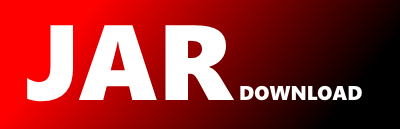
sibModel.GetInboundEmailEventsByUuid Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sib-api-v3-sdk Show documentation
Show all versions of sib-api-v3-sdk Show documentation
SendinBlue's API v3 Java Library
The newest version!
/*
* SendinBlue API
* SendinBlue provide a RESTFul API that can be used with any languages. With this API, you will be able to : - Manage your campaigns and get the statistics - Manage your contacts - Send transactional Emails and SMS - and much more... You can download our wrappers at https://github.com/orgs/sendinblue **Possible responses** | Code | Message | | :-------------: | ------------- | | 200 | OK. Successful Request | | 201 | OK. Successful Creation | | 202 | OK. Request accepted | | 204 | OK. Successful Update/Deletion | | 400 | Error. Bad Request | | 401 | Error. Authentication Needed | | 402 | Error. Not enough credit, plan upgrade needed | | 403 | Error. Permission denied | | 404 | Error. Object does not exist | | 405 | Error. Method not allowed | | 406 | Error. Not Acceptable |
*
* OpenAPI spec version: 3.0.0
* Contact: [email protected]
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package sibModel;
import org.apache.commons.lang3.ObjectUtils;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import org.threeten.bp.OffsetDateTime;
import sibModel.GetInboundEmailEventsByUuidAttachments;
import sibModel.GetInboundEmailEventsByUuidLogs;
/**
* GetInboundEmailEventsByUuid
*/
@javax.annotation.Generated(value = "io.swagger.codegen.languages.JavaClientCodegen", date = "2023-02-28T15:16:18.937+05:30")
public class GetInboundEmailEventsByUuid {
@SerializedName("receivedAt")
private OffsetDateTime receivedAt = null;
@SerializedName("deliveredAt")
private OffsetDateTime deliveredAt = null;
@SerializedName("recipient")
private String recipient = null;
@SerializedName("sender")
private String sender = null;
@SerializedName("messageId")
private String messageId = null;
@SerializedName("subject")
private String subject = null;
@SerializedName("attachments")
private List attachments = null;
@SerializedName("logs")
private List logs = null;
public GetInboundEmailEventsByUuid receivedAt(OffsetDateTime receivedAt) {
this.receivedAt = receivedAt;
return this;
}
/**
* Date when email was received on SMTP relay
* @return receivedAt
**/
@ApiModelProperty(example = "2019-05-25T11:53:26Z", value = "Date when email was received on SMTP relay")
public OffsetDateTime getReceivedAt() {
return receivedAt;
}
public void setReceivedAt(OffsetDateTime receivedAt) {
this.receivedAt = receivedAt;
}
public GetInboundEmailEventsByUuid deliveredAt(OffsetDateTime deliveredAt) {
this.deliveredAt = deliveredAt;
return this;
}
/**
* Date when email was delivered successfully to client’s webhook
* @return deliveredAt
**/
@ApiModelProperty(value = "Date when email was delivered successfully to client’s webhook")
public OffsetDateTime getDeliveredAt() {
return deliveredAt;
}
public void setDeliveredAt(OffsetDateTime deliveredAt) {
this.deliveredAt = deliveredAt;
}
public GetInboundEmailEventsByUuid recipient(String recipient) {
this.recipient = recipient;
return this;
}
/**
* Recipient’s email address
* @return recipient
**/
@ApiModelProperty(value = "Recipient’s email address")
public String getRecipient() {
return recipient;
}
public void setRecipient(String recipient) {
this.recipient = recipient;
}
public GetInboundEmailEventsByUuid sender(String sender) {
this.sender = sender;
return this;
}
/**
* Sender’s email address
* @return sender
**/
@ApiModelProperty(value = "Sender’s email address")
public String getSender() {
return sender;
}
public void setSender(String sender) {
this.sender = sender;
}
public GetInboundEmailEventsByUuid messageId(String messageId) {
this.messageId = messageId;
return this;
}
/**
* Value of the Message-ID header. This will be present only after the processing is done.
* @return messageId
**/
@ApiModelProperty(value = "Value of the Message-ID header. This will be present only after the processing is done.")
public String getMessageId() {
return messageId;
}
public void setMessageId(String messageId) {
this.messageId = messageId;
}
public GetInboundEmailEventsByUuid subject(String subject) {
this.subject = subject;
return this;
}
/**
* Value of the Subject header. This will be present only after the processing is done.
* @return subject
**/
@ApiModelProperty(value = "Value of the Subject header. This will be present only after the processing is done. ")
public String getSubject() {
return subject;
}
public void setSubject(String subject) {
this.subject = subject;
}
public GetInboundEmailEventsByUuid attachments(List attachments) {
this.attachments = attachments;
return this;
}
public GetInboundEmailEventsByUuid addAttachmentsItem(GetInboundEmailEventsByUuidAttachments attachmentsItem) {
if (this.attachments == null) {
this.attachments = new ArrayList();
}
this.attachments.add(attachmentsItem);
return this;
}
/**
* List of attachments of the email. This will be present only after the processing is done.
* @return attachments
**/
@ApiModelProperty(value = "List of attachments of the email. This will be present only after the processing is done.")
public List getAttachments() {
return attachments;
}
public void setAttachments(List attachments) {
this.attachments = attachments;
}
public GetInboundEmailEventsByUuid logs(List logs) {
this.logs = logs;
return this;
}
public GetInboundEmailEventsByUuid addLogsItem(GetInboundEmailEventsByUuidLogs logsItem) {
if (this.logs == null) {
this.logs = new ArrayList();
}
this.logs.add(logsItem);
return this;
}
/**
* List of events/logs that describe the lifecycle of the email on SIB platform
* @return logs
**/
@ApiModelProperty(value = "List of events/logs that describe the lifecycle of the email on SIB platform")
public List getLogs() {
return logs;
}
public void setLogs(List logs) {
this.logs = logs;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
GetInboundEmailEventsByUuid getInboundEmailEventsByUuid = (GetInboundEmailEventsByUuid) o;
return ObjectUtils.equals(this.receivedAt, getInboundEmailEventsByUuid.receivedAt) &&
ObjectUtils.equals(this.deliveredAt, getInboundEmailEventsByUuid.deliveredAt) &&
ObjectUtils.equals(this.recipient, getInboundEmailEventsByUuid.recipient) &&
ObjectUtils.equals(this.sender, getInboundEmailEventsByUuid.sender) &&
ObjectUtils.equals(this.messageId, getInboundEmailEventsByUuid.messageId) &&
ObjectUtils.equals(this.subject, getInboundEmailEventsByUuid.subject) &&
ObjectUtils.equals(this.attachments, getInboundEmailEventsByUuid.attachments) &&
ObjectUtils.equals(this.logs, getInboundEmailEventsByUuid.logs);
}
@Override
public int hashCode() {
return ObjectUtils.hashCodeMulti(receivedAt, deliveredAt, recipient, sender, messageId, subject, attachments, logs);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class GetInboundEmailEventsByUuid {\n");
sb.append(" receivedAt: ").append(toIndentedString(receivedAt)).append("\n");
sb.append(" deliveredAt: ").append(toIndentedString(deliveredAt)).append("\n");
sb.append(" recipient: ").append(toIndentedString(recipient)).append("\n");
sb.append(" sender: ").append(toIndentedString(sender)).append("\n");
sb.append(" messageId: ").append(toIndentedString(messageId)).append("\n");
sb.append(" subject: ").append(toIndentedString(subject)).append("\n");
sb.append(" attachments: ").append(toIndentedString(attachments)).append("\n");
sb.append(" logs: ").append(toIndentedString(logs)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy