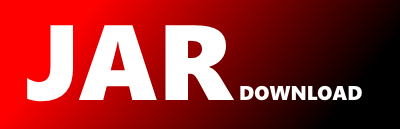
com.senzing.cmdline.RepeatedOptionException Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of senzing-commons Show documentation
Show all versions of senzing-commons Show documentation
Utility classes and functions common to multiple Senzing projects.
The newest version!
package com.senzing.cmdline;
import java.util.Set;
/**
* Thrown when a command-line option is illegally specified more than once.
*/
public class RepeatedOptionException extends CommandLineException {
/**
* The option that was repeated.
*/
private CommandLineOption option;
/**
* The flags used to specify the option.
*/
private Set flags;
/**
* Constructs with the {@link CommandLineOption} that was repeated and the
* flags used to specify it. This is constructed with a {@link Set} of
* flags that were used in case the same option can be specified with synonym
* flags.
*
* @param option The {@link CommandLineOption} that was not repeated.
* @param flags The {@link Set} of flags that were used to specify the option.
*/
public RepeatedOptionException(CommandLineOption option, Set flags)
{
super(buildErrorMessage(option, flags));
this.option = option;
this.flags = (flags == null) ? Set.of() : Set.copyOf(flags);
}
/**
* Return the {@link CommandLineOption} that was repeated.
*
* @return The {@link CommandLineOption} that was repeated.
*/
public CommandLineOption getOption() {
return this.option;
}
/**
* Returns the unmodifiable {@link Set} of command-line flags used to
* specify the repeated {@link CommandLineOption}.
*
* @return The unmodifiable {@link Set} of command-line flags used to
* specify the repeated {@link CommandLineOption}.
*/
public Set getFlags() {
return this.flags;
}
/**
* Builds the error message describing the repeated option.
*
* @param option The {@link CommandLineOption}.
*
* @param flags The {@link Set} of {@link String} command-line flags that
* were specified.
*
* @return The formatted error message.
*
* @throws NullPointerException If either of the specified parameters is
* null
.
*
* @throws IllegalArgumentException If the specified {@link Set} is empty.
*/
public static String buildErrorMessage(CommandLineOption option,
Set flags)
throws IllegalArgumentException
{
StringBuilder sb = new StringBuilder("Option specified more than once: ");
String prefix = "";
int count = 0;
for (String repeatedFlag: flags) {
sb.append(prefix).append(repeatedFlag);
count++;
prefix = (count == flags.size()) ? " and " : ", ";
}
return sb.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy