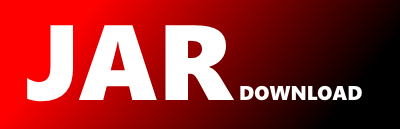
sibModel.GetReportsReports Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sib-api-v3-sdk Show documentation
Show all versions of sib-api-v3-sdk Show documentation
SendinBlue's API v3 Java Library
The newest version!
/*
* SendinBlue API
* SendinBlue provide a RESTFul API that can be used with any languages. With this API, you will be able to : - Manage your campaigns and get the statistics - Manage your contacts - Send transactional Emails and SMS - and much more... You can download our wrappers at https://github.com/orgs/sendinblue **Possible responses** | Code | Message | | :-------------: | ------------- | | 200 | OK. Successful Request | | 201 | OK. Successful Creation | | 202 | OK. Request accepted | | 204 | OK. Successful Update/Deletion | | 400 | Error. Bad Request | | 401 | Error. Authentication Needed | | 402 | Error. Not enough credit, plan upgrade needed | | 403 | Error. Permission denied | | 404 | Error. Object does not exist | | 405 | Error. Method not allowed | | 406 | Error. Not Acceptable |
*
* OpenAPI spec version: 3.0.0
* Contact: [email protected]
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package sibModel;
import org.apache.commons.lang3.ObjectUtils;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import org.threeten.bp.LocalDate;
/**
* GetReportsReports
*/
@javax.annotation.Generated(value = "io.swagger.codegen.languages.JavaClientCodegen", date = "2023-02-28T15:16:18.937+05:30")
public class GetReportsReports {
@SerializedName("date")
private LocalDate date = null;
@SerializedName("requests")
private Long requests = null;
@SerializedName("delivered")
private Long delivered = null;
@SerializedName("hardBounces")
private Long hardBounces = null;
@SerializedName("softBounces")
private Long softBounces = null;
@SerializedName("clicks")
private Long clicks = null;
@SerializedName("uniqueClicks")
private Long uniqueClicks = null;
@SerializedName("opens")
private Long opens = null;
@SerializedName("uniqueOpens")
private Long uniqueOpens = null;
@SerializedName("spamReports")
private Long spamReports = null;
@SerializedName("blocked")
private Long blocked = null;
@SerializedName("invalid")
private Long invalid = null;
@SerializedName("unsubscribed")
private Long unsubscribed = null;
public GetReportsReports date(LocalDate date) {
this.date = date;
return this;
}
/**
* Date of the statistics
* @return date
**/
@ApiModelProperty(example = "2017-04-06", required = true, value = "Date of the statistics")
public LocalDate getDate() {
return date;
}
public void setDate(LocalDate date) {
this.date = date;
}
public GetReportsReports requests(Long requests) {
this.requests = requests;
return this;
}
/**
* Number of requests for the date
* @return requests
**/
@ApiModelProperty(example = "65", required = true, value = "Number of requests for the date")
public Long getRequests() {
return requests;
}
public void setRequests(Long requests) {
this.requests = requests;
}
public GetReportsReports delivered(Long delivered) {
this.delivered = delivered;
return this;
}
/**
* Number of delivered emails for the date
* @return delivered
**/
@ApiModelProperty(example = "63", required = true, value = "Number of delivered emails for the date")
public Long getDelivered() {
return delivered;
}
public void setDelivered(Long delivered) {
this.delivered = delivered;
}
public GetReportsReports hardBounces(Long hardBounces) {
this.hardBounces = hardBounces;
return this;
}
/**
* Number of hardbounces for the date
* @return hardBounces
**/
@ApiModelProperty(example = "1", required = true, value = "Number of hardbounces for the date")
public Long getHardBounces() {
return hardBounces;
}
public void setHardBounces(Long hardBounces) {
this.hardBounces = hardBounces;
}
public GetReportsReports softBounces(Long softBounces) {
this.softBounces = softBounces;
return this;
}
/**
* Number of softbounces for the date
* @return softBounces
**/
@ApiModelProperty(example = "1", required = true, value = "Number of softbounces for the date")
public Long getSoftBounces() {
return softBounces;
}
public void setSoftBounces(Long softBounces) {
this.softBounces = softBounces;
}
public GetReportsReports clicks(Long clicks) {
this.clicks = clicks;
return this;
}
/**
* Number of clicks for the date
* @return clicks
**/
@ApiModelProperty(example = "6", required = true, value = "Number of clicks for the date")
public Long getClicks() {
return clicks;
}
public void setClicks(Long clicks) {
this.clicks = clicks;
}
public GetReportsReports uniqueClicks(Long uniqueClicks) {
this.uniqueClicks = uniqueClicks;
return this;
}
/**
* Number of unique clicks for the date
* @return uniqueClicks
**/
@ApiModelProperty(example = "5", required = true, value = "Number of unique clicks for the date")
public Long getUniqueClicks() {
return uniqueClicks;
}
public void setUniqueClicks(Long uniqueClicks) {
this.uniqueClicks = uniqueClicks;
}
public GetReportsReports opens(Long opens) {
this.opens = opens;
return this;
}
/**
* Number of openings for the date
* @return opens
**/
@ApiModelProperty(example = "58", required = true, value = "Number of openings for the date")
public Long getOpens() {
return opens;
}
public void setOpens(Long opens) {
this.opens = opens;
}
public GetReportsReports uniqueOpens(Long uniqueOpens) {
this.uniqueOpens = uniqueOpens;
return this;
}
/**
* Number of unique openings for the date
* @return uniqueOpens
**/
@ApiModelProperty(example = "52", required = true, value = "Number of unique openings for the date")
public Long getUniqueOpens() {
return uniqueOpens;
}
public void setUniqueOpens(Long uniqueOpens) {
this.uniqueOpens = uniqueOpens;
}
public GetReportsReports spamReports(Long spamReports) {
this.spamReports = spamReports;
return this;
}
/**
* Number of complaints (spam reports) for the date
* @return spamReports
**/
@ApiModelProperty(example = "0", required = true, value = "Number of complaints (spam reports) for the date")
public Long getSpamReports() {
return spamReports;
}
public void setSpamReports(Long spamReports) {
this.spamReports = spamReports;
}
public GetReportsReports blocked(Long blocked) {
this.blocked = blocked;
return this;
}
/**
* Number of blocked emails for the date
* @return blocked
**/
@ApiModelProperty(example = "0", required = true, value = "Number of blocked emails for the date")
public Long getBlocked() {
return blocked;
}
public void setBlocked(Long blocked) {
this.blocked = blocked;
}
public GetReportsReports invalid(Long invalid) {
this.invalid = invalid;
return this;
}
/**
* Number of invalid emails for the date
* @return invalid
**/
@ApiModelProperty(example = "0", required = true, value = "Number of invalid emails for the date")
public Long getInvalid() {
return invalid;
}
public void setInvalid(Long invalid) {
this.invalid = invalid;
}
public GetReportsReports unsubscribed(Long unsubscribed) {
this.unsubscribed = unsubscribed;
return this;
}
/**
* Number of unsubscribed emails for the date
* @return unsubscribed
**/
@ApiModelProperty(example = "0", required = true, value = "Number of unsubscribed emails for the date")
public Long getUnsubscribed() {
return unsubscribed;
}
public void setUnsubscribed(Long unsubscribed) {
this.unsubscribed = unsubscribed;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
GetReportsReports getReportsReports = (GetReportsReports) o;
return ObjectUtils.equals(this.date, getReportsReports.date) &&
ObjectUtils.equals(this.requests, getReportsReports.requests) &&
ObjectUtils.equals(this.delivered, getReportsReports.delivered) &&
ObjectUtils.equals(this.hardBounces, getReportsReports.hardBounces) &&
ObjectUtils.equals(this.softBounces, getReportsReports.softBounces) &&
ObjectUtils.equals(this.clicks, getReportsReports.clicks) &&
ObjectUtils.equals(this.uniqueClicks, getReportsReports.uniqueClicks) &&
ObjectUtils.equals(this.opens, getReportsReports.opens) &&
ObjectUtils.equals(this.uniqueOpens, getReportsReports.uniqueOpens) &&
ObjectUtils.equals(this.spamReports, getReportsReports.spamReports) &&
ObjectUtils.equals(this.blocked, getReportsReports.blocked) &&
ObjectUtils.equals(this.invalid, getReportsReports.invalid) &&
ObjectUtils.equals(this.unsubscribed, getReportsReports.unsubscribed);
}
@Override
public int hashCode() {
return ObjectUtils.hashCodeMulti(date, requests, delivered, hardBounces, softBounces, clicks, uniqueClicks, opens, uniqueOpens, spamReports, blocked, invalid, unsubscribed);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class GetReportsReports {\n");
sb.append(" date: ").append(toIndentedString(date)).append("\n");
sb.append(" requests: ").append(toIndentedString(requests)).append("\n");
sb.append(" delivered: ").append(toIndentedString(delivered)).append("\n");
sb.append(" hardBounces: ").append(toIndentedString(hardBounces)).append("\n");
sb.append(" softBounces: ").append(toIndentedString(softBounces)).append("\n");
sb.append(" clicks: ").append(toIndentedString(clicks)).append("\n");
sb.append(" uniqueClicks: ").append(toIndentedString(uniqueClicks)).append("\n");
sb.append(" opens: ").append(toIndentedString(opens)).append("\n");
sb.append(" uniqueOpens: ").append(toIndentedString(uniqueOpens)).append("\n");
sb.append(" spamReports: ").append(toIndentedString(spamReports)).append("\n");
sb.append(" blocked: ").append(toIndentedString(blocked)).append("\n");
sb.append(" invalid: ").append(toIndentedString(invalid)).append("\n");
sb.append(" unsubscribed: ").append(toIndentedString(unsubscribed)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy