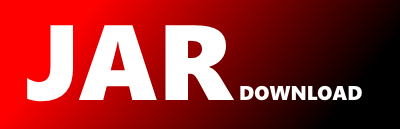
sibModel.GetCampaignOverview Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sib-api-v3-sdk Show documentation
Show all versions of sib-api-v3-sdk Show documentation
SendinBlue's API v3 Java Library
The newest version!
/*
* SendinBlue API
* SendinBlue provide a RESTFul API that can be used with any languages. With this API, you will be able to : - Manage your campaigns and get the statistics - Manage your contacts - Send transactional Emails and SMS - and much more... You can download our wrappers at https://github.com/orgs/sendinblue **Possible responses** | Code | Message | | :-------------: | ------------- | | 200 | OK. Successful Request | | 201 | OK. Successful Creation | | 202 | OK. Request accepted | | 204 | OK. Successful Update/Deletion | | 400 | Error. Bad Request | | 401 | Error. Authentication Needed | | 402 | Error. Not enough credit, plan upgrade needed | | 403 | Error. Permission denied | | 404 | Error. Object does not exist | | 405 | Error. Method not allowed | | 406 | Error. Not Acceptable |
*
* OpenAPI spec version: 3.0.0
* Contact: [email protected]
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package sibModel;
import org.apache.commons.lang3.ObjectUtils;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
/**
* GetCampaignOverview
*/
@javax.annotation.Generated(value = "io.swagger.codegen.languages.JavaClientCodegen", date = "2023-02-28T15:16:18.937+05:30")
public class GetCampaignOverview {
@SerializedName("id")
private Long id = null;
@SerializedName("name")
private String name = null;
@SerializedName("subject")
private String subject = null;
/**
* Type of campaign
*/
@JsonAdapter(TypeEnum.Adapter.class)
public enum TypeEnum {
CLASSIC("classic"),
TRIGGER("trigger");
private String value;
TypeEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static TypeEnum fromValue(String text) {
for (TypeEnum b : TypeEnum.values()) {
if (String.valueOf(b.value).equals(text)) {
return b;
}
}
return null;
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final TypeEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public TypeEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return TypeEnum.fromValue(String.valueOf(value));
}
}
}
@SerializedName("type")
private TypeEnum type = null;
/**
* Status of the campaign
*/
@JsonAdapter(StatusEnum.Adapter.class)
public enum StatusEnum {
DRAFT("draft"),
SENT("sent"),
ARCHIVE("archive"),
QUEUED("queued"),
SUSPENDED("suspended"),
IN_PROCESS("in_process");
private String value;
StatusEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static StatusEnum fromValue(String text) {
for (StatusEnum b : StatusEnum.values()) {
if (String.valueOf(b.value).equals(text)) {
return b;
}
}
return null;
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final StatusEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public StatusEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return StatusEnum.fromValue(String.valueOf(value));
}
}
}
@SerializedName("status")
private StatusEnum status = null;
@SerializedName("scheduledAt")
private String scheduledAt = null;
@SerializedName("abTesting")
private Boolean abTesting = null;
@SerializedName("subjectA")
private String subjectA = null;
@SerializedName("subjectB")
private String subjectB = null;
@SerializedName("splitRule")
private Integer splitRule = null;
@SerializedName("winnerCriteria")
private String winnerCriteria = null;
@SerializedName("winnerDelay")
private Integer winnerDelay = null;
@SerializedName("sendAtBestTime")
private Boolean sendAtBestTime = null;
public GetCampaignOverview id(Long id) {
this.id = id;
return this;
}
/**
* ID of the campaign
* @return id
**/
@ApiModelProperty(example = "12", required = true, value = "ID of the campaign")
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public GetCampaignOverview name(String name) {
this.name = name;
return this;
}
/**
* Name of the campaign
* @return name
**/
@ApiModelProperty(example = "EN - Sales Summer 2017", required = true, value = "Name of the campaign")
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public GetCampaignOverview subject(String subject) {
this.subject = subject;
return this;
}
/**
* Subject of the campaign. Only available if `abTesting` flag of the campaign is `false`
* @return subject
**/
@ApiModelProperty(example = "20% OFF for 2017 Summer Sales", value = "Subject of the campaign. Only available if `abTesting` flag of the campaign is `false`")
public String getSubject() {
return subject;
}
public void setSubject(String subject) {
this.subject = subject;
}
public GetCampaignOverview type(TypeEnum type) {
this.type = type;
return this;
}
/**
* Type of campaign
* @return type
**/
@ApiModelProperty(example = "classic", required = true, value = "Type of campaign")
public TypeEnum getType() {
return type;
}
public void setType(TypeEnum type) {
this.type = type;
}
public GetCampaignOverview status(StatusEnum status) {
this.status = status;
return this;
}
/**
* Status of the campaign
* @return status
**/
@ApiModelProperty(example = "sent", required = true, value = "Status of the campaign")
public StatusEnum getStatus() {
return status;
}
public void setStatus(StatusEnum status) {
this.status = status;
}
public GetCampaignOverview scheduledAt(String scheduledAt) {
this.scheduledAt = scheduledAt;
return this;
}
/**
* UTC date-time on which campaign is scheduled (YYYY-MM-DDTHH:mm:ss.SSSZ)
* @return scheduledAt
**/
@ApiModelProperty(example = "2017-06-01T12:30:00Z", value = "UTC date-time on which campaign is scheduled (YYYY-MM-DDTHH:mm:ss.SSSZ)")
public String getScheduledAt() {
return scheduledAt;
}
public void setScheduledAt(String scheduledAt) {
this.scheduledAt = scheduledAt;
}
public GetCampaignOverview abTesting(Boolean abTesting) {
this.abTesting = abTesting;
return this;
}
/**
* Status of A/B Test for the campaign. abTesting = false means it is disabled, & abTesting = true means it is enabled.
* @return abTesting
**/
@ApiModelProperty(example = "true", value = "Status of A/B Test for the campaign. abTesting = false means it is disabled, & abTesting = true means it is enabled.")
public Boolean isAbTesting() {
return abTesting;
}
public void setAbTesting(Boolean abTesting) {
this.abTesting = abTesting;
}
public GetCampaignOverview subjectA(String subjectA) {
this.subjectA = subjectA;
return this;
}
/**
* Subject A of the ab-test campaign. Only available if `abTesting` flag of the campaign is `true`
* @return subjectA
**/
@ApiModelProperty(example = "Discover the New Collection!", value = "Subject A of the ab-test campaign. Only available if `abTesting` flag of the campaign is `true`")
public String getSubjectA() {
return subjectA;
}
public void setSubjectA(String subjectA) {
this.subjectA = subjectA;
}
public GetCampaignOverview subjectB(String subjectB) {
this.subjectB = subjectB;
return this;
}
/**
* Subject B of the ab-test campaign. Only available if `abTesting` flag of the campaign is `true`
* @return subjectB
**/
@ApiModelProperty(example = "Want to discover the New Collection?", value = "Subject B of the ab-test campaign. Only available if `abTesting` flag of the campaign is `true`")
public String getSubjectB() {
return subjectB;
}
public void setSubjectB(String subjectB) {
this.subjectB = subjectB;
}
public GetCampaignOverview splitRule(Integer splitRule) {
this.splitRule = splitRule;
return this;
}
/**
* The size of your ab-test groups. Only available if `abTesting` flag of the campaign is `true`
* @return splitRule
**/
@ApiModelProperty(example = "25", value = "The size of your ab-test groups. Only available if `abTesting` flag of the campaign is `true`")
public Integer getSplitRule() {
return splitRule;
}
public void setSplitRule(Integer splitRule) {
this.splitRule = splitRule;
}
public GetCampaignOverview winnerCriteria(String winnerCriteria) {
this.winnerCriteria = winnerCriteria;
return this;
}
/**
* Criteria for the winning version. Only available if `abTesting` flag of the campaign is `true`
* @return winnerCriteria
**/
@ApiModelProperty(example = "open", value = "Criteria for the winning version. Only available if `abTesting` flag of the campaign is `true`")
public String getWinnerCriteria() {
return winnerCriteria;
}
public void setWinnerCriteria(String winnerCriteria) {
this.winnerCriteria = winnerCriteria;
}
public GetCampaignOverview winnerDelay(Integer winnerDelay) {
this.winnerDelay = winnerDelay;
return this;
}
/**
* The duration of the test in hours at the end of which the winning version will be sent. Only available if `abTesting` flag of the campaign is `true`
* @return winnerDelay
**/
@ApiModelProperty(example = "50", value = "The duration of the test in hours at the end of which the winning version will be sent. Only available if `abTesting` flag of the campaign is `true`")
public Integer getWinnerDelay() {
return winnerDelay;
}
public void setWinnerDelay(Integer winnerDelay) {
this.winnerDelay = winnerDelay;
}
public GetCampaignOverview sendAtBestTime(Boolean sendAtBestTime) {
this.sendAtBestTime = sendAtBestTime;
return this;
}
/**
* It is true if you have chosen to send your campaign at best time, otherwise it is false
* @return sendAtBestTime
**/
@ApiModelProperty(example = "true", value = "It is true if you have chosen to send your campaign at best time, otherwise it is false")
public Boolean isSendAtBestTime() {
return sendAtBestTime;
}
public void setSendAtBestTime(Boolean sendAtBestTime) {
this.sendAtBestTime = sendAtBestTime;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
GetCampaignOverview getCampaignOverview = (GetCampaignOverview) o;
return ObjectUtils.equals(this.id, getCampaignOverview.id) &&
ObjectUtils.equals(this.name, getCampaignOverview.name) &&
ObjectUtils.equals(this.subject, getCampaignOverview.subject) &&
ObjectUtils.equals(this.type, getCampaignOverview.type) &&
ObjectUtils.equals(this.status, getCampaignOverview.status) &&
ObjectUtils.equals(this.scheduledAt, getCampaignOverview.scheduledAt) &&
ObjectUtils.equals(this.abTesting, getCampaignOverview.abTesting) &&
ObjectUtils.equals(this.subjectA, getCampaignOverview.subjectA) &&
ObjectUtils.equals(this.subjectB, getCampaignOverview.subjectB) &&
ObjectUtils.equals(this.splitRule, getCampaignOverview.splitRule) &&
ObjectUtils.equals(this.winnerCriteria, getCampaignOverview.winnerCriteria) &&
ObjectUtils.equals(this.winnerDelay, getCampaignOverview.winnerDelay) &&
ObjectUtils.equals(this.sendAtBestTime, getCampaignOverview.sendAtBestTime);
}
@Override
public int hashCode() {
return ObjectUtils.hashCodeMulti(id, name, subject, type, status, scheduledAt, abTesting, subjectA, subjectB, splitRule, winnerCriteria, winnerDelay, sendAtBestTime);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class GetCampaignOverview {\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" subject: ").append(toIndentedString(subject)).append("\n");
sb.append(" type: ").append(toIndentedString(type)).append("\n");
sb.append(" status: ").append(toIndentedString(status)).append("\n");
sb.append(" scheduledAt: ").append(toIndentedString(scheduledAt)).append("\n");
sb.append(" abTesting: ").append(toIndentedString(abTesting)).append("\n");
sb.append(" subjectA: ").append(toIndentedString(subjectA)).append("\n");
sb.append(" subjectB: ").append(toIndentedString(subjectB)).append("\n");
sb.append(" splitRule: ").append(toIndentedString(splitRule)).append("\n");
sb.append(" winnerCriteria: ").append(toIndentedString(winnerCriteria)).append("\n");
sb.append(" winnerDelay: ").append(toIndentedString(winnerDelay)).append("\n");
sb.append(" sendAtBestTime: ").append(toIndentedString(sendAtBestTime)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy