
@ApiModelProperty(example = "50", value = "Choose the duration of the test in hours. Maximum is 7 days, pass 24*7 = 168 hours. The winning version will be sent at the end of the test. Mandatory if 'splitRule' >= 1 and < 50. If splitRule = 50, 'winnerDelay' is ignored if passed")
public Long getWinnerDelay() {
return winnerDelay;
}
public void setWinnerDelay(Long winnerDelay) {
this.winnerDelay = winnerDelay;
}
public CreateEmailCampaign ipWarmupEnable(Boolean ipWarmupEnable) {
this.ipWarmupEnable = ipWarmupEnable;
return this;
}
/**
* Available for dedicated ip clients. Set this to true if you wish to warm up your ip.
* @return ipWarmupEnable
**/
@ApiModelProperty(example = "true", value = "Available for dedicated ip clients. Set this to true if you wish to warm up your ip.")
public Boolean isIpWarmupEnable() {
return ipWarmupEnable;
}
public void setIpWarmupEnable(Boolean ipWarmupEnable) {
this.ipWarmupEnable = ipWarmupEnable;
}
public CreateEmailCampaign initialQuota(Long initialQuota) {
this.initialQuota = initialQuota;
return this;
}
/**
* Mandatory if ipWarmupEnable is set to true. Set an initial quota greater than 1 for warming up your ip. We recommend you set a value of 3000.
* @return initialQuota
**/
@ApiModelProperty(example = "3000", value = "Mandatory if ipWarmupEnable is set to true. Set an initial quota greater than 1 for warming up your ip. We recommend you set a value of 3000.")
public Long getInitialQuota() {
return initialQuota;
}
public void setInitialQuota(Long initialQuota) {
this.initialQuota = initialQuota;
}
public CreateEmailCampaign increaseRate(Long increaseRate) {
this.increaseRate = increaseRate;
return this;
}
/**
* Mandatory if ipWarmupEnable is set to true. Set a percentage increase rate for warming up your ip. We recommend you set the increase rate to 30% per day. If you want to send the same number of emails every day, set the daily increase value to 0%.
* minimum: 0
* maximum: 100
* @return increaseRate
**/
@ApiModelProperty(example = "70", value = "Mandatory if ipWarmupEnable is set to true. Set a percentage increase rate for warming up your ip. We recommend you set the increase rate to 30% per day. If you want to send the same number of emails every day, set the daily increase value to 0%.")
public Long getIncreaseRate() {
return increaseRate;
}
public void setIncreaseRate(Long increaseRate) {
this.increaseRate = increaseRate;
}
public CreateEmailCampaign unsubscriptionPageId(String unsubscriptionPageId) {
this.unsubscriptionPageId = unsubscriptionPageId;
return this;
}
/**
* Enter an unsubscription page id. The page id is a 24 digit alphanumeric id that can be found in the URL when editing the page. If not entered, then the default unsubscription page will be used.
* @return unsubscriptionPageId
**/
@ApiModelProperty(example = "62cbb7fabbe85021021aac52", value = "Enter an unsubscription page id. The page id is a 24 digit alphanumeric id that can be found in the URL when editing the page. If not entered, then the default unsubscription page will be used.")
public String getUnsubscriptionPageId() {
return unsubscriptionPageId;
}
public void setUnsubscriptionPageId(String unsubscriptionPageId) {
this.unsubscriptionPageId = unsubscriptionPageId;
}
public CreateEmailCampaign updateFormId(String updateFormId) {
this.updateFormId = updateFormId;
return this;
}
/**
* Mandatory if templateId is used containing the {{ update_profile }} tag. Enter an update profile form id. The form id is a 24 digit alphanumeric id that can be found in the URL when editing the form. If not entered, then the default update profile form will be used.
* @return updateFormId
**/
@ApiModelProperty(example = "6313436b9ad40e23b371d095", value = "Mandatory if templateId is used containing the {{ update_profile }} tag. Enter an update profile form id. The form id is a 24 digit alphanumeric id that can be found in the URL when editing the form. If not entered, then the default update profile form will be used.")
public String getUpdateFormId() {
return updateFormId;
}
public void setUpdateFormId(String updateFormId) {
this.updateFormId = updateFormId;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
CreateEmailCampaign createEmailCampaign = (CreateEmailCampaign) o;
return ObjectUtils.equals(this.tag, createEmailCampaign.tag) &&
ObjectUtils.equals(this.sender, createEmailCampaign.sender) &&
ObjectUtils.equals(this.name, createEmailCampaign.name) &&
ObjectUtils.equals(this.htmlContent, createEmailCampaign.htmlContent) &&
ObjectUtils.equals(this.htmlUrl, createEmailCampaign.htmlUrl) &&
ObjectUtils.equals(this.templateId, createEmailCampaign.templateId) &&
ObjectUtils.equals(this.scheduledAt, createEmailCampaign.scheduledAt) &&
ObjectUtils.equals(this.subject, createEmailCampaign.subject) &&
ObjectUtils.equals(this.replyTo, createEmailCampaign.replyTo) &&
ObjectUtils.equals(this.toField, createEmailCampaign.toField) &&
ObjectUtils.equals(this.recipients, createEmailCampaign.recipients) &&
ObjectUtils.equals(this.attachmentUrl, createEmailCampaign.attachmentUrl) &&
ObjectUtils.equals(this.inlineImageActivation, createEmailCampaign.inlineImageActivation) &&
ObjectUtils.equals(this.mirrorActive, createEmailCampaign.mirrorActive) &&
ObjectUtils.equals(this.footer, createEmailCampaign.footer) &&
ObjectUtils.equals(this.header, createEmailCampaign.header) &&
ObjectUtils.equals(this.utmCampaign, createEmailCampaign.utmCampaign) &&
ObjectUtils.equals(this.params, createEmailCampaign.params) &&
ObjectUtils.equals(this.sendAtBestTime, createEmailCampaign.sendAtBestTime) &&
ObjectUtils.equals(this.abTesting, createEmailCampaign.abTesting) &&
ObjectUtils.equals(this.subjectA, createEmailCampaign.subjectA) &&
ObjectUtils.equals(this.subjectB, createEmailCampaign.subjectB) &&
ObjectUtils.equals(this.splitRule, createEmailCampaign.splitRule) &&
ObjectUtils.equals(this.winnerCriteria, createEmailCampaign.winnerCriteria) &&
ObjectUtils.equals(this.winnerDelay, createEmailCampaign.winnerDelay) &&
ObjectUtils.equals(this.ipWarmupEnable, createEmailCampaign.ipWarmupEnable) &&
ObjectUtils.equals(this.initialQuota, createEmailCampaign.initialQuota) &&
ObjectUtils.equals(this.increaseRate, createEmailCampaign.increaseRate) &&
ObjectUtils.equals(this.unsubscriptionPageId, createEmailCampaign.unsubscriptionPageId) &&
ObjectUtils.equals(this.updateFormId, createEmailCampaign.updateFormId);
}
@Override
public int hashCode() {
return ObjectUtils.hashCodeMulti(tag, sender, name, htmlContent, htmlUrl, templateId, scheduledAt, subject, replyTo, toField, recipients, attachmentUrl, inlineImageActivation, mirrorActive, footer, header, utmCampaign, params, sendAtBestTime, abTesting, subjectA, subjectB, splitRule, winnerCriteria, winnerDelay, ipWarmupEnable, initialQuota, increaseRate, unsubscriptionPageId, updateFormId);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class CreateEmailCampaign {\n");
sb.append(" tag: ").append(toIndentedString(tag)).append("\n");
sb.append(" sender: ").append(toIndentedString(sender)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" htmlContent: ").append(toIndentedString(htmlContent)).append("\n");
sb.append(" htmlUrl: ").append(toIndentedString(htmlUrl)).append("\n");
sb.append(" templateId: ").append(toIndentedString(templateId)).append("\n");
sb.append(" scheduledAt: ").append(toIndentedString(scheduledAt)).append("\n");
sb.append(" subject: ").append(toIndentedString(subject)).append("\n");
sb.append(" replyTo: ").append(toIndentedString(replyTo)).append("\n");
sb.append(" toField: ").append(toIndentedString(toField)).append("\n");
sb.append(" recipients: ").append(toIndentedString(recipients)).append("\n");
sb.append(" attachmentUrl: ").append(toIndentedString(attachmentUrl)).append("\n");
sb.append(" inlineImageActivation: ").append(toIndentedString(inlineImageActivation)).append("\n");
sb.append(" mirrorActive: ").append(toIndentedString(mirrorActive)).append("\n");
sb.append(" footer: ").append(toIndentedString(footer)).append("\n");
sb.append(" header: ").append(toIndentedString(header)).append("\n");
sb.append(" utmCampaign: ").append(toIndentedString(utmCampaign)).append("\n");
sb.append(" params: ").append(toIndentedString(params)).append("\n");
sb.append(" sendAtBestTime: ").append(toIndentedString(sendAtBestTime)).append("\n");
sb.append(" abTesting: ").append(toIndentedString(abTesting)).append("\n");
sb.append(" subjectA: ").append(toIndentedString(subjectA)).append("\n");
sb.append(" subjectB: ").append(toIndentedString(subjectB)).append("\n");
sb.append(" splitRule: ").append(toIndentedString(splitRule)).append("\n");
sb.append(" winnerCriteria: ").append(toIndentedString(winnerCriteria)).append("\n");
sb.append(" winnerDelay: ").append(toIndentedString(winnerDelay)).append("\n");
sb.append(" ipWarmupEnable: ").append(toIndentedString(ipWarmupEnable)).append("\n");
sb.append(" initialQuota: ").append(toIndentedString(initialQuota)).append("\n");
sb.append(" increaseRate: ").append(toIndentedString(increaseRate)).append("\n");
sb.append(" unsubscriptionPageId: ").append(toIndentedString(unsubscriptionPageId)).append("\n");
sb.append(" updateFormId: ").append(toIndentedString(updateFormId)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy