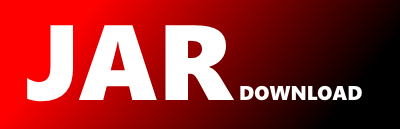
sibModel.MasterDetailsResponsePlanInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sib-api-v3-sdk Show documentation
Show all versions of sib-api-v3-sdk Show documentation
SendinBlue's API v3 Java Library
The newest version!
/*
* SendinBlue API
* SendinBlue provide a RESTFul API that can be used with any languages. With this API, you will be able to : - Manage your campaigns and get the statistics - Manage your contacts - Send transactional Emails and SMS - and much more... You can download our wrappers at https://github.com/orgs/sendinblue **Possible responses** | Code | Message | | :-------------: | ------------- | | 200 | OK. Successful Request | | 201 | OK. Successful Creation | | 202 | OK. Request accepted | | 204 | OK. Successful Update/Deletion | | 400 | Error. Bad Request | | 401 | Error. Authentication Needed | | 402 | Error. Not enough credit, plan upgrade needed | | 403 | Error. Permission denied | | 404 | Error. Object does not exist | | 405 | Error. Method not allowed | | 406 | Error. Not Acceptable |
*
* OpenAPI spec version: 3.0.0
* Contact: [email protected]
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package sibModel;
import org.apache.commons.lang3.ObjectUtils;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.math.BigDecimal;
import java.util.ArrayList;
import java.util.List;
import sibModel.MasterDetailsResponsePlanInfoFeatures;
/**
* Plan details
*/
@ApiModel(description = "Plan details")
@javax.annotation.Generated(value = "io.swagger.codegen.languages.JavaClientCodegen", date = "2023-02-28T15:16:18.937+05:30")
public class MasterDetailsResponsePlanInfo {
@SerializedName("currencyCode")
private String currencyCode = null;
@SerializedName("nextBillingAt")
private Long nextBillingAt = null;
@SerializedName("price")
private BigDecimal price = null;
/**
* Plan period type
*/
@JsonAdapter(PlanPeriodEnum.Adapter.class)
public enum PlanPeriodEnum {
MONTH("month"),
YEAR("year");
private String value;
PlanPeriodEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static PlanPeriodEnum fromValue(String text) {
for (PlanPeriodEnum b : PlanPeriodEnum.values()) {
if (String.valueOf(b.value).equals(text)) {
return b;
}
}
return null;
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final PlanPeriodEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public PlanPeriodEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return PlanPeriodEnum.fromValue(String.valueOf(value));
}
}
}
@SerializedName("planPeriod")
private PlanPeriodEnum planPeriod = null;
@SerializedName("subAccounts")
private Integer subAccounts = null;
@SerializedName("features")
private List features = null;
public MasterDetailsResponsePlanInfo currencyCode(String currencyCode) {
this.currencyCode = currencyCode;
return this;
}
/**
* Plan currency
* @return currencyCode
**/
@ApiModelProperty(value = "Plan currency")
public String getCurrencyCode() {
return currencyCode;
}
public void setCurrencyCode(String currencyCode) {
this.currencyCode = currencyCode;
}
public MasterDetailsResponsePlanInfo nextBillingAt(Long nextBillingAt) {
this.nextBillingAt = nextBillingAt;
return this;
}
/**
* Timestamp of next billing date
* @return nextBillingAt
**/
@ApiModelProperty(value = "Timestamp of next billing date")
public Long getNextBillingAt() {
return nextBillingAt;
}
public void setNextBillingAt(Long nextBillingAt) {
this.nextBillingAt = nextBillingAt;
}
public MasterDetailsResponsePlanInfo price(BigDecimal price) {
this.price = price;
return this;
}
/**
* Plan amount
* @return price
**/
@ApiModelProperty(value = "Plan amount")
public BigDecimal getPrice() {
return price;
}
public void setPrice(BigDecimal price) {
this.price = price;
}
public MasterDetailsResponsePlanInfo planPeriod(PlanPeriodEnum planPeriod) {
this.planPeriod = planPeriod;
return this;
}
/**
* Plan period type
* @return planPeriod
**/
@ApiModelProperty(value = "Plan period type")
public PlanPeriodEnum getPlanPeriod() {
return planPeriod;
}
public void setPlanPeriod(PlanPeriodEnum planPeriod) {
this.planPeriod = planPeriod;
}
public MasterDetailsResponsePlanInfo subAccounts(Integer subAccounts) {
this.subAccounts = subAccounts;
return this;
}
/**
* Number of sub-accounts
* @return subAccounts
**/
@ApiModelProperty(value = "Number of sub-accounts")
public Integer getSubAccounts() {
return subAccounts;
}
public void setSubAccounts(Integer subAccounts) {
this.subAccounts = subAccounts;
}
public MasterDetailsResponsePlanInfo features(List features) {
this.features = features;
return this;
}
public MasterDetailsResponsePlanInfo addFeaturesItem(MasterDetailsResponsePlanInfoFeatures featuresItem) {
if (this.features == null) {
this.features = new ArrayList();
}
this.features.add(featuresItem);
return this;
}
/**
* List of provided features in the plan
* @return features
**/
@ApiModelProperty(value = "List of provided features in the plan")
public List getFeatures() {
return features;
}
public void setFeatures(List features) {
this.features = features;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
MasterDetailsResponsePlanInfo masterDetailsResponsePlanInfo = (MasterDetailsResponsePlanInfo) o;
return ObjectUtils.equals(this.currencyCode, masterDetailsResponsePlanInfo.currencyCode) &&
ObjectUtils.equals(this.nextBillingAt, masterDetailsResponsePlanInfo.nextBillingAt) &&
ObjectUtils.equals(this.price, masterDetailsResponsePlanInfo.price) &&
ObjectUtils.equals(this.planPeriod, masterDetailsResponsePlanInfo.planPeriod) &&
ObjectUtils.equals(this.subAccounts, masterDetailsResponsePlanInfo.subAccounts) &&
ObjectUtils.equals(this.features, masterDetailsResponsePlanInfo.features);
}
@Override
public int hashCode() {
return ObjectUtils.hashCodeMulti(currencyCode, nextBillingAt, price, planPeriod, subAccounts, features);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class MasterDetailsResponsePlanInfo {\n");
sb.append(" currencyCode: ").append(toIndentedString(currencyCode)).append("\n");
sb.append(" nextBillingAt: ").append(toIndentedString(nextBillingAt)).append("\n");
sb.append(" price: ").append(toIndentedString(price)).append("\n");
sb.append(" planPeriod: ").append(toIndentedString(planPeriod)).append("\n");
sb.append(" subAccounts: ").append(toIndentedString(subAccounts)).append("\n");
sb.append(" features: ").append(toIndentedString(features)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy