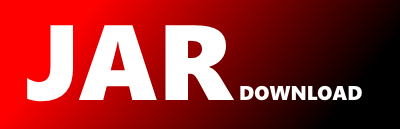
sibModel.CreateSmtpTemplate Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sib-api-v3-sdk Show documentation
Show all versions of sib-api-v3-sdk Show documentation
SendinBlue's API v3 Java Library
The newest version!
/*
* SendinBlue API
* SendinBlue provide a RESTFul API that can be used with any languages. With this API, you will be able to : - Manage your campaigns and get the statistics - Manage your contacts - Send transactional Emails and SMS - and much more... You can download our wrappers at https://github.com/orgs/sendinblue **Possible responses** | Code | Message | | :-------------: | ------------- | | 200 | OK. Successful Request | | 201 | OK. Successful Creation | | 202 | OK. Request accepted | | 204 | OK. Successful Update/Deletion | | 400 | Error. Bad Request | | 401 | Error. Authentication Needed | | 402 | Error. Not enough credit, plan upgrade needed | | 403 | Error. Permission denied | | 404 | Error. Object does not exist | | 405 | Error. Method not allowed | | 406 | Error. Not Acceptable |
*
* OpenAPI spec version: 3.0.0
* Contact: [email protected]
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package sibModel;
import org.apache.commons.lang3.ObjectUtils;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import sibModel.CreateSmtpTemplateSender;
/**
* CreateSmtpTemplate
*/
@javax.annotation.Generated(value = "io.swagger.codegen.languages.JavaClientCodegen", date = "2023-02-28T15:16:18.937+05:30")
public class CreateSmtpTemplate {
@SerializedName("tag")
private String tag = null;
@SerializedName("sender")
private CreateSmtpTemplateSender sender = null;
@SerializedName("templateName")
private String templateName = null;
@SerializedName("htmlContent")
private String htmlContent = null;
@SerializedName("htmlUrl")
private String htmlUrl = null;
@SerializedName("subject")
private String subject = null;
@SerializedName("replyTo")
private String replyTo = null;
@SerializedName("toField")
private String toField = null;
@SerializedName("attachmentUrl")
private String attachmentUrl = null;
@SerializedName("isActive")
private Boolean isActive = null;
public CreateSmtpTemplate tag(String tag) {
this.tag = tag;
return this;
}
/**
* Tag of the template
* @return tag
**/
@ApiModelProperty(example = "OrderConfirmation", value = "Tag of the template")
public String getTag() {
return tag;
}
public void setTag(String tag) {
this.tag = tag;
}
public CreateSmtpTemplate sender(CreateSmtpTemplateSender sender) {
this.sender = sender;
return this;
}
/**
* Get sender
* @return sender
**/
@ApiModelProperty(required = true, value = "")
public CreateSmtpTemplateSender getSender() {
return sender;
}
public void setSender(CreateSmtpTemplateSender sender) {
this.sender = sender;
}
public CreateSmtpTemplate templateName(String templateName) {
this.templateName = templateName;
return this;
}
/**
* Name of the template
* @return templateName
**/
@ApiModelProperty(example = "Order Confirmation - EN", required = true, value = "Name of the template")
public String getTemplateName() {
return templateName;
}
public void setTemplateName(String templateName) {
this.templateName = templateName;
}
public CreateSmtpTemplate htmlContent(String htmlContent) {
this.htmlContent = htmlContent;
return this;
}
/**
* Body of the message (HTML version). The field must have more than 10 characters. REQUIRED if htmlUrl is empty
* @return htmlContent
**/
@ApiModelProperty(example = "The order n°xxxxx has been confirmed. Thanks for your purchase", value = "Body of the message (HTML version). The field must have more than 10 characters. REQUIRED if htmlUrl is empty")
public String getHtmlContent() {
return htmlContent;
}
public void setHtmlContent(String htmlContent) {
this.htmlContent = htmlContent;
}
public CreateSmtpTemplate htmlUrl(String htmlUrl) {
this.htmlUrl = htmlUrl;
return this;
}
/**
* Url which contents the body of the email message. REQUIRED if htmlContent is empty
* @return htmlUrl
**/
@ApiModelProperty(example = "https://html.domain.com", value = "Url which contents the body of the email message. REQUIRED if htmlContent is empty")
public String getHtmlUrl() {
return htmlUrl;
}
public void setHtmlUrl(String htmlUrl) {
this.htmlUrl = htmlUrl;
}
public CreateSmtpTemplate subject(String subject) {
this.subject = subject;
return this;
}
/**
* Subject of the template
* @return subject
**/
@ApiModelProperty(example = "Thanks for your purchase !", required = true, value = "Subject of the template")
public String getSubject() {
return subject;
}
public void setSubject(String subject) {
this.subject = subject;
}
public CreateSmtpTemplate replyTo(String replyTo) {
this.replyTo = replyTo;
return this;
}
/**
* Email on which campaign recipients will be able to reply to
* @return replyTo
**/
@ApiModelProperty(example = "[email protected]", value = "Email on which campaign recipients will be able to reply to")
public String getReplyTo() {
return replyTo;
}
public void setReplyTo(String replyTo) {
this.replyTo = replyTo;
}
public CreateSmtpTemplate toField(String toField) {
this.toField = toField;
return this;
}
/**
* To personalize the «To» Field. If you want to include the first name and last name of your recipient, add {FNAME} {LNAME}. These contact attributes must already exist in your SendinBlue account. If input parameter 'params' used please use {{contact.FNAME}} {{contact.LNAME}} for personalization
* @return toField
**/
@ApiModelProperty(example = "{FNAME} {LNAME}", value = "To personalize the «To» Field. If you want to include the first name and last name of your recipient, add {FNAME} {LNAME}. These contact attributes must already exist in your SendinBlue account. If input parameter 'params' used please use {{contact.FNAME}} {{contact.LNAME}} for personalization")
public String getToField() {
return toField;
}
public void setToField(String toField) {
this.toField = toField;
}
public CreateSmtpTemplate attachmentUrl(String attachmentUrl) {
this.attachmentUrl = attachmentUrl;
return this;
}
/**
* Absolute url of the attachment (no local file). Extension allowed: xlsx, xls, ods, docx, docm, doc, csv, pdf, txt, gif, jpg, jpeg, png, tif, tiff, rtf, bmp, cgm, css, shtml, html, htm, zip, xml, ppt, pptx, tar, ez, ics, mobi, msg, pub and eps
* @return attachmentUrl
**/
@ApiModelProperty(example = "https://attachment.domain.com", value = "Absolute url of the attachment (no local file). Extension allowed: xlsx, xls, ods, docx, docm, doc, csv, pdf, txt, gif, jpg, jpeg, png, tif, tiff, rtf, bmp, cgm, css, shtml, html, htm, zip, xml, ppt, pptx, tar, ez, ics, mobi, msg, pub and eps")
public String getAttachmentUrl() {
return attachmentUrl;
}
public void setAttachmentUrl(String attachmentUrl) {
this.attachmentUrl = attachmentUrl;
}
public CreateSmtpTemplate isActive(Boolean isActive) {
this.isActive = isActive;
return this;
}
/**
* Status of template. isActive = true means template is active and isActive = false means template is inactive
* @return isActive
**/
@ApiModelProperty(example = "true", value = "Status of template. isActive = true means template is active and isActive = false means template is inactive")
public Boolean isIsActive() {
return isActive;
}
public void setIsActive(Boolean isActive) {
this.isActive = isActive;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
CreateSmtpTemplate createSmtpTemplate = (CreateSmtpTemplate) o;
return ObjectUtils.equals(this.tag, createSmtpTemplate.tag) &&
ObjectUtils.equals(this.sender, createSmtpTemplate.sender) &&
ObjectUtils.equals(this.templateName, createSmtpTemplate.templateName) &&
ObjectUtils.equals(this.htmlContent, createSmtpTemplate.htmlContent) &&
ObjectUtils.equals(this.htmlUrl, createSmtpTemplate.htmlUrl) &&
ObjectUtils.equals(this.subject, createSmtpTemplate.subject) &&
ObjectUtils.equals(this.replyTo, createSmtpTemplate.replyTo) &&
ObjectUtils.equals(this.toField, createSmtpTemplate.toField) &&
ObjectUtils.equals(this.attachmentUrl, createSmtpTemplate.attachmentUrl) &&
ObjectUtils.equals(this.isActive, createSmtpTemplate.isActive);
}
@Override
public int hashCode() {
return ObjectUtils.hashCodeMulti(tag, sender, templateName, htmlContent, htmlUrl, subject, replyTo, toField, attachmentUrl, isActive);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class CreateSmtpTemplate {\n");
sb.append(" tag: ").append(toIndentedString(tag)).append("\n");
sb.append(" sender: ").append(toIndentedString(sender)).append("\n");
sb.append(" templateName: ").append(toIndentedString(templateName)).append("\n");
sb.append(" htmlContent: ").append(toIndentedString(htmlContent)).append("\n");
sb.append(" htmlUrl: ").append(toIndentedString(htmlUrl)).append("\n");
sb.append(" subject: ").append(toIndentedString(subject)).append("\n");
sb.append(" replyTo: ").append(toIndentedString(replyTo)).append("\n");
sb.append(" toField: ").append(toIndentedString(toField)).append("\n");
sb.append(" attachmentUrl: ").append(toIndentedString(attachmentUrl)).append("\n");
sb.append(" isActive: ").append(toIndentedString(isActive)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy