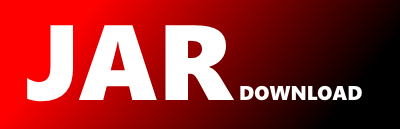
sibModel.RequestContactExportCustomContactFilter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sib-api-v3-sdk Show documentation
Show all versions of sib-api-v3-sdk Show documentation
SendinBlue's API v3 Java Library
The newest version!
/*
* SendinBlue API
* SendinBlue provide a RESTFul API that can be used with any languages. With this API, you will be able to : - Manage your campaigns and get the statistics - Manage your contacts - Send transactional Emails and SMS - and much more... You can download our wrappers at https://github.com/orgs/sendinblue **Possible responses** | Code | Message | | :-------------: | ------------- | | 200 | OK. Successful Request | | 201 | OK. Successful Creation | | 202 | OK. Request accepted | | 204 | OK. Successful Update/Deletion | | 400 | Error. Bad Request | | 401 | Error. Authentication Needed | | 402 | Error. Not enough credit, plan upgrade needed | | 403 | Error. Permission denied | | 404 | Error. Object does not exist | | 405 | Error. Method not allowed | | 406 | Error. Not Acceptable |
*
* OpenAPI spec version: 3.0.0
* Contact: [email protected]
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package sibModel;
import org.apache.commons.lang3.ObjectUtils;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
/**
* Set the filter for the contacts to be exported.
*/
@ApiModel(description = "Set the filter for the contacts to be exported.")
@javax.annotation.Generated(value = "io.swagger.codegen.languages.JavaClientCodegen", date = "2023-02-28T15:16:18.937+05:30")
public class RequestContactExportCustomContactFilter {
/**
* Mandatory if neither actionForEmailCampaigns nor actionForSmsCampaigns is passed. This will export the contacts on the basis of provided action applied on contacts as per the list id. * allContacts - Fetch the list of all contacts for a particular list. * subscribed & unsubscribed - Fetch the list of subscribed / unsubscribed (blacklisted via any means) contacts for a particular list. * unsubscribedPerList - Fetch the list of contacts that are unsubscribed from a particular list only.
*/
@JsonAdapter(ActionForContactsEnum.Adapter.class)
public enum ActionForContactsEnum {
ALLCONTACTS("allContacts"),
SUBSCRIBED("subscribed"),
UNSUBSCRIBED("unsubscribed"),
UNSUBSCRIBEDPERLIST("unsubscribedPerList");
private String value;
ActionForContactsEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static ActionForContactsEnum fromValue(String text) {
for (ActionForContactsEnum b : ActionForContactsEnum.values()) {
if (String.valueOf(b.value).equals(text)) {
return b;
}
}
return null;
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final ActionForContactsEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public ActionForContactsEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return ActionForContactsEnum.fromValue(String.valueOf(value));
}
}
}
@SerializedName("actionForContacts")
private ActionForContactsEnum actionForContacts = null;
/**
* Mandatory if neither actionForContacts nor actionForSmsCampaigns is passed. This will export the contacts on the basis of provided action applied on email campaigns. * openers & nonOpeners - emailCampaignId is mandatory. Fetch the list of readers / non-readers for a particular email campaign. * clickers & nonClickers - emailCampaignId is mandatory. Fetch the list of clickers / non-clickers for a particular email campaign. * unsubscribed - emailCampaignId is mandatory. Fetch the list of all unsubscribed (blacklisted via any means) contacts for a particular email campaign. * hardBounces & softBounces - emailCampaignId is optional. Fetch the list of hard bounces / soft bounces for a particular / all email campaign(s).
*/
@JsonAdapter(ActionForEmailCampaignsEnum.Adapter.class)
public enum ActionForEmailCampaignsEnum {
OPENERS("openers"),
NONOPENERS("nonOpeners"),
CLICKERS("clickers"),
NONCLICKERS("nonClickers"),
UNSUBSCRIBED("unsubscribed"),
HARDBOUNCES("hardBounces"),
SOFTBOUNCES("softBounces");
private String value;
ActionForEmailCampaignsEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static ActionForEmailCampaignsEnum fromValue(String text) {
for (ActionForEmailCampaignsEnum b : ActionForEmailCampaignsEnum.values()) {
if (String.valueOf(b.value).equals(text)) {
return b;
}
}
return null;
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final ActionForEmailCampaignsEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public ActionForEmailCampaignsEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return ActionForEmailCampaignsEnum.fromValue(String.valueOf(value));
}
}
}
@SerializedName("actionForEmailCampaigns")
private ActionForEmailCampaignsEnum actionForEmailCampaigns = null;
/**
* Mandatory if neither actionForContacts nor actionForEmailCampaigns is passed. This will export the contacts on the basis of provided action applied on sms campaigns. * unsubscribed - Fetch the list of all unsubscribed (blacklisted via any means) contacts for all / particular sms campaigns. * hardBounces & softBounces - Fetch the list of hard bounces / soft bounces for all / particular sms campaigns.
*/
@JsonAdapter(ActionForSmsCampaignsEnum.Adapter.class)
public enum ActionForSmsCampaignsEnum {
HARDBOUNCES("hardBounces"),
SOFTBOUNCES("softBounces"),
UNSUBSCRIBED("unsubscribed");
private String value;
ActionForSmsCampaignsEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static ActionForSmsCampaignsEnum fromValue(String text) {
for (ActionForSmsCampaignsEnum b : ActionForSmsCampaignsEnum.values()) {
if (String.valueOf(b.value).equals(text)) {
return b;
}
}
return null;
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final ActionForSmsCampaignsEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public ActionForSmsCampaignsEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return ActionForSmsCampaignsEnum.fromValue(String.valueOf(value));
}
}
}
@SerializedName("actionForSmsCampaigns")
private ActionForSmsCampaignsEnum actionForSmsCampaigns = null;
@SerializedName("listId")
private Long listId = null;
@SerializedName("emailCampaignId")
private Long emailCampaignId = null;
@SerializedName("smsCampaignId")
private Long smsCampaignId = null;
public RequestContactExportCustomContactFilter actionForContacts(ActionForContactsEnum actionForContacts) {
this.actionForContacts = actionForContacts;
return this;
}
/**
* Mandatory if neither actionForEmailCampaigns nor actionForSmsCampaigns is passed. This will export the contacts on the basis of provided action applied on contacts as per the list id. * allContacts - Fetch the list of all contacts for a particular list. * subscribed & unsubscribed - Fetch the list of subscribed / unsubscribed (blacklisted via any means) contacts for a particular list. * unsubscribedPerList - Fetch the list of contacts that are unsubscribed from a particular list only.
* @return actionForContacts
**/
@ApiModelProperty(value = "Mandatory if neither actionForEmailCampaigns nor actionForSmsCampaigns is passed. This will export the contacts on the basis of provided action applied on contacts as per the list id. * allContacts - Fetch the list of all contacts for a particular list. * subscribed & unsubscribed - Fetch the list of subscribed / unsubscribed (blacklisted via any means) contacts for a particular list. * unsubscribedPerList - Fetch the list of contacts that are unsubscribed from a particular list only. ")
public ActionForContactsEnum getActionForContacts() {
return actionForContacts;
}
public void setActionForContacts(ActionForContactsEnum actionForContacts) {
this.actionForContacts = actionForContacts;
}
public RequestContactExportCustomContactFilter actionForEmailCampaigns(ActionForEmailCampaignsEnum actionForEmailCampaigns) {
this.actionForEmailCampaigns = actionForEmailCampaigns;
return this;
}
/**
* Mandatory if neither actionForContacts nor actionForSmsCampaigns is passed. This will export the contacts on the basis of provided action applied on email campaigns. * openers & nonOpeners - emailCampaignId is mandatory. Fetch the list of readers / non-readers for a particular email campaign. * clickers & nonClickers - emailCampaignId is mandatory. Fetch the list of clickers / non-clickers for a particular email campaign. * unsubscribed - emailCampaignId is mandatory. Fetch the list of all unsubscribed (blacklisted via any means) contacts for a particular email campaign. * hardBounces & softBounces - emailCampaignId is optional. Fetch the list of hard bounces / soft bounces for a particular / all email campaign(s).
* @return actionForEmailCampaigns
**/
@ApiModelProperty(value = "Mandatory if neither actionForContacts nor actionForSmsCampaigns is passed. This will export the contacts on the basis of provided action applied on email campaigns. * openers & nonOpeners - emailCampaignId is mandatory. Fetch the list of readers / non-readers for a particular email campaign. * clickers & nonClickers - emailCampaignId is mandatory. Fetch the list of clickers / non-clickers for a particular email campaign. * unsubscribed - emailCampaignId is mandatory. Fetch the list of all unsubscribed (blacklisted via any means) contacts for a particular email campaign. * hardBounces & softBounces - emailCampaignId is optional. Fetch the list of hard bounces / soft bounces for a particular / all email campaign(s). ")
public ActionForEmailCampaignsEnum getActionForEmailCampaigns() {
return actionForEmailCampaigns;
}
public void setActionForEmailCampaigns(ActionForEmailCampaignsEnum actionForEmailCampaigns) {
this.actionForEmailCampaigns = actionForEmailCampaigns;
}
public RequestContactExportCustomContactFilter actionForSmsCampaigns(ActionForSmsCampaignsEnum actionForSmsCampaigns) {
this.actionForSmsCampaigns = actionForSmsCampaigns;
return this;
}
/**
* Mandatory if neither actionForContacts nor actionForEmailCampaigns is passed. This will export the contacts on the basis of provided action applied on sms campaigns. * unsubscribed - Fetch the list of all unsubscribed (blacklisted via any means) contacts for all / particular sms campaigns. * hardBounces & softBounces - Fetch the list of hard bounces / soft bounces for all / particular sms campaigns.
* @return actionForSmsCampaigns
**/
@ApiModelProperty(value = "Mandatory if neither actionForContacts nor actionForEmailCampaigns is passed. This will export the contacts on the basis of provided action applied on sms campaigns. * unsubscribed - Fetch the list of all unsubscribed (blacklisted via any means) contacts for all / particular sms campaigns. * hardBounces & softBounces - Fetch the list of hard bounces / soft bounces for all / particular sms campaigns. ")
public ActionForSmsCampaignsEnum getActionForSmsCampaigns() {
return actionForSmsCampaigns;
}
public void setActionForSmsCampaigns(ActionForSmsCampaignsEnum actionForSmsCampaigns) {
this.actionForSmsCampaigns = actionForSmsCampaigns;
}
public RequestContactExportCustomContactFilter listId(Long listId) {
this.listId = listId;
return this;
}
/**
* Mandatory if actionForContacts is passed, ignored otherwise. Id of the list for which the corresponding action shall be applied in the filter.
* @return listId
**/
@ApiModelProperty(example = "2", value = "Mandatory if actionForContacts is passed, ignored otherwise. Id of the list for which the corresponding action shall be applied in the filter.")
public Long getListId() {
return listId;
}
public void setListId(Long listId) {
this.listId = listId;
}
public RequestContactExportCustomContactFilter emailCampaignId(Long emailCampaignId) {
this.emailCampaignId = emailCampaignId;
return this;
}
/**
* Considered only if actionForEmailCampaigns is passed, ignored otherwise. Mandatory if action is one of the following - openers, nonOpeners, clickers, nonClickers, unsubscribed. The id of the email campaign for which the corresponding action shall be applied in the filter.
* @return emailCampaignId
**/
@ApiModelProperty(example = "12", value = "Considered only if actionForEmailCampaigns is passed, ignored otherwise. Mandatory if action is one of the following - openers, nonOpeners, clickers, nonClickers, unsubscribed. The id of the email campaign for which the corresponding action shall be applied in the filter.")
public Long getEmailCampaignId() {
return emailCampaignId;
}
public void setEmailCampaignId(Long emailCampaignId) {
this.emailCampaignId = emailCampaignId;
}
public RequestContactExportCustomContactFilter smsCampaignId(Long smsCampaignId) {
this.smsCampaignId = smsCampaignId;
return this;
}
/**
* Considered only if actionForSmsCampaigns is passed, ignored otherwise. The id of sms campaign for which the corresponding action shall be applied in the filter.
* @return smsCampaignId
**/
@ApiModelProperty(example = "12", value = "Considered only if actionForSmsCampaigns is passed, ignored otherwise. The id of sms campaign for which the corresponding action shall be applied in the filter.")
public Long getSmsCampaignId() {
return smsCampaignId;
}
public void setSmsCampaignId(Long smsCampaignId) {
this.smsCampaignId = smsCampaignId;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
RequestContactExportCustomContactFilter requestContactExportCustomContactFilter = (RequestContactExportCustomContactFilter) o;
return ObjectUtils.equals(this.actionForContacts, requestContactExportCustomContactFilter.actionForContacts) &&
ObjectUtils.equals(this.actionForEmailCampaigns, requestContactExportCustomContactFilter.actionForEmailCampaigns) &&
ObjectUtils.equals(this.actionForSmsCampaigns, requestContactExportCustomContactFilter.actionForSmsCampaigns) &&
ObjectUtils.equals(this.listId, requestContactExportCustomContactFilter.listId) &&
ObjectUtils.equals(this.emailCampaignId, requestContactExportCustomContactFilter.emailCampaignId) &&
ObjectUtils.equals(this.smsCampaignId, requestContactExportCustomContactFilter.smsCampaignId);
}
@Override
public int hashCode() {
return ObjectUtils.hashCodeMulti(actionForContacts, actionForEmailCampaigns, actionForSmsCampaigns, listId, emailCampaignId, smsCampaignId);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class RequestContactExportCustomContactFilter {\n");
sb.append(" actionForContacts: ").append(toIndentedString(actionForContacts)).append("\n");
sb.append(" actionForEmailCampaigns: ").append(toIndentedString(actionForEmailCampaigns)).append("\n");
sb.append(" actionForSmsCampaigns: ").append(toIndentedString(actionForSmsCampaigns)).append("\n");
sb.append(" listId: ").append(toIndentedString(listId)).append("\n");
sb.append(" emailCampaignId: ").append(toIndentedString(emailCampaignId)).append("\n");
sb.append(" smsCampaignId: ").append(toIndentedString(smsCampaignId)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy