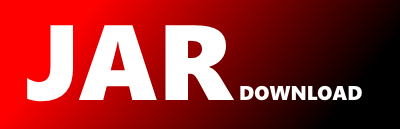
com.senzing.util.CollectionUtilities Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of senzing-commons Show documentation
Show all versions of senzing-commons Show documentation
Utility classes and functions common to multiple Senzing projects.
The newest version!
package com.senzing.util;
import java.util.*;
/**
* Provides utility functions for working with collections.
*/
@SuppressWarnings("unchecked")
public class CollectionUtilities {
/**
* Private default constructor.
*/
private CollectionUtilities() {
//
}
/**
* Returns a recursively unmodifiable view of the specified {@link List}.
* The behavior of this recursion depends on the values of the specified
* {@link List}:
*
* - {@link Set} - Uses {@link Collections#unmodifiableSet(Set)}
* to make the {@link Set} unmodifiable, but does not recurse further
* because the elements of a {@link Set} cannot be modified in place.
*
* - {@link List} - Recursively calls {@link
* #recursivelyUnmodifiableList(List)} on the {@link List} value to
* make it unmodifiable.
*
* - {@link Map} - Calls {@link #recursivelyUnmodifiableMap(Map)} on the
* {@link Map} value to make it unmodifiable.
*
* - All other values (including
null
values) are left as-is
*
*
* @param list The {@link List} to make recursively undmodifiable.
* @return The recursively unmodifiable view of the specified {@link List}.
* @param The type of element in the specified {@link List}.
*/
public static List recursivelyUnmodifiableList(List list) {
ListIterator iter = list.listIterator();
while (iter.hasNext()) {
T elem = iter.next();
if (elem instanceof Set) {
iter.set((T) Collections.unmodifiableSet((Set) elem));
} else if (elem instanceof List) {
iter.set((T) recursivelyUnmodifiableList((List) elem));
} else if (elem instanceof Map) {
iter.set((T) recursivelyUnmodifiableMap((Map) elem));
}
}
return Collections.unmodifiableList(list);
}
/**
* Returns a recursively unmodifiable view of the specified {@link Map}.
* The keys of the {@link Map} are left unmodifiable, but the behavior of this
* recursion depends on the values of the specified {@link Map}:
*
* - {@link Set} - Uses {@link Collections#unmodifiableSet(Set)}
* to make the {@link Set} unmodifiable, but does not recurse further
* because the elements of a {@link Set} cannot be modified in place.
*
* - {@link List} - Calls {@link #recursivelyUnmodifiableList(List)} on
* the {@link List} value to make it unmodifiable.
*
* - {@link Map} - Recursively calls {@link
* #recursivelyUnmodifiableMap(Map)} on the {@link Map} value to make
* it unmodifiable.
*
* - All other values (including
null
values) are left as-is
*
*
* @param map The {@link Map} to make recursively undmodifiable.
* @return The recursively unmodifiable view of the specified {@link List}.
* @param The type of keys in the specified {@link Map}.
* @param The type of values in the specified {@link Map}.
*/
public static Map recursivelyUnmodifiableMap(Map map) {
Iterator> iter = map.entrySet().iterator();
while (iter.hasNext()) {
Map.Entry entry = iter.next();
V value = entry.getValue();
if (value != null) {
if (value instanceof Set) {
entry.setValue((V) Collections.unmodifiableSet((Set) value));
} else if (value instanceof List) {
entry.setValue((V) recursivelyUnmodifiableList((List) value));
} else if (value instanceof Map) {
entry.setValue((V) recursivelyUnmodifiableMap((Map) value));
}
}
}
return Collections.unmodifiableMap(map);
}
/**
* Utility method for creating a modifiable {@link List} containing the
* specified elements.
*
* @param elements The zero or more elements in the list.
*
* @return The {@link Set} of elements.
* @param The type of elements for the {@link List}.
*/
public static List list(T... elements) {
List list = new ArrayList<>(elements.length);
for (T element : elements) {
list.add(element);
}
return list;
}
/**
* Utility method for creating a modifiable {@link Set} containing the
* specified elements.
*
* @param elements The zero or more elements in the set.
*
* @return The {@link Set} of elements.
* @param The type of elements for the {@link Set}.
*/
public static Set set(T... elements) {
Set set = new LinkedHashSet<>();
for (T element : elements) {
set.add(element);
}
return set;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy